JavaScript Arithmetic Operators
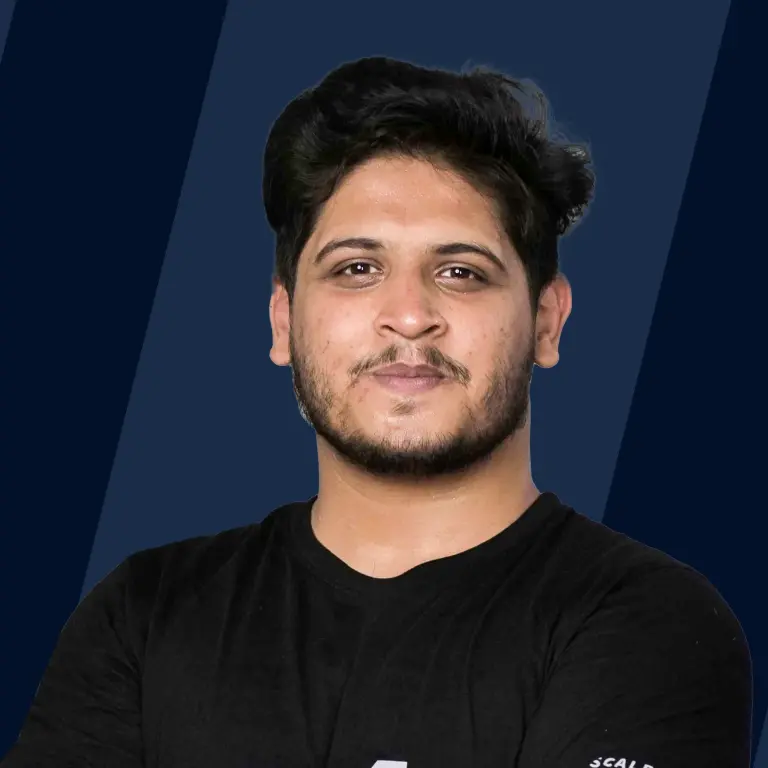
Overview
An arithmetic operator is a mathematical function that takes two operands and performs a calculation on them. JavaScript Arithmetic Operators use a specific symbol in order to be used within the program. JavaScript Arithmetic Operators use two operands and an operator between them in order to calculate the result. JavaScript Arithmetic Operators are simply sign’s that we use in the mathematical equations in order to compute results.
Introduction
Before starting this article, I assume that you know a few important concepts, such as how to store an object in the fields and how to name storage, by calling them variables. You also know how you can use an array, the container object that stores many elements or data types. Now, the question is, what do we do with them? In many cases, you have assigned a value or initialized the value by using an assignment operator(=). After assigning a value to two operands, you can add them by using a + symbol. You can perform many types of operations on one or more than one operand by using such symbols. These symbols are called operators.
Let's take an example, As a student, I often find student's trying to calculate how much they should score on the next exam so that their average is a passing grade, something like the following:
Let’s see each one of them in detail:
Addition Operator
This JavaScript arithmetic operator adds two numeric operands together. The add(+) operator is a bit different:
If one of the operands is a string, the result of the operation is a string, according to these rules:
- If both operands are strings, the second string is concatenated to the first.
- If only one operand is a string, the other operand is converted to a string, and the result is the concatenation of the two strings.
- If single or both operands are decimal values, then it returns the decimal values.
- Infinity added to –Infinity results NaN.
This behavior of add operator is a source of common mistakes, as this code snippet shows:
Syntax: You just need to write two integers. These two integers are stored in variables num1 and num2, respectively. Then, these two numbers are added using the + operator, and the result is printed in the console.
Example:
Explanation:
- Addition Operator(+) is ambiguous. It means "concatenate" or "add". The expression in the first console.log() method converts num1 to a string, because the left operand of add is another string. The result is a string, so num2 is converted to a string, too.
- In the second expression, the parentheses change the operator precedence, and so first, the num1 + num2 expression is evaluated to 50, then this value is converted to a string.
JavaScript Arithmetic operators can handle special values, such as NaN, Infinity, and –Infinity.
- If any of the operands is NaN, the operation result will be NaN, too.
- If an operation provides a result that is higher than the maximum value that can be represented by Number, the result is 'Infinity'.
- Similarly, if the result is lower than the lowest negative value that can be represented by Number, the result is '–Infinity'.
Subtraction Operator:
This JavaScript arithmetic operator subtracts the right operand from the left. Subtraction Operator(-), however has only one meaning subtract. So it subtracts only. There is no opposite of concatenation (I think) and the subtraction operator(-) only performs subtraction regardless of the type of operands.
- 'Infinity' subtracted from 'Infinity' results NaN.
Syntax: You just need to write two integers. These two integers are stored in variables num1 and num2 respectively. Then, these two numbers are subtracted using the - operator, and the result is printed in the console.
Examples:
Explanation:
- The expression in the first console.log() method converts num1 to a string, because the left operand of subtraction is another string. The result is a NaN, If the string value cannot be converted into a Number.
- In the second expression, the parentheses change the operator precedence, and so first, the num1 - num2 expression is evaluated to 6, then this value is converted to a string.
- In the third expression, the decimal value is subtracted.
Multiplication Operator:
This JavaScript arithmetic operator multiplies the two operands together. Multiplication Operator is defined as (*) and multiply two value and return a product.
- Infinity multiplied by 0 results NaN.
- Infinity multiplied by Infinity results Infinity.
- If Infinity is multiplied by any finite number other than 0, the result is either Infinity or –Infinity, depending on the sign of the second operand.
Syntax: You just need to write two integers. These two integers are stored in variables num1 and num2, respectively. Then, these two numbers are multiply using the * operator, and the result is printed in the console.
Examples:
Explanation:
- In the expression, the parentheses change the operator precedence, and so first, the num1 * num2 expression is evaluated to 616, then this value is converted to a string.
Division Operator:
This JavaScript arithmetic operator divides the left operand by the value of the right operand. Division Operator is defined as (/) and divides two value and return a quotient.
- 'Infinity divided by Infinity results NaN.
- 'Zero' divided by zero results NaN.
- If a nonzero finite number is divided by zero, the result is either Infinity or –Infinity, depending on the sign of the first operand.
- If Infinity is divided by any number, the result is either Infinity or –Infinity, depending on the sign of the second operand.
Syntax: You just need to write two integers. These two integers are stored in variables num1 and num2, respectively. Then, these two numbers are divided using the / operator, and the result is printed in the console.
Examples:
Explanation:
- In the expression, the parentheses change the operator precedence, and so first, the num1 / num2 expression is evaluated to 1.2727272727272727, then this value is converted to a string.
Modulus Operator:
This JavaScript arithmetic operator gives the remainder of the left operand when divided by the right operand. It is also known as the Remainder operator. Modulus Operator is defined as (%) and divides two value and return a remainder. Using the Modulus operator, we can find the number is odd or even if we do a number modulo 2 answer is zero then it means the number is even. If we do a number modulo 2 answer is one, then it means the number is odd.
- If the dividend is an infinite number and the divisor is a finite number, modulus results NaN.
- If the dividend is zero and the divisor is nonzero, modulus results zero.
Generally, the remainder operator is useful only when its operands are positive integers.
Another application of the Modulus Operator is to strip the digits from a number.
Syntax: You just need to write two integers. These two integers are stored in variables num1 and num2, respectively. Then, these two numbers use the % operator to calculate the modulus of the number, and the result is printed in the console.
Examples:
Explanation:
- In the expression, the parentheses change the operator precedence, and so first the num1 % num2 expression is evaluated to 6, then this value is converted to a string.
- The last example is interesting since 2 cannot divide 1 at all, the remainder is just the number being divided.
Exponentiation Operator:
This JavaScript arithmetic operator gives the exponent of the left operand raised to the power of the right operand. Exponentiation Operator is defined as (**) and raises the first operand to the power second operand and returns an exponent.
Syntax: You just need to write two integers. These two integers are stored in variable num1 and num2, respectively. Then, these two numbers are Exponentiation using the ** operator, and the result is printed in the console.
Examples:
Explanation:
- In the expression, the parentheses change the operator precedence, and so first the num1 ** num2 expression is evaluated to 4, then this value is converted to a string.
Increment Operator:
This JavaScript arithmetic operator increases the value of the operand by one. Increment Operator is defined by a double plus sign (++). This is a unary operator, x++ produces a value of x before adding 1 to x. This is called the post-increment operator, since the value is produced, and then the variable is incremented. On the other hand,++x produces a value of x after adding 1 to x. This is called the pre-increment operator,
Syntax: You just need to write integers. This integer is stored in variable x respectively. Then, this numbers is incremented using the ++ operator, and the result is printed in the console.
Examples:
Explanation:
- In the first expression, variable x value is 20 and then it's increment by 1 because we perform Increment operator on it, so the result will be 21.
- In the second expression, variable x value is 20 then we perform increment operator on x, and save into y but the value of y is 20 because increment happens post assignment.
- In the third expression, variable x value is 20 then we perform increment operator on x and save into y but the value of y is 21 because increment happens before assignment.
Decrement Operator:
This JavaScript arithmetic operator decreases the value of the operand by one. Decrement Operator is defined by a double minus sign (--). This is a unary operator, x-- produces a value of x before subtracting 1 to x. This is called the post-decrement operator, since the value is produced, and then the variable is decremented. On the other hand, --x decrements the variable first, and then produces the value the pre-decrement operator.
Syntax: You just need to write integers. This integer is stored in variable x respectively. Then, this numbers is decremented using the -- operator, and the result is printed in the console.
Examples:
Explanation:
- In the first expression, variable x value is 20 and then it's decrement by 1 because we perform decrement operator on it, so the result will be 19.
- In the second expression, variable x value is 20 then we perform decrement operator on x and save into y but the value of y is 20 because decrement happens post assignment.
- In the third expression, variable x value is 20 then we perform decrement operator on x and save into y but the value of y is 19 because decrement happens before assignment.
Unary Negation (-) Operator:
The unary negation operator converts a non-number or something to a number because it doesn't perform any operations on numbers. It can convert a string into integer and float values, and it can also convert Boolean values true, false, NULL into a number. It also supports decimal, hexadecimal and negative values.
Syntax: You just need to write integers. This integer is stored in variable x respectively. Then, this number negates the value using the - operator, and the result is printed in the console.
Examples:
Explanation:
- In the first example value of x is 3 and we perform the unary negation operator on to it.
- In the second example value of x as a hexadecimal number 0xFF so it's converted into a decimal number and applies unary negation(-) operator, so it prints -255.
Unary Plus (+) Operator:
Unary plus operator(+) tries to convert If the operand is not a number. if the operand is already a number, it does nothing. It is the fastest and preferred way of converting something into a number because it does not perform any other operations on the number. It can convert a string, boolean values, and NULL to a number. If the operand cannot be converted into a number, the unary plus operator(+) will return NaN.
Syntax: You just need to write integers. This integer is stored in variable x respectively. Then, this converts its operand to Number type using the + operator, and the result is printed in the console.
Examples:
Explanation:
- In the first example value of x is 1, and we perform the unary plus operator on it, but it does nothing.
- In the second example, we apply the unary plus operator on boolean values and NULL, so it converts all into number.
Working of Math Operators With Different Data Types
Now we are going to see how math operators work on different data types like strings, numbers, and booleans.
+ operator
- Number with Number
While both the operands are numbers, + operator simply adds them.
Output:
- Number with String
While one of the operands is string and the other is the number, + operator concatenates them.
Output:
- Number with boolean
During arithmetic operations boolean true is converted to 1 and false to 0. Hence while adding numbers with booleans like true and false, they are treated as 1 and 0.
Output:
- operator
- Number with Number
While both the operands are numbers, - operator subtracts them.
Output:
- Number with String
While one of the operands is a string and the other is the number, no arithmetic operation is done as it shows NaN (Not a Number) in the output.
Output:
- Number with boolean
During arithmetic operations boolean true is converted to 1 and false to 0. Hence while subtracting numbers with booleans like true and false, they are treated as 1 and 0.
Output:
Conclusion:
- JavaScript Arithmetic operators are operators that allow us to apply mathematical operations on numerical data operands.
- JavaScript Arithmetic operators are used to perform numeric operations on operands.
- JavaScript Arithmetic operators perform addition, subtraction, multiplication, division, etc. results.
- These JavaScript arithmetic operators work as they do in most other programming languages when used with floating-point numbers (in particular, note that division by zero produces Infinity).
- All other arithmetic operators will attempt to convert their arguments into numbers before evaluating.