Reverse a Number in Javascript
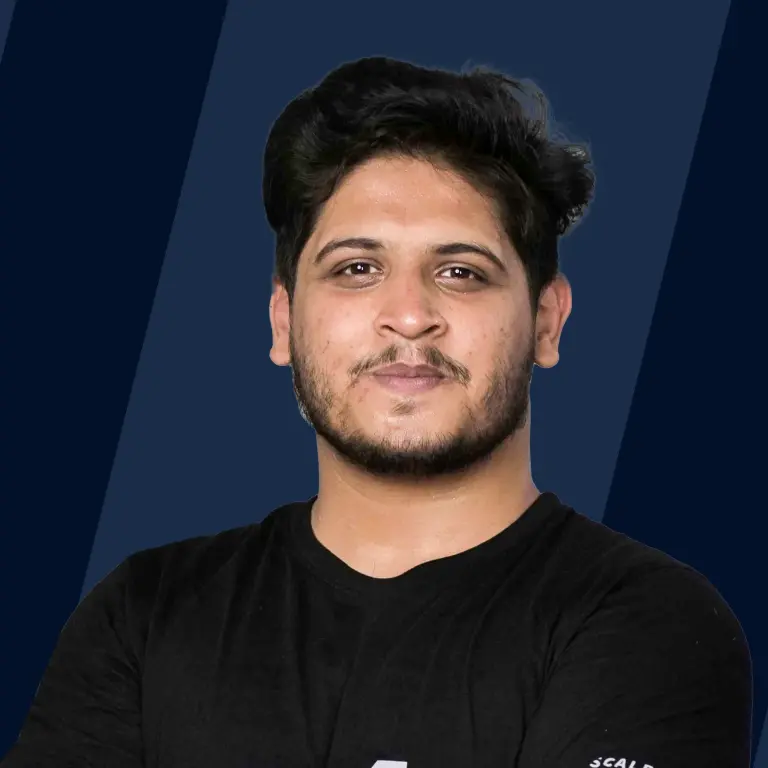
Overview
Reversing a number is a task that everyone might have encountered while coding in any language. In JavaScript, numbers can be reversed by using many approaches. These approaches will be discussed further in this article.
Introduction
We might come across a situation while coding where we have to reverse a number. There are many approaches that can be used to do our task. It may seem tricky, but in actuality, these approaches are pretty simple and can be implemented easily. Let's take a look at the most popular ways of creating new lines with JavaScript.
Algorithm to Reverse a Number
Basically, this approach works on the removal of the last digit of the number, and that last digit can be appended to the right of a new value. So let's see the steps for this algorithm:
- Iterate a loop until the number's value is greater than 0.
- Iterate a loop until the number's value is greater than 0. Set the result variable as 0.
- Use modulo to get the last digit of the number at each iteration.
- Multiply the result by ten and then add the modulo digit to it.
- Replace the number with the floor(number/10) to remove the last digit of the number.
Let's take an example for reversing 12 so for that; we will initialize the result as 0 and start a loop. In the first iteration, we will take the modulo of 12 by 10 and divide 12 by 10 to get the value 2 and add this value to result variable's right, Now the result variable is 2, and the number to reverse is 1. In the next iteration by modulo, we will get 1 and will append the result to make the result variable 21. Hence, the number is reversed.
JavaScript Program to Reverse a Number
Now we know about the algorithm that will be used to reverse the number, now let's apply this algorithm to a JavaScript program:
Example
Output:
By Converting the Number to a String
We can convert the number to a string value, split the string value, and put all of the string's characters in an array, then, reverse the array and finally convert that array to a string by connecting all characters, and then finally convert that string back to a number. In JavaScript, this approach can be completed in only one line using the below 3 functions:
- split() for splitting the characters of the string value and adding them to an array
- reverse() for reversing the array values
- join() for finally joining all the array values to a string
Now let us see the JavaScript program for the above:
Example
Output:
Solving with an Arrow Function
Arrow functions can be used to make the above approach more concise and professional. Also, we can handle the case of reversing a negative number by explicitly checking the sign on the given number.
Now let's see the JavaScript program for the above:
Example
The arrow function makes the code concise and fast.
Output:
Solving with a Regular Function
Let's write a regular function for the above Arrow function that performs the same task as the above one. We have handled the case for reversing a negative number by explicitly checking the sign on the given number.
Now let's see the JavaScript program for the above:
Example
The arrow function makes the code concise and fast.
Output:
Difference Between an Arrow Function & Regular Function
In the above examples, the only difference between the Arrow Function and the Regular Function is that the Regular Function requires a return value, while the Arrow functions have an implicit return value, and the value is returned according to the statements.
Conclusion
- There are many methods that can be used to reverse a number in JavaScript:
- Using the algorithm to reverse a number
- By Converting the number to a string and using the below methods:
- split()
- reverse()
- join()
- Solving with an Arrow Function
- Solving with a Regular Function
- Algorithm works on the removal of the last digit of the number, and that last digit can be appended to the right of a new value.
- The number can be a string value, split the string value, and put all of the string's characters in an array, then reverse the array and finally convert that array to a string by connecting all characters
- Also, the Arrow function and regular function can be made particularly for our task.