What is a JavaScript Function Parameter?
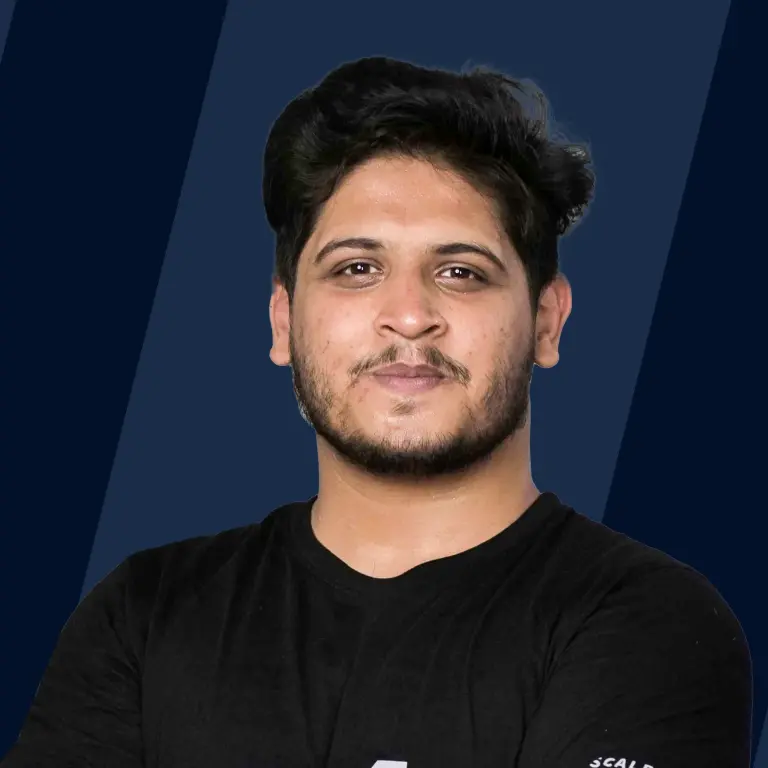
What is a JavaScript Function Parameter?
In Javascript, Despite having a major difference, the phrases "parameters" and "arguments" of a function" are frequently used synonymously. The names defined in the function definition are known as function parameters. The javascript function parameters are part of the definition of a function. We can give multiple parameters inside a function which is separated by commas inside parentheses.
Syntax
Example
Let's take a code to understand the concept of javascript function parameters in which we will find the largest element among the given element:
Output:
Explanation:
Firstly, we have made a function named "max_3num" which takes 3 parameters and returns the maximum value out of those numbers; then, we have used nested if else loop to check which number is greater among all those three numbers.
Function Arguments in JavaScript
A function's argument is the value we supply when calling a function. Real arguments are provided whenever we call the function in the program.
For example, suppose a block of code:
Here, 12 and 15 are the arguments passed to the "xyz" function.
Let's sum up the concept of argument and parameter together inside a block of code:
We will write a program in which we will create a function that will take two arguments and multiply both the numbers and return the resulting value:
Example
Output:
Explanation:
Here, we have made a function named "mulNumber" in which (a,b) are the javascript function parameters and the value (12,20) are the function argument which is passed then it gives multiplication of both the numbers as the output.
Function Parameter Rules in JavaScript
- Data types for parameters are not specified in JavaScript function declarations.
- The given arguments are not type checked by JavaScript functions.
- The number of received arguments is not checked by JavaScript functions.
Parameters
Name
The name of the function is specified using it.
Arguments
It is provided in the function's argument field.
Adding Function Parameters in JavaScript
We can add multiple javascript function parameters in our Javascript function according to your program requirements.
For instance, we will create a function named "demo()", which will have zero parameters and will return a string.
Now, we will create a function named "demo1()". It will take a single parameter, "name," and when this function is called, a string containing the provided "name" argument will be returned:
Now we will create another function named subNumber(). The subNumber() will take two arguments passed in the "a" and "b" parameters, and it will return their difference:
Now, let's call the all the three functions we have made above by passing the values as an argument to the functions:
Output
Unmatched Function Parameters in JavaScript
When the number of arguments you pass is greater than or less than the number of parameters, the JavaScript function won't throw an error.
For example, suppose we have created a function subNumber() which takes 2 parameter, "a" and "b"
Code
The subNumber() JavaScript function will not throw an error if it is called without any arguments. The additional arguments, however, will be initialized as "undefined".
So, in the above situation, when we call the function without passing any argument, then "a" and "b" are initialized as "undefined".
The JavaScript interpreter will, however, ignore any additional arguments if the number of arguments is more than the number of parameters.
Now suppose we pass a single argument, that is, "a" as a parameter, then "b" will be initialized as "undefined".
Suppose we have passed more arguments than the defined parameter in the function; than, in this condition, the "a" and "b" will be initialized with the values, and the third argument will be ignored.
Concluding all the above three cases which is shown below:
Code
Output
Default Function Parameters in JavaScript
You can specify the javascript function parameter default values in JavaScript as well. In the function declaration, the assignment operator "=" is used to combine these values.
Let's take an example to understand the concept of default function:
Example
Output:
Explanation
Here in the above case, in subNumber() function we have assigned "0" to both the variables of our function, that is "a" and "b". So, now if we don't pass any argument to the function, it will initialize and it's value to be zero.
Using Argument Object in JavaScript
There is a built object in Javascript functions called an Argument object. The arguments of a function are maintained in an array-like object. You can refer to the arguments supplied to a function in the following ways:
arguments[j]
where j is the ordinal number starting from 0, so, the first argument passed to a function would be arguments[0].
Similarly, the total number of arguments is indicated by
arguments.length
You can call a function with more parameters than it is explicitly specified to take by using the arguments object.
Let's consider an example in which we will multiply the arguments of a function:
Code
Output
Explanation
Here, we have made a function named "mulArgs" which takes the argument passed and treats it as an array of elements, and then initializes a variable named "mul" to 1 then a for loop is used to iterate through the length of argument and in the for loop each element of the loop is multiplied and then returned as an output.
Pass by Value and Pass by Reference in JavaScript
Pass by Value
When a parameter is sent by value in JavaScript, a copy of the parameter's real value is created in memory (a new memory allocation is established), and all changes are applied to that new value (i.e., copied value). The original value and the copied value are independent of one another since they both occupy a separate amount of memory; as a result, changing the value of the variable inside the function has no impact on the variable outside the function.
Read our Pass By Value vs. Pass By Reference in JavaScript article for detailed information. Let's take an example to understand the concept of Pass the value:
Code
Explanation:
In the above code, we have created a variable named res1 and assigned a value of 25 to it, which creates space in the memory by the name res1 and suppose its address is 1001. Then we create a variable res2 and assign res1 to it; subsequently, the equals operator detects that we are working with primitive values and builds a new space in memory with address 1002 and gets a copy of res1 value i.e., 25. Now, we can see that both the variables have the same value(i.e., 25), but both of them have different spaces. So if a change is done in one variable, then it does not affect another variable value.
Output
Pass by Reference
In the javascript function parameter, instead of creating a new memory location like the pass by value does in JavaScript, pass-by-reference allows the function to retrieve the variable's initial value by passing the reference or address of the actual parameter. Therefore, the original value also changes if we modify the value of the variable inside the function.
Let's take an example to understand the concept of Pass the reference:
Code
Explanation:
Here, we call the demo(student) function, and a new variable "obj" will be created, which will get details from the student object. Both student and obj variables refer to the same object. So, in such cases, any changes done to the "obj" will be reflected in the "student".
Output
Conclusion
- In the javascript function parameter, the terminology related to the function in JavaScript is arguments and parameters.
- The variables listed in the function definition are known as function parameters, and the values supplied as parameters are known as function arguments.
- We can use the default parameter when the arguments are missing when the function is called.
- The function's arguments are stored in a special object called arguments that resembles an array.
- In JavaScript, the pass-by-value creates a copy of the original variable in order to prevent any modifications to the copied variable from affecting the original variable.
- In JavaScript, pass the reference is defined as the real parameter when we pass by reference. There is no memory creation of a copy.