Sort an Array of Objects in JavaScript
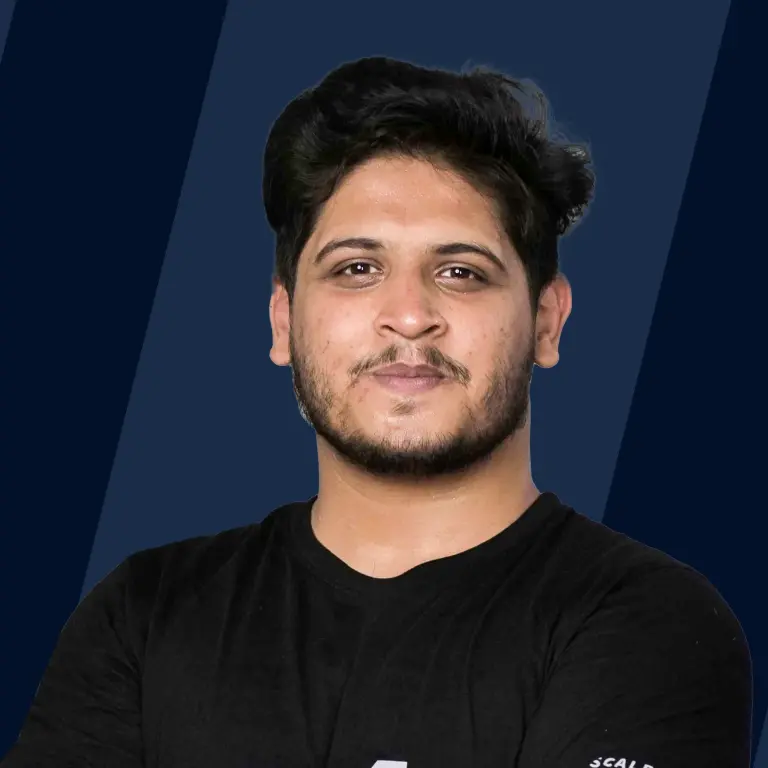
Overview
Sorting refers to the process of arranging the data into a particular order, either increasing or decreasing. We might have sorted different kinds of data in javascript, for example, array, list, map, etc.
Sorting an array of objects in javascript can be done using the regular array.sort() method in javascript and mandatorily passing the compare function, which will determine on what basis our array of objects will be sorted.
Introduction
An array of objects is a collection of multiple objects in an array. We must be aware that an object is an unordered collection of key-value pairs. Hence our array of objects contains key-value pairs, that can be accessed by a '.' (dot) operator.
In JavaScript, arrays come with a built-in function sort(), whose major goal is to sort any element based on alphabetical order.
The sort() method sorts the elements of the array in place and returns the sorted array, by default sorting it in ascending order.
Usually, we can sort an array of objects using the Array.sort function in javascript. The sorting is based on the value of the property that every object has. However, we need to provide the sort() method, a function that defines how our sorting should work, or simply, the sorting mechanism.
Typically, the sorting is based on a value of a property every object has. We can use the Array.sort function, but we need to provide a function that defines our sorting mechanism.
For example, there are multiple blocks of different lengths and widths in the above image. We decide to sort them based on their widths and hence we got that result.
Had we sorted them based on their lengths, we would have got the below result :
Hence, while sorting an array of objects in JavaScript, it majorly depends upon the function we define for sorting our array.
Syntax
The syntax for the sorting of an array of objects in JavaScript is given below :
In the above syntax, you can see that we can sort our array of objects in JavaScript using the normal Arrays.sort() method. Also, here we mandatorily need to pass a function in our sort() method (only in case of an array of objects compare function is mandatory, otherwise it is optional), which will determine our sorting mechanism. Let us understand each parameter taken by the sort function below.
Become a sought-after JavaScript developer with our JavaScript course. Join now and open doors to exciting career opportunities.
Parameters
The Arrays.sort() function takes a comparing function as its parameter. The compareFunction is an optional parameter generally, but when it comes to the array of objects, it becomes vital.
The compare function basically specifies a function that defines the sort order of our array of objects. The compare function can be passed to the sort method in the form of an arrow function, an inline compare function, or a normal function.
Return Value
The sort() method returns a sorted array of objects. Also, the sorting is done in-place. By in-place sorting, we mean that there is no separate copy of the array made after sorting. Hence, it takes a constant amount of space for sorting the array of objects.
Description
We can sort an array of objects in JavaScript by using our built-in Arrays.sort() method, however, we must pass a custom function to our built-in sort method. The function is needed for the comparison of the properties based on which we want to sort our array of objects.
The regular Arrays.sort() method does not work on an array of objects. For example,
In the above image, you can see that when we sort an array of strings using the Arrays.sort() method, it gets easily sorted. But when we try to sort an array of objects.
For example, here we have an array of objects: Flower names and their prices, in that case, our normal Arrays.sort() method does not work. Hence, we need to pass a custom function to our sort method, based on which it will be able to sort our array of objects.
The compare Function
To sort an array of objects in JavaScript, use the sort() method with a compare function. A compare function helps us to write our logic in the sorting of the array of objects. They allow us to sort arrays of objects by strings, integers, dates, or any other custom property.
The compare function returns a negative, zero, or positive value, depending on the arguments passed to it.
When the sort() function compares two values, it sends the values to the compare function, and sorts the values according to the returned (negative, zero, positive) value by the compare function. Let us see, when a compare function returns a particular value :
- If the compare function returns a negative value, then x is kept before y in the sorted result.
- Else if, the compare function returns a positive value, then y is kept before x in the sorted result
- Else, if the compare function returns a zero, there are no swaps done between x and y into the sorted result, and their order remains the same.
Essentially, it can be visualized as below :
Creating, Displaying, and Sorting an Array
Let us create, display, and then sorts an array or an object based on its value. We will also see how to write the sorting function.
Code :
Output :
Explanation :
In the above example, we defined an array of objects that consisted of an array of products containing different products, their types, manufacture date,s, and prices. To sort them, we wrote our custom function in the inbuilt sort method of javascript, as given below :
Code :
Output :
The Array.sort compares any two objects (prices here) and if the price of any product is less than the price of another product, it returns a "positive value", resulting in sorting them in descending order. In case, the price of the given product is less,it returns a negative value. If the price of both the products is equal, it returns 0, signifying that there is no need to swap those objects because their prices are necessarily the same.
Note: We should handle the case of zero, when the value of both the objects is the same, to avoid unnecessary swaps.
Now, let us also handle a case where we sort our product array based on the manufacture date in ascending order.
Code:
Output :
Explanation :
In the above example, we have tried to sort our array based on the manufacture date, in ascending order. We have written our custom function, which compares two dates and returns a positive value, negative value, or a zero-based on the dates. Likewise, we sort the complete array of objects based on this property.
Sort an Array of Objects by Numbers
Let us create an array of objects and sort it based on the numbers. Below given is an example to depict the same.
Code :
Output :
Explanation :
In the above example, we sort an array of objects, that is, the array students here, based on the property "roll number". We create our custom function, which takes roll numbers as parameters and compares them.
Let us look at an even simpler way to sort our array of students, based on their roll numbers :
Code :
Output :
Explanation:
In the above code, we are just calculating the differences between each roll. By doing this, we get 3 different values based on which we can perform our sorting.
Say we are comparing two roll numbers and . If our compare function returns a value that is less than 0, a will appear before . If it is greater than 0, will appear before . If it’s exactly , then the original order will be maintained.
Sort an Array of Objects by Strings
Let us now take an array of objects and sort it based on the string values present in the array.
Below, the following code snippet depicts how can we sort the names of the students by their first name, in an ascending order and case-insensitively :
Code:
Output :
Explanation:
In the above example, we sorted the names of the students in descending order. For that, we wrote our inline custom compare function. In that function, we compared the names of the students. But firstly, we converted them into uppercase to perform a case-insensitive sort.
Now, depending upon the value our search function returns, we will sort our array. As we have already seen, it can return positive, negative, and zero values.
Displaying in descending order :
To display the names in a descending order, we just reverse the order of our comparison. Let us look at the below code for a clear understanding.
Code:
Output:
Explanation:
By just reversing the order of our comparison operators, we can sort our data in descending order. Please refer to how the sort function works on different return values of the compare function.
Displaying data using a forEach loop:
You might have noticed, that we are using the "console.log" statements to log our output. Let us also learn to display the data using forEach loop into a particular format.
Code:
Output:
Explanation :
In the above example, we have used the forEach loop to display the student name and roll number.
Sort an Array of Objects by Dates
Suppose that we have an array of student objects as given in the code example below. We will try to sort it out based on their registration date. Let us learn how to sort an array of objects by date.
To sort an array of objects by date strings, all we need to do is, provide a compare function that parses the date string first, using the new Date() constructor, and subtracting them from each other.
The following code illustrates the main concept of sorting by date in an array of objects :
Code :
Output :
Explanation:
In the above example, we are sorting our array of objects in ascending order, based on the registration date values. To sort them, we fill them with dummy dates and then sort them by comparing two dates in our custom inline sort function.
For example, the below piece of code will be used for sorting our dates :
Code:
Output :
Here we are taking 2 strings (which are dates) and creating them into dates using the new Date() constructor. After that, we find out the difference between the two dates, and based on the return value, that is, either positive, negative, or zero return value, we are sorting the dates.
Sort Stability
In general, the stable sorting algorithms are the ones, that maintain the relative order or position of the sorting of the items. In simple words, stable sorting algorithms maintain the position of two equal elements relative to one another.
Array.sort is stable according to the specifications, since ES or version . Before that, the sorting stability was not guaranteed.
For example, say we have an array of players, where each player has a name and a score.
Now, if we sort the players in descending order of their scores, we will see that the players with the same scores will maintain their relative positions. For example, we sort the above player object in the code given below.
After that, we will display our data using the forEach loop in javascript.
Code:
Finally, we will get the below output :
Output:
Please note, in the above output, the players Jon and Ron have the same scores, and the order of Jon coming before Ron is maintained. This is what stable sort guarantees in general.
Before version 10 (or EcmaScript ), sort stability was not guaranteed, which means, we could also get some output as shown below :
Output :
Conclusion
- An array of objects is a collection of multiple objects into an array. The objects can contain a key-value pair and have properties and values.
- We can sort the array of objects using the sort() method in javascript and then provide a comparison function that will ultimately determine the order of the objects.
- A compare Function applies rules to sort arrays that are defined by our logic.
- Compare Function allows us to sort arrays of objects by strings, integers, dates, or any other custom property.
- We can sort an array of objects on the property string by comparing them and returning -1, 1, and 0, depending on the string comparison.
- To sort an array of objects by date, we can convert the joined dates from strings to date objects. And then sorting our array based on dates.
- Similarly, we can sort the numbers in an array of objects by calculating the difference between them and returning positive, negative, or zero values. Based on that, our order of sorts will be defined.
- Array.sort() is stable since version or ES .