Iterable Interface in Java
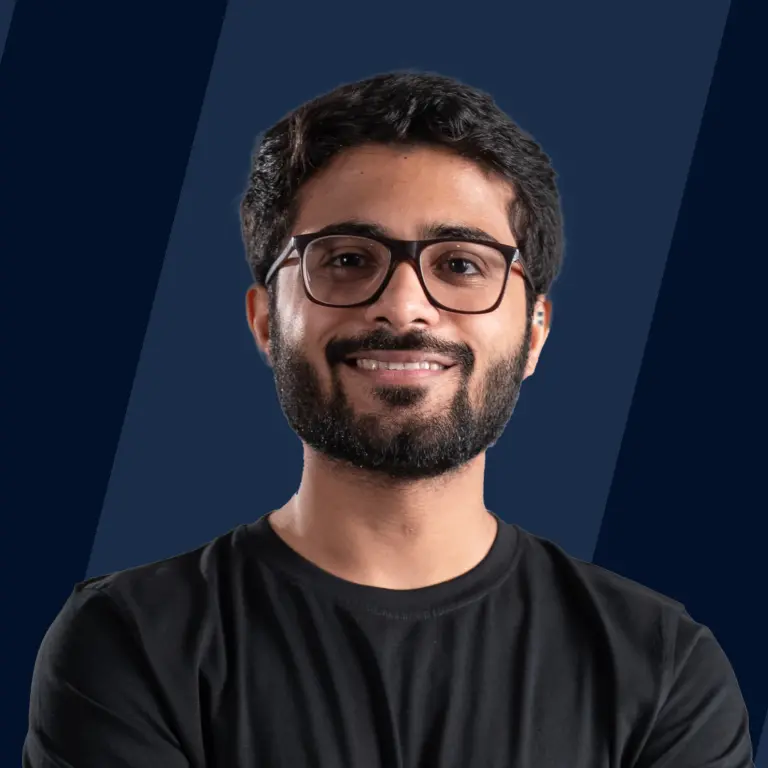
Overview
The Iterable interface is present in java.lang.Iterable package. It was introduced in JDK 1.5. It allows users to iterate through elements sequentially from a collection. It returns each element of the collection one after the other, beginning from the front, moving in a forward direction. There are three ways in which elements can be iterated in Java, using enhanced for loop, forEach() method and by calling iterator() method. The Collection interface extends the Iterable interface thus, all the classes implementing the Collection interface are iterable.
Introduction to Iterable in Java
Iterable in Java is an interface that provides the functionality of accessing elements of a collection one by one. ELements of collections like arrays, sets, queues, maps, etc. can be transversed easily using Iterable.
The Collection framework extends the Iterable interface, thus all the classes implementing the collections framework also implement the Iterable interface. Thus, objects of these classes can use the Iterable feature of Java.
By using Iterator we can access each item in the collection, one item at a time. Here, we have an array of 5 elements, iterator named iter of this array returns each element of the array one-by-one starting from 0^th^ index.
Iterator
Iterator and Iterable both interfaces sound similar and are often confusing. Any class that implements an Iterable interface, overrides the iterator() method present in the Iterable interface. This iterator() method calls an Iterator interface which then returns an iterator. This iterator is used to iterate over an object of that class. The Iterator interface has four methods namely next(), hasNext(), remove() and forEachRemaining().
Syntax to Implement Iterable
Import java.lang.Iterable package to access Iterable.
Declaration of an Iterator
We create an object of Iterator named iter. iter will return data of the specified type in Angular Brackets (<>). If not mentioned, it returns an object.
Initialization of an Iterator
Obj is a name of a collection like Stack, Queue, HashMap, etc. And DataType is the type of data filled in the DataStructure such as String, Integer, etc. if we don't mention DataType in Iterator it will return an Object.
For example:
In the above example, Queue named myQueue is created which is going to store String in it. We create an Iterator named iter that returns String. Queue class Overrides iterator method from Iterable Interface, that we are calling on myQueue an Object of Queue Class.
How Java Iterable Works?
Collection can be array, list, set, etc. When collection calls the Iterable interface, it calls the iterator() method. This method returns the Iterator of a specified type. Now we check the Iterator if it has any elements using the hasNext() method, if it returns true we call the next() method to retrieve the element else we terminate the loop. Let us take an example to understand the working of Iterator.
We create an object of Iterator on Stack, check if the Stack has elements that are not yet visited, using the hasNext() method. If it returns true, we call next() and retrieve that element. If it returns false implies we visited all the elements of the Stack or Stack is empty. Thus, we exit from the while loop.
Output:
Objects of Iterable can be Iterated in three ways:
- Enhanced for loop(for-each loop)
- Iterable forEach loop
- Iterator<T> interface
Iterate an Iterable With the for-each Loop
The first way to iterate the elements of a Java Iterable is using Enhanced for loop. It is also known as for-each loop.
Objects of Classes implementing Collection Interface can be transversed using the for-each loop as Collection interface extends Iterable interface. Elements of the collection can only be read. They cannot be removed, updated, or added to the collection. Index of the element also cannot be accessed. We cannot skip any index or visit alternate positions.
Syntax for enhanced for loop:
Where DataType is DataType of the variable_name, type of data stored in the Collection. CollectionName is the name of the Collection to be iterated.
Example:
Output:
We create a 2D array with rows and columns. Elements of this array are retrieved using nested for each loop. Array, Stack, Queue, Map, Set, etc. can be iterated using for-each loop.
Iterate an Iterable via its forEach() Method
The forEach() method uses Lamda Expression as a parameter. This Expression is called for each element of the Collection. forEach() method cannot be used to update, add or remove elements of the collection. We can read elements of the collection and print them.
Syntax for forEach() Method
Collection_name is the name given to any kind of collection, variable_name is the name to be given to the element inside the collection. -> is known as Lamda Expression.
Example
Output:
Printing even numbers from a stream of numbers. filter checks if the number is even. If the number is even then action is performed on the number.
Iterate an Iterable via an Iterator
Elements of java Iterable can be iterated by calling the iterator() method that returns Iterator.
Iterator Interface in Java has 4 methods:
- next() - It returns the next item in the collection. If all the elements of the collection are transversed or the collection is empty, it throws a NoSuchElementException.
- hasNext() - it returns a boolean. If all the items of the collection are covered then it returns false, else returns true.
- remove() - This method removes the last element returned by the next() method. If the next() method is not called then it throws an IllegalStateException. If the iterator doesn't support remove operation for that collection, then it throws the UnSupportedOperationException. remove() method must be called only once, for one next() call.
- forEachRemaining(CakeShop<String> action) - It performs the given action for all the remaining elements in the collection, in sequential (one by one) method.
Syntax:
Declaration of an Iterator
An iter is an object of Iterator, it returns element of the specified DataType in the Angular brackets.
Initialization of an Iterator
Obj is the collection name. Obj class overrides iterator method to Iterable Interface. This method is called to create an iterator of Obj.
Example:
Output:
Student data is stored in a HashMap named Student. This data is printed using the iterator() method.
Iterable Interface Definition
The Iterable interface in Java has three methods, out of which only the iterator() method needs to be implemented. The other two have default implementations. The definition of Java Iterable Interface is given below:
Note: T in the above interface refers to the type of element returned by the Iterator.
Methods of Iterable
Iterable has 3 methods:
- void forEach(Consumer<? super T> action) - It acts as each item of the Iterable until all the items are processed or the method throws an exception.
- Iterator<T> iterator() - It calls the Iterator Interface. Iterator interface returns an iterator, which is used to iterate the elements in the container and perform some action on it.
- Spliterator<T> spliterator() - It creates a Spliterator over the items described by the Iterable. Spliterator is an object for traversing and partitioning the elements of a collection, where a collection can be array, set, tree, or generator function. Spliterator can also be known as a splittable iterator. A Spliterator can traverse elements individually or all together.
Implementations of Iterable in Java
The Java Iterable interface (java.lang.Iterable) is among the root interfaces of the Java Collections API. Therefore, there are several classes in Java that implement the Java Iterable interface. These classes can thus have their internal elements iterated.
Implementing the Iterable Interface
- Let us take an example to understand the implementation of the Iterable Interface in our own class CakeShop.
- We create a class CakeShop that implements Iterable. We create an ArrayList to store different types of cakes, and int variable totalFlavors to keep count of all types of cakes.
- addCake() method adds the cake flavor to the cakeFlavors ArrayList. As CakeShop class implements Iterable, it must Override the iterator() method and return the Iterator of String type.
- This iterator can be used to iterate the objects of the CakeShop class. Iterator is also used in the back-end when we call for-each loop. Thus, most of the code is hidden and some part of it is present in the implementation of our class so that, in the main method code is short and clean.
- While Iterating currentIndex starts from 0 and goes till totalFlavors-1. hasNext() method returns a boolean. It returns true if currentIndex is less than totalFlavors else returns false.
- next() method returns value in ArrayList at currentIndex. If hasNext() is false it throws an exception.
Main Method:
Output:
We first create an object of CakeShop. We add different types of cake flavors to it using the addCake() method. Since the CakeShop class implements Iterable, we can call it the iterator() method. So, we create an object of Iterator named iter of String type and call iterator() method that returns an Iterator for CakeShop object named menu. Thus, if hasNext() is true while loop calls next() and print its value. If hasNext() is false while loop is terminated.
Difference between Iterator and Iterable in Java
Iterable Interface | Iterator Interface |
---|---|
Collection Framework extends Iterable Interface. Thus, Objects of classes implementing Collection Interface can be iterated. These classes Override the iterator() method which calls the Iterator interface. | Iterator interface returns an iterator of a specific class to transverse over a collection of elements. To iterate, hasNext() and next() methods are used in a loop. |
Iterable interface is located in java.lang.Iterable | Iterator interface is located in java.util.Iterator |
Classes that implement the Iterable interface need to override the iterator() method. | Classes that implement Iterator interface need to override hasNext(), next() and remove() methods. |
Iterable doesn't store any iteration state. Iteration state is the pointer to the element in a collection which the iterator is going to return next. | Iterator instance maintains the iteration state. This implies the user can check if the next element exists, move forward to the next element, etc. |
The Iterable method produces a new instance for an Iterator every time it is called. | Iterator instance maintains the iteration state. |
Iterable Interface has 3 methods i.e. iterator(), spliterator() and forEach() | Iterator has 4 methods i.e. next(), hasNext(), remove(), foreachRemaining(). |
For Iterable, we can move only in the forward direction. | Iterator's sub-instance like ListIterator can move in both directions i.e. forward and backward. |
Advantages of Iterable in Java
- Iteration is made easy using for-each loop.
- All the classes of Collection Interface can be transversed easily as it implements Iterable Interface.
- Elements can be read, removed, or operations can also be performed with them.
- It is considered to be the Universal Cursor for the Collection API.
- Methods are easy to use and implement.
Limitations of Iterable in Java
- We can move only in a forward direction while iterating. Reverse transversal is not possible.
- We can either read or remove elements. Both cannot be performed simultaneously. Also, Fail-Fast iterators throw in Java, if there is structural modification like addition, removal, updation of elements of the collection.
- Replacement or extension of objects is not facilitated.
- Elements cannot be added to the collection using an iterator.
- Java Iterator supports only Sequential transversal. We cannot skip elements or visit only even or odd positions in a collection.
- for-each loop is slower as for every iteration it calls iterator() method.
Conclusion
- Any class implementing Iterable Interface overrides the iterator() method, which returns an Iterator, that allows the user to iterate through the collection of that class.
- Iterable interface and Iterator interface are different and should not be confused with.
- Collection Interface extends Iterable interface, thus, all its classes have implementation for iterator() method.
- Iterable provides three methods namely iterator(), spliterator() and forEach().
- Iterator facilitates four methods namely next(), hasNext(), remove(), foreachRemaining().