JavaScript Array Iteration
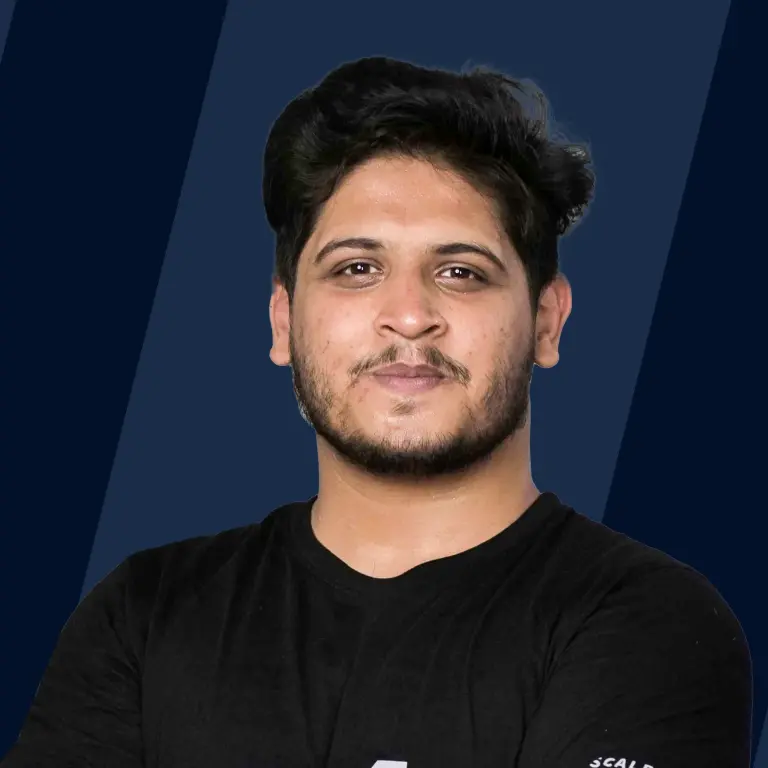
Overview
An array is a container that is used to store different elements. We traverse across the array using the loop to access the array's elements. In JavaScript iterate array, there are many ways through which we can iterate over the array. To repeat a particular section of code a predetermined number of times, loops are useful. In essence, they provide a quick and efficient means of repetition.
Ways of Iterating Over an Array in JavaScript
Using For Loop
In javascript iterate array, a for loop we will check if a particular condition is true, and an action is repeated in for loop. When the condition is ultimately false, the action repeating mechanism is stopped.
Syntax:
- expression1: is executed (one time) before the execution of the code block.
- expression2: defines the condition for executing the code block.
- expression3: is executed (every time) after the code block has been executed.
Example:
Output:
Explanation:
Here in the above code, we have first initialized the array with the integer type elements after that we used the for loop which accepts three expressions- first is the initialization of the variable, and we have specified the stopping condition after that, in the end, we have incremental expression. Inside the for loop in the curly braces, we have specified the statement for printing the elements of the array.
Using While Loop
Syntax:
The while loop loops through a block of code as long as a specified condition is true.
Example:
Output:
Explanation:
Here in the above code, we have first initialized a variable idx to iterate over the array and after that, we have initialized the array with the elements. Next, we used a while loop to iterate over the array after that inside the while loop, we used console.log () to print the current element of the array and at last, we incremented the variable to move to the next position of the array.
Using forEach Method
In contrast to the conventional "for loop," the forEach method uses a function to iterate through arrays.
The following parameters are passed to the forEach method along with a callback function for each entry of an array:
- Current Value(required): The value of the currently selected array element.
- Index(optional): The index of the currently selected element.
- Array(optional): The array object that this element is a part of.
Syntax:
Parameters:
- callback_function: Function to execute on each element.
- val: The value of the current element.
- idx: the array's element's index.
- array: The array of the current element.
- this_Arg: Value to use as this when executing callbackFn.
Example 1: Here we are using an in-line callback function:
Output:
Explanation:
Here in the above code, we have initialized an array with elements inside it. After that, we used the forEach() method using the Inline callback function to print the elements of the array.
Example 2:
forEach() method using callback function:
Output:
Explanation:
Here in the above code, we have defined an array named directions, in which the elements are stored after that we have made a function named demo which has three parameters val, idx, and the array itself. Inside the function, we have printed the elements of the array and we have also used an if condition to check whether we have reached the end of the array at last it prints a statement that ends the forEach() loop.
Using Every Method
In javascript iterate array, every() determines whether each entry in an array passes a test (provided as a function).
Example:
Output:
Explanation:
Here in the above code, we have initialized an array with elements inside it. Next, we have defined an anonymous function that looks over the array and keeps track that whether how many elements are there which is less than 100. At last, using if-else statements we have printed how many elements are under 100.
Using Map
A map creates a new array by applying a function to each member, then returning the results.
Example:
Output:
Explanation:
Here in the above code, we have initialized an array with the elements after that we have variable sqr which has an anonymous function that contains the square of each element in the array next. We have mapped each element using the map method which is squared to an array that is stored in the variable powers after that we have printed the original array and next the squared element of the array.
Using Filter
It's used to filter values out of an array and then provide back the new filtered array.
Example:
Output:
Explanation:
Here in the above code, we have initialized an array with the elements after that we have defined an anonymous function named even_num. After that, we used the filter method to filter the array from the condition given and store it in the variable name even_arr. At last, we printed first the original array and then the filtered array.
Using Reduce
In javascript iterating an array using some functional logic, it reduces the array to a single value.
Syntax:
- function(): It is made to iterate on each element in the array.
- total: the function's initialValue, or the last value it returned.
- current_Value: The value of the current element.
- current_Idx: (Optional). The index of the current element.
- arr: (Optional). The array the current element belongs to.
- initial_Value: (Optional). A value that will be used as the function's starting point.
Example:
Output:
Explanation:
Here in the above code, we have initialized an array with elements after that we have initialized a variable summation which has an anonymous function that sums the element of the array. After that, we used the reduce method which takes two parameters- the first which has the summation and the second parameter is the initial value and the return value of reduce method is stored in the total. At last, we first printed the original array and in the next line, we printed the total summation of the array.
Using Some
It is utilized to determine if certain array values pass a test.
Syntax:
Parameter:
- value: The current element's value. (Required)
- index: the current element's index. (Optional)
- arr: The array that contains the current element. (Optional)
- this: a parameter that is supplied to the function and is utilized as the "this" value. (Optional)
Example:
Output:
Explanation:
Here in the above code, we have initialized an array named arr with elements. After that, we initialized a variable named lessthanFourteenCheck which has defined an anonymous function inside it which count that how many elements in the array are less than 14. After that in the next line, we have variable lessthanFourteen which checks if is there any element in the array that is less than fourteen. If it is it will be True otherwise false. At last, we have used the if-else statement to print whether the array has at least one element less than fourteen.
When to Use forEach()
If we want to do an operation on each element of the array, we utilize the forEach function. In this loop, we are unable to break or skip iterations. Additionally, using a forEach has a more functional and declarative syntax, which is why many developers choose it.
The easiest way to iterate array elements without breaking and simultaneously having some side effects is to utilize the forEach() method.
Examples of side effects include changes to a variable's outer scope, I/O operations (such as HTTP requests), DOM manipulations, and similar actions.
Browser Compatibility
Browser | Support |
---|---|
Safari | Yes |
Chrome | Yes |
Opera | Yes |
Firefox | Yes |
Edge | Yes |
Conclusion
- An array is a container that is used to store different elements.
- In JavaScript, there are many ways through which we can iterate over the array.
- Loops are useful when you want to repeat a certain amount of code repeated number of times.
- There are many ways defined in JavaScript that are- for, while, forEach method, every method, map, filter, Reduce, and Some.
- forEach method is used when you want to perform some operation on each element of the array.
- In forEach we cannot break or skip the iteration.
- forEach is supported on every browser that is- Safari, chrome, opera, edge, and Firefox.