JavaScript Comparison and Logical Operators
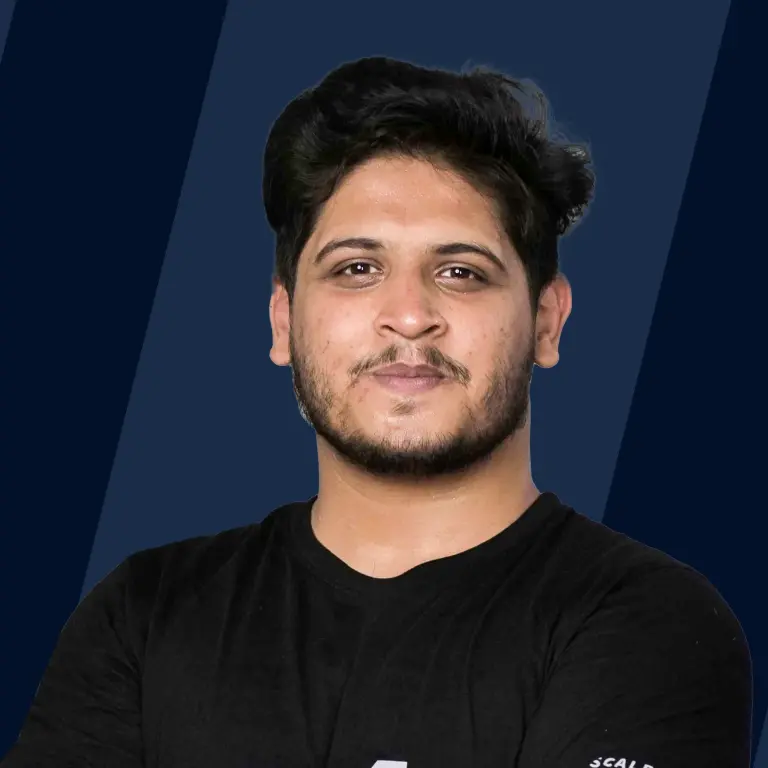
Logical operators in javascript are vital for decision-making and controlling loop terminations. They consist of the NOT (!), AND (&&), and OR (||) operators. The NOT operator flips a boolean value, while the AND operator returns true only if all operands are true. Conversely, the OR operator returns true if at least one operand is true, offering flexibility in condition evaluation
|| (OR)
The “OR” operator is represented with two vertical line symbols:
The || operator in JavaScript returns the first truthy operand encountered, or the value of the last falsy operand if all values are falsy. In short, it returns true if any operand is true and false only if all operands are false.
Operand One | Operand Two | One && Two |
---|---|---|
true | false | true |
false | true | true |
true | true | true |
false | false | false |
Examples:
Using || in a if condition
Output:
OR "||" finds the first truthy value
Given multiple OR’ed values:
The behaviour of OR operator is following:
- Left-to-Right Evaluation: The OR (||) operator assesses operands in a left-to-right sequence.
- Boolean Conversion: Each operand undergoes boolean conversion. If the result is true, the original value of that operand is promptly returned.
- Early Termination on Truthy: When a truthy value is found, the evaluation halts, and the original value of that operand is returned.
- Fallback to Last Operand: If none of the operands yields a truthy value (all are false), the OR operator returns the value of the last operand.
Getting the first truthy value from a list of variables or expressions.
In this scenario, the code aims to select the first truthy value among variables city, country, and continent, representing geographic locations. Utilizing the logical OR (||) operator, it evaluates these variables sequentially, choosing the first truthy value encountered. If none of the variables contain a truthy value, it defaults to "Unknown". In the provided example, as country holds a truthy value ("Canada"), it is chosen and outputted.
Short-circuit evaluation.
In short-circuit evaluation, the OR (||) operator stops processing its arguments as soon as it encounters the first truthy value. This behavior is crucial when operands involve expressions with side effects, like variable assignments or function calls.
For instance, in the code snippet below:
We have an object user representing a user with authentication status (isAuthenticated) and a username. We want to assign a default username ("guest") to the user if they are not authenticated. We use the || operator to check if user.isAuthenticated is falsy. If it is, the default username "guest" is assigned to user.username.
&& (AND)
The AND operator is represented with two ampersands &&:
If both operands are true, it returns true. Otherwise, it returns false. It is often used to combine multiple conditions in conditional statements, ensuring that all conditions must be true for the overall expression to be true.
Operand One | Operand Two | One && Two |
---|---|---|
true | false | false |
false | true | false |
false | false | false |
true | true | true |
Examples:
An example with if-else
An Example with OR (||)
The precedence of AND && operator is higher than OR ||.
The person must be a student (isStudent) with a valid ID (hasValidID) OR have parental consent (hasParentalConsent).
AND “&&” finds the first falsy value
Given multiple AND’ed values:
The behaviour of AND operator is following:
- Left-to-Right Evaluation: The AND operator assesses operands sequentially from left to right.
- Boolean Conversion: Each operand undergoes boolean conversion. If the result is false, the original value of that operand is immediately returned.
- Early Termination on Falsy: If any operand evaluates to false, the evaluation halts, and the falsy value is returned without evaluating the remaining operands.
- Fallback to Last Operand: If all operands evaluate to true, the last operand's original value is returned.
! (NOT)
The boolean NOT operator is represented with an exclamation sign !.
The syntax is :
Whenever there is a need to inverse the boolean value, the logical NOT (!) operator is used. This logical operator in JavaScript is also called logical complement as it converts the truthy value to the falsy value and the falsy value to the truthy value.
Operand | !Operand |
---|---|
true | false |
false | true |
Example:
Using ! in a if condition
Output:
Explanation:
In the given code, with one positive and one negative variable, we utilized the || operator in the if statement. By attaching a logical NOT (!) to the OR expression's result, the else block executed. The OR operator returns true if either or both operators are true. However, the NOT operator inverted the result, making it false. This led to the execution of the else block.
A double NOT !! is sometimes used for converting a value to a boolean type
Double NOT (!!) means Not of a Not. This logical operator in JavaScript is used to get the boolean value of any type of value.
Operand | !!Operand |
---|---|
true | true |
false | false |
Example:
Output:
To understand clearly, we are breaking down the conversion of the last example, For !!true, first we get !true, which is false then we do !false which is true. Hence the final result is true.
A chain of || operators
When there are multiple || operators in a single expression, it's called a chain of || operators. There can be any number of || operators in a single expression as per the situation. The || operator evaluates the expression from left to right and returns the first truthy value that it encounters. In case it does not encounter any truthy value in the whole expression, then it simply returns the last value.
Example:
Explanation: Case 1: In case one, when the given expression evaluates, 1 is returned as its first truthy value. Case 2: In case two, when the given expression evaluates, true is returned as its the first truthy value. Case 3: In case three, 0 is returned as there's no truthy value, and its(0) is the last falsy value.
Logical Operator Precedence
Multiple logical operators in javascript are evaluated in a single expression by following operator precedence. This determines the order in which operators are evaluated. For example, the AND operator (&&) is evaluated before the OR operator (||), ensuring higher priority for certain operators during evaluation.This priority is in the following order ranging from highest to lowest.
- Logical NOT (!)
- Logical AND (&&)
- Logical OR (||)
Code:
Output:
As we know that the logical NOT (!) operator is given precedence over the remaining two logical operators in JavaScript, it is evaluated first, hence after the first step of the evaluation, the above-given expression will look like (false || false && true) then for the next evaluation, the Logical AND (&&) is given precedence hence the expression will look like (false || false), then, at last, the logical || operator gets evaluated and false gets printed in the console.
Conclusion
- The Javascript logical operators are Logical AND (&&), Logical OR (||), and Logical NOT(!).
- We can use logical operators in JavaScript to make decisions when there are multiple conditions.
- When there is a chain of && operators, the first falsy value is returned after the evaluation of the expression.
- When there is a chain of || operators, the first truthy value is returned after the evaluation of the expression.
- The Logical AND (&&) and Logical OR (||) operators support short-circuit evaluation.
- The operator precedence is in the following order: 1. Logical NOT (!) 2. Logical AND (&&) 3. Logical OR (||).