Console in JavaScript
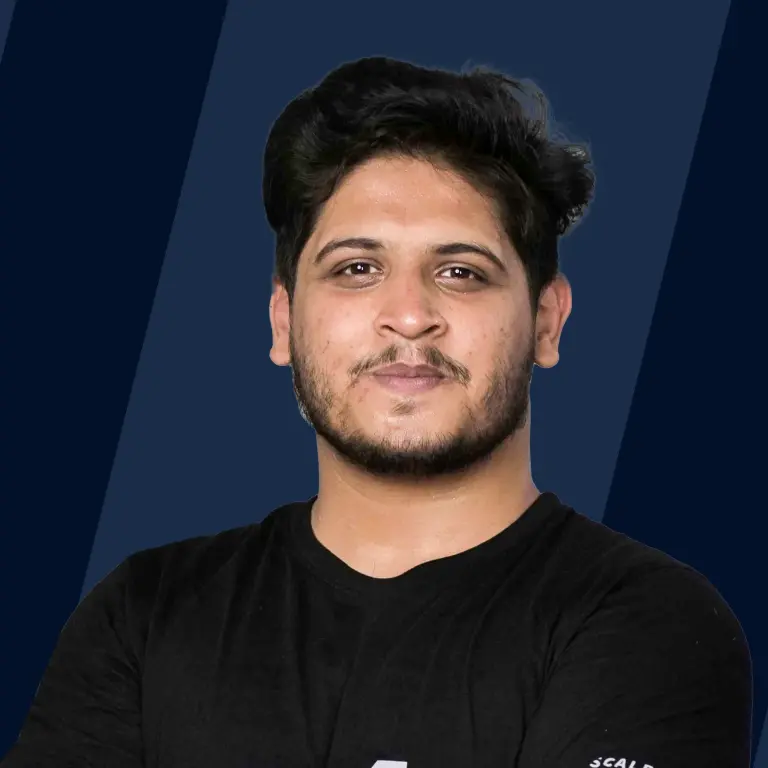
Overview
The JavaScript Console gives us a quick and powerful way of executing JavaScript code inside the browser only. It is widely used for various purposes like logging the output of some code or debugging the code using the console. Apart from the well-known console log method, there are some other methods too that can be used for different purposes like logging errors, warnings, etc.
What is a Web Console?
A Web console is a debugging tool of the browser that logs various details and information of the web page like its JavaScript, network requests, warnings, CSS, errors, etc. In simple words: We can easily interact with the web page by executing the JavaScript code in the Web console. Remember that the changes that we see in the web page by executing the code in the console are just visible to us, and it does not change or modify the original content of that web page. All of the modifications done inside the console for any web page will disappear once we refresh that web page.
Set Up DevTools
Before moving ahead, let us set up the DevTools that we will use throughout this article. DevTools simply means Developer tools that aim to make package development easier and quicker. By using DevTools, We can edit the web pages in real-time and diagnose the problems of the web page more quickly and easily. There are different ways of opening DevTools in the respected browser you use. For this article, we will be referring to Google Chrome. console interface is present in the DevTools, hence can be accessed from there.
1st Method
The first way of opening DevTools is using the menu icon on the top left corner of chrome. Refer to the image given below.
DevTools:
Basic Console log statement inside the Console.
2nd Method
Another way of doing this is using the inspect option. If you right-click on any web page, you will find an inspect option, usually the last one among all the options. Clicking on the inspect option will open the DevTools, and you can easily access the console from there. Refer to the image given below.
The Shortcut Method
Last but not least is the shortcut method that uses the shortcut keys ctrl+shift+I or ctrl+shift+J. Enter this shortcut now to see the JavaScript Console immediately on this page.
On opening the DevTools, you will see various sections like Element selector icon, responsive page icon, Elements, Console, Sources, Network, Performance, Memory, etc. These sections are the part of DevTools that help us in analyzing the page's HTML, such as getting the HTML element, analyzing CSS, such as getting the class name, analyzing JS, and a lot more things like network requests, memory, etc. Let us see how we can view and change the JavaScript of any web page.
View and Change the Page's JavaScript or DOM (Document Object Model)
Let us see an example of sending an alert message to the web page by executing the JavaScript inside the console. Refer to this image:
The alert() function is used to send an alert message to the user. The user can only browse the web page after clicking the OK button in the alert message. This is just a simple example of how we can add or modify the web page's JavaScript using the console. Apart from these use cases, we can also run arbitrary JavaScript that is not related to the page.
Running Random JavaScript Code in the Console.
We can run any random JavaScript code in the console, be it a simple calculation, creating an object, logging an error, or any such thing.
For example:
Code:
Output:
The Console Object
The console is an object(a property of window object) that gives us access to the debugging tool of the browser, which is nothing but a Web Console. The console object has some built-in methods that we have discussed below. These methods can help the developers if utilized effectively. As console is a property of the window object, we can access it by: window.console or only console (mostly used way).
Console Object Methods
Let us see the built-in JavaScript Console object methods sequentially.
assert() Method
Details:
The console.assert() method displays an error message in the console when the assertion proves to be false. It displays nothing when the assertion proves to be true. This method is made available via Web Workers API.
This is one of the rarely used JavaScript Console object methods.
Syntax:
This method accepts a boolean expression followed by the object containing the error message. If the boolean expression returns false, the given error message is thrown. Let us see an example for a better understanding.
Example:
Reference Output Image:
Explanation:
In the above-given code, our assertion was num > 0 which means we asserted for positive values only hence for all the negative values, the assertion proved to be false, which is why this method displayed error messages for those values.
log() Method
Details:
The log() is the most popular JavaScript Console object method which simply logs(or outputs) the information(or message) in the console.
Syntax:
Example:
Explanation:
In the above-given example, first, we logged a message that said: "I am a basic console log" then we logged the whole body of the page using document.body which gave us the body element of the page. If you click on that body element, you will see the whole content of the body. Refer to this image:
error() Method
Details:
The error() method as the name suggests, gives an error message as the output in the console. It can be identified by the red-colored error icon with the message.
Syntax:
Example:
Explanation:
In this example, as the age was less than 18, we printed an error in the console using the error() method.
warn() Method
Details:
The warn() method as the name suggests, gives a warning message as the output in the console. It can be identified by the yellow-colored warning icon with the message.
Syntax:
Example:
Explanation:
In this example, for the secure login, we checked if the password is equal to the user's password, as the password is not equal to the user's password, we gave a warning using the warn() method that says, "Password didn't match!".
clear() Method
Details:
The clear() method, as the name suggests, removes/clears everything present in the console, and you get to see a fully cleared console screen.
Syntax:
Example:
Before:
After:
Explanation:
In this example, we cleared everything that was there on the console using the clear() method.
time() Method and timeEnd() Method
Details:
The time() method accepts an argument as the label of a timer and starts a timer in milliseconds. We need to pass the same label to the timeEnd() method to stop the timer and get the elapsed(time from starting the timer till its termination) time as the output in the console.
Syntax:
Example:
Explanation:
In this example, first, we started a timer labeled (named) as "timer1" using the time() method and then ended the timer using the timeEnd() method. Similarly, we started another timer and ended it. These timers can be very useful when we want to know the complete execution time required for a particular task or piece of code.
timeLog() Method
Details:
The timelog() method logs the running time of the passed timer. We need to pass the label(name) of the timer in this method.
Syntax:
Example:
Output:
Explanation:
In this example, we have a timer called "timer1" then we have the alert() message and after that, we have the timeLog() method followed by another alert() message then, at last, we end the timer. In the output, the first time is the time used by the user to click the ok button of the alert() method and the second time is the total time of the timer from its initiation to its termination.
In case no timer is passed, it returns a warning that says timer "default" doesn't exist. Similar is the case when we pass a timer that does not exist.
table() Method
Details:
The table() method logs the information of the passed array or object in the tabular format in the JavaScript Console. This method can be very useful to log 2d arrays in the JavaScript Console as each piece of information can be seen distinctly and the array gets more readable. This table has the left-most column and the top-most row fixed for indices.
Syntax:
Example:
1D array:
2D array:
Explanation:
In the above-given image, you can easily see the difference between the tabular formatted array and the non-tabular formatted array. The former is more easily readable than the latter.
count() Method
Details:
The count() method, as the name suggests, counts the number of times it was called. We can pass a string value as an argument to refer to some value with the count. If no value is passed, the count() method refers to it as default.
Syntax:
Example:
Output:
Explanation:
In this example, first, we used the count() method in the function called sayHello() with a string value "Scaler" then, whenever we called the sayHello() function, the count of Scaler increased but when we called the count() method without any argument, the count of default increased.
countReset() Method
Details:
The countReset() method as the name suggests, resets the value of counter set with count() method.
Syntax:
Example:
Explanation:
In this example, first, we used the count() method to start the counter for some values then we used the countReset() method to reset the counter to zero.
group() Method and groupEnd() Method
Details:
The group() method as the name suggests, can be used for grouping the related statements in JavaScript Console. If we call this method again, then the statements after this method will get added to another new group. Let us understand it with reference to levels.
The first group is the default at level 0. When we call the group() method, the statements after that method will get added inside the new level, which is level 1. If we call the group() method again, then the statements after that will get added inside the new level, which is level 2. But if we want to end the group or level, we can call the groupEnd() method, which exits that specific group and tells us that the statements after this will get added inside the previous group.
Syntax:
Example:
Explanation:
In this example, we used the group() method to group some statements and the groupEnd() method to end the particular group. When we use a group() method inside "another" group, a new group gets created in that "another" group.
groupCollapsed() Method
Details:
The groupCollapsed() method creates a group that is uncollapsed(or not expanded) by default in the JavaScript Console which means that it expands(or collapses) only when we click it, Otherwise, it remains uncollapsed. Here also we need to call the groupEnd() method to exit this group.
Syntax:
Example:
Explanation:
In this example, we used the groupCollapsed() method to create a group that is not collapsed(or not expanded) by default.
info() Method
Details:
The info() method as the name suggests, outputs the informational message in the console. If you are thinking about the difference between the info() and the log(), then technically, there is none.
Syntax:
Example:
In Chrome Browser:
Explanation:
As you can see in the above-given image, there's no difference in both messages. But if we console the same message in the Firefox browser, we will get to see a small i icon before the info message, which acts as an indicator for the informational message.
In Firefox Browser:
trace() Method
Details:
The trace() traces the stack (where the program statements get executed) and logs the information in the JavaScript Console itself.
Syntax:
Example:
Explanation:
In this example, we used the trace() method to trace the program execution. Here we read the output from bottom to top, which says that first the Global Execution Context was created (anonymous), then parentFunc() was called, and then childFunc() was called. This is how we can trace the execution of a program using the trace() method.
debug() Method
Details:
The debug() method can be used for debugging. It also acts the same as the log() or console.info() method. The main difference lies in displaying the information. While the log() method displays the information directly at the user's console level, the debug() method displays it at the "debug" log level.
Syntax:
Example:
Explanation:
In this example, we used the debug() method to display the debug message which says "I am debug," which gets displayed at the "debug" log level. Hence it is not visible to the user's console level.
dir() Method
Details:
The dir() method accepts only an object as an argument and can be useful when dealing with objects in JavaScript. This method displays all the properties of the specified object.
Syntax:
Example:
Explanation:
In this example, we have used the dir() method to display all the properties of the object a.
dirxml() Method
Details:
The dirxml() method displays the directory structure or the content of any HTML/XML element in the JavaScript Console. If the XML/HTML element is not found, then it displays the data in the object form.
Syntax:
Example:
Explanation:
In this example, we have used the dirxml() method to display the content of the HTML element that we got using document.getElementById.
Styling Console Output
We can give different styling to the console output. Yes, it is possible with the help of CSS. Like we can give different colors to different kinds of messages on a simple console.log() statement. We just need to use backticks that include the %c directive followed by the message that needs to be decorated. Then we have to add a comma and then again backticks which include the styling of that message.
Examples:
Browser Compatibility
All the console methods mentioned and explained in this article are supported by almost all the major browsers out there, such as Chrome, Firefox, Safari, etc.
Conclusion
- A Web console logs various details about the web page like its JavaScript, network requests, warnings, CSS, errors, etc.
- All of the modifications done inside the console for any web page will disappear once we refresh that web page.
- The shortcut keys that can be used to open the console for different browsers are:
- Chrome: ctrl+shift+I or ctrl+shift+J
- Firefox: ctrl+shift+K or ctrl+shift+J
- Safari: Option + ⌘ + C
- console is an object in JavaScript that gives us access to the debugging tool of the browser, which is nothing but a Web Console.
- console object has various methods that make the debugging easier.
- We can give different styling to the console output with the help of CSS.