Static Method in Java With Examples
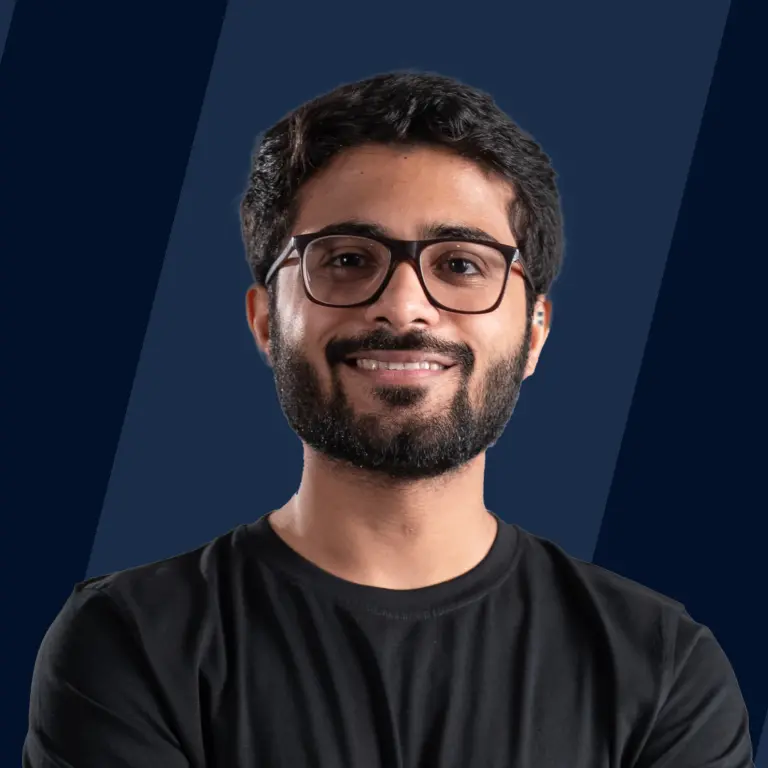
The static methods are class methods that don't belong to instances of the class. They are designed to be shared among all instances of the same class and are accessed by the class name of the particular class. In Java, a static keyword is used for declaring the static method.
The static method in Java cannot be overridden because static methods are linked to the class during the compilation time, and we know very well that method overriding is based on the runtime polymorphism. That's why the static methods cannot be overridden. The static methods can be accessed by the outside environment very easily. We have already discussed the main() method example in the above section.
The main() method is of static type because when the Java runtime starts, no object from the class is present. The main method has to be static so the JVM loads the class into the main memory and calls it.
Features of Static Method in Java
- In Java, a static method is a part of the class itself rather than an instance of a class.
- The method is available to each instance of the class.
- The method is available to each class instance. They have access to class variables (static variables) (instance) without requiring the class's object.
- A static method has only access to static data. It cannot access dynamic data (instance variables).
- Static methods can be accessed directly in non-static and static methods.
Syntax to Declare the Static Method
The return_type of the static method can be int, float, etc, any user-defined datatype.
Syntax to Call a Static Method
We can call the static methods using the class name because they belong to the class itself and have no relation with its instances.
Syntax:
The classname is the name of the class followed by the name of the static method.
Let's understand what a static method is called using an example.
Output:
Examples of Static Method in Java
After discussing static methods and when you might use them, let's look at real-world examples to understand how they function.
Example 1: Creating a Utility Class
Let's say we want to develop a utility class that includes operations for performing math. To include static methods for addition, subtraction, multiplication, and division, we may make a class called MathUtils. This is how the MathUtils class might appear:
We have developed a utility class with four static methods for mathematical operations in the code above. These MathUtils class methods can be called without first constructing an object of that class. For instance:
Example 2: Implementing the Singleton Design Pattern
Singletons are frequently utilised to manage resources like database connections or thread pools. Suppose our goal is to develop a class that symbolises a database connection. We can use the singleton design pattern to ensure that there can only be one connection to the database at a time.
Given below is the Java implementation to demonstrate the above explanation:
The DBConnection class's constructor has been designated private in the code above. By doing this, other classes cannot instantiate the DBConnection class. Moreover, a static method for obtaining the class instance and a static instance of the class are declared.
First, the getInstance() method verifies whether a class instance has already been created. If not, it builds one and returns it. If not, it returns the current instance. This guarantees that only one instance of the DBConnection class can exist at any one moment.
Why Use Static Methods?
This question always arises in the mind of the programmer: why use a static method in Java programs? Let's understand some common uses for static methods so that we can efficiently use them.
- If the implementation of the method's code is not changed by the instances of the class. In this case, we can make the method a static method.
- When we want to access static and other non-object-based static methods, then, we have to use static methods
- If we want to define some utility functions, such as the user-defined sorting method in the class, we can define them using static concepts because the utility function can easily be shared among all class objects due to the static method's property.
Restrictions of Static Method in Java
When the methods are declared as static, there are lots of restrictions applied to the static method that make it different from the instance methods of the class.
Let's discuss some restrictions on the static method in Java.
- The static method cannot invoke the instance member and class methods. Static methods are accessed without the class's object reference, but we cannot access the instance variables and methods without an object reference. If we try to access the instance variable or methods inside the static method, this will cause an' error'.
Let's try to understand this using an example.
Output:
In the above program, we are trying to access the nonstatic method from the class's static method, which will cause an error. Use this Free Online Compiler to compile your Java code.
- The static methods can only access other static members and methods. Because static members and methods are linked to the class during compilation, they can access each other without creating an instance of the class.
- We cannot use this and super keyword in the body of the static method because the this keyword is the reference of the current object, but without creating an object, we can access the static method. This will cause an error, and in the case of the super keyword, we know super is the reference of the parent class very well, but we cannot use a reference in the body of the static method. We have already discussed the reason behind this.
main Method in Java Static
Java's main() method is static since an object doesn't need to call a static method. If the function weren't static, JVM would create an object before executing the main() method, making memory allocation more complex.
Important Points to Remember about Static Methods
- The static methods are the class methods and are accessed by the name of the class.
- The static methods cannot be overridden because static methods are resolved at the compilation time of the Java program, and we know very well the method overriding is the runtime polymorphism.
- The keywords such as super and this cannot be used in the body of the static method; we have already discussed the reason behind this in the above section.
- The static methods cannot access the instance members and methods of the class.
- The state of the static methods is equally shared among all the class objects.
Difference between the Static Method and Instance Method
Static method | Instance method |
---|---|
Static method can be considered as pass-by-reference programming. | While instance methods are considered as pass-by-value programming. |
The static method doesn't require an object from a class. | Instance methods require an object of a class. |
Its syntax - classname.methodname() | Its syntax - objref.methodname() |
Static method has only access of static attribute. | While Instance method has access to all of the attribute. |
Static method is only accessible by classname. | Instance methods are only accessible by object reference. |
Conclusion
- The static members and methods belong to the class rather than the instance of the class.
- They are accessed by the name of the class.
- The keywords such as this and super are not used in the body of the static method.
- Modification of the static field value is not allowed.