What is JavaScript String Format?
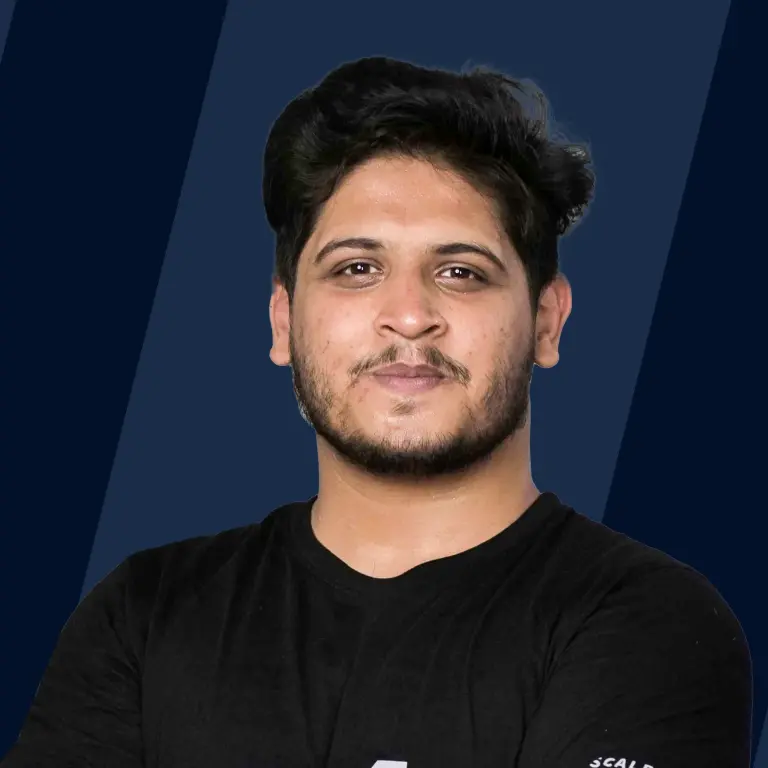
Strings in JavaScript are defined as a series of characters wrapped within double or single quotes and are indexed from zero. The first character of the string is at index 0, the second at index 1, and so on. In simple language string formatting means inserting variables into a string. The variables that are inserted into the string are called placeholders. These placeholders later get replaced by the actual values of these variables.
JavaScript allows us to format a string with variables and expressions from our code in three different ways which are as follows:
- By concatenation i.e. inserting variables into the string using the + operator.
- By string interpolation i.e by using template literals (strings wrapped within backticks) and ${variable/expression} as placeholders.
- By defining a custom function ourselves.
Normally strings are either wrapped in single quotes or double quotes.
Here since 'Scaler Topics' is a simple string so we can't use any methods and properties on it. But a variable in JavaScript is treated as an object, and thus it has access to the methods and properties.
Methods Of JavaScript String
There are different methods of the javascript string which are listed below:
-
charAt(): This method returns the character present at the specified position, location, or index.
Syntax:
Here, the index parameter is optional. By default, its value is 0. It returns an empty string if the index is out of range.
Example:
Output:
-
charCodeAt(): This method returns the Unicode(ASCII standard) of the character present at the specified position, location, or index in the form of an integer ranging from 0 to 65535.
Syntax:
Here index parameter is optional with the default value as 0. If the index is invalid then it returns NaN
Example:
Output:
-
concat(): This method is used to join two or more two strings. It does not change the original string i.e. it returns a new string.
Syntax:
Example:
Output:
-
endsWith(): This is a case-sensitive method. It returns a boolean value. If the string ends with the specified string value it returns true else it returns false.
Syntax:
Here, searchvalue is a required parameter while, length is an optional parameter that returns the length of the string which is to be searched.
Example:
Output:
-
fromCharCode(): This method changes the Unicode values to their respective characters.
Syntax
Example:
Output:
-
includes(): This method checks whether a specified character or string is present in the string and returns true if present else, return false. This method is case-sensitive.
Syntax:
Here searchvalue is a required parameter while, start is an optional parameter that describes the position from where you want to start the search. By default, its value is 0.
Example:
Output
-
indexOf(): This method finds a specified character and returns the index of its first-time occurrence. If the character is not found then it returns -1. This method is case-sensitive.
Syntax
Here, start is an optional parameter and by default, its value is 0.
Example:
Output:
-
lastIndexOf(): This method searches the specified character and returns the index of its last occurrence. It searches the character from the end to the start. If the character is not found then it returns -1. This method is case-sensitive.
Syntax
Here, start is an optional parameter and by default, its value is 0.
Example:
Output:
-
localeCompare(): This method compares two strings in the current locale (depends on the browser's language settings). This method returns -1 if the string is sorted before the compareString, 1 if the string is sorted after the compareString, and 0 if the two strings are equal.
Syntax
Example:
Output:
-
match(): This method matches the string against a regular expression. If the value to be matched is a string then it is converted to a regular expression. This method returns an array with all the matches and returns null if no match is found.
Syntax
Example:
Here, /g refers to global search, /i means case insensitive search, and, /gi means global search with case insensitivity.
Output:
-
repeat(): This method takes count as a parameter and returns the copies of the original string. The method does not change the original string.
Syntax:
Example:
Output:
-
replace(): This method searches the complete string for the specified value and replaces it with the newly mentioned value or an expression. This method does not change the original string instead it returns a new modified string.
Example:
Output:
-
search(): This method searches the complete string for a particular value/expression and returns the position of its first occurrence. This method is case-sensitive. If no match is found it returns -1.
Syntax
Example:
Output:
-
slice(): This method extract a specified part of the string and returns it. It does not change the original string. The start parameter specifies the starting position and the end parameter and end parameters specify the ending position(not including) of the string which is to be extracted. To select the string from the end we must give a negative number.
Syntax
Example:
Output:
-
split(): This method is used to split the string into an array of substrings. This method does not change the original string. This method takes two optional parameters. The separator parameter is an expression or a string for splitting. The limit parameter takes an integer that specifies the limit of the number of splits.
Syntax
Example:
Output:
-
startsWith(): This method checks whether the string starts with the specified characters or not. If so then it returns true, else it returns false. This is a case-sensitive method. It takes two parameters where searchValue is a required parameter which is the string you want to search for, and the start parameter is optional which is defined as the position to start the search from. By default, its value is 0.
Syntax
Example
Output:
-
substr(): This method takes the starting index and length of the characters which are to be extracted from the given string and returns the extracted string. It does not change the original string. Here, length is an optional parameter, if not given then it extracts the rest of the string.
Syntax
Example:
Output:
-
substring(): This method takes the starting and the ending index of the characters which are to be extracted from the given string and returns the extracted string. This method returns the substring and does not changes the original string. Here, the end parameter is optional, if not given then it extracts the rest of the string.
Syntax
Example:
Output:
-
toLocaleLowerCase(): This method converts the string to lowercase based on the current locale. The current locale is based on the browser's language setting. This method does not change the original string.
Syntax
Example:
Output:
-
toLocaleUpperCase(): This method converts the string to upper case based on the current locale. This method does not change the original string.
Syntax
Example:
Output:
-
toLowerCase(): This method is used to convert the string to lowercase characters without changing the original string.
Syntax
Example:
Output:
-
toUpperCase(): This method is used to convert the string uppercase characters without changing the original string.
Syntax
Example
Output:
-
trim(): This method is used to remove spaces from both sides/ends of the string. This method does not change the original string.
Syntax
Example:
Output:
-
valueOf(): This method is used to return the primitive value of the string without changing the original string. This is the default method of JavaScript stings and is thus used internally by JavaScript.
Syntax
Example:
Output:
Ways to Format a String in Javascript
JavaScript does not provide any built-in string formatting function. But there are three ways to format strings in JavaScript which are as follows:
- By using ${} brackets within backticks ``
- By using the plus (+) operator
- By creating a format function
Let us see the above methods in detail.
By using ${} brackets within backticks ``
This is one of the most convenient methods to format strings in JavaScript. In this method, the strings are simply wrapped within the backticks ( `` ) and the variables to be inserted are wrapped within curly braces ( {} ) preceded by a dollar sign ($). This is also called template literals or template strings.
Here’s a basic code example of the template literal to understand better:
Here, the text wrapped within the curly braces i.e yourName and college are variables that act as placeholders that get replaced by their original value.
Output:
Here, we can see in the output string that the variable which was acting as a placeholder got replaced by its value. Use this JavaScript Compiler to compile your code.
Remember: While using template literals make sure to wrap the string within backticks only and not in single quotes ('text') or double quotes ("text").
Apart from normal variables, we can also use any javascript expression or functions within these backticks.
String formatting in JavaScript is easier with template literals (introduced in ES6) as compared to other string formatting methods.
Template literals make the strings more readable and cleaner.
Let us see some more uses of template literals over other methods:
- It also allows us to create multi-line strings. Before the introduction of template literals for new lines, we used \n which looked confusing for multiple lines.
Output:
This looks so clean whereas, it would have looked so confusing and complex doing it with a single quote or double quotes.
String interpolation also allows us to use a ternary operator inside a string which facilitates us to check the value of a particular variable, and show different outputs based on those values.
Let's look at the example below:
Output:
In the above example 1, we are calculating the total marks, and based on the total marks we are showing different outputs.
If the amount is more than ten, we let the user know that the card payment will be declined. Otherwise, the card payment is accepted. As you can see, string interpolation allows us to do cool things with strings.
Thus, we can conclude that template literals allow us to:
- write multi-line strings
- use expressions/functions
- use ternary operator within strings
Format String Using Plus (+) Operator
The plus (+) operator when used with JavaScript strings is treated as a concatenation operator. The plus operator when used with string, joins/ concatenates variables and strings. Thus this method is also called string concatenation in JavaScript.
Let us see a basic example to understand how the plus operator is used in string concatenation:
Output:
Here, the variable is replaced by its value and concatenated with the string.
Variables, functions, and ternary operators can even be used with the plus operator but it is not recommended as looks quite confusing to read with single quotes and double quotes, and also it might sometimes give unexpected results when not used in acorrect format.
Let us see some examples with unexpected results:
Now let us see the correct way to write the above code snippets (although not recommended):
Thus we can conclude that string concatenation using the + operator can sometimes produce unexpected results for complex expressions and ternary conditions so we should avoid using it.
Javascript String Format Function
Unlike JavaScript, many other programming languages have built-in functions to format the strings like python. Python has a built-in method called format() to format the strings. The format method accepts a list of parameters and replaces the placeholders in the strings with the parameters i.e. the value of the placeholders/ variables. The placeholders are the integer values starting from 0 (first placeholder) till n (last placeholder) wrapped inside curly braces ({}).
Example of python code using format function:
Expected output:
We can create a similar function in JavaScript as well.
Example of formatting JavaScript string with a custom function
Output:
In the above example, {0} is replaced by the value of the first parameter i.e. yourName and {1} is replaced by the value of the second parameter i.e. college.
So thus, we have created a custom format() method in JavaScript which searches the template numbers ( {0}, {1}, {2} .... so on) and replaces them with the arguments that we passed.
Note: Although the above function works well, it is not recommended to use a custom function for the prototype object. Instead, we can directly use template literals.
Embrace the power of JavaScript and build a career you're passionate about. Enroll in our certification course and open up new possibilities.
Examples
Javascript String Format Example
Let us see some more examples of string formatting in JavaScript:
Using template literals with JavaScript expressions:
Output:
JavaScript String Methods
Let us see some examples of the most commonly used string methods in JavaScript:
Output:
Example of String Comparison
Let us see the example of string comparison in JavaScript:
Output:
Conclusion
- A group of characters wrapped inside single or double quotes is known as strings in JavaScript.
- Injecting variables into a string is known as string formatting in JavaScript.
- There are three ways of string formatting in JavaScript:
-
- By using the + operator
-
- By using template literals
-
- By using a custom format function
- Template literals were introduced in ES6 and are so far the most recommended method for string formatting.
- Apart from writing cleaner code, template literals also allows us to embed expressions and functions into the string.
- JavaScript also provides us various string method like lastIndexOf(), toUpperCase() etc.