How to Use Optional Chaining in JavaScript?
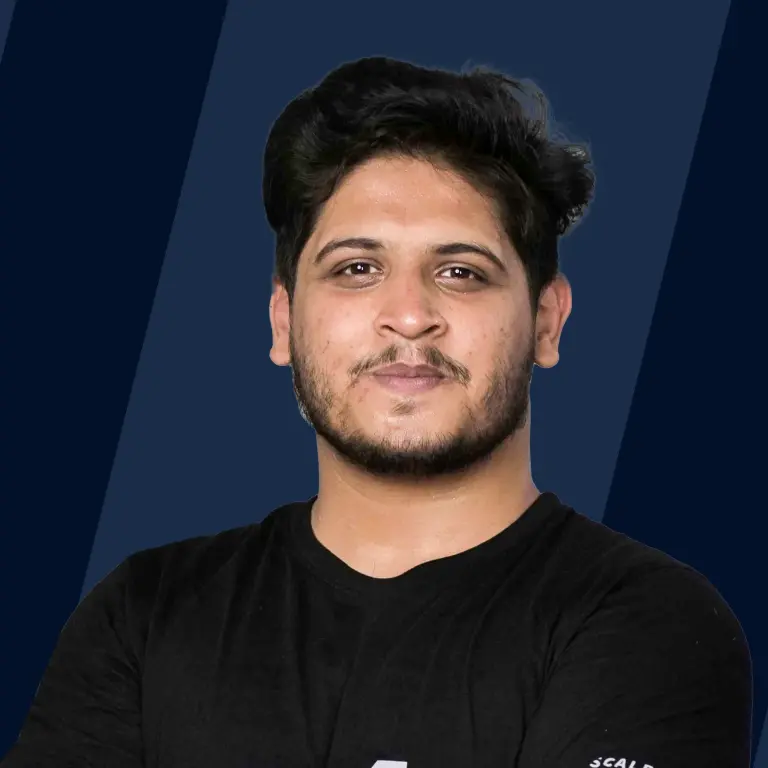
How to Use Optional Chaining in JavaScript?
Javascript optional chaining is used to access nested object properties. Introduced in ES20, Javascript Optional Chaining changes how we access objects.
Suppose there is an object with a lot of nested properties, and we need to access a nested property. One method to do this is to check if each property exists, and then access the property nested below it. To do this in a single line and more efficiently, we can use the Optional Chaining in Javascript. Even if we aren't sure whether some intermediate property exists or not, we can access the nested property using Javascript optional chaining.
Syntax
The Javascript optional chaining parameter ?. is put next to the reference to be checked. If the reference is NULL, the checking will be stopped, and undefined will be returned. Else, the value of the nested property will be accessed. Let us see the syntax below.
The Non-existing Property Problem
An object in Javascript can grow pretty complex pretty soon. Therefore it can get messy to access the inner properties of such objects. We shall see what happens when we try to access a non-existing property or a null property of an object. Let us imagine a student object that contains an address property and each address contains an inner property called postcode.
If we try to access student.Address.PostCode directly without checking its existence, we might get an error. We have to write code that checks the existence of student.Address.PostCode before we use it.
One way to do that is to use if statements as follows:
Imagine if there would have been 50 nested properties. Writing the above if statement would soon get tedious. In such cases Javascript optional chaining comes to the rescue.
Let us see how we can re-write the above condition in a single line using Optional Chaining.
Thus, using Optional Check we did not have to explicitly check the existence of the student and Address, before accessing PostCode. If any of the above is NULL or does not exist, Javascript will return undefined instead of throwing an error. Thus the below line of code will throw undefined.
However, the below line will throw an error.
This is because StreetName is a non-existing property in the student object.
Optional Chaining with Function Calls
We can use Javascript Optional Chaining in case of function calls as well. This is useful in cases when some method of an API that we are using is unavailable. Instead of throwing an error, Javascript will return undefined if Optional Chaining is used.
Suppose we have a student object with a homework function that prints something to the console. Here is how we can use Optional Chaining while calling the homework function.
The above code will print "Homework is done" to the console.
Note that in the above example, we know that the student object already exists. Thus we did not use ?. after student. If we would not know the existence of the student object beforehand, we would have to use student?.homework?.().*
Now let us see an example where we try to call a function that does not exist.
The first statement would not throw any error, while the second will return the Type-Error "student.homework is not a function".
Optional Chaining with Expressions
Optional Chaining in Javascript can be used with bracket expressions that evaluate the property name.
Suppose we have a student object with the name Jake. We can access the expression name using Javascript Optional Chaining. Let us see the code snippet for the same.
We can also use Optional Chaining with array indices. Suppose we want to access the element at index 5. The following expression would return the 5th index if the array exists, and is not NULL.
Optional Chaining is Not valid on the left-hand side of an assignment
Optional chaining is invalid on the left-hand side of an assignment, that is we cannot assign a value to it.
Let us understand it with the below code snippet.
The above operations lead to syntax errors as we can't assign value to the Javascript optional chaining operation.
Short-circuiting
Javascript Optional Chaining stops the execution of the expression (returns undefined) if the left side of ?. does not exist or is NULL. The remaining properties would not be accessed anymore. This is known as short-circuiting of the expression.
Example:
The above expression will return undefined since evaluation has already stopped at student.Address. Thus the expression has been short-circuited before student.Address.PostCode was accessed. This is known as Short-Circuiting in Javascript.
Examples
We shall now go through some examples to better understand the applications of Javascript Optional Chaining.
Example 1: Basic Example
In the following example, we shall see a student object with some nested properties. Consider we have a student object with name and address properties.
Since the teacher object does not exist, accessing teacher.Name throws an error. However, if we use Optional Chaining, we just get undefined instead of the error.
Example 2: Dealing with Optional Callbacks or Event Handlers
When callbacks and fetch methods are used from an object, with a destructuring assignment, we might have non-existing values. These values, thus cannot be called functions until they are proven to exist. To prevent this, we can use optional chaining when dealing with callbacks. Sometimes a method might not get called if the optional callback is not defined. To avoid such discrepancies we use the Javascript optional chaining.
Let us see what happens when we do not use optional chaining.
If the error is undefined, an exception will be thrown. We can prevent the error with the help of optional chaining.
Now, an exception will not be thrown even if the error is undefined.
Example 3: Stacking the Optional Chaining Operator
As we have seen above, we can stack the optional chaining operator multiple times. The execution stops if any intermediate parameter has null values.
Suppose we nest our student object with more properties. We introduce the Grades property which contains the grades for certain subjects. We shall now see how we can access the inner properties of Grades and Address by stacking Optional Chaining in Javascript.
As seen in the above code example, we have stacked optional chaining more than once in all of the return statements.
- The first one checks for the Postcode property inside of the address property and returns 123.
- In the second return statement, we try to access the PostCode property inside of the Street property which does not exist. Thus Optional Chaining stops the execution on the Street Property itself and returns undefined.
- In the third return statement we access the Maths property inside of the Grades property and return 82.
- In the fourth statement we access the English property inside of a non-existent Total property. But the error is not thrown since the execution stops at Total property and undefined is returned.
- However in the fifth statement, we do not use Optional Chaining. Hence error is thrown.
Combining with the Nullish Coalescing Operator
The nullish coalescing operator ?? is a logical operator. It returns the left-hand side operand if it is not null or undefined. Else, it returns the right-hand side operand. Javascript Optional Chaining can be combined with the nullish coalescing operator to return default values if the required expression on the left side returns undefined. In the following example, we have a function that returns the Physics grade of a student if it exists, else it returns Grade Unknown.
Browser Compatibility
Javascript Optional Chaining is compatible with all major browsers including:
Column 1 | Column 2 |
---|---|
Chrome | ✔️ |
Edge | ✔️ |
Firefox | ✔️ |
Opera | ✔️ |
It can also be used for other browsers such as:
- Safari
- Chrome Android
- Firefox for Android
- Opera for Android
- Safari on IOS
- Webview Android
- Samsung Internet
- Deno
Conclusion
- We use optional chaining in Javascript to access nested properties of objects.
- It returns undefined if some intermediate property of the object does not exist.
- It solves the problem that occurs due to non-existing properties of objects.
- Optional chaining can be used with function calls, expressions, optional call-backs, etc.
- We can stack multiple optional chaining together.
- We can combine optional chaining with the nullish coalescing operator to return default values if the left side of the operand is undefined.
- We should not overuse optional chaining, and only use it when we are not sure about the existence of the intermediate values. Else it might lead to errors and loopholes in code.