What is DOM Manipulation in JavaScript?
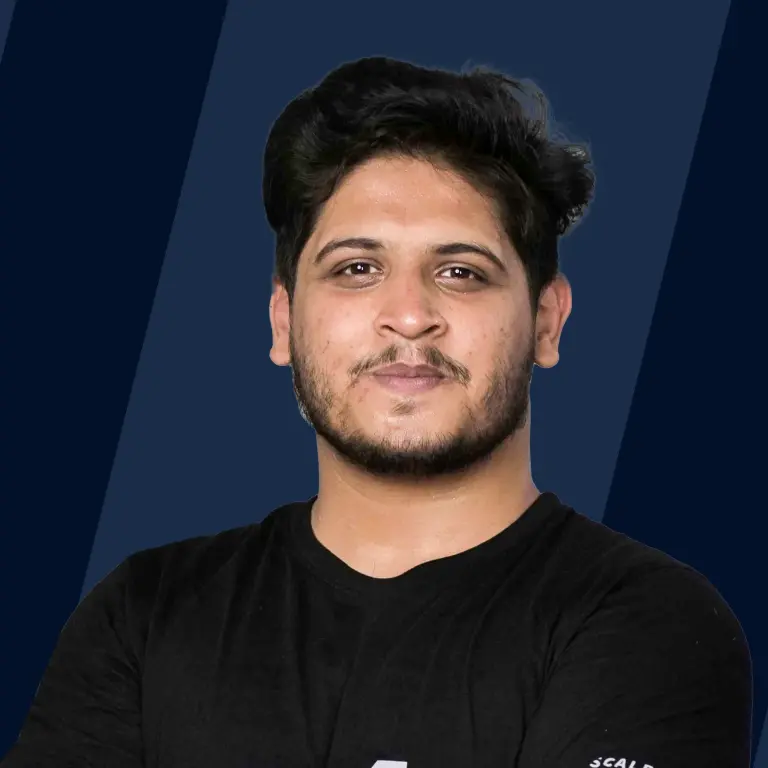
DOM manipulation in javascript is an important factor while creating a web application using HTML and JavaScript. It is the process of interacting with the DOM API to change or modify an HTML document that will be displayed in a web browser. This HTML document can be changed to add or remove elements, update existing elements, rearrange existing elements, etc.
By manipulating the DOM, we can create web applications that update the data in a web page without refreshing the page and can change its layout without doing a refresh. Throughout the document, items can be deleted, moved, or rearranged.
Definition of the DOM and Basic concepts
The DOM in dom manipulation in javascript stands for Document Object Model. The Document Object Model (DOM) is a tree-like structure illustrating the hierarchical relationship between various HTML elements. It can be easily explained as a tree of nodes generated by the browser. Each node has unique properties and methods that can be changed using JavaScript.
A visual representation of the DOM tree is shown in the image below.
- Document is the core/foundation of the DOM.
- HTML root element is the child of the document object.
- Body and Head elements are the children of the HTML element and siblings to each other.
- Title element is the parent to the text node: "my text” and the child of the head element.
- a tag and h1 tag are the children of the body element and siblings to each other.
- href attribute is the children of the a(anchor) tag.
The DOM is referred to as a programming API for XML and HTML documents. DOM defines the logical structure of documents and the methods for accessing and changing them are specified by the DOM.
Few Points to Keep in Mind
- A node can have more than one child.
- Siblings are nodes with the same parent like brothers or sisters.
- Except for the top node, which has no parent, each node has exactly one parent.
How to Select Elements in the DOM
In order to change or modify an element in the DOM, you need to select that specific element. Thus, JavaScript has six methods to select an element from a document in dom manipulation in javascript.
- getElementById: returns an element whose id matches a passed string. Since the ids of elements are unique, this is the fastest way to select an element.
- getElementsByTagName: returns a collection of all the elements present in the document that have the specified tag name, in the order of their appearance in the document.
- getElementsByClassName: returns an HTMLCollection of elements that match the passed class name. Bypassing the class names separated by whitespace, we can search for multiple class names.
- getElementsByName: returns a NodeList Collection of the elements that match the value of the name attribute with the passed string.
- querySelector: returns the very first element within the document that matches the given selector. It only returns the element that matches with one of the specified CSS selectors, or a group of selectors.
- querySelectorAll: returns a static NodeList of elements that matches with one or a group of selectors. If no element matches, an empty NodeList is returned.
How to Traverse and Move Around the DOM
With the HTML DOM, we can navigate through the tree nodes and access them using node relationships that we have discussed earlier parent, children, siblings, etc.
In this section, we will learn how to get the parent element, and children of an element, and siblings of an element.
Get the Parent Element
To get the parent node of a particular node in the DOM tree, we can use the parentNode property. for example,
ParentNode is a read-only object. There is no parent for the Document and DocumentFragment nodes. As a result, the parentNode is always empty. The parentNode of a newly created node that hasn't been connected to the DOM tree will also be null.
Get Child Elements
To Get all child elements use the firstChild property that returns the first child element of a specified element.
To Get the last child element use the lastChild property that returns the first element node, text node, or comment node.
To Get all child elements use the childNodes property that returns a live NodeList of child elements of a specified element.
Get Siblings of an Element
To Get the next siblings use the nextElementSibling property
To Get the previous siblings use the previousElementSibling property.
How to Manipulate Elements in the DOM
Create a New Element
The document.createElement() returns a new Node with the Element type. It takes an HTML tag name as a parameter.
Get and Set the Text Content of a Node
The textContent property returns the concatenation of the textContent of all child nodes and does not include the comments.
Get and Set the HTML Content of an Element
The innerHTML is a property of the Element that allows us to get or set the HTML markup contained within the element.
Append a Node to a List of Child Nodes of a Particular Parent Node
The appendChild() method allows us to insert a node at the end of the list of child nodes of a particular parent node.
Insert One Element Before an Existing Node as a Child Node of a Specified Parent Node
The insertBefore() JavaScript method takes two parameters, the newNode, and the existingNode. insertBefore() returns the inserted child node.
Replace a Child Element by a New Element
The replaceChild() JavaScript method takes two parameters to replace our first element with the newly created one.
Remove Child Elements of a Node
The removeChild() JavaScript method takes just one parameter i.e the element you want to remove.
Clone an Element and All of Its Descendants
This method allows us to clone an element. The cloneNode() method takes a parameter deep that is optional. If the deep is true, then the original node and all of its descendants are already cloned, false otherwise.
Working with Events
Here are some events used in dom manipulation in javascript:
- Handling events: includes HTML event handler attribute, element’s event handler property, and addEventListener().
- Page Load Events: includes DOMContentLoaded, load, beforeunload, and unload.
- load event: includes dependent resources like JavaScript files, CSS files, and images.
- Unload event: The unload event is fired after before unload event and pagehide event.
- Mouse events: includes mousedown, mouseup, and click.
- Keyboard events: includes keydown, keypress, and keyup.
- Scroll events: includes scrollX and scrollY properties that returns the number of pixels that the document is currently scrolled horizontally and vertically.
Manipulating Element’s Styles
style property
The style property returns the read-only CSSStyleDeclaration object that includes a list of CSS properties.
getComputedStyle()
The getComputedStyle() method returns the object that contains the computed style of an element.
className Property
The className property returns a space-separated list of CSS classes of the element as a string.
classList Property
The classList returns a live collection of CSS classes. It is a read-only property of an element.
Event Handling in the DOM
Since the beginning of the language, event handling has been a part of dom manipulation in javascript. They correspond to specific, user-imitated actions within the webpage, such as the moving of your mouse over a link, clicking on a link, or submitting a form. Thanks to event handling, our scripts are more interactive and are able to perform certain actions depending on the users.
The DOM of modern web browsers such as NS6+, IE5+, and Firefox provide expanded methods and flexibility for capturing events.
Scripting Web Forms
JavaScript Form
To create a form in HTML, you use the <form> element, for example
Output
Radio Button
To know which radio button is checked, we need to use the value attribute, For example
Output
Checkbox
To create a checkbox, you use the <input> element with the type of checkbox, for example
Output
Select Box
To create a <select> element, you use the <select> and <option> elements. For example:
Output
Handling Input Event
The input event fires each time whenever the value of the <input>, <select>, or <textarea> element updates.
Output
Conclusion
- DOM manipulation in javascript is the process of interacting with the DOM API to change or modify an HTML document that will be displayed in a web browser.
- By manipulating the DOM, we can create web applications that update the data in a web page without refreshing the page.
- The DOM stands for Document Object Model. The Document Object Model (DOM) is a tree-like structure illustrating the hierarchical relationship between various HTML elements.
- In order to change or modify an element in the DOM, you need to select that specific element. Thus, JavaScript has six methods to select an element from a document.
- We can navigate through the tree nodes and access them using node relationships like a parent, children, siblings, etc. We have learned how to get the parent, children, and siblings.
- In dom manipulation in javascript, A node can have more than one child. Siblings are nodes with the same parent like brothers or sisters. Except for the top node, which has no parent, each node has exactly one parent.
- We have discussed How to Traverse and Move Around the DOM, and How to Manipulate Elements in the DOM.
- We have also seen working with Events and Manipulating Element’s Styles and different Scripting Web Forms with examples.