JavaScript Number
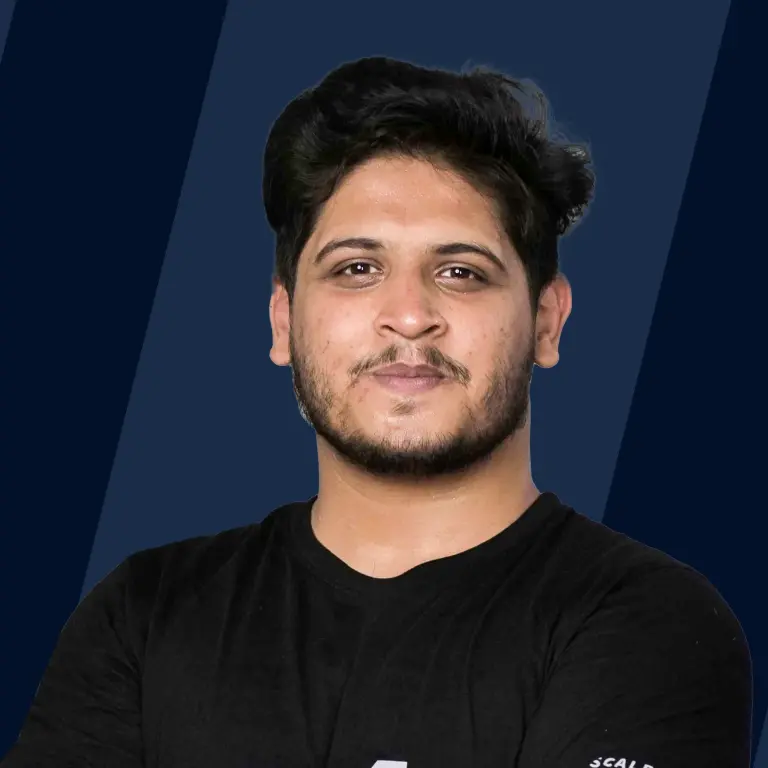
Overview
A number object in javascript holds primitive numeric values. It can be created by number constructor, for example, new Number(value) or Number (value), where "value" is the numeric value of the Number object to be created or value to be converted to a number. It specifies the function that creates an object's prototype.
The Number object represents the numerical date, either integers or floating-point numbers. In general, you do not need to worry about Number objects because the browser automatically converts number literals to instances of the number class.
What is the JavaScript Number Object?
As we have wrappers to cover the chocolates or candies, similarly, wrappers(it can be any class) in JavaScript are used to wrap up similar functions(methods) and properties into one wrapper to make them easy to access.
JavaScript Number Object is a primitive wrapper object for Number primitive data type and is used for the representation and manipulation of numbers(positive as well as negative) that are used in programming like 44, -32.3. As we have the double keyword in Java, in the same way, the JavaScript Number type is a double-precision 64-bit binary format IEEE 754 floating-point value.
It has a number constructor that contains various constants and methods to make it easy to work with numbers.
A Constructor is a function that creates an instance of a class, and this created instance is generally called an 'Object'. Constructor is automatically called in javaScript when an object is declared using the 'new' keyword.
Syntax
Number can be used as a function that helps in converting other data type's values like numeric strings to the Number data type values. In the cases in which other values can not be converted, the Number function returns NaN (Not a Number).
Literal syntax
This is the literal syntax(literal or direct way) that can be followed to create a JavaScript Number.
Function syntax
This is the function syntax(functional way) that can be followed to create JavaScript Number.
NaN (Not a Number) is explained ahead in this article separately.
Assigning a number to a variable
Assigning a number to a variable is very simple. You just need to write the keyword(var/ let/ const), variable name(according to variable name convention), = sign and a value.
For example:
Constructor
The Number() Constructor is used to create a new Number Object.
Syntax
In the below-given syntax, a parameter called as value is accepted which is the numeric value of the object that is to be created.
Examples
- In the code below, we have created a variable num_1 by using the Number Constructor. When we console.log num_1, Number{255} is returned since num_1 is an instance of Number. Number{255} contains two things, one is the Number Prototype and another is the Primitive value, i.e. 255. Refer to the below-given console log image.
Console log image for reference -
- In the code below, we have created a variable num_2 without using the Number Constructor. When we console.log num_2, 255 is returned since num_2 is not an instance of Number. Refer to the below-given console log image.
Console log image for reference -
3. The JavaScript instanceof operator is used for checking the object type at the run time itself.
Integers
Normally integers are the numbers without the fractional part or numbers without the decimal point or period. But integers in javascript are, by default, floating-point values that are stored in 64 bits.
These 64 (0 to 63) bits are generally divided into three parts as-
- Value takes 52 bits i.e., 0 to 51.
- Exponent takes 11 bits, i.e., 52 to 62.
- Sign(+ or -) takes 1 bit i.e. 63
Note that the integers over 15 digits may give inaccurate(sometimes rounded off, up, or down) results.
Examples
- In the case of integer_1, the assigned value is not rounded off; hence we get the same value.
- In the case of integer_2, since the assigned value is of 17 digits, it is rounded off to the nearest lower integer value possible, i.e. 12345678901234568
- In the case of integer_3, the assigned value is not rounded-off as the last digit was not 9; hence we get the same value.
- In the case of integer_4, the assigned value is rounded off to the nearest higher integer value possible, i.e., 10000000000000000 because the last digit of the assigned value was 9.
To solve this unwanted rounding-off problem of large values in JavaScript, BigInt can be used in JavaScript. Let us see this in more detail below.
BigInt
In the above examples, we have seen that the integers show some rounding-off issues when they are of more than 15 digits. Hence, to solve this problem, we have BigInt (Big Integer) in JavaScript, which is a numeric primitive data type used to represent the whole numbers greater than 2^53-1. It simply means that it provides us the facility to store very large numbers that may give inaccurate results if represented using the normal integers.
To make any integer a BigInt, just add (n) with it, and it becomes BigInt or BigInt value. You can see this in the below examples also.
- In the case of integer_1, even though the assigned value has 9 as the last digit, it is not rounded off as it is a BigInt in JavaScript.
- In the case of integer_2, even though the assigned value is 17 digits, it is not rounded off as it is a BigInt in JavaScript.
- In the case of integer_3, even though the assigned value is of 8 digits, it is not rounded off as it is a BigInt in JavaScript. Now we will verify or test the BigInt values.
In JavaScript, we use the typeof operator for checking the data type of the javascript variable.
Floating-point Numbers
The Floating-point Numbers in JavaScript are just the numbers with decimal points or periods. As mentioned above also, every number in JavaScript is a float by default. It can contain digits up to 17 decimal places only. Hence numbers above 17 digits are automatically rounded off.
Examples
Arithmetic operations on Floating-point may give inaccurate results.
Here are a few examples below.
Binary, Octal, Hexadecimal, Exponential
In JavaScript, Binary numbers, Octal numbers, Hexadecimal numbers, and Exponential numbers are also allowed.
Binary numbers: Binary number system has only two digits, i.e., 0 and 1. (base 2)
Octal numbers: Octal number system has eight digits, i.e., 0 to 7. (base 8)
Hexadecimal numbers: Hexadecimal number system has sixteen digits, i.e., 0 to 9 and A to F. (base 16)
Exponential numbers: Exponential numbers have a beN form where b is base or integer, e is a character, and N is power.
Generally, 0b(zero b, b is for binary), 0o(zero 0 o, o is for octal) and 0x(zero x, x is for hexadecimal) are used as the initials in binary, octal and hexadecimal numbers respectively.
Refer to the table given below to see the differences.
Binary Number | Octal Number | Hexadecimal Number | Exponential Number |
---|---|---|---|
Binary number system has only two digits i.e., 0 and 1 | Octal number system has eight digits i.e., 0 to 7 | Hexadecimal number system has sixteen digits i.e., 0 to 9 and A to F. | Exponential numbers have a beN form where b is base or integer, e is character and N is power |
base 2 | base 8 | base 16 | base b |
eg: 0b1100 | eg: 0o040 | eg: 0xbb8 | eg: 134e+5 |
Refer to the examples given below.
- A binary value 0b1100 is assigned to the 'binar' variable above, which equals 12 in decimal.
- An octal value 0o040 is assigned to the 'oct' variable above, which equals 32 in decimal.
- A hexadecimal value 0xbb8 is assigned to the 'hexa_dec' variable above, which equals 3000 in decimal.
- An exponential value 134e+5 is assigned to the 'expo' variable above, which equals 13400000 in decimal.
+ Operator with Numbers
Unlike other programming languages, + operator in JavaScript behaves very differently. You can see its different behavior by observing the examples given below.
- Here, you can see that when we added two numbers, the result was accurate.
- Here, you can see that when we tried to add a numeric string to a number, the result was inaccurate. Here instead of addition, concatenation happened.
In JavaScript, Concatenation is the process of combining two strings into one string by using the + operator.
Therefore, the result we got is a concatenated string instead of an addition number.
- The results are accurate while using numeric strings in other arithmetic operations like subtraction, multiplication, and division. You can observe the examples given below.
Static properties
To simplify some tasks with Numbers, there are static properties of Number in JavaScript like MAX_VALUE and MIN_VALUE, which have predefined values and NaN, which is used to check if the value is not a number. Let us see them in more detail.
- EPSILON : This property gives the smallest or least interval between two representable numbers in JavaScript.
- MAX_SAFE_INTEGER : This property gives the maximum safe integer in JavaScript that is (*2^53-1*)
- MIN_SAFE_INTEGER : This property gives the minimum safe integer in JavaScript that is [*-(2^53-1)*]
- MAX_VALUE : returns the largest possible value
- MIN_VALUE : This property gives the smallest possible positive value that is closest to zero.
- NaN : This property is used for the representation of the 'Not-a-Number' value. In simple words, the value that is not a number is NaN.
- POSITIVE_INFINITY : This property represents positive infinity in JavaScript.
- NEGATIVE_INFINITY : This property represents negative infinity in JavaScript.
- prototype : This property allows the addition of different properties to the Number objects.
Examples
Static methods
In JavaScript, there are some Static methods of Number. Let's have a look at them.
- isFinite() : This method identifies whether the passed value is a finite number or not.
- isNaN() : This method identifies whether the passed value is NaN or not.
- isInteger() : This method identifies whether the passed value is an integer or not.
- isSafeInteger() : This method identifies whether the passed value is a safe integer or not.
- parseInt(string, [radix]) : This method is used to convert the numeric string to an integer
- parseFloat(string) : This method is used to convert the numeric floating string to floating-point number(number with a decimal point)
Examples
- In the 1st example below, we used the parseInt() static method to parse or convert the numeric string '06' into a number 6.
- In the 2nd example below, we used is NaN() static method to see whether the value assigned to variable bis not a number.
- In the 3rd example below, we used the isInteger() static method to verify whether the value assigned to variable c is an integer.
Instance methods
There are some instance methods of Numbers in JavaScript that act on a particular instance. Let us have a look at them.
- toFixed(fractionDigits) : This method returns a string value for a number in a fixed-point notation.
- toExponential(fractionDigits) : This method returns a string value for a number in exponential notation
- valueof() : This method returns the actual value of the number.
- toPrecision(precisionNumber) : This method returns a string value for a number to a specified precision or length.
- toString(radix) : It is used for representing number values into strings. Here radix is optional which means it is not needed to be passed on.
If radix or base is not provided in the toString() method, then 10 is assumed as the radix.
- toLocaleString() : This method returns a string as per the browser's locale settings.
Example
- In the 1st example below, we used the toFixed() instance method to fix the value of c up to 2 digits only. Hence it returns 6.25 only.
- In the 2nd example below, we used the toExponential() instance method to convert the value of d into exponential form. Hence the value 254687 is returned as 2.54687e+5.
- In the 3rd example below, we used valueOf() instance method to see the value of variable b. Hence it returns 77.
- In the 4th example below, we used toPrecision() instance method to get the number to a specified precision which is 2 in the given an example. Hence 25 is the result.
- In the 5th example below, we used toString() instance method converts a number 45 into a string 45.
- In the 6th example below, we used toLocaleString() instance method to get the locale format of the given number. Here we have also passed an object called fObj to specify the format which is Indian Rupee in our case where hi-IN is the language-specific format for Hindi(India). Hence, for 100 we got ₹100.00
Rounding
As we have discussed above, sometimes we get some errors or unpredicted results while rounding off happens in JavaScript. This is because of the unpredicted behavior of JavaScript while rounding off. Let us see some more examples here to analyze this.
- The value assigned to variable a1 is of 15 digits and it returns as it is.
- The value assigned to variable b1 is of 16 digits and it returns as a rounded off value from 9999999999999999 to 10000000000000000.
- The value assigned to variable c1 is of 16 digits and it returns as it is.
- The value assigned to variable a2 is of 16 digits and it returns as it is.
- The value assigned to variable b2 is of 17 digits and it returns as a rounded off value from 1.9999999999999999 to 2.
- The value assigned to variable c2 is of 17 digits and it returns as a rounded off value from 11.999999999999999 to 11.999999999999998.
From the above-mentioned examples, we can conclude that in the case of large numbers, rounding off gives unpredictable results in JavaScript. But why? It's because of exceeding or crossing the maximum safe integer (Number.MAX_SAFE_INTEGER) possible in JavaScript. In fact, the word MAX_SAFE_INTEGER itself says that it is the maximum value of safe integers and integers after this value may give unpredictable results.
JavaScript Number Constants
There are five number constants in JavaScript. They are already discussed above in the Static Properties Section. For simplicity, they are separately listed below.
- MIN_VALUE
- MAX_VALUE
- POSITIVE_INFINITY
- NEGATIVE_INFINITY
- NaN
For more details, you may refer to the Static Properties Section of this article.
Special numeric values
We have two special numeric values in JavaScript. They are Infinity and NaN (Not a Number). Let us see them in more detail below.
Infinity
In JavaScript, if the calculation goes beyond the largest possible value or smallest possible value, we get positive Infinity or negative infinity in return. Refer to the JavaScript Infinity example given below.
NaN
NaN (Not a Number) is a special numeric value that is used to verify a non-numeric value. It returns a boolean value like true when the specified value is not a number and false when the specified value is a number. Refer to the JavaScript NaN example given below.
Precision Problems
As we have discussed above, every number in JavaScript is a float by default which contains digits upto 17 decimal places only, hence numbers above 17 digits are automatically rounded off. This unwanted rounding off leads to precision problems. Let us see some examples below.
Examples
- In the first example below, we get the predicted output i.e. 12.5
- In the second example below, we get the unpredicted output due to precision problems.
To solve this problem, we can use this method -
Conclusion
- In this article, we have discussed JavaScript Number Object.
- We have seen various static properties of JavaScript Number like NaN, Max_Safe_Integer, Min_Safe_Integer, etc.
- We have seen many static methods of JavaScript Number like isFinite(), isNaN(), isInteger(), etc.
- We got to know about BigInt in JavaScript, its need, and its usage.
- We got to know about the inaccuracies during rounding off and precision problems in JavaScript.
- We have seen the application of Number() that how a Number() function converts a numeric string into a number data type.
- JavaScript special values are also discussed with examples.