Java toString() Method
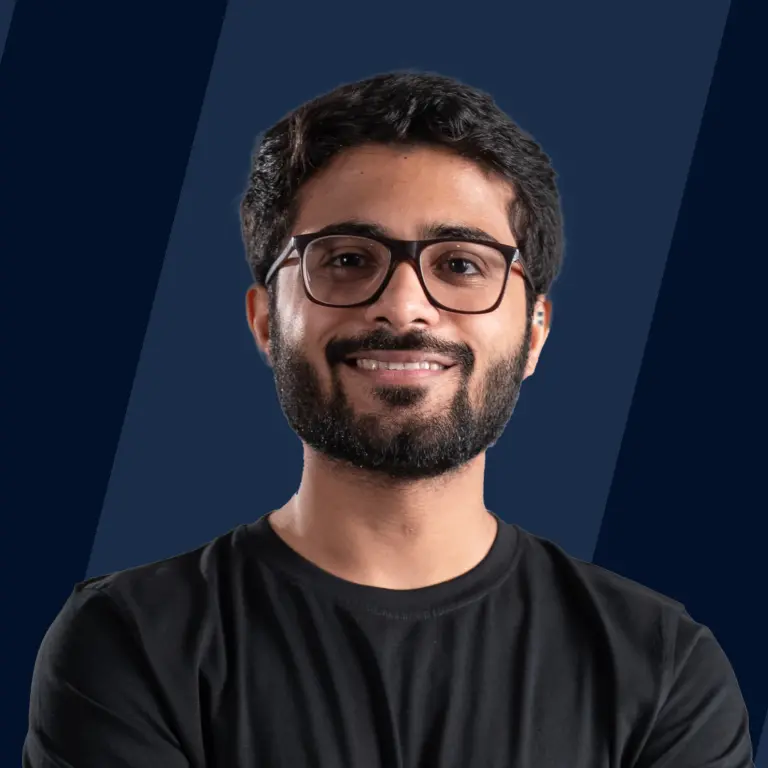
Overview
The toString() method of the Object class returns the string representation of an object in Java. If we print any object, the Java Compiler internally invokes the toString() method on that object. The value returned by the toString() method is then printed.
Since toString() method is defined in Object class and all classes inherit from the Object class, toString() method can be invoked on objects of all classes.
Override the toString() method in Java
Let's override the toString() method to custom define the string representation of Student class objects.
Output:
Explanation:
- Here we can see, we have written our custom implementation, where we have concatenated the name and rollNo of the student to the returning string.
- On running the code we get Student{name='Aniket', rollNo=12}.
- Now this is some useful information regarding the Student object std. In the similar way if you want to have the String representation of an object, you need to override the existing implementation.
Java Classes with toString() Overridden by Default
Let’s talk about some of the classes in Java that have implemented the toString() method to give some meaningful String representation of the objects.
In Java, all the wrapper classes like Byte, Integer, Long, Float, Double, Boolean, Character have overridden the toString() method. The collection classes such as String, StringBuilder and StringBuffer have also overridden the toString() method as well. Let’s check the running code to make it more clear.
Code:
Output:
Here we can notice two things,
- We have not used the toString() to print the String value of objects, because System.out.println() method by default uses toString() method internally.
- We have not implemented the toString() method, Java has itself overridden it for us.
Using static toString() method in Wrapper Classes
The Wrapper classes in Java have a static implementation of the toString() method as well. We can directly get the String value without defining an object of the class.
Code:
Output:
Using valueOf() method in String Class
One more way to get the String representation of an object is to use the String.valueOf() method of the String class, which internally invokes the toString() method on that particular object.
Code:
Output:
Conclusion
- The toString() method is defined in the Object class. It returns the String representation of objects in Java.
- The default implementation of toString() method returns the concatenation of class name and hashcode value of the object separated by @.
- We can override the definition of toString() method in Java in a class to custom define the string representation of its objects.
- System.out.println() invokes the toString() method internally to print an object in the console.
- Wrapper and Collection classes have their own implementation of toString() method.