Java Methods
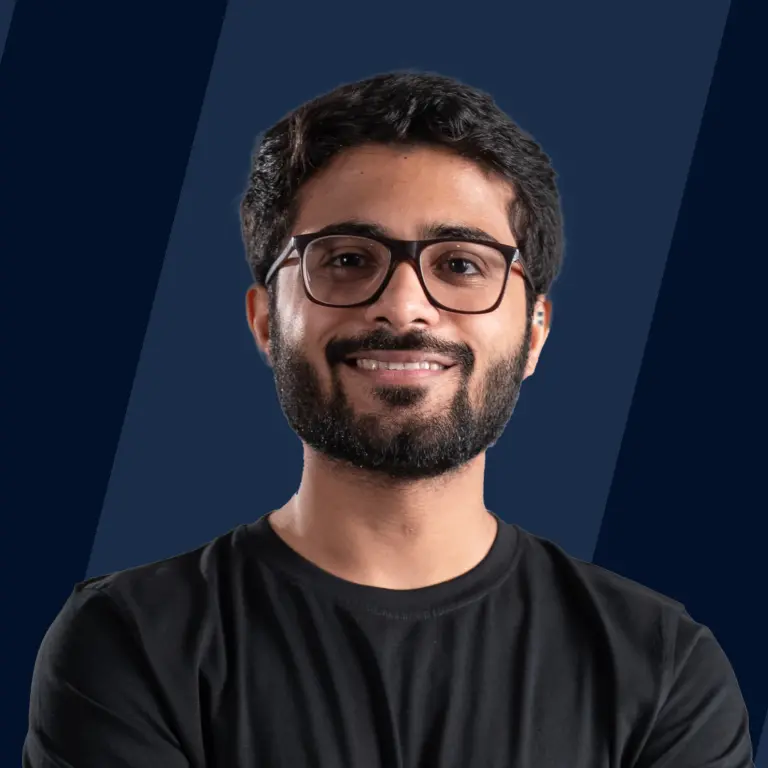
Methods in Java are some blocks of code that perform a specific function. A method runs only when it is called. A method can accept data as its arguments. Methods provide for easy modification and code reusability. It will get executed only when invoked/called. The primary usability of methods is in code reuse: define the code once and use it many times.
Method Declaration
A method in Java has various attributes like access modifier, return type, name, parameters, etc.
Methods can be declared using the following syntax:
Let us understand what each attribute means -
- accessModifier: It defines the method's access type, i.e., from where it can be accessed in your application.
- returnType: It represents the data type of the value returned by the function. For example, a method in Java declared with int return type should return an integer value.
- methodName: Represents the identifier that can be used to call the method when required.
- parameters: These are the arguments passed into a method necessary for the function's logic. We can pass data to the methods by specifying them within the parentheses if the methods have data.
See Also- Complete Java Tutorial
Naming a Method
The naming convention for a method in Java is generally a verb, in mixed case, with the first letter in lowercase and the first letter of each internal word capitalized. This naming convention is called the Camel case.
Example:
Method Calling
What we saw above is declaring a method. To use the method's functionality, you need to "call" a method. This is as simple as writing the method's name by passing its parameters. We should also take note of the function's return type.
Example
Output:
In the above code snippet, the method findFactorial expects an Integer type parameter to be passed.
To use this method, we need to call it. In the main method (Driver method), we call the function by passing the integer 7 as the value of the argument number. The value returned by the method is stored in the variable result.
Types of Methods in Java
Predefined Method
These are methods that Java class libraries define. They are also called standard library methods or built-in methods. They can be used by directly calling them. Some examples include print() in the package java.io.PrintStream, min() and max() as defined in Math class, etc.
User-defined Method
As you might have guessed, a method with custom logic is a user-defined method. The above example of converting temperature from Celsius to Fahrenheit is an example of a user-defined method in Java.
How to Call or Invoke a User-defined Method?
To invoke a user-defined method in Java, specify its name and provide any required arguments. Then, In the main method, call the method using its name followed by parentheses containing the arguments. Handle the return value if applicable by storing it in a variable or using it directly.
Static Method
A method in a class declared as static does not need an object of the class to invoke it. All the above built-in methods are static, so you could invoke the sqrt() method without creating an object of the Math class. In the example of a user-defined method to convert Celsius to Fahrenheit, the method is also static.
Instance Method
Instance methods in Java are attached to the objects of a class rather than the class itself. Here, the method belongs to a class whose object must be created to call the function. This is seen in the code snippet below, where an object obj of the Demo class is created to call the addNumbers() method.
Abstract Method
A method without any implementation but only the method signature is called an abstract method. An abstract method can be declared in an abstract class or an interface. A regular class can implement an abstract method by extending the abstract class containing the abstract method.
Abstract methods are used where you need to share the code among closely related classes or have many standard parameters.
- Abstract method in an abstract class
Factory method
The factory method creates and returns the objects to the client. A factory method may accept an input that denotes the type of object that needs to be created. Factory methods belong to a specific design pattern called "Factory pattern" which is a way to dynamically return an object of a class it belongs to, at run-time based on the user's choice.
For example, if we have a program to customize the choice of condiments you can add to your coffee, you can get the object for the chosen condiment on the go when it is created.
Memory Allocation for Methods Calls
Whenever we call a method, the Java Virtual Machine (JVM) allocates memory in two main ways - Heap and Stack.
Heap
Heap memory in Java dynamically allocates memory for objects and JRE classes at runtime. Objects created within methods are stored in the heap, with references managed in the stack. When heap space is full, Java throws java.lang.OutOfMemoryError. Accessing the heap memory is slower than accessing the stack memory.
Unlike the stack, heap memory isn't automatically deallocated; the Garbage Collector handles this task to maintain memory efficiency. However, the heap isn't inherently threadsafe and requires proper synchronization. Thus, managing heap memory efficiently is crucial to prevent memory errors and ensure application stability in Java programs.
Stack
It is used for static memory allocation and execution of a thread. Whenever we call a new method, as seen in the "Calling a method" section, the primitive values and references to objects used in the method will be pushed into the call stack as a stack frame. The access to this is Last In First Out (LIFO). After the execution of the method finishes, the stack frame is popped out.
Why Do We Need Methods in Java?
- Methods are essentially used to divide the logic of the whole program into manageable chunks with logic separation.
- Code can be reused throughout the program by calling the same method in multiple places. This is the single best use of methods in Java.
- Often, methods are used to hide or encapsulate implementation details.
- Methods also improve code readability, making it easier to understand the logic of the program.
Conclusion
- Java Methods are an integral part of the Java language used to maintain modularity in code.
- Methods in Java can have different access modifiers, such as public, private, protected, and default.
- There are three main types of methods: built-in, user-defined, and abstract methods.
- this keyword can be used to refer to current class instance variables and invoke the instance methods.
- Static methods in Java can be invoked directly without needing to create an object of its class. However, instance methods require objects of the class where they are declared to be invoked.
FAQs
Q: How many Varargs can we have in a function?
A: A method can have only one varargs parameter. Additionally, the varargs parameter must be the last argument to the method.
Q: Do we need to create an instance of the Main class to invoke the main method?
A: The Main Method in Java is static, which implies there is no need to create an instance of the Main class to invoke the main method.
Q: What are memory allocation methods?
A: The two fundamental memory allocation methods are static and dynamic. The static memory (stack memory) allocation method assigns the memory to a process before its execution. On the other hand, heap memory is allocated dynamically at runtime.
Q: Can Varargs be null?
A: As Java cannot determine the type of literal null, you must explicitly inform the literal null type to Java. Please do so to avoid a NullPointerException.