final Keyword in Java
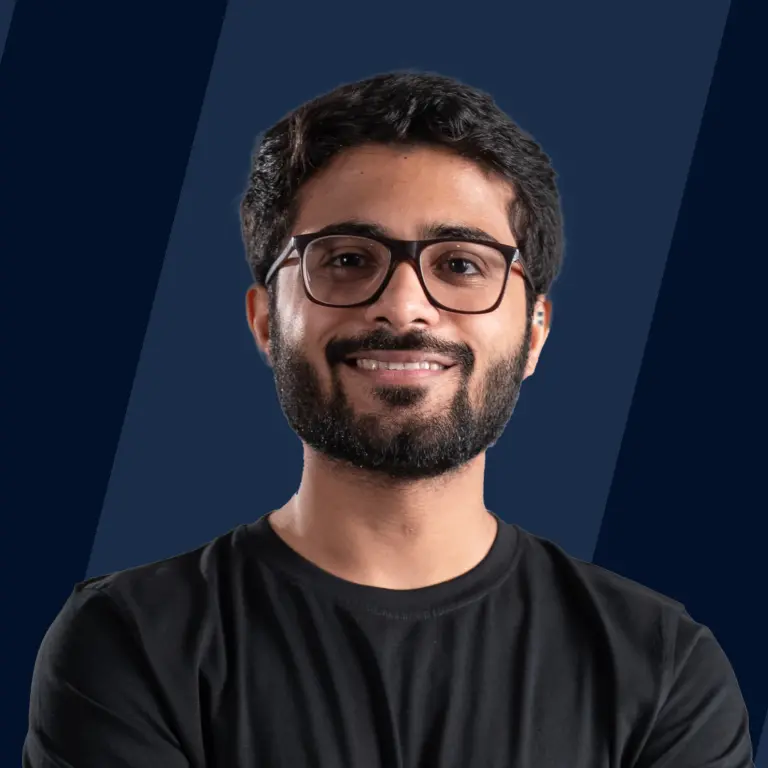
In Java, the final keyword is a non-access modifier that is used to define entities that cannot be changed or modified.
Consider the below table to understand where we can use the final keyword:
Type | Description |
---|---|
Final Variable | Variable with final keyword cannot be assigned again |
Final Method | Method with final keyword cannot be overridden by its subclasses |
Final Class | Class with final keywords cannot be extended or inherited from other classes |
Characteristics of final keyword in Java
- Creates constants (immutable variables).
- Prevents method overriding.
- Prohibits subclassing.
Final Variable in Java
Programmers can create a final variable when they need to create a constant variable, which means after initializing it cannot be modified or updated.
If we try to modify this variable, the compiler will throw an error. The same way they can do with objects but, in this case we can change the property of objects as we only finalize the reference variable of the object.
Final variables can be used with primitive data types (int, float, double, and char) and non-primitive data types (object references).
Syntax
Initializing Final Variable in Java
Let's understand it more clearly with a Java program.
Output -
In the above code, as you can see we have declared the integer variable called count as final. After which we were assigning the final variable again with value 15. As a result, we are facing the compilation error by saying that the final variable cannot be re-assigned.
Java Final Variable
Let's understand the use of final variable in Java through this short piece of code:
Blank Final Variable
When using the final keyword, we can declare a blank-final variable to assign its value after some lines of execution. It is recommended, though not mandatory, for good programmers to initialize the value at the time of declaration.
Syntax -
Let's understand it more clearly with a Java program.
Output -
In the above code, as you can see we have declared the integer variable called count as final. But, instead of initializing it while declaring, we initialize it later and it works completely fine. However, re-assigning it after this step will throw a compilation error.
Final Reference Variable
Final variables referencing objects cannot be reassigned to point to another object, but you can modify the properties of the object they reference. This applies to class objects, arrays, and similar data structures.
Syntax -
Example -
Let's understand it more clearly with a Java program.
Output -
In the above code, as you can see we have declared and initialized final reference variable of type StringBuffer with value "Hellow". When we tried to add new string "World" in StringBuffer reference variable, it works fine as we did not change the reference variable but we changed only object's property.
Final Fields
In Java programming language, a field is a variable inside a class, which can be declared as final. The final keyword ensures that once a field is declared as final, its value cannot be changed, making it a "write-once field." When a field is declared as static and final, it is referred to as a "constant."
After declaring a field as final, its value cannot be modified, even from another class. Due to this immutability, it is sensible to declare the field as static since its value will remain constant throughout the program's execution.
Example -
Where to initialize -
-
We can initialize static final fields at -
- while declaration
- Inside the static initializer block
-
We can initialize instance final fields -
- while declaration
- Inside the instance initializer block
- Inside the constructor
The final field initialization apart from the above-mentioned places causes compile-time error.
When to use Java Final Variable?
- While creating constant for a program we can use the Final variable (Most of the programmers highly recommended writing the final keyword in uppercase only)
- To restrict the class variable value to update or modify in sub-class.
- To fix some mathematical constant i.e. final double PI = 3.141592653589793;
Convention:
According to the Java Code Convention, all the declared final variables are treated as a constant in Java program, which is advised to be written in all caps (Uppercase).
Final Method in Java
In Java, the final keyword can be used with methods to prevent them from being overridden by subclasses. It enforces method immutability. There is no significant difference in performance speed between final and non-final methods.
Syntax -
Let's understand it more clearly with a Java program.
Output -
In the above code, we have declared two classes Scaler and InterviewBit. Class InterviewBit inherited class Scaler, and so it also tried to override the final method of class Scaler. As the final method cannot be overridden, the program throws the compilation time error by saying - display() in InterveiwBit cannot override display() in Scaler.
Purpose of Java Final Methods
Final methods in Java are used to prevent unexpected behavior in child classes by disallowing the addition or modification of methods that could disrupt the class's consistency.
Using final methods is preferred when there's a need to maintain the integrity of the class. For example, the Thread class in Java has a final method isAlive(), which cannot be overridden by subclasses. This ensures that the behavior of the method remains consistent across all thread instances.
Let's understand it from a Java program.
Output -
As you can see, there are two methods in class Calculation - premiumCalculation and fixedCalculation. Here, we defined fixedCalculation as a final method so no other can override the logic of this class. On the other hand, premiumCalculation can be overriden.
Final Class in Java
Likewise with the final variables and final methods, we can also use the final keyword with Java classes. These classes are called the final classes, which can not be inherited by any other classes.
So, if we try to inherit a class which is declared as a final, the system will throw a compile time error.
Classes like Integer, String, and many other wrapper classes are an example of Final classes.
Syntax -
Example -
Let's understand it more clearly with a Java program.
Output -
Note:
Declaring class with final keyword doesn't mean we can not change/modify the object of that class. We just can not inherit it.
Advantages and Disadvantages of Final variable in java
Advantages
- Final variable improves performance during runtime. In JVM the compiled code can improve the performance at a runtime, and for the JVM final variables are compiled code.
- Final variable can be stored in cache, not just by JVM but also by the application.
- Final keyword is needed to implement an immutable class.
Disadvantages
- Final variable works seamlessly only with primitive or immutable type variables. Moreover, it doesn't give you a guarantee that you can not modify the state/value of a variable (Non-primitive). It just does not allow us to reassign the reference to any other objects after initialization.
For instance -
That means in many cases, final variable still doesn't make it easier for coders to know what exactly is happening inside the code. Which may lead to misunderstanding and bugs in the application.
Note:
All the declared variables in the Java interface are inherently final.
Conclusion
- The final keyword is used with local variables, member variables, methods, and classes in Java.
- It can be applied to both primitive and non-primitive data type variables.
- final prevents changing the value of a variable, method overriding, and class inheritance.
- Final methods maintain their functionality, unchangeable by subclasses.
- A blank final variable must be initialized during declaration or inside the constructor.
- Final reference variables cannot change, but the properties of the object they refer to can.
- A final class cannot be inherited, but its object properties can still be modified.
- Final method arguments cannot be changed within the method body.