What is equals() Method in Java?
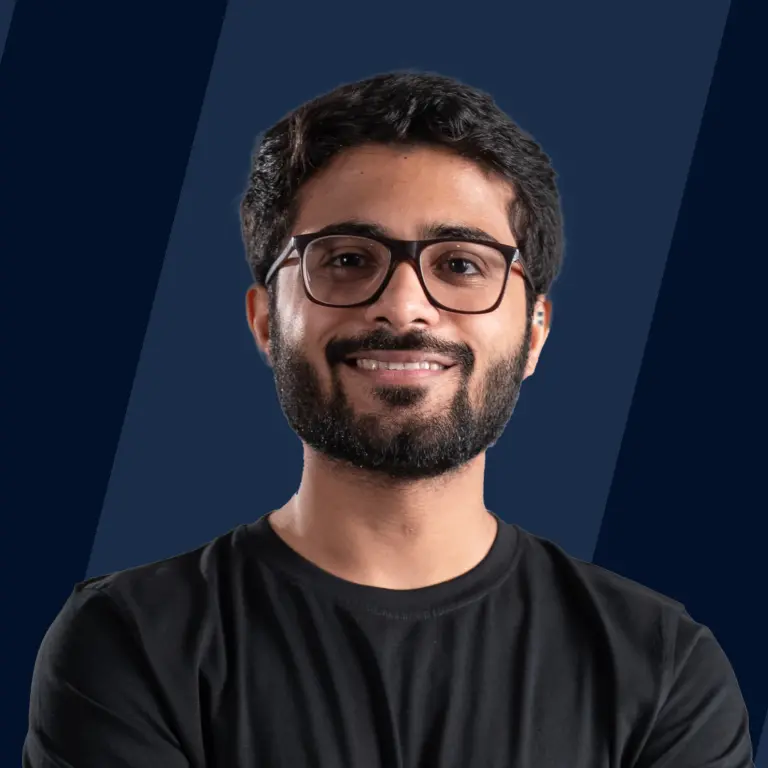
In Java, the "==" operator and the equals() method are used to test equality between two variables. While "==" compares object memory locations, equals(), defined in the Object class, can be customized for comparing object contents. For instance, in a student database, these tools can identify students with over 90% scores or those enrolled in a Computer Science course.
What is the equals() Method in Java?
When we want to compare two objects based on some logic, we need to override the equals() method in the corresponding class of those objects. Thus equals() methods compare two entities and return true if they are logically the same. Since equals() is a method defined in the Object class thus the default implementation of the equals() method compares the object references or the memory location where the objects are stored in the heap. Thus by default the equals() method checks the object by using the “==” operator.
Also, we need to override the hashCode() method if we are overriding the equals() method to avoid breaking the contract. If two objects when compared with the equals() method are equal then their hashCode must also be the same.
Default implementation of .equals() method defined in Object class
Let's see via an example by creating a Student class.
Now, let's define three students who have enrolled in some of the available courses.
Now if you want to find out all those students who have enrolled in Computer Science course, you would use equals() method like below.
Similarly you would compare other student's courseName. Why we are using equals() here? Because getCourseName() method returns a String object and equals() method of String class is overriden to compare the contents of the string and not object location as shown below.
Internal Implementation:
Let's see another example where we want to know if two Student objects are same or not. Let's see how we will override equals() method for Student class. The unique key to identify a student is enrollment number. So, two students are equal if they have same value of enrollmentNumber variable. Let's define three students as following:
Here, secondStudent and thirdStudent variables are equal because they have same enrollment number. Hence when we compare these two objects with equals() method, it should return true logically.
Let's see how will we override equals() method and hashCode() methods to achieve the same.
Override equals() method:
Explanation:
- According to the implementation of hashCode() method, hashcode of all the three objects - firstStudent, secondStudent as well as thirdStudent comes out to be 5.
- But first student is not same as second and third students as enrollmentNumber of the first student is different from both other objects.
- According to the implementation of equals() method, secondStudent and thirdStudent objects are equal. This implies they should have same hashcode but vice-versa is not true.
Hashcode of two objects must be same if both objects are equal. However, if two objects have same hashcode, it does not imply those objects are equal.
What is the "==" operator in Java?
“==” operator is a binary equality operator used to compare the primitive data types in Java (like int, short, long, char, boolean, double, float and byte). It also compares the memory location or the references of the two objects stored in the heap memory.
Now if you have the same three students enrolled into the course as follows:
We would use == operator as mentioned below to check if some student have got 100% score
Interesting Example
Let's see another example, if we want to see that the second student and third student are enrolled in the same course, then == operator will not give the desired result.
Output:
Explanation:
- This returns false as here the contents are not getting compared but the memory locations where these two String objects are stored are getting compared.
- Since, these are two different String objects stored at different memory locations in the heap, hence it returns false.
Example of "==" vs equals() Method
Let's see how a "==" operator is used.
Code
Output
Explanation:
- firstStudent and secondStudent point to Student objects stored in different memory locations.
- firstStudent and firstStudentCopy point to the same Student object.
- Both strings are not String objects but interned strings stored in a special memory space called String Pool. Strings with same value created using literals are stored in the String Pool at the same location. Read more.
- Primitive variables are compared by their value using "==" operator by default.
Let's see how an equals() method is used
Code
Output
Explanation:
- ".equals()" operator is not overridden in the code above, hence the default implementation provided in Object class is used.
- According to the default implementation, if the reference variables point to the same object, output is true.
- Primitive variables are compared by their value not memory location.
Difference between "==" and equals() Method in Java
- == operator is a binary equality operator whereas equals() is a method defined in the Object class.
- == operator compares the memory locations of two objects and returns a boolean value whereas equals() is used to compare the two objects by some business logic which can be defined.
- Use == operator when you want to compare the values of two primitive data types or you want to compare two references are same or not. Use equals() method when you want to compare two objects by functionality.
- == operator cannot be overridden in Java. However, equals() method can be overridden in a class. By default, the equals() method uses the == operator.
Comparing two objects with "==" and equals() method
Here, we are not overriding the equals() method. Hence, equals() method will use the default implementation in Object class to compare the object references.
Using Default Implementation of .equals() method
Code
Output
Explanation:
- In the first comparison, we are comparing the memory locations using the equals() method, hence false.
- Similar to the first comparison, but this time with == operator.
- In the final comparison, we are comparing two objects pointing to the same Student object.
Overriding equals() Method in Student Class
In this code, we are overriding the .equals() method by specifying that two students shall be equal when they have same name and enrollment number. Hence we are writing some business logic by which our objects will be comparable.
Code
Output
Explanation:
firstStudent and secondStudent have different enrollmentNumber, hence false according to the implementation of equals() method. Similar logic is used for other comparisons as well. Use this Java Online Compiler to compile your code.
Conclusion
- Use the “==” operator to compare the references of two objects.
- Use the equals() method to compare the values of the two objects by a business logic or functionality.
- equals() method has default implementation provided in Object class and compares the memory location of two objects if it's not overridden.
- Remember to override hashcode() method defined in the Object class as well if you are overriding the equals() method. As if two objects are logically same then their hashCode should also be same whereas the vice-versa is not true.