JavaScript Data Types
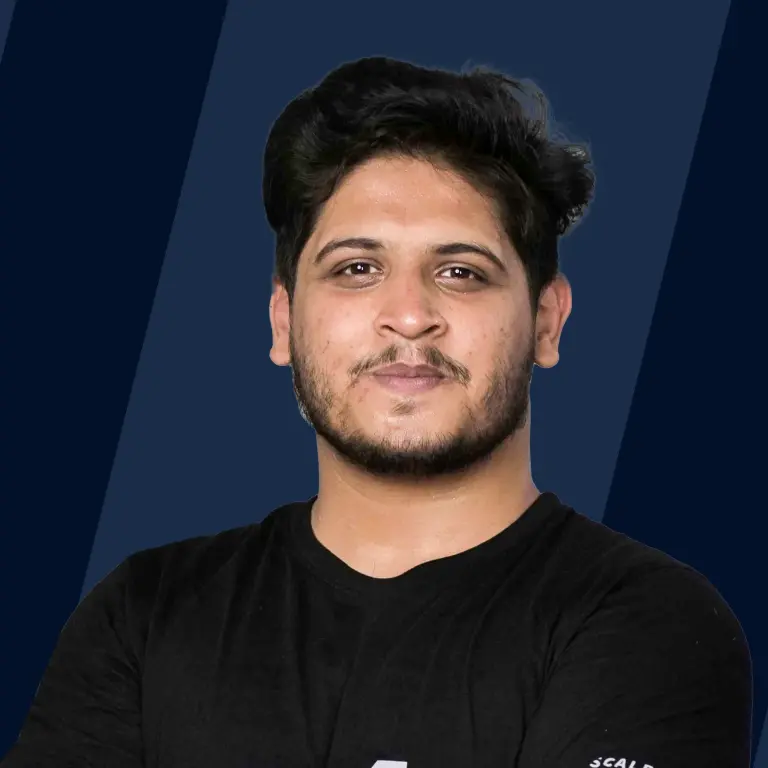
Overview
Every day we encounter data in our usual chores. Be it the price of groceries or writing our names on documents, all of these involve data. An important attribute of these data elements is that it has a particular type. The price or amount of grocery items is numbers whereas the names are texts or strings. This data type acts as an identity for the element. Assigning a specific type to the data helps us in operating it smoothly.
Similarly, in JavaScript, we classify the data into multiple types in order to function more smoothly and precisely.
What are Data Types in JavaScript?
Data types in JavaScript refer to the attribute associated with the kind of data we are storing or working on. It associates the declared variable (or item) with a particular type so that the compiler can perform operations in an organized manner.
There are mainly two types of data types in JavaScript:
- Primitive data types.
- Non-primitive data types.
Primitive Data Types in JavaScript
Primitive data types in javascript refer to those data types that are defined on the most basic level of the language. These Javascript data types cannot be destructured further, nor do they have any pre-defined properties or methods. They are only used to store items of their type.
There are 7 primitive data types in JavaScript:
- Numbers
- BigInt
- String
- Boolean
- Undefined
- Null
- Symbol
Following is the table for all the primitive data types in javascript with their descriptions and examples.
Data Type | Description | Example |
---|---|---|
undefined | Represents an undefined value. | let x; // x is undefined |
null | Represents an null value. | let y = null; // y is null |
boolean | Represents a boolean value, which can be true or false | let a = true; // a is true |
number | Represents a numeric value, either integer or floating point. | let b = 5; // b is 5 |
string | Represents a sequence of characters, enclosed in quotes. | let c = "hello"; // c is "hello" |
symbol | Represents a unique identifier, used primarily for object properties. | let d = Symbol("description"); // d is a unique symbol |
BigInt | Represents integers with arbitrary precision. | let e = 9007199254740992n; // e is a BigInt |
Numbers Type
Everyday, we encounter numbers in multiple forms. Be it sharing our phone number with someone or calculating the square root of a value in a maths problem, all these include numbers in integer, decimal, fraction, or any other form.
In javascript, the integers, float values (or decimals), and all the other numeric values are represented by the number datatype.
Unlike other languages (like C++), javascript doesn't define separate data types for integers, decimals, long integers, etc.
Declaration:
Numbers can be declared by using var, let, or const keyword followed by the variable name, and the number is assigned to it.
Syntax:
Example:
- Note: The Javascript number type is a double-precision 64-bit binary format, i.e., it occupies 64 bits and ranges between a dynamic range to .
- The numbers that overflow this range are handled by BigInt datatype.
Due to the existence of this range, there is an upper limit as well as a lower limit to storing numbers in javascript. Also, there are certain keywords related to numbers in javascript. Following are some of these keywords related to numbers in javascript:
Largest safe Integer: It represents the largest integer value that can be represented by javascript without any overflow.
Representation: Number.MAX_SAFE_INTEGER
Value: 253 - 1 or 9007199254740991
Largest value: Represents the largest possible value that can be stored by javascript.
Representation: Number.MAX_VALUE
Value: 21024 - 1
Note: The largest safe integer refers to the largest value that can be represented while largest value refers to the largest value that can be stored.
Smallest value: It represents the smallest positive value in javascript.
Representation: Number.MIN_VALUE
value: 2-1074 or 5e-324
Epsilon: It represents the difference between 1 and the least number greater than 1.
Representation: Number.EPSILON
value: 2-52
Infinity: It is a symbolic value that occurs in the case of an overflow.
To check whether a number has reached infinity (or -infinity): Number.isFinite
NaN: It represents anything that is not a number in javascript. The NaN case usually occurs during type conversion of the number or due to its concatenation with a string.
Number.isNaN can be used to verify whether a number is NaN or not.
BigInt Type
We often encounter cases, where the number to be represented, is really large, for example, the population of the world or the total number of stars in the sky. Though it is possible to represent these huge numbers on paper certain data types come with memory constraints.
The BigInt data type in javascript is used to represent numeric values that exceed the largest safe integer limit. It is basically used to represent numbers greater than .
Note: Unlike number, the BigInt data type doesn't represent decimal values. It only represents whole numbers.
Declaration:
BigInt values can be declared by two methods:
- By appending n at the end of the numeric value.
- By passing the number as an argument to the BigInt() constructor.
-
By appending n at the end of the numeric value:
BigInt can be declared by appending n at the end of the numeric value that we are assigning to our variable.
Syntax:
-
By passing the number as an argument to the BigInt() constructor.
BigInt can be declared by calling the BinInt constructor and passing the numeric value as an argument. We do not need to append n at the end of the argument.
Note: the new keyword is not used while calling the BigInt() constructor.
Syntax:
Example:
In the last example, the argument passed is a string. In the constructor calling method, we can even pass the string of a number, and it would return a BigInt number.
The output of the BigInt numbers will always be appended with n.
Example:
The BigInt operator acts as Number with +, -, *, **, % operators but acts as a boolean entity with if, ||, &&, Boolean, ! operators.
String Type
We often encounter elements that are presented in a textual manner, be it our name or a paragraph in the textbook. These elements are different from the numbers and have specific operations and constraints. In javascript, these kinds of texts are represented by strings.
String type is used to store elements in a textual form. The elements are stored in 16-bit integer form.
Declaration:
Strings are declared by assigning the text to the declared literals. The literal can be declared by either const, var, or let. In Javascript, a string can be declared in the following three ways:
-
Single tick declaration:
Strings are declared by enclosing the text between two single ticks ('')
Syntax:
-
Double tick declaration:
Strings are declared by enclosing the text between two double ticks ("")
Syntax:
-
Backticks declaration:
Strings are declared by enclosing the text between two backticks ticks (``)
Syntax:
Example:
String properties:
The string type has various properties that assist in its operations. Following are some of the properties of strings:
Indexing:
Strings in javascript follow 0-based indexing, i.e., the first element of the string is considered at the 0th position, the second in the 1st position, and so on.
Example:
Thus, in order to access the elements of the string, we need to provide the string name follower by a square bracket ([]) and its index inside it.
Immutability:
In javascript, once the string is created, it cannot be further manipulated.
Example:
In the above example, the 'Scaler' string wouldn't change as the reference string object is immutable.
Although we can assign a new string to an existing string object.
Concatenation:
We can concatenate two strings using the + operator.
Syntax:
Note: In strings that are declared using backticks we can include external variables using ${}
Example:
Boolean Type
We often encounter situations where there are two possible values, either true or false. For example, imagine logging into your social media account; the website takes your password and compares it with the one stored in its database. If it matches, i.e., the result of the comparison is true, it proceeds; otherwise, it terminates.
In javascript, the boolean data type is used to check the truthy or falsy condition. It is also known as logical data type.
Declaration:
It can be declared by using the bool keyword followed by the variable name. It can be assigned only two values, true or false.
Syntax:
The boolean data type is generally used in conditional statements or at places where authentication is required.
Example:
Note: The statement flag is equivalent to flag == true, similarly !flag is equivalent to flag == false
Undefined Type
Sometimes, we encounter things that are obscure. For eg, the mode of communication of a recently discovered reptile in the amazon forests. Thus it cannot be put under any pre-defined set. In that case, the mode of communication remains undiscovered or undefined.
In javascript, undefined is a primitive data type in javascript that gets assigned to variables or function arguments when their values aren't initialized.
Declaration:
undefined data types in JavaScipt can be declared by simply declaring a literal by using var, let, or const keyword. We don't assign anything to the literal.
Syntax:
Example:
In the above example, the first function call will give out the output as undefined as the argument that is passed has been declared but not assigned any value.
Note: Even if we call the function without passing ay value ti would output undefined as the parameter isn't assigned any value.
Null Type
We often encounter certain elements that do not hold any value, for e.g., as simple as the no signal message on the TV during rains to the vacuum in the space. These elements do not hold any value. In Javascript, these kinds of values are represented by null.
The null type in javascript refers to an empty object pointer. It holds only one value. It is used to represent intentional absence of any object.
Declaration: It is declared by declaring a variable using var, let or const keyword and it is assigned the value null.
Syntax:
Example:
In the above example the output of b is null as the argument passed is a null value.
If no value is passed to the function as parameter, then the output will be undefined only.
null properties:
-
null is considered as falsy:
Which means that under conditional situaltion null will return false result.
-
The typeof null is object.
Symbol Type
The symbol is a primitive data type in javascript that returns a unique symbol upon being called. Symbols do not have a literal form and are unique.
Declaration:
The symbol is declared by calling the Symbol() function. The parameter field doesn't default; thus it can be called either by passing an argument or empty.
Note: the Symbol() isn't a constructor thus, if it is called with the new keyword, it will throw an error.
Syntax:
The Symbol() creates a new symbol everytime it is called and the symbol has nothing to do with the key passed.
Example:
In the above example, even though the key passed is same still the symbols s1 and s2 will be different.
Non-primitive Data Types in JavaScript
Non-primitive data types are those data types that aren't defined at a basic level but are complex data types formed upon operations with the primitive data types. The non-primitive data types in javascript refer to objects and can perform multiple functions using their methods.
There are mainly 3 types of non-primitive (or complex) data types in JavaScript:
- Object
- Array
- RegExp
Following is the table for all the non-primitive data types in javascript with their descriptions and examples.
Data Type | Description | Example |
---|---|---|
object | Represents a collection of key-value pairs, or properties, where each key is a string and each value can be any data type, including other objects. | let obj = {name: "John", age: 30}; // obj is an objectd |
array | Represents a collection of elements, where each element can be any data type, including other arrays and objects. Arrays are also objects in JavaScript. | let arr = [1, 2, "three"]; // arr is an array |
RegExp | Represents a regular expression, which is a pattern used to match character combinations in strings. | let pattern = /[a-z]+/; // pattern is a regular expression |
Object Type
During school, all of us were provided with identity cards. This used to contain fields like our name, class, section, roll no, etc. These were used to identify students according to multiple attributes. In reality, we often encounter such situations where we need to associate one identity with different attributes. In javascript, such identities are stored using objects
Object in javascript is a collection of data that is kept in a key-value pair manner.
Declaration:
Objects in javascript can be created by two methods:
-
Literal method:
In this method, a literal is created using var, let or const keyword, and we assign a set of key-value pairs to it, which is wrapped inside braces {}.
Syntax:
-
By calling the constructor:
In this method we can declare the object by calling Object() constructor using a new keyword and then add the key-value pair using the . operator.
Syntax:
Example:
In a literal declaration, the key-value pair is separated by commas ,
Two keys can not be the same in an object.
Accessing objects items:
Object items can be accessed by two ways:
-
By using dot operator:
Object items can be accessed by calling the object name followed by a dot operator followed by the key name.
Syntax:
example:
-
By array method:
Objects can also be accessed by the array method. In this method, we state the name of the object and pass the key inside [] as a string.
Syntax:
Example:
Using functions as a key-value:
Inside the objects, functions can also be stored as a value for a key.
Declaration: Inside the object definition, the function is declared after the key.
Syntax:
Example:
note: Here, the this keyword refers to the parent object.
Array Type
All of us have made to-do lists (though we never complete the tasks mentioned on it ). We keep adding or removing tasks on it. Similarly, we do often use to store a collection of items under one hood. For example, the grocery list or semester scores. In javascript, we do use containers to store these kinds of things.
Arrays in javascript are abstract data types that are used to store a set of elements. These can be multiple elements of the same or different types. Arrays are basically containers in javascript.
Declaration:
Javascript arrays can be declared by three methods:
-
By array literal:
In this method, arrays are declared using const, let, or var keywords, and the values are assigned to them using [].
Syntax:
-
Constructor method:
Arrays can be declared by calling Array() constructor using the new keyword.
Syntax:
Example:
Note: In javascript, the elements of an array can be of different types
Example:
Array properties:
Following are some important properties of an array:
- Indexing
The array in javascript follows 0 based indexing, i.e. the first element is considered at index-0, second at index-1 and so on.
Example:
In the above example, the ar[0] outputs Red as it is in the 0th position, similarly, ar[2] outputs Orange as it is in the 2nd position.
-
Accessing elements of an array:
In order to access elements of an array, we need to provide the array name followed by its index inside the bracket.
Syntax:
Example:
-
Array methods:
The javascript arrays have several methods that are used to manipulate the array and produce desired results. Following are some common array methods:
-
Push:
To add elements to the end of an array.
Syntax:
Parameter: The element to be pushed return type: An array with the element added to the end of the array.
-
Pop:
To remove elements from the end of the array.
Syntax:
Parameter: None return type: An array with the element from the end of the array.
-
isArray():
Checks if the passed object is an array.
Syntax:
Parameter: The object that has to be checked. return type: Returns true if the parameter is an array; otherwise, it returns false.
-
forEach()
It is used to invoke the specified function for each element. It can be used to iterate through each element as well.
Syntax:
Parameter: A function that needs to be operated. return type: The function's response on each element.
-
There are several other operations that can be done on the array using map(), reverse() etc.
RegExp Type
We all have seen detective movies; remember how words are decoded and read using patterns? Even in real life, we encounter many instances where we need to validate the texts with particular patterns.
The RegExp in javascript is an object which is used to match a string with a particular pattern.
Declaration:
Regular Expressions (RegExp) can be created by two methods:
-
By using forward slashes:
In this method, we declare literals and then assign them the pattern which follows a forward slash, and it is followed by a modifier.
Syntax:
-
By Constructor:
Regular expressions can be declared by calling RegExp() constructors by passing the pattern as a parameter.
Syntax:
Note: The parameter is passed as a string.
Example:
Here p1, and p2 are regular expressions for javascript.
Here the /javascript/ part is the RegExp and i is the modifier.
RegExp modifiers:
Modifiers are added after the declaration of RegExp. Their task is to modify the search operation according to their property.
Following are some common modifiers in Javascript:
- g: It is added to make sure the RegExp doesn't stop after finding the first match. It performs global matches.
- i: It performs a case-insensitive matching.
- m: It performs multiline matching.
Conclusion
-
The Javascript data types are used to classify the data into different forms in order to use it properly.
-
There are mainly two types of data types in javascript:
- Primitive data type.
- Non-primitive data type.
-
The primitive data types in Javascript are those which are defined at the basic level and cannot be destructured further, whereas non-primitive data types in javascript are those that can be manipulated using methods and can create objects.
-
Numbers, BigInt, String, Boolean, Undefined, Null, Symbol are examples of primitive data types in javascript.
-
Objects, array, and RegExp are examples of non-primitive data types in javascript.