What is a Multidimensional Array in JavaScript?
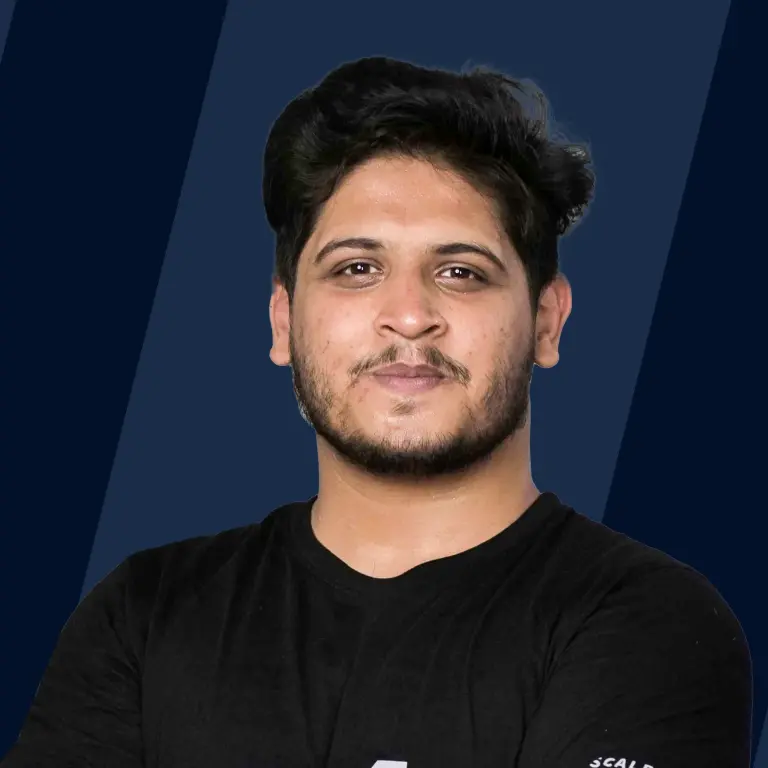
What is a Multidimensional Array in JavaScript?
A multidimensional array in javascript is an array that has one or more nested arrays. A normal array, we know, is a one-dimensional array that can be accessed using a single index, arr[i] where as a two-dimensional array can be accessed using two indices, arr[i][j], Similarly for three-dimensional array arr[i][j][k] and so on.
In simple words, a multidimensional array is known as an array of arrays and It holds a matrix of elements as shown in the image below.
Creating a Multidimensional Array
A multidimensional array is not present natively in JavaScript. Hence there is no direct way to create a multidimensional array in JavaScript. However, We can define an array of elements to create a multi-dimensional array in such a way so that each element stored in an array is also another array.
There are two ways to create a multidimensional array in JavaScript:
- Using array literal: The quickest way to create a Multi-Dimensional Array in JavaScript is to use the array literal notation.
- Using array constructor: Another way is to use an an array constructor. This is done by using the Array() method.
Access Elements of an Array
To access the elements of a 2D array, we use two square brackets where the first square bracket will give you an an array present at the specified row index of the outer array, and the second square bracket is used to access the particular element of the inner array present on the index of the given outer array.
A multidimensional array is accessed in the same way as a one-dimensional array. The only difference is that we have to use two or more indices to access the elements of the multidimensional array in javascript.
Properties of Multi-Dimensional Array in JavaScript
There are some properties of Multi-Dimensional Array in JavaScript:
- length: The length function is used to return the size of the specified array.
- typeof: The typeof operator is used to return the type of the specified array.
- isArray( ): The return type of isArray() function is Boolean. It helps to determine whether the passed array is an array or not.
Add an Element to a Multidimensional Array
To add elements in a multidimensional array in javascript, we can use Array's push() method or use index notation. The push() method will add the element at the end of the given array, whereas the splice() method will add an element at a specified index.
Adding Element to the Outer Array
We can add the Element to the Outer Array as shown below:
Output :
Adding Element to the Inner Array
We can add the Element to the Inner Array as shown below:
Using index notation :
Output :
Using push() method :
Output :
We can add a new element to any inner array or can push an entire array at the end of the array.
Remove an Element from a Multidimensional Array
We can use the pop method to remove elements from a multidimensional array in javascript. The pop method returns an array after removing the last index element of the particular array.
Remove Element from Outer Array
Output :
Remove Element from Inner Array
Output :
Use the splice() Method to Remove an Element at a Specified Index
The splice() method can also be used to remove an element from a specific index in a multidimensional array in javascript.
Output :
Methods in Multi-Dimensional Array in JavaScript
Method () | Description |
---|---|
push() | This method is used to insert an element into the array's last index. As a result, the array length will eventually increase by 1. |
pop() | This method is used to remove the element from the array's last index. As a result, the array length will eventually decrease by 1. |
sort() | Depending on the type of array specified, this method sorts the array elements alphabetically or numerically. The array's order will also be permanently changed by the Sort method. |
reverse() | The reverse ( ) method is used to reverse the elements in an array. Making the last index element the first and the first index element the last is the result of this method. |
indexOf() | This method is used to find the array's first occurrence of a specific element. t will return -1 if the element is not present. |
shift() | Using this method, the first element of the array is removed, and the other elements are moved to the left. |
unshift() | This method is used to shift all the elements in an array to the right while adding a new element to the 0 indexes. |
splice() | Any number of elements can be added or removed from the array using this method. |
Iterating over Multidimensional Array
Using the Array's forEach() Method to Iterate Over the Outer Array Elements
We can use the forEach() method to iterate over the outer array elements of a multidimensional array in javascript.
Output :
Using the Array's forEach() Method to Iterate Over the Inner Array Elements
We can use the forEach() method to iterate over the inner array elements of a multidimensional array in javascript also.
Output:
Use the for Loop to Iterate Over a Multidimensional Array
We can also use the for loop to traverse through the elements of a multidimensional array in javascript.
Output:
20
Pranshu
18
Sameer
Conclusion
- A multidimensional array in javascript is an array that has one or more nested arrays. A normal array we know is a one-dimensional array.
- A one-dimensional array can be accessed using a single index arr[i] where, as a two-dimensional array can be accessed using two indices, arr[i][j]; similarly for a three-dimensional array, arr[i][j][k] and so on.
- In simple words, a multidimensional array in javascript is known as an array of arrays, and a multidimensional array holds a matrix of elements.
- A multidimensional array is not present natively in JavaScript. We can define an array of elements to create a multi-dimensional array in such a way that each element stored in an array is also another array.
- There are two ways to create a multidimensional array in JavaScript: Using array literal and Using array constructor.
- There are various properties and Methods in Multidimensional Array in JavaScript that we have discussed in the article.