Exit Method in Java
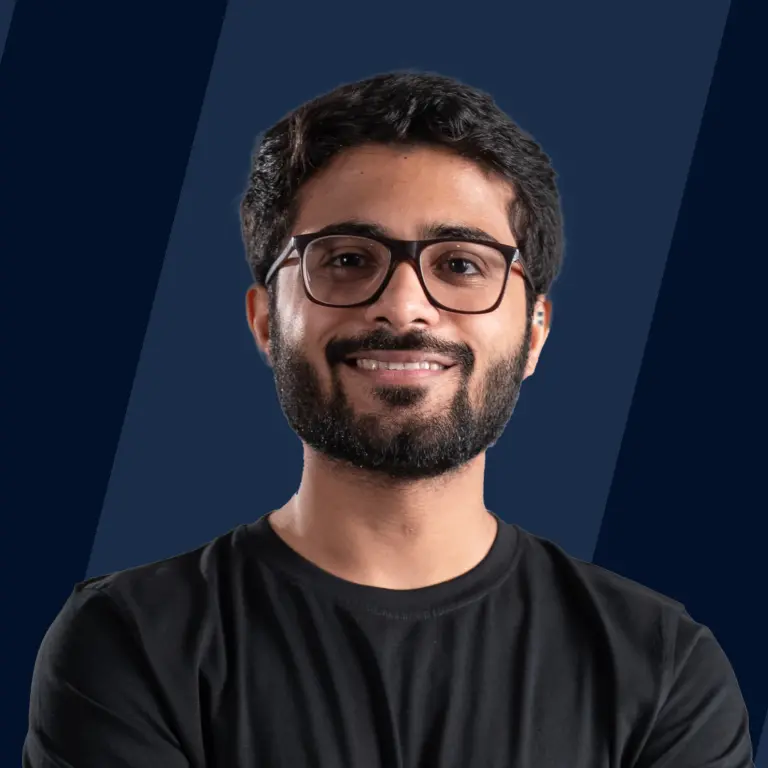
Renowned for its versatility, Java includes essential control mechanisms like the System.exit() method for terminating a Java Virtual Machine (JVM) instance. Unlike other jump statements (break, continue, return), System.exit() offers a direct way to halt a program. This article explores how to effectively use System.exit() in Java, complemented by examples and a look into label-based control flow management, ensuring you can easily navigate through Java's program control options.
What is exit() Method in Java?
Java provides exit() method to exit the program at any point by terminating running JVM, based on some condition or programming logic. In Java exit() method is in java.lang.System class. This System.exit() method terminates the current JVM running on the system which results in termination of code being executed currently. This method takes status code as an argument.
Uses
- exit() method is required when there is an abnormal condition and we need to terminate the program immediately.
- exit() method can be used instead of throwing an exception and providing the user a script that mentions what caused the program to exit abruptly.
- Another use-case for exit() method in java, is if a programmer wants to terminate the execution of the program in case of wrong inputs.
Syntax of exit() in Java
Parameters of exit() in jJava
System.exit() method takes status as a parameter, these status codes tell about the status of termination. This status code is an integer value and its meanings are as follows:
- exit(0) - Indicates successful termination
- exit(1) - Indicates unsuccessful termination
- exit(-1) - Indicates unsuccessful termination with Exception
Note: Any non-zero value as status code indicates unsuccessful termination.
Return Value for exit() in Java
As the syntax suggests, it is a void method that does not return any value.
Exit a Java Method using System.exit(0)
As mentioned earlier, System.exit(0) method terminates JVM which results in termination of the currently running program too. Status is the single parameter that the method takes. If the status is 0, it indicates the termination is successful.
Let's see practical implementations of System.exit(0) method in Java.
Example 1
Here is a simple program that uses System.exit(0) method to terminate the program based on the condition.
Output:
Explanation:
In the above program, an array is being accessed till its index is 4. Until if condition is false i.e., array index <= 4, JVM will give Array index and element stored at that index as the output.
Once the if condition is true i.e., array index > 4, JVM will execute statements inside the if condition. Here it will first execute the print statement and when exit(0) method is called it will terminate JVM and program execution.
Example 2
Let's see one more example of exit() method in a try-catch-finally block.
Output:
Explanation:
The piece of code above is trying to read a file and print a line from it if the file exists. If a file doesn't exist, the exception is raised and execution will go in catch block and code exits with System.exit(0) method in the catch block.
- We know, finally block is executed every time irrespective of try-catch blocks are being executed or not. But, in this case, the file is not present so JVM will check the catch block where System.exit(0) method is used that terminates JVM and currently running the program.
- As the JVM is terminated in the catch block, JVM won't be able to read the final block hence, the final block will not be executed.
Exit a Java Method using Return
exit() method in java is the simplest way to terminate the program in abnormal conditions. There is one more way to exit the java program using return keyword. return keyword completes execution of the method when used and returns the value from the function. The return keyword can be used to exit any method when it doesn't return any value.
Let's see this using an example:
Output:
Explanation:
In the above program, SubMethod takes two arguments num1 and num2, subtracts num2 from num1 giving the result in the answer variable. But it has one condition that states num2 should not be greater than num1, this condition is implemented using if conditional block.
If this condition is true, it will execute if block which has a return statement. In this case, SubMethod is of void type hence, return doesn't return any value and in this way exits the function.
Does goto Exist in Java?
No, goto does not exist in Java. Because Java has reserved it for now and may use it in a later version.
In other languages, goto is a control statement used to transfer control to a certain point in the programming. After goto statement is executed program starts executing the lines where goto has transferred its control and then other lines are executed. goto statement works with labels that are identifiers, these labels are used to the state where goto has to transfer the control of execution.
Java does not support goto() method but it supports label. No support for goto method is due to the following reasons:
- Goto-ridden code hard to understand and hard to maintain
- These labels can be used with nested loops. A combination of break, continue and labels can be used to achieve the functionality of goto() method in Java.
- Prohibits compiler optimization
Example:
Output:
In the above code, the outer label is created for the first for loop. When if the condition is true break outer; statement will go back to first for loop and break the execution. Use this Compiler to compile your Java code.
Conclusion
- exit() method is used to terminate JVM.
- Status code other than 0 in exit() method indicates abnormal termination of code.
- goto does not exist in Java, but it supports labels.
- It is better to use exception handling or plain return statements to exit a program while execution.
- System.exit() method suit better for script-based applications or wherever the status codes are interpreted.