Difference Between == and === in Javascript
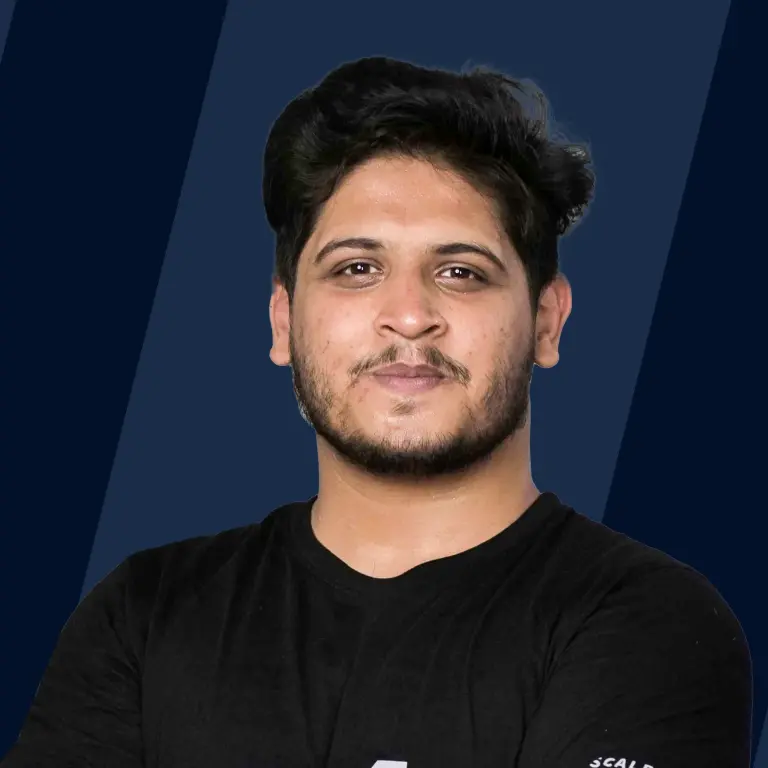
Overview
The equality operator in javascript is used to compare if two values are equal. The comparison is made by == and === operators in javascript. The main difference between the == and === operator in javascript is that the == operator does the type conversion of the operands before comparison, whereas the === operator compares the values as well as the data types of the operands.
Introduction
In daily life, we encounter multiple situations where we need to compare two things. For example, imagine logging into your Instagram. Upon visiting the site, you'll see a login page asking for your username and password. Once you submit the details, the website goes through its database and compares the provided details with the details available. If the details match, it allows you to log in; otherwise, it doesn't.
This is one of the many instances where two objects are compared, and further action is decided upon the result.
In Javascript, to compare two values, we use comparison operators. There exists a special case of comparison where two values are compared and decided whether the values are equal (or unequal). In that case, we can use the following two operators in javascript:
- == operator.
- === operator.
Note: To check if two values are unequal, we replace the first equal ( = ) sign with an exclamation ( ! ) mark. Thus the != and !== operators, respectively, are used to check the inequality of two values.
Before getting onto the Difference between == and === in Javascript, let's see what are == and !== operators in javascript.
What are == and != in JavaScript?
The == and != operators in javascript are used to compare two values. The == operator checks if two values are equal. The != operator checks if two values are not equal. It is also known as the loose equality operator because it checks abstract equality, i.e., it tends to convert the data types of operands in order to carry the comparison when two operands aren't of the same data type.
Syntax:
For equality:
For inequality:
Where x and y are operands, and the middle portion is for the operator. In the case of equality comparison, we use == operator, and in the case of inequality comparison, we use != operator.
Return type: boolean
It either returns true or false.
The == operator returns true if both operands are of the same data type and have the same value or if both are of different data types, but either of them can be converted to the data type of the other operand and have the same value. If both operands have different values, then it returns false.
The != operator returns true if both operands have the same data type and different values or if both have a different data type and none of them can be compared to the other operand's type. It returns false if both operands are of the same data type and have the same value or if both are of different data types, but either of them can be converted to the data type of the other operand and have the same value.
Note: The == or != operator does type conversion of elements before comparing them.
What is type conversion?
The == and != operators loosely compare two operands, i.e., while comparing two operands, if both operands aren't of the same data type, then the operator tends to convert either of them into other operand's type and then compares their values.
This is one of the most important difference between == and === in Javascript
Example:
If we are about to compare two values, one string, and another number, then it will convert the string value to the number type and then compare the values.
In the above example, b is converted to number type by the operator, and then it is compared to a. Since the numeric value of both a and b are the same, so it outputs true.
Behaviour of == and != operator when both operands are of the same type:
When both operands that are to be compared are of the same type, then the == and != operators behave in the following manners:
-
If both are of number data type, then the == operator will return true if both hold the same value; otherwise, false. The != operator will do vice versa.
Example:
In the above example, the first output is true as the value of a and b are the same, and the second value is false as a and c have different sign. Similarly, the third output is true as b, and c aren't the same, and the fourth is true as a and b are the same.
-
If a number is compared with NaN, it will still output false for the == operator.
-
If both operands are of the string type, then the == operator will return true only if each element of the first operand matches with each element of the second operand. The != operator will do vice versa.
Example:
In the above example, the first output is true as str1 and str2 are exactly the same, but the second output is false as str1 and str3 aren't exactly the same. The third output is true as str1 and str3 aren't the same.
Thus we can conclude that the comparison is case-sensitive as well.
- If both operands are of the boolean type, then the == operator will return true only if both operands are true, otherwise, it will return false. The != operator will do vice versa.
Example:
Behaviour of == and != operator when both operands are not of the same type:
When the type of operands aren't the same, the == and != operators use abstract equality comparison algorithm to compare two the two operands. This algorithm loosens the checking and tries to modify the operands into the same type before performing any operation.
Following are the highlights of the behaviour of == and != when the type of operands are not the same:
- If we are comparing string and number, then the string is converted into a number before the comparison.
- If we are comparing boolean and number (0 or 1), then it treats 0 as false and 1 as true.
- If we are comparing two objects, then the operator will check if both refer to the same object. If yes, then the == operator will return true, and != will return false, otherwise == will return false, and != will return true.
- In case we are comparing null and undefined, then == operator will return true, and != operator will return false.
Examples
Example 1: comparing string and number
Explanation:
In this example the first operand is of number type and the second operand is of string type. The == operator does the type conversion of string into a number.
The first output is true as 10, and 10 are equal, thus output true for == operator, the second output is false as 10 and 99 aren't equal.
The third output is true as 10 and 99 aren't equal, thus output is true for != operator, the fourth output is false as 10 and 10 are equal.
Example 2: comparing boolean and number
Explanation:
In this example the first operand is of boolean type and the second operand is of number type(0 or 1). The == operator does the type conversion of number into boolean.
The first output is true as true and 1 are equal (as 1 is considered true and 0 is considered false), thus output is true for == operator, the second output is false as true and 0 aren't equal.
Example 3: comparing null and undefined
Explanation:
It outputs true as for == operator, the comparison of null and undefined is true.
Example 4: comparing objects
Explanation:
In the last example, the first output is true because car1 and car1 refer to the same instance whereas the second output is false as car1 and car2 refer to different instances.
Note: Never compare the objects with == operators.
This only works if it's the same object. Different objects with the same value are not equal. Thus == operator returns false when we compare two different objects with the same value.
What is === and !== in JavaScript?
The === operator is called strict equality operator (and !== is called strictly inequality operator). The === operators follow Strictly equality comparison algorithm, i.e., it doesn't do the type conversion of the operands before comparing their values and returns false even if the data type of the operands aren't the same.
Syntax:
For equality:
For inequality:
Where x and y are operands and the middle portion is for the operator. In the case of equality comparison, we use === operator, and in the case of inequality comparison, we use !== operator.
Return type: boolean
It returns either true or false.
The === operator compares operands and returns true if both operands are of the same data type and have some value, otherwise, it returns false.
The !== operator compares operands and returns true if both operands are of different data types or are of the same data type but have different values. If both operands are of the same data type and have the same value, then it returns false.
Note: Unlike == operator, === doesn't does type conversion.
Example:
Here the first output is false as a is a number type whereas b is a string type, the second output is true as both a and c have the same data type and value.
The === and !== operators follow strict equality comparison algorithm to compare two operands.
Following are some of the highlights of the Strict equality comparison algorithm:
- If the operands that we are comparing are of different data type, then it returns false.
- If any one of the two operands that we are comparing is NaN, then it will return false.
- If the operands that we are comparing are null or undefined, then it will return true.
- If the operands that we are comparing are objects, then it will return true if both refer to the same object, else it will return false.
Examples
Example 1: When both operands are of different data type
Explanation:
Here, the first operand is of number data type, and the second operand is of string data type, so the === operator returns false and the !== operator returns true.
Example 2: When either of the operands is NaN
Explanation:
Since the first operand is of NaN form so the === operator returns false and the !== operator returns true.
Example 3: When both operands are null or undefined
Explanation:
Here, the first two comparison output true as both operands are of same type and have the same value, but the last output is false as one operand is null and the other is undefined.
Example 4: When both operands are object
Explanation:
In this example, the first output is true because car1 and car1 refer to the same instance whereas the second output is false as car1 and car2 refer to different instances.
Uses of == and === Operands in JavaScript
Amidst the Difference between == and === in Javascript, both == and === operators are used for comparison of operands.
The == and === operands are used to compare if two values are equal.
The == operand loosely compares two values, thus it can be used in cases where the data type of the operand isn't important. For e.g., imagine a form entry where you've asked students their roll no. It is possible that some will enter it in string form and some in number form. In such cases, we can use the == operator to verify the data without the database.
The === operand strictly compares two values, thus it is used in the places where the data type of the operand is important. Imagine an online coding contest, where an answer is a number in string format. In that case, we'll use the === operator to compare and validate answers.
Key Difference Between == and === in Javascript
The main difference between == and === in JavaScript is how they handle data types during comparisons.
- == (loose equality) performs type coercion, which means it converts the operands to a common data type before making the comparison. This can sometimes lead to an unexpected result when comparing values of different types.
- === (strict equality), on the other hand, does not perform type coercion and requires both the value and data type of the operands to match for the comparison to return true. It offers a more precise and predictable way to compare values in JavaScript.
In essence, == focuses on value equality after type conversion, while === ensures both value and data type equality, making it a safer choice in many situations where data integrity is critical.
Comparison and Difference Between == and === in Javascript
S.no | == | === |
---|---|---|
1 | Compares two operands | Compares two operands |
2 | returns true if operands have the same data type and same value, returns false if the values differ. | returns true only if operands are of same data type and same value, otherwise returns false. |
3 | In case both operands are of different data types, it performs type conversion of one operand in order to make the data types of the operands the same. | In case both operands are of different data type, it doesn't perform type conversion of the operands. |
4 | Also known as loose equality | Also known as strict equality |
5 | Follows abstract equality comparison algorithm. | Follows strict equality comparison algorithm. |
6 | Type coercion is done. Even if the values are of different types, the values are considered equal. | No type coercion is done. If the values are of different types, the values are considered unequal. |
7 | In JavaScript, null and undefined are considered equal when compared using ==. | When using ===, null and undefined are not considered equal because they are of different types. |
Examples
- Following are some examples of the behavior of the == operator:
- Following are some examples of the behavior of the === operator:
Conclusion
- JavaScript has two equality operators, == (loose equality) and === (strict equality), used for comparing values.
- == performs type conversion if needed and returns true if the operands have the same value after conversion, otherwise, it returns false.
- === does not perform type conversion and returns true only if both operands are of the same data type and have the same value, otherwise, it returns false.
- == is also known as the “loose equality” operator, while === is referred to as the “strict equality” operator.
JavaScript is the language that powers the modern web. Join Scaler Topics certification course and become a front-end development expert.