How to Read a File in Java
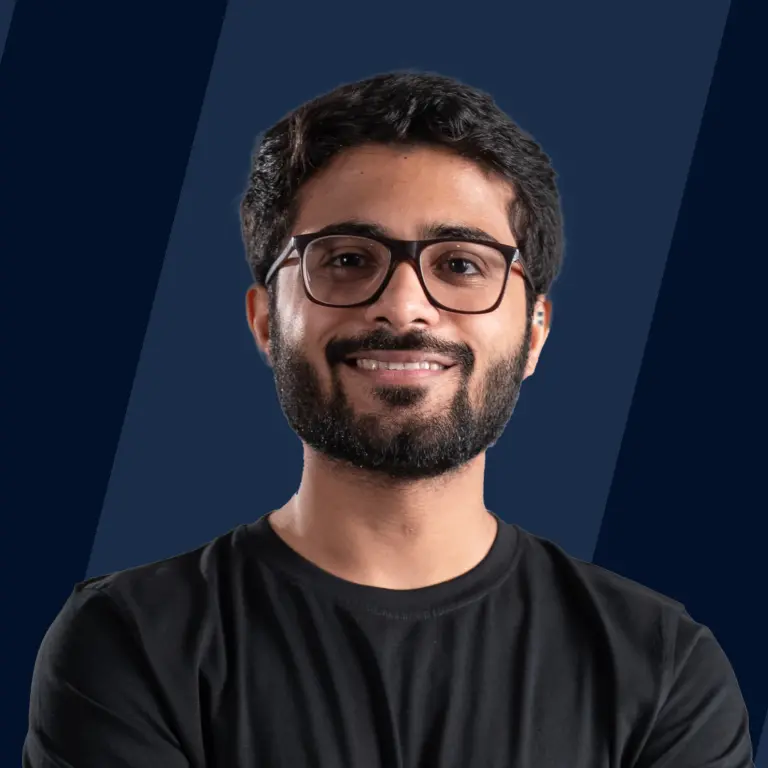
Mastering how to read a file in Java is crucial for accessing the plethora of data stored on computers, from application data to multimedia. Java offers versatile tools like FileReader and BufferedReader for efficient file access, essential for manipulating and managing diverse data types within Java applications.
Different Ways of Reading a Text File in Java
Understanding how to read a file in Java is essential for a wide range of applications, from processing large datasets to parsing configuration files. Java provides several methods for this purpose, including:
- Using Scanner class
- Using BufferedReader class
- Using File Reader class
- Using Files Class
Now, let's delve deeper into each method, implementing them through clean Java programs for comprehensive understanding.
Reading a File in Java Using Scanner Class
After discussing the BufferedReader method, and saying it is the fastest why do we need Scanner?
The Scanner class has more useful methods like next(), nextInt(), nextByte(), nextLine() etc, and we can assign any delimiter to divide the string rather than the default space. Since the Scanner class also reads the data from the stream Line by Line, we can use large text files to read.
Syntax:
Explanation:
We are creating a Scanner object, and then we can use the methods of Scanner class on it.
Code:
Output:
Remember: Closing the file after reading is crucial to free up system resources.
Reading a File in Java Using BufferedReader Class
When it comes to how to read a file in Java efficiently, especially large files, BufferedReader is often the go-to choice due to its buffering capability, which minimizes I/O operations.The BufferedReader class was there in Java from the start and is the fastest method to read the data, from a given file, Line by Line. It is used to read the data using the input stream. As the name suggests, the BufferedReader class buffers the data in the form of small packets of size 8 KB. Since it is only processing the data of 8 KB at a particular time, it is very efficient and can be used for large files.
Syntax:
Explanation:
We are creating a BufferedReader object, and then we can use the methods of BufferedReader class on it.
Code:
Since readLine() returns null as we reach the end, we can use it as the stopping condition.
Output:
Reading a File in Java Using FileReader Class
FileReader is a convenient class for reading character files, making it a straightforward choice for how to read a file in Java, especially when working with text files. This class is crafted with default assumptions on character encoding and buffer size, streamlining the file reading process.
Key Constructors:
- FileReader(File file): Initiates a new FileReader instance, with the specified File object for reading.
- FileReader(FileDescriptor fd): Constructs a FileReader object, utilizing the provided FileDescriptor for reading operations.
- FileReader(String fileName): Creates a FileReader object, taking the file name as input for reading.
Example Implementation:
Output:
To explore further coding examples, visit Scaler.
Reading a File in Java Using Files Class
In Java, the java.nio.file.Files class provides a straightforward approach to reading text files. This class offers methods to efficiently read the entire content of a file into a byte array or to read all lines into a list of strings. Introduced in Java 7, the Files class is particularly useful for loading complete file contents into memory. However, it's advisable to utilize this approach only for small files due to memory considerations.
Example Usage:
This concise approach using the Files class simplifies the process of reading text files in Java, providing flexibility and efficiency.
Conclusion
- Understanding how to read a file in Java is vital, as Java streams, akin to a continuous flow of data, facilitate efficient data transportation without storing elements, enhancing program efficiency.
- The java.io.File class represents file paths, while java.io.FileNotFoundException handles file access errors, crucial for robust Java file operations when learning how to read files in Java.
- Various methods such as Scanner, BufferedReader, and FileReader exist for reading text files in Java, each with distinct advantages and suitability for different tasks, highlighting the importance of mastering how to read a file in Java.
- The Scanner class offers flexibility with methods like next() and nextLine(), making it useful for extracting data line by line, particularly beneficial for handling large files, and is a key technique in learning how to read a file in java.
- BufferedReader stands out for its efficiency in reading large text files line by line, thanks to its buffering mechanism, which enhances performance in file processing tasks, an essential aspect of how to read file in Java.