Javascript String Search()
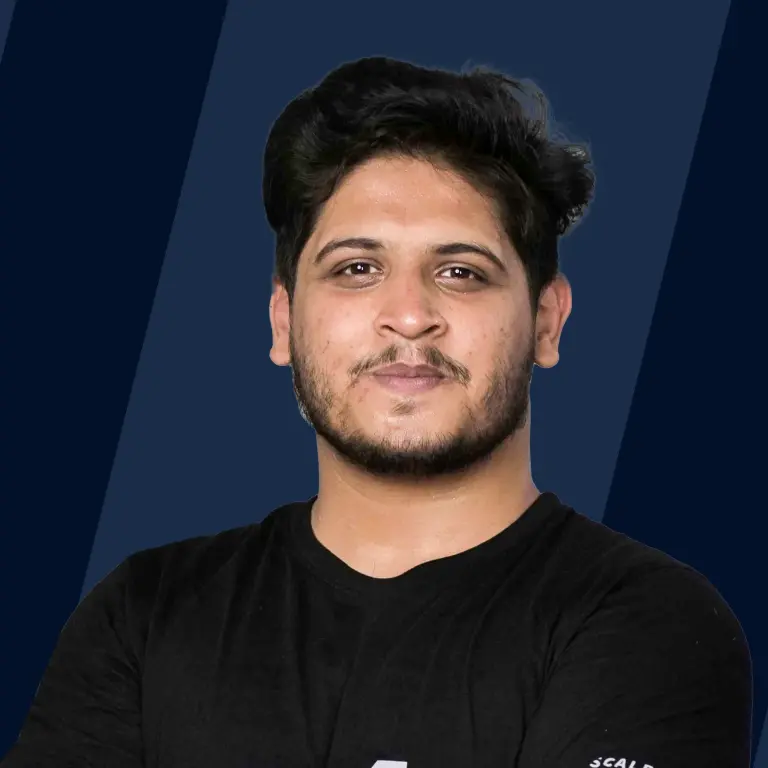
Overview
JavaScript is one of the most popular scripting languages because of the wealth of in-built methods offered by it. One of the most utilitarian methods offered by JavaScript is the string.search() JavaScript method.
Introduction to search() String JavaScript
Let us suppose that you read a very interesting and inspiring storybook. There was a quote somewhere in the story which you appreciated. You wish to relocate that quote and underline it for future reference.
Even though you know that the quote is somewhere in the book, you will probably have difficulty locating it. You may even have to read the entire book again. Human error and negligence are bound to tamper with the searching process.
However, a computer is not prone to committing mistakes. It is liable to conduct impeccable searches and produce accurate results.
Juxtaposing the notebook with a particular string or expression, JavaScript methods like search() can be used to scrounge throughout a given string for a regular expression.
In simpler words, search() is a JavaScript method of the String object used to identify the presence and position of the starting point of a matching pattern or a regular expression in the textual data given.
Parameters and Arguments
Regular Expressions
A regular expression(also called regexp) is a pattern of characters creating a search pattern. The regex example mentioned below can be used to denote the data you are looking for in the text. It is used to perform search-and-replace functions on text. For example:
This regexp example can be used for encryption and pattern-matching.
Parameters Table
The following is a list of regexp parameters that could be passed in the search() method.
Values | Description |
---|---|
^ | It can match the beginning of a line anywhere within the given expression. |
$ | It can match the end of a line anywhere within the given expression. |
* | Can match 0 or more occurrences |
? | Can match 0 or 1 occurrences |
+ | Can match 1 or more occurrences |
. | Can match all characters besides NULL |
[] | Can be used to specify matching lists |
[^ ] | Can be used to specify a non-matching list |
() | Can be used to group expressions |
\b | Can be used for matching word boundaries |
\B | Can be used for Matching non- worded boundaries |
{m} | Can be used for matching m times |
{m,} | Can be used for matching m times only |
{m,n} | Can be used to match at least m times, but for a maximum of n times. |
\n | n is a number between 1 and 9. Used for matching the nth expression that is found within brackets before encountering \n. |
[..] | Can be used for matching one collation element that can be more than one character. |
[::] | Can be used for matches character classes. |
[==] | Can be used for matching equivalence classes. |
\d | Can be used for matching a digit or a numeral. |
\D | Can be used for matching a non-digit character. |
\w | Can be used for matching a word character. |
\W | Can be used for matching a non-worded character. |
\s | Can be used for matching a whitespace character. |
\S | Can be used for matching a non-whitespace character. |
\t | Can be used for matching a horizontal tab character. |
\v | Can be used for matching a vertical tab character. |
\r | Can be used for matching a return character. |
\f | Can be used for matching a form feed character. |
\n | Can be used for matching a line feed character. |
[\b] | Can be used for matching a backspace character. |
\0 | Can be used for matching NULL characters only. |
*? | Can be used for matching the previous pattern's zero or further occurrences. |
+? | Can be used for matching the previous pattern's one or more occurrences. |
?? | Can be used for matching the previous pattern's zero or one occurrence. |
{n}? | Can be used for matching the previous pattern n times. |
{n,}? | Can be used for matching the previous pattern at least n times. |
{n,m}? | Can be used for matching the previous pattern for a minimum of m times, but not more than m times. |
Syntax
Here is the syntax for using the search() method.
In the aforementioned syntax, the regexp or regular expression is passed into the search() method as a parameter. The aim of the code above is to locate the regular expression(regexp) in the given string named str. The regular expression is stored as an object.
If a non-regexp object is passed as a parameter, it is converted into a regexp object first.
This syntax is how a non-regexp could be converted to a new regexp.
Return Values
Since the search() method is used to locate the index of a matched regular expression, the return value is a number corresponding to the index of the regexp (strings in JavaScript are indexed 0).
If a match is found for the expression, the return value is the index.
If a match is not found in the string, the return value is -1.
Description
The search() string JavaScript method is used when a pattern of characters has to be identified in a given string. Consequently, search() is used when the user intends to know whether the pattern is found or not, and if found, then the index of the beginning of the pattern in the string.
If the user only intends to know whether the pattern is present or not, the test() method may be put to use on the regexp. A boolean value is returned.
Here, regexp is the regular expression whose presence we will test in the string.
search() | test() |
---|---|
search() is used when the index location of the regular expression is to be known. | test() is used when only the presence of the regular expression is to be known. |
search() returns a numeric value | test() returns a boolean value. |
For a more descriptive output, the match() method may also be used. However, this extra information renders its execution time a lot more than that of the test() or search() in JavaScript.
Here, string is the input string within which the regexp is to be located.
An alternative to search() string JavaScript could be the indexOf() method in JavaScript.
Here, searchval is the string that needs to be located in the given string, and starting denotes the position to start searching from. If this parameter is not specified, it is taken to be 0. indexOf cannot accept regular expressions.
Examples
Here are some examples to understand the working of the search() string JavaScript method.
Example 1:
Let us look at a typical example of searching for the expression 'is' in the given string and printing its index.
The output for the code above is: 14 Remember that the index of the first character is 0 and not 1.
Example 2:
Let us look at a typical example to search for an expression not present in the given string.
The output for the code above is: -1 This is clearly because the letter 'z' is not present anywhere in the input string.
Example 3
Let us look at a typical example to determine that search() is a case-sensitive method.
The output for the code above is: 46
Note that the index would have simply been 3 if the user was looking for l. However since the user is looking for the string beginning with L, the correct index is 46.
Case-sensitive behaviours could be ignored by using the ignore flags. It is only part of the regex input and could not be used with strings.
For example:
The output for the code above is: 3 'i' stands for the ignore flag, which ignores case sensitivity.
A way to print case-sensitive outputs is:
Note that regular expressions can comprise different types of characters for pattern matching. The output for the code above is: 0
This is because the first capital letter in the A-Z sequence is the string's first character. To ignore case sensitivity in strings, the indexOf method may be used.
If text is a string, it would first be converted to all lower case characters, and then the index of the regular expression would be evaluated. This helps ignore case sensitivity.
Example 4
Let us look at a typical example to determine that in the case of multiple occurrences of a regexp, search() only considers the first occurrence of a regexp.
The output for the code above is 2. This is the first occurrence of the string 'a'.
Specification
ECMAScript Language Specification (ECMAScript): String.prototype.search()
Here, the search() method executes a search for a match between a regular expression and this String object.
Browser Compatibility
The browsers supporting the search() method in JavaScript are:
- Google Chrome-versions 1 and above
- Microsoft Edge-versions 12 and above
- Firefox-versions 1 and above
- Internet Explorer-versions 4 and above
- Opera versions 4 and above
- Safari-versions 1 and above
- WebView Android versions 1 and above
- Chrome Android versions 18 and above
- Safari on iOS-versions 1 and above
Conclusion
- Search() is a JavaScript method to locate the presence and the index of a regular expression in a string.
- It returns the index of the desired regular expression.
- If the regular expression is not present in the string, it returns -1.
- If the user only intends to know whether the pattern is present or not, the test() method may be used instead of the search() method.
- The search() method exhibits case sensitivity.