Getting File Information Using Java IO streams
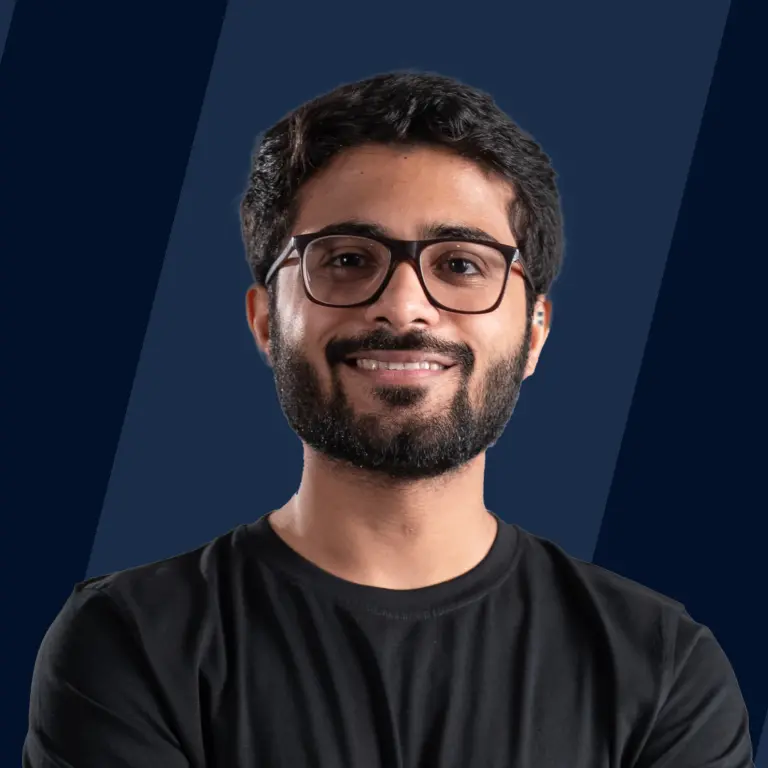
The Java programming language includes a lot of APIs that help developers to do more efficient coding. One of them is Java IO API which is designed to read and write data (input and output). For example, read data from a file or over the network and then write a response back over the network.
Introduction to Java IO Streams
The Java IO API is found in the java.io package. The Java IO package focuses mainly on input and output to files, network streams, internal memory buffers, etc. On the other hand, it lacks classes for opening network sockets, which are required for network communication. We need to use the Java Networking API for this purpose.
The Java IO package provides classes that include methods that are used to obtain metadata of a file. The definition of metadata is "data about other data". With a file system, the data is contained in its files and directories, and the metadata tracks information about each of these objects. In this tutorial, we are going to learn about various ways to determine the size of a file in Java.
File size is a measure of how much data it contains or how much storage it usually takes. The size of a file is usually measured in bytes. In Java, the following classes will help us to get file size:
- Java get file size using File class
- Get file size in java using FileChannel class
- Java get file size using Apache Commons IO FileUtils class
Takeaway:
- To speed up I/O operations, Java uses the concept of a stream.
- All classes required for input and output operations are included in the java.io package except the one for opening network sockets.
Java Getting File Size Using File Class
The Java File class is an abstract representation of file and directory pathnames. It is found in the java. io package. This class contains various methods which can be used to manipulate the files like creating new files and directories, searching and deleting files, enlisting the contents of a directory, as well as determining the attributes of files and directories. This is the oldest API to find out the size of a file in Java.
The File class in java contains a length() method that returns the size of the file in bytes. To use this method, we first need to create an object of the File class by calling the File(String pathname) constructor. This constructor creates a new File instance by converting the given pathname string into an abstract pathname.
An abstract pathname consists of an optional prefix string, such as disk drive specifiers, “/” for Unix, or “\” for Windows, and a sequence of zero or more string names.
The prefix string is platform-dependent. The last name in the abstract pathname represents a file or directory. All other names represent directories.
For example, "c:\data\inputfile.txt"
Now we can apply the File class length() method to the File object. It will return the length, in bytes, of the file denoted by this abstract pathname, or 0L if the file does not exist. The return value is unspecified if this pathname denotes a directory. So, we need to make sure the file exists and isn't a directory before invoking this java method to determine file size.
Here is the input file:
A simple java program to determine file size using the File class is shown below:
Output:
Explanation: Since the file exists, it will return the file size in bytes, else it would have return the “File does not exist!” statement.
Takeaway:
- If the pathname argument is null in File(String pathname), it will throw NullPointerException.
- Even if the file does not exist, it won't throw an exception, it will return 0L.
Get File Size In Java Using FileChannel Class
The Java FileChannel class is a channel that is connected to a file by which we can read data from a file and write data to a file or access file metadata. It is found in java.nio package (NIO stands for non-blocking I/O) which is a collection of Java programming language APIs that offer features for intensive I/O operations.
File channels are safe for use by multiple concurrent threads, making Java NIO more efficient than Java IO. However, only one operation that involves updating a channel's position or changing its file size is allowed at a time. If other threads are performing a similar operation, it will block them until the previous operation is completed.
Note: Although FileChannel is a part of the java.nio package, its operations cannot be set into non-blocking mode, it always runs in blocking mode.
Also, we can't create objects of the FileChannel class directly, we need to create them by invoking the open() method defined by this class. This method opens or creates a file, returning a file channel to access the file. After creating a FileChannel instance we can call the size() method which will return the current size of this channel's file, measured in bytes.
Here is the input file we need to parse −
Hello World in Java!
A simple java program to determine file size using the FileChannel class is shown below.
Output:
Explanation: Since the file exists, it will return the file size in bytes, else it would have thrown the java.nio.file.NoSuchFileException error.
Takeaway:
- We can’t create objects of FileChannel class directly, we have to create it by invoking the open() method.
- FileChannel is thread-safe but allows only one operation at a time that involves the channel's position or changing its file's size, blocking other similar operations.
Java Getting File Size Using Apache Commons IO FileUtils Class
The Java FileUtils are general file manipulation utilities. This class is found in the org.apache.commons.io package. It includes methods to perform various operations like writing to a file, reading from a file, making directories, copying and deleting files and directories, etc.
The Java FileUtils class provides the sizeOf() method that returns the size of the file in bytes. Firstly, we need to create a File instance and then pass it to the sizeOf() method. It will return the size of the specified file or directory. If the provided File is a regular file, then the file's length is returned. If the argument is a directory, then the size of the directory is calculated recursively.
Note that overflow is not detected, and the return value may be negative if overflow occurs. We can use sizeOfAsBigInteger(File) for an alternative method that does not overflow.
Here is the input file we need to parse −
Hello World in Java!
canonical_url: https://www.scaler.com/topics/java-file-size/ title: How to get Java File Size - Scaler Topics description: This article explains Java IO streams in brief & different ways to get java file size, along with java programs & various classes such as File, FileChannel, and FileUtils. author: Soma Chandra category: Java amphtml: https://www.scaler.com/topics/java-file-size/amp/ publish_date: 2022-04-18
The Java programming language includes a lot of APIs that help developers to do more efficient coding. One of them is Java IO API which is designed to read and write data (input and output). For example, read data from a file or over the network and then write a response back over the network.
Scope
- This article briefly explains Java IO streams and the different ways to get file size, along with java programs.
- We also learn about various classes such as File, FileChannel, and FileUtils.
Introduction to Java IO Streams
The Java IO API is found in the java.io package. The Java IO package focuses mainly on input and output to files, network streams, internal memory buffers, etc. However, it lacks classes for opening network sockets, which are required for network communication. We need to use the Java Networking API for this purpose.
The Java IO package provides classes that include methods used to obtain a file's metadata. The definition of metadata is "data about other data". With a file system, the data is contained in its files and directories, and the metadata tracks information about each of these objects. In this tutorial, we will learn about various ways to determine the size of a file in Java.
File size measures how much data it contains or how much storage it usually takes. The size of a file is usually measured in bytes. In Java, the following classes will help us to get file size:
- Java get file size using the File class
- Get file size in java using the FileChannel class
- Java get file size using Apache Commons IO FileUtils class
Takeaway:
- To speed up I/O operations, Java uses the concept of a stream.
- All classes required for input and output operations are included in the java.io package except for opening network sockets.
Java Getting File Size Using File Class
The Java File class is an abstract representation of file and directory pathnames. It is found in the java.io package. This class contains various methods for manipulating files, such as creating new files and directories, searching and deleting files, enlisting the contents of a directory, and determining the attributes of files and directories. This is the oldest API for finding out the size of a file in Java.
The File class in java contains a length() method that returns the file size in bytes. To use this method, we first need to create an object of the File class by calling the File(String pathname) constructor. This constructor creates a new File instance by converting the given pathname string into an abstract pathname.
An abstract pathname consists of an optional prefix string, such as disk drive specifiers, “/” for Unix or “\” for Windows, and a sequence of zero or more string names.
The prefix string is platform-dependent. The last name in the abstract pathname represents a file or directory. All other names represent directories.
For example, "c:\data\inputfile.txt"
Now, we can apply the File class length() method to the File object. It will return the file's length, in bytes, denoted by this abstract pathname, or 0L if the file does not exist. The return value is unspecified if this pathname denotes a directory. So, we need to ensure the file exists and isn't a directory before using this Java method to determine file size.
Here is the input file:
A simple java program to determine file size using the File class is shown below:
Output:
Explanation: Since the file exists, it will return its size in bytes; otherwise, it would have returned the “File does not exist!” statement.
Takeaway:
- If the pathname argument is null in File(String pathname), it will throw NullPointerException.
- Even if the file does not exist, it won't throw an exception; it will return 0L.
Get File Size In Java Using FileChannel Class
The Java FileChannel class is a channel that is connected to a file by which we can read data from a file and write data to a file or access file metadata. It is found in java.nio package (NIO stands for non-blocking I/O), a collection of Java programming language APIs offering features for intensive I/O operations.
File channels are safe for multiple concurrent threads, making Java NIO more efficient than Java IO. However, only one operation that involves updating a channel's position or changing its file size is allowed at a time. If other threads perform a similar operation, it will block them until the previous operation is completed.
Note: Although FileChannel is part of the Java.nio package, its operations cannot be set to non-blocking mode; it always runs in blocking mode.
Also, we can't create objects of the FileChannel class directly; we need to invoke the open() method defined by this class. This method opens or creates a file, returning a file channel to access the file. After creating a FileChannel instance, we can call the size() method, which will return the current size of this channel's file, measured in bytes.
Here is the input file we need to parse −
Hello World in Java!
A simple java program to determine file size using the FileChannel class is shown below.
Output:
Explanation: Since the file exists, it will return the file size in bytes; otherwise, it would have thrown the java.nio.file.NoSuchFileException error.
Takeaway:
- We can’t create objects of the FileChannel class directly; we have to create them by invoking the open() method.
- FileChannel is thread-safe but allows only one operation at a time involving the channel's position or changing its file's size, blocking similar operations.
Java Getting File Size Using Apache Commons IO FileUtils Class
The Java FileUtils are general file manipulation utilities. This class is found in the org.apache.commons.io package. It includes methods to perform various operations like writing to a file, reading from a file, making directories, copying and deleting files and directories, etc.
The Java FileUtils class provides the sizeOf() method, which returns the file's size in bytes. First, we need to create a File instance and then pass it to the sizeOf() method. It will return the size of the specified file or directory. If the provided File is a regular file, then the file's length is returned. If the argument is a directory, then the directory size is calculated recursively.
Overflow is not detected, and the return value may be negative if overflow occurs. We can use sizeOfAsBigInteger(File) for an alternative method that does not overflow.
Here is the input file we need to parse −
Hello World in Java!
A simple java program to determine file size using the FileUtils class is shown below.
Output:
Explanation: Since the file exists, it returns its size in bytes; otherwise, it would have thrown an exception.
Takeaway:
- The sizeOf() method will return the size of the specified file in bytes.
- If the file is null, it will throw NullPointerException, and if the file does not exist, it will throw IllegalArgumentException,
Conclusion
- The java.io package provides for system input and output through data streams, serialization, and the file system.
- Java provides various classes to determine the file size, i.e. File, FileChannel, and FileUtils class.
- The File class is found in the java.io package and provides a length() method to get file size.
- The FileChannel class is found in the java.nio package and provides size() method to determine the file size.
- FileChannel is thread-safe, making Java NIO more efficient than Java IO.
- The operations of FileChannel are blocking and can’t be set into non-blocking mode.
- The Java FileUtils class is found in the org.apache.commons.io package and provides the sizeOf() method to determine the file size.
Output:
Explanation: Since the file exists, it returns the file size in bytes, else it would have thrown an exception.
Takeaway:
- The sizeOf() method will return the size of the specified file in bytes.
- If the file is null, it will throw NullPointerException, and if the file does not exist, it will throw IllegalArgumentException, :::
Conclusion
- The java.io package provides for system input and output through data streams, serialization, and the file system.
- Java provides various classes to determine the file size i.e. File, FileChannel, and FileUtils class.
- The File class is found in the java.io package and provides a length() method to get file size.
- The FileChannel class is found in the java.nio package and provides size() method to determine the file size.
- FileChannel is thread-safe, making Java NIO more efficient than Java IO.
- The operations of FileChannel are blocking and can’t be set into non-blocking mode.
- The Java FileUtils class is found in org.apache.commons.io package and provides sizeOf() method to determine the file size.