JavaScript Classes
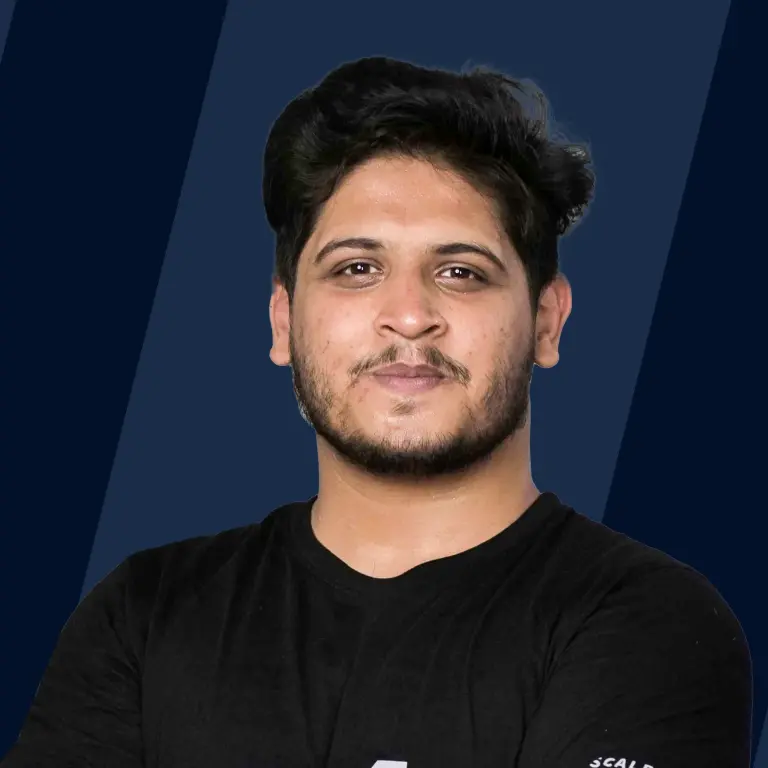
Overview
A JavaScript class is a blueprint used to create objects in Javascript. Classes in Javascript can be defined as a template that encapsulates the data with code to work on that data. The classes in Javascript are often referred to as the syntactical sugar provided in the ES6 addition to extend object properties and methods in a more elegant manner (which was earlier done using prototypes and inheritance).
Introduction to JavaScript Classes
In daily life, we often handle data or items with similar properties. For example, creating virtual identity cards for 100 students involves attributes like name, class, and roll number. This demonstrates the use of classes and objects. JavaScript, a prototype-based language, utilizes a hidden [[Prototype]] property to extend object properties and methods. ES6 introduced the concept of classes in JavaScript, offering a cleaner syntax for writing blueprints that can be extended to create objects. Note: You don't need to know about prior ES6 ways or any prior knowledge of classes to understand this article.
JavaScript Class Syntax
Explanation of the Syntax:
- The class keyword is used to create classes in javascript.
- The Classname is the name of the class. It is user-defined. (Conventional method is to start the class name with a capital letter).
- The constructor() is a method used to initialize the object properties. It is executed automatically when a new object of the given class is created. (It may take parameters).
Creating JavaScript Class
Let's create a class for students studying in a class. This class will consist of student's first name, last name, age, and roll no.
Code:
The this keyword in Javascript is used to refer to the current object in a method or constructor. It is used to stamp out the confusion between class attributes and parameters that are passed to the constructor with the same name.
In the above code block, we are creating a class named Student (by convention, the name of a class starts with a capital letter). The Student class has four attributes, named firstName, lastName, age, rollNo. The constructor method takes four parameters and assigns them to the class attributes.
Note: In the above example, the class attribute names and the constructor parameters names are the same, but it should be noted that they are not the same.
The constructor parameters are the values passed by the user while creating an object which is assigned to the class attributes.
The class attributes and the constructor parameters can have different names, although it is a common practice to write similar names for both. For example:
Javascript Class Methods
So far, we've created a class Student with different attributes to store the data. Now imagine if we have to display that data. How do we do that?
The javascript class methods are used to
code:
In the above code, the name() method is used to return the name of the Student. Upon being called, the name() method will return a string that has the class attributes firstName and lastName.
The methods of classes in javascript are mainly categorized into three kinds:
- Instance methods
- Static methods
- Getter and Setter methods
We have discussed all three with detailed examples in further sections.
Using a Javascript Class
Now that we've declared a class in Javascript, the question is how to use it? Since the classes in Javascript are just a blueprint, they cannot be used directly. To use the classes in Javascript, we need to create an object.
The object in Javascript is a standalone entity created by the user that has properties and types. It can be defined as the physical entity built upon a class definition.
Example
The new keyword in JavaScript creates an instance of an object that has a constructor function.
Explanation of the Example
The above code will create an object s1 from the Student. The parameters ' Peter', 'Parker', 16, 48 will be passed to the constructor, which will be assigned to the class attributes.
The Constructor Method
The constructor() is a method used to initialize the attributes in Javascript classes. When an object is created, its class's constructor() method is called.
- If the classes in Javascript are defined without any constructor, the Javascript class, upon initialization, automatically creates an empty constructor.
- The constructor method in the Javascript class can either be empty or may take parameters.
Syntax
- The constructor method inside Javascript classes is created using the constructor keyword.
- The () contains parameters that may be assigned to the Javascript class attributes.
- The {} contains the definition of the method.
Example
Explanation of the Example:
In the above example, we have created a Javascript class Programming, inside which we have created a constructor which will print "Programming class is called" in the console upon being called. When the object p1 is made, it initiates the Programming class, which automatically initiates the constructor method inside the javascript class, thus "Programming class is called" is being displayed in the console.
Extending a Class
One of the most useful features of OOP in javascript is inheritance. Inheritance allows us to define a new class that will take all the functionality from a parent class, thus extending that class while having its own functionalities.
Classes in Javascript are extended (or inherited) from other classes using the extends keyword.
Example
Note: The super keyword is used to access and call functions on an object's parent.
Explanation of the Example
In the above code, we have defined the class Coder with the attribute language and method displayLanguage() to display the language. The class Coder extends the class Student thus, it would have access to the attributes and methods of Student.
Class Fields
Fields are the variables of the classes in Javascript that are used to store the information. There are two types of fields in a Javascript class:
- Instance field
- Static field
Both Instance field and Static field are further divided into public and private.
Instance Field
Private Instance Field
By default, the attributes of classes in javascript are public, thus, they can be accessed and modified outside the class. So, in order to prevent this, we declare a private class field.
Private instance fields are declared by adding a # prefix before the variable name.
Public instance Field
By default the attributes of classes in javascript are public. Thus public instance fields can be declared by simply declaring variables in javascript classes.
Example
Explanation of the Example:
In the above example, the variable age is declared as a private field, thus when we call p1.birthday it initiates the birthday method inside the class, which increments the age by 1, but when p1.age is assigned the value 10, it returns an error as we cannot access the private fields outside the class.
The variable name is declared as a public field thus, when we make an object with the constructor parameter Jon, it sets the value of name as Jon, but when we assign the value Peter to the name attribute outside the class, it updates its value.
Static field
Private Static Field
A private static field is created by adding the static keyword before a private instance field declaration.
Public Static Field
A public static field is created by adding the static keyword before a variable name.
Example
Explanation of the example
In the above example, the variable #age is declared as a static private field, thus it is accessible only within the User class thus, p1.#age would display an error while name being a public static method can be accessed from anywhere thus p1.name outputs Jon.
Getters and Setters Method
So far, we have learned how to create classes, assign attributes, how to make methods and objects.
The private attributes of a class cannot be accessed directly by an object. Also, it is common practice not to directly interact with the attributes inside the classes in javascript.
Thus, in order to access the attributes of the Javascript class, we use getter and setter methods.
Getter method
The getter methods are used to get or access the values of attributes of classes in Javascript.
- The getter methods are declared by adding the get keyword before the method name.
Setter method
The setter methods are used to set or manipulate the values of attributes of classes in Javascript.
- The setter methods are declared by adding the set keyword before the method name.
Example
Explanation of the Example
In the above example, the get language() method is the getter method which returns the language attribute of the javascript class, and the set language() method is the setter method which takes a parameter and assigns that to the language attribute of the javascript class.
Upon creating the p1 object, since we have not assigned any value to the language attribute, so the getter p1.getLanguage will output undefined. Now, we will use the setter p1.setLanguage to set the value of language attribute to Javascript. Now, upon calling the getter p1.getLanguage, it will output Javascript.
Hoisting
Hoisting in Javascript is the process where the interpreter appears to move the declarations variables of functions, or classes to the top of their scope, before the execution of the code.
The classes in Javascript, unlike the functions, are not hoisted on the top of the scope, i.e. a Javascript class cannot be accessed before its a declaration in the program.
Example
Explanation of the example
In the above example, the line let p1 = new Programming(); will output a ReferenceError as the interpreter couldn't find the Programming class.
'use strict'
The 'use-strict’ or strict mode in Javascript is an ES5 addition that is used to specify that the Javascript code should be executed in the strict mode.
Following are some of the changes that the use-strict introduces to the Javascript:
- It replaces silent errors in Javascript code to throw errors.
- Adds syntax restrictions, i.e., it prohibits some declarations that could have been done in Javascript code if the 'use-strict' were not present.
- Due to rigorous checking, it makes the program more optimal.
The classes in Javascript always follow the 'use-strict', i.e., the code inside the Javascript classes is always in the strict mode.
Computed Names […]
The Computed Names property was added to Javascript in the ES6 adaptation to allow the names of object properties in JavaScript object literals to be determined dynamically.
The JavaScript objects are a kind of dictionary, thus the idea behind this feature was to use a key-value kind of syntax (object[‘keyStr’] = value) where 'keyStr' is a dynamically created string used as a key.
Example
Explanation of the example
In the above example, we have created an object literal Person with three keys firstName, lastName and [keyStr]. Here the third key holds dynamic value and depends on the value of keyStr. Thus when we call 'Person.age' it outputs the value of [keyStr] in the string.
Making Bound Methods With Class Fields
As discussed earlier, this keyword in Javascript is used to refer to the object it belongs to. Thus it may keep obtaining different values according to the context (i.e. the value depends on where it is used).
Thus when an object method is passed around and called some other context, the object reference of the this keyword will change.
This problem is often known as losing 'this' in Javascript.
Example
In the above example, we have declared a class Person with an attribute name. Now the constructor is supposed to set the value of an attribute to the parameter (i.e., Peter), and the sayName() method is supposed to print Peter.
But the output is undefined, because the setTimeout() method has got the p1.sayName separately from the object, thus it has lost the user context.
Note: The Javascript setTimeout method acts differently in the browser, i.e., it sets this=window for the function call. Thus in the case of this.name it tries to get window.name, which apparently does not exist, thus in such cases, it usually becomes undefined.
There are two ways to fix this issue:
-
By Passing a Wrapper-function:
The loosing this issue can be fixed by passing the wrapper functions such as setTimeout(() => p1.sayName(), 100) to tackle the issue of setTimeout() function.
Example
-
By Binding the Method to Object:
The losing this issue can also be solved by binding the object with the method. This could be done inside the constructor or any defined method.
No Arrow Function
The arrow function was introduced in ES6. An arrow function expression can be defined as an alternative to regular functions, having compact syntactical features although it does not have its own bindings to the keywords like this, super, arguments, or new.target etc.
An arrow function cannot be used as a constructor, and they are considered as methods.
As already discussed in the first section, classes in Javascript are described as the syntactical sugar provided over prototypes and inheritance, the arrow function could be defined as the syntactical sugar in order to offer a cleaner and more elegant syntax.
Following are the two major differences that this syntactical sugar provides:
Arrow Function has No Prototype Property
The arrow function does not have the prototype property.
Example
Explanation of the Example
In the above example, the declaration 'Programming1.prototype.framework' is valid as it is done using the function approach but the declaration 'Programming2.prototype' returns undefined as the arrow functions do not support the prototype property.
Arrow Function has No New Operator
i.e. arrow functions can not be used as constructors.
Example
Explanation of the Example
In the above example, the declaration 'coder1' is valid as it is done using the function approach but the declaration 'coder2' returns TypeError as the arrow functions do not support the new keyword thus making the new Programming2 an invalid declaration.
Class Features Availability
So far, the features of classes in Javascript that we have discussed were presented in the ES2015 proposal at stage 3. In the 2019 proposal, the class features were split between:
- The Public and private static fields and private static methods have been made part of the Class static features proposal.
- The Public and private instance fields have been made part of Class fields proposal.
- The Private instance methods and accessors have been made part of Class private methods proposal.
- The rest of the part is still based on the ES2015 standard.
Class vs. Custom type
Class | Custom type |
---|---|
Class declarations are not hoisted, i.e. they cannot be accessed above their declaration in the code. | function declarations are hoisted, i.e. they can be accessed above their declaration in the code. |
The code definition inside the classes in Javascript, by default, executes in the 'use-strict' mode. | The code definition inside the function in javascript do not execute in the 'use-strict' mode. |
Classes in Javascript are non-enumerable, i.e. its objects can have properties that don't show up when iterated. | Functions in javascript are enumerable. |
We need the new keyword to call the class while making an object. | No additional keywords are required in the custom type of functional method. |
Browser Support
Class properties and methods | Chrome | Firefox | Opera | Safari | Internet Explorer | Edge |
---|---|---|---|---|---|---|
classes | 49 | 45 | 36 | 9 | n/a | 13 |
constructor | 49 | 45 | 36 | 9 | n/a | 13 |
private class fields | 49 | 45 | 36 | 9 | n/a | 13 |
private class methods | 74 | 90 | 62 | 14.1 | n/a | 79 |
public class field | 84 | 69 | 60 | 14.1 | n/a | 79 |
static class field | 72 | 75 | 36 | 14.1 | n/a | 79 |
- The first column contains properties and methods of classes in javascript.
- The first row contains the names of the web browsers
- The number mentioned is the minimum version of the web browser required to support the property mentioned in the respective column.
- Note: n/a means that the web browser doesn't support that particular property.
FAQs
Q. What is a JavaScript class?
A. A JavaScript class is a blueprint or template used to create objects with similar properties and methods. It encapsulates data and code to work on that data, providing a more organized and structured approach to object-oriented programming in JavaScript.
Q. How do you define a JavaScript class?
A. In JavaScript, you can define a class using the keyword called class followed by the name of the class and a constructor method. The constructor method is responsible for initializing the object's properties. Here's an example syntax:
Q. How do you create an object from a JavaScript class?
A. To create an object from a JavaScript class, we can use the new keyword followed by the name of class and any necessary constructor arguments. This process is known as instantiation. Here's an example:
Q. Can a JavaScript class inherit from another class?
A. Yes, JavaScript classes can inherit properties and methods from other classes using the extends keyword. This concept is known as inheritance and allows you to create a hierarchy of classes. The child class can access and extend the functionality of the parent class. Here's an example:
Q. What are getter and setter methods in a JavaScript class?
A. Getter and setter methods are used to access and modify the attributes of a JavaScript class. Getters retrieve the value of an attribute, while setters modify the value. These methods provide an abstraction layer and allow you to control how attributes are accessed and manipulated. Here's an example:
Explore Scaler Topics Javascript Tutorial and enhance your Javascript skills with Reading Tracks and Challenges.
Conclusion
- Classes in Javascript are blueprints to create objects.
- Javascript classes consist of attributes and methods
- Attribute in a Javascript class is used to store data.
- Methods are used to access the attributes of classes in javascript.
- The constructor() is a method used to initialize the objects in Javascript classes.
- Objects are physical entities declared upon a Javascript class declaration.
- The classes in Javascript follow the use-strict prototype to define the code inside them.
- Fields are basically variables defined inside a Javascript class. There are two types of variables: static and instance.
- The ES6 addition has added new features like no arrow function, computed names, etc., to provide the syntactical sugar over the old syntax.