Heap Memory and Stack Memory in Java
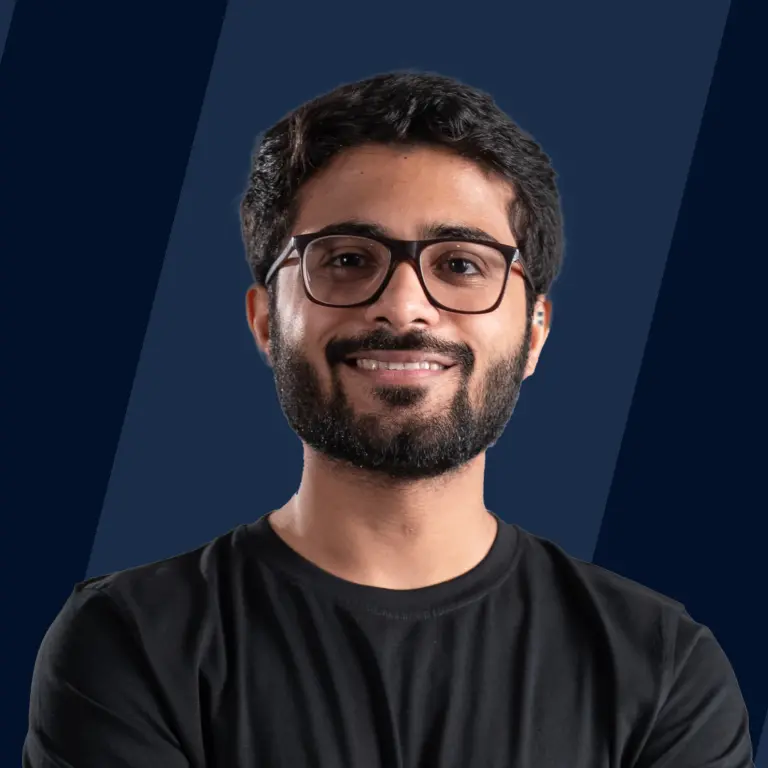
In Java, Stack and Heap memory are crucial aspects the Java Virtual Machine (JVM) allocates. This article delves into their roles and functionalities.
Stack Memory employs a Static Memory Allocation Scheme, storing function calls, method-specific primitive data, and object references. Access is in the Last-In-First-Out (LIFO) order.
Heap Memory facilitates Dynamic Memory Allocation for Java objects and JRE classes during program execution. Objects in the heap have global access, available from any part of the application.
Stack Allocation in Java
The Stack Memory utilizes Static Memory Allocation, storing function calls, method-specific data, and object references. It has a fixed size that doesn't change during execution. The stack memory, a segment in the RAM, is dynamically allocated to each thread during runtime. This allocation occurs upon thread creation, following a Last-In-First-Out (LIFO) order due to its global accessibility. The lifespan of the memory allocated to the stack is confined to the duration of the function, and it ceases to exist once the function concludes.
Example:
Consider a Java program with methods main, addOne, and addTwo. The stack usage is explained step by step.
Output:
Explanation:
The main method starts executing, and local variables a and b are stored in the stack memory. The addNumbers method is called, and local variables x, y, and result are stored in the stack memory for the duration of the method execution.
Stack OverflowError in Java
In cases where there's insufficient space to create new objects, the system may raise a java.lang.StackOverFlowError. Notably, the elements within the stack memory have a scope limited to their respective threads. Each thread in the Java Virtual Machine (JVM) has a dedicated stack. An example demonstrates how this error occurs.
Explanation: The main method is called recursiveMethod with an initial counter value of 1. The recursiveMethod prints the current counter value and then calls itself with an incremented counter. This process continues indefinitely, leading to an infinite recursion. Eventually, the stack memory is exhausted, resulting in a StackOverflowError.
Heap Allocation in Java
Heap memory is established during the Java Virtual Machine (JVM) initiation and remains in use throughout the application's runtime. It is the storage ground for objects and Java Runtime Environment (JRE) classes. When objects are created, they claim space in the heap memory, with their references stored in the stack.
Differing from the stack's orderly Last-In-First-Out (LIFO) approach, heap memory operates dynamically, handling memory blocks without adhering to a specific order. In Java, memory management is automated, thanks to the garbage collector, which is responsible for deallocating objects no longer in use.
Heap memory persists until one of two events occurs: the program terminates, or a memory-freeing event occurs. The elements within heap memory are globally accessible throughout the application and are shared among all threads.
The heap memory is further categorized into distinct memory areas: the Young generation, Survivor space, Old generation, Permanent generation, and Code Cache.
Examples:
An example illustrates the allocation of a variable in the stack and an object in the heap.
Output:
Explanation: In the above program, Objects of the Person class are created using the new keyword and stored in the heap memory.
Why is OutOfMemoryError thrown in Java?
OutOfMemoryError occurs when there's insufficient space in the heap to create and store new objects, or if the heap space reaches total capacity, the system raises java.lang. OutOfMemoryError. An example showcases this error.
Explanation:
A List of Objects is continuously populated in a while loop. The loop runs indefinitely, attempting to add new objects to the list. Eventually, the heap space is exhausted, leading to an OutOfMemoryError.
Mixed Example of Both Memory Allocation
An example demonstrates a Java program's simultaneous usage of stack and heap memory.
Output:
Key Difference Between Heap and Stack Memory in Java
In the realm of memory management, distinctions arise between stack and heap allocation:
- Automatic Management:
- Stack: Compiler automates both allocation and de-allocation of memory.
- Heap: Manual intervention by the programmer is required for memory management.
- Cost of Handling Frames:
- Stack: More efficient and less resource-intensive.
- Heap: Handling heap frames incurs higher costs.
- Memory Shortage and Issues:
- Stack: Prone to memory shortage issues.
- Heap: Faces challenges primarily related to fragmentation.
- Frame Access and Cache Considerations:
- Stack: Easier frame access is cache-friendly due to a compact memory region.
- Heap: Frame access is more complex as frames are dispersed, increasing cache misses.
- Flexibility of Memory Size:
- Stack: Inflexible, memory size is fixed.
- Heap: Flexible, allowing for dynamic adjustments to the allotted memory.
- Access Time:
- Stack: Faster access time.
- Heap: Slower access time compared to the stack.
Heap Vs Stack Memory Allocation in Java: Comparative Analysis
A tabular overview comparing various aspects of Heap and Stack memory in Java is given below-.
Property | Stack Memory | Heap Memory |
---|---|---|
Size | Smaller, fixed size | Larger, dynamic size |
Order | LIFO | No specific order |
Speed | Faster access | Slower access |
Resizing | Not allowed | Allowed |
Allocation | Automatic for method scope | Manual for objects, GC reclaim |
Storage | Method-specific and references | Objects and JRE classes |
Exception | StackOverflowError | OutOfMemoryError |
Thread Safety | Thread-specific, thread-safe | Shared, not thread-safe |
Scope | Method-specific | Global |
Lifetime | Short-lived | From start to end of execution |
Conclusion
- The Stack Memory utilizes Static Memory Allocation, storing function calls, method-specific data, and object references.
- Stack follows LIFO Order.
- Heap is the storage ground for objects and Java Runtime Environment (JRE) classes.
- Heap follow FIFO Order.