Map and Set in JavaScript
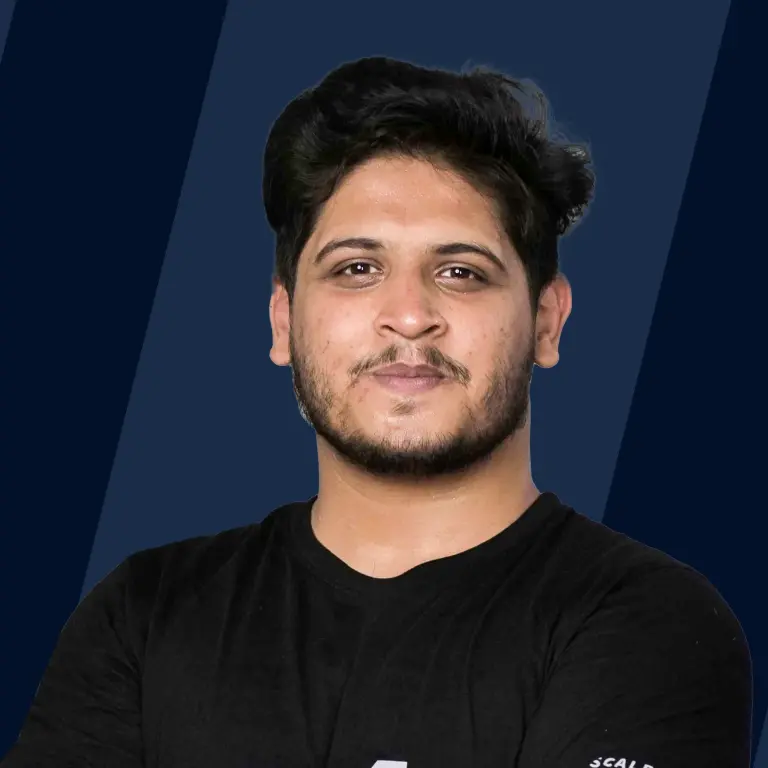
Overview
Map and Set are the newly introduced collection types in JavaScript that are used for storing multiple values in a single variable. Both of them have different use cases which make them unique in their own way. While Map is used for storing data in the form of Key:Value pairs, Set is used for storing unique data items without any keys, just indices.
What is Map in JavaScript?
Map is one of the two newly introduced(in ES6) data structures (of collection type) in JavaScript that is used for storing the data in the form of key: value pairs, inside a single variable. Wait we already have objects to do that right? Yes, We have Objects to store the data in the form of key:value pairs but there are certain limitations in Objects that are not in Maps. Hence, Maps have more functionalities compared to objects in JavaScript. We will discuss more about Map vs objects later in this article, so stay tuned.
Let us see how to create and initialize Maps in JavaScript.
How to Create and Initialize a Map in JavaScript?
The process of creating and initializing Maps in JavaScript is the same as that for the Objects in JavaScript. The syntax is:
Syntax:
Example:
Output:
This way we can create and initialize a new empty map in JavaScript. Let us see how can we add/insert values into the empty map that we just created.
Note: The Time Complexity for insertion, searching and deletion of entries or elements in Map and Set in JavaScript is O(log(n)).
Inserting/Adding Values to a Map
Map in JavaScript has a predefined method called the set() method that can be used to add new values or update the existing data of the Map.
Example:
Output:
Explanation: In the above-given example, we have used the set() method to add keys and values in the map deviceColors. As you can see in the output that all the key: value pairs inside the map are indexed, unlike Objects. This is one of the main differences between Map and Object that the data collection stored inside the Map is indexed(0 based) hence ordered while the data collection inside the Object is unindexed hence unordered(not always). If you notice the output you will find that the key: value pair is shown in the arrow format like key => value.
Getting the values and size of a Map
Getting the values:
If we want to get the value that is associated with a particular key, then we just need to pass that key inside the get() method of Map. The get() method accepts a key and returns the value associated with that key.
Example:
Output:
In case the key that you passed in the get() method is not present in that Map then it returns undefined.
Example:
Output:
Getting the size The Map.prototype.size is a property of the Map that shows the number of elements(here entries of map) present in that map. It is similar to the length property of an Array. Map has an added advantage that the size of a Map is already given in the output Whenever we console.log a map, which means that we do not have to get the size of the map explicitly to know its size, unlike Objects.
But there are times when we need to explicitly mention the size of the map and in such situations, we can use the size property of the Map.
Keys, Values, and Entries for Maps
We can directly get the keys, values, and entries of a map by using the prototype methods of Map. These prototype methods are Map.prototype.keys(),Map.prototype.values(), and Map.prototype.entries(). It should be noted that all of these three prototype methods return a Map Iterator.
To be more specific, the Map.prototype.keys() method returns an iterable over all the keys of the map, the Map.prototype.values() method returns an iterable over all the values of the map, and the Map.prototype.entries() method returns an iterable having a [key:value] pair for each entry of the Map.
The length of each of these pairs is 2, one for the key and the other for the value.
Examples:
Explanation: There are three different examples in the above-given code, Firstly we used the keys() method on the map deviceColors to get the keys of this map, hence we got its keys in the output. Then we used the values() method on the map deviceColors to get the values of this map, hence we got its values in the output.
Then we used the entries() method on the same map to get the entries, hence we got its entries in the output.
Iteration over Map
Iteration over the map keys There are times when we need to iterate over the Map in JavaScript as we do in the case of Arrays or Objects. And here comes the main difference or say an advantage of Map over Object. As we iterate over the Arrays using its in-built forEach() method to get the Array item, index, and the Array itself, the same way we can iterate over the Map using the in-built forEach() method of the Map which iterate over the Map keys, values and the Map itself.
Hence for the understanding purpose, we may say that ==the Object with the power of Array is said to be a Map==. This is not the official definition of a Map; I just mentioned it for a clear understanding as we already saw that Maps hold the keyed collection in a particular order with the index values assigned to each pair in the same way as Arrays hold the collection of items in a particular order with the index values assigned to each item.
Iterating using the forEach() method
Iteration over the Map
Output:
In the above-given example, we used the Map.forEach() method to iterate over the values of Map, keys of Map and the Map itself.
Iteration over the Map keys only
Output:
In the above-given example, we used the Map.forEach() method to iterate over the keys of Map.
Iteration over the Map values only
Output:
In the above-given example, we used the Map.forEach() method to iterate over the values of Map.
Iterating using the for..of loop
We can also iterate over the Map in JavaScript using the for..of loop. Here we will see how to iterate over the map entries, map keys, and then map values using the for..of loop.
Iteration over the Map entries
Output:
In the above-given example, we used the for..of loop to iterate over the entries of Map.
Iterating over the Map keys
Output:
In the above-given example, we used the for..of loop to iterate over the keys of Map with the help of Map.keys() method.
Iterating over the Map values
Output:
In the above-given example, we used the for..of loop to iterate over the values of Map with the help of Map.values() method.
Iterating over the Map keys and values simultaneously by destructuring key and value of Map entry
Output:
In the above-given example, we used the for..of loop to iterate over the keys and values of Map simultaneously with the help of destructuring technique.
Note: You can learn more about destructuring here
Map can also use objects as keys
The keys of Map in JavaScript are amazing as we can specify any kind of value(such as boolean, number, function, or even object) as a key. But as far as Objects are concerned, they lack this functionality. For example:
Output:
As you can see in the above-given example that we can assign any kind of value as a key, like here we have assigned an empty object. Similarly, we can assign an object with data, a function, or anything as a key.
Map vs. Object: When should you use them?
Both Map, as well as Object, are used to store a collection of keyed elements or keyed data inside a single variable. The object is one of the oldest members of JavaScript while Map was introduced in ES6 which means that Map in JavaScript is the newly introduced collection type. Let us see some advantages of Map over Objects.
1. Index and order
Objects are unindexed which means that they are not always in a particular order of insertion while Maps are indexed hence they are always in a particular order of insertion. We know about Arrays in JavaScript which also follows the same indexing and order pattern. Hence, just for the understanding purpose, we may say that Maps are the combination of Objects and Arrays as in Maps we have the power of key: value pair as seen in Objects and the power of indexing and order of insertion as seen in Arrays.
2. Simple and Easy iteration
Iteration in Objects is not as simple as it is in Maps because Maps have some in-built methods like the forEach() method for iteration which makes it a cakewalk to iterate over a Map; its entries, its keys, and its values also.
3. The Amazing Keys
Map in JavaScript can have keys that belong to any data type be it number, boolean, a function, or even an object and this functionality is not there in the Objects.
4. The size of Map
The size property comes in-built in Maps which is an added advantage of using Maps over Objects as Objects have no such in-built way of getting their size.
Now the question arises which collection type to use when?
There are some advantages of Objects also like they are great when dealing with JSON.parse() and JSON.stringify() functions which make it easy to work with JSON. It should be noted that JSON is a widely used data format that deals with many REST APIs. So after going through the above-mentioned points, it can be easier for you to select which one best suits best for your requirement.
Object.entries(): Creating a Map from an Object
The Map can also be created with the pre-specified [Key, Value] paired Array of Arrays. We just need to pass this Array inside the Map constructor while creating a new Map.
Example:
Output:
The Object.entries() is an in-built method that just converts the plain object to an array of arrays. And this array of arrays is passed into the map constructor to create a new map.
Example:
Output:
In the above-given example, we used the data of object called myObj to create a Map having the same data, with the help of Object.entries() method.
Object.fromEntries(): Creating an Object from a Map
What if we want to get an Object from that Map? In such cases, we can use the Object.fromEntries() in-built method. The Object.fromEntries() method accepts the entries of Map as a parameter. We can pass the map entries using Map.entries() method. After getting the map entries, the Object.fromEntries() method creates an object using those map entries. This is how we get an Object from a Map.
Example:
Output:
In the above-given example, we used the entries of map called myMap to create an Object from those map entries by using Object.fromEntries() method.
How to Convert a Map into an Array in JavaScript?
There can be situations when we want to have an array of all the keys of a map or all the values of a map. To get an array of the keys of Map we can use the Map.keys() method and to get an array of values of Map we can use the Map.Values() method with the spread operator which spreads the given data into an array.
Example:
Output:
In the above-given example, we used the spread operator with keys() method and values() method to get the array of keys and array of values of the specified Map.
Note: You can learn more about the spread operator here
WeakMaps in JavaScript
In addition to Map in JavaScript, we have something called WeakMaps which are nothing but Maps with limited functionalities. As we create a Map, the same way we create a WeakMap, we just need to replace the Map() constructor with the WeakMap() constructor.
Syntax:
As WeakMaps are comparatively weak in power, they are called "Weak" Maps. WeakMaps are different from Maps because of the following aspects:
1. Only Objects as keys
The functionality of having any desirable key is not present in WeakMaps, unlike Maps. To be specific, we have only one option for keys in WeakMaps which is Object, doesn't matter whether it is an empty Object or not.
Example:
2. No overview
No overview simply means that we can not iterate over the WeakMap entries, their keys, or their values. It is because WeakMaps does not have the in-built prototype method for iteration unlike the forEach() method in Maps.
Example:
Output:
3. Can't clear WeakMap
It is not possible to clear a WeakMap. Yes, we literally can not clear a WeakMap, unlike Maps where we can clear the Map using its Map.prototype.clear() method.
Example:
In the above-given example, you can see that we easily cleared the Map. Now let us try to do the same with WeakMap.
Example:
In the above-given example, you can see that when we tried to clear the WeakMap we got a TypeError in the console. And the third console.log statement proves that the WeakMap can not be cleared as its data is still the same as before.
Now you might be thinking that if WeakMaps have so many limitations and are so weak then why would anyone use them or why do they even exist at all? Because WeakMaps provide a better way than Map for security and privacy purposes.
Map Properties and Methods
Here is a table that consists of all the important Methods and Properties of the Map with their details and return value.
Method/Property | Details | Return Value |
---|---|---|
set(key, value) | It adds a key => value pair (an entry) to a Map | A Map Object having the newly added entry |
get(key) | It is used for getting the value of a key | It returns the value associated with the specified key |
delete(key) | It removes a key => value pair from a Map using a key | A Boolean value (true if removal is successful, false otherwise) |
keys() | It gives all the keys present in a Map | A MapIterator object having all the keys of the Map |
values() | It gives all the values present in a Map | A MapIterator object having all the values of the Map |
entries() | It gives all the entries present in the map | A MapIterator object having all the entries of the Map |
has(key) | It checks if the element is present in the Map or not using a key | A Boolean value (true if the Map has the specified key, false otherwise) |
clear() | It clears the Map by removing all the items from a Map | - It returns nothing |
size | It gives the number of entries present in a Map | A number that shows the size of the Map |
forEach() | It iterates over the Map | - It returns nothing |
What is Set in JavaScript?
Set is one of the two newly introduced(in ES6) collection types in JavaScript that is used for storing the unique data items inside a single variable. In simple words: a Set is used to store a collection of inter-related unique data items. But we already have Arrays for that right? No, In Arrays there can be duplicate data items while in Sets there can not be any duplicate data items as they are not allowed. Hence, Sets have more functionalities compared to Arrays in JavaScript. We will discuss more about Set vs Arrays later in this article, so stay tuned. Let us see how to create and initialize Sets in JavaScript.
How to Create and Initialize a Set in JavaScript?
The process of creating and initializing a Set in JavaScript is the same as that for the Map in JavaScript. The syntax is:
Syntax:
Example:
Output:
This way we can create and initialize a new empty Set in JavaScript. Let us see how can we add/insert values into the empty Set that we just created.
Inserting/Adding Values to a Set
Set in JavaScript has a predefined method known as the add() method that can be used to add new elements to the set or update the existing elements of the Set.
Example:
Output:
Explanation: In the above-given example, we have used the add() method to add elements in the Set myBooks. If you notice the output you can see that the set elements are enclosed in curly brackets, unlike Arrays where the elements are enclosed in square brackets.
Note: Do not get confused between the Set and set() method of Map. Both of them are entirely different. Set is a collection type while set() is a Map method used to add data to the Map.
Size of a Set
The Set.prototype.size is a property of Set in JavaScript that shows the number of elements(here items of set) present in that set. It is similar to the length property of an Array. Sets have an added advantage that the size of a Set is already given in the output Whenever we console.log a set, which means that we do not have to get the size of the set explicitly to know its size, unlike the Arrays.
But there are times when we need to explicitly mention the size of the set and in such situations, we can use the size property of the Set.
Keys, Values, and Entries for Sets
As we have different methods for keys, values, and entries of Map, similarly we have different methods for Set as well. But as Sets do not have keys and entries, it is of no use to use these methods. Hence, We should always use the values() method of Sets to get its data items.
Note: The keys() method returns the same SetIterator as the values() method while the entries() method returns the value twice.
Examples:
Explanation: We used the values() method on the Set myBooks to get the values of this Set, Hence we got its values in the output.
Iteration over Set
Iteration over the Set keys As we have an in-built forEach() method in Maps for iteration, similarly we have an in-built forEach() method in Sets for iteration. We can also use the for..of loop for iteration over Sets in JavaScript. Let us first see the iteration using the forEach() method and then using the for..of loop.
Iterating using the forEach() method
Iteration over the Set
Output:
As you can notice in the above-given output that the values and keys of the Set are the same and it makes no sense to iterate over them seperately. Therefore, we will be iterating over the values only.
Iteration over the Set values
Output:
In the above-given example, we used the Set.forEach() method to iterate over the items or values of the Set myBooks.
Iterating using for..of loop
Let us now iterate over the Set in JavaScript using the for..of loop. Here also it does not make any sense to iterate over the keys and entries of the Set. Hence we will be iterating over the Set values only.
Iteration over the Set values
Output:
In the above-given example, we used the for..of loop to iterate over the items or values of the Set myBooks.
Set vs. Arrays: When Should you Use Them?
Both Set, as well as Array, are used to store a collection of elements/items inside a single variable. Array is one of the oldest members of JavaScript while Set was introduced in ES6 which means that Set in JavaScript is the newly introduced collection type. Let us see some advantages of Set over Arrays.
While Set can be great when working with values without any duplicate, for finding the intersection and union of two different Sets, Arrays can be great when we want to manipulate data as there are many awesome Array methods such as map() and filter() that give Arrays an edge over the Sets in terms of data manipulation.
So again which data structure to use mainly depends on your requirements.
Unique values from an array using the Set in JavaScript
There can be a situation when you want to get unique values from an array, Set can be a great fit in such situations. Let us see how can we extract unique values from an array. To extract unique values from an Array, we just need to pass that array to a Set constructor and store the result in a variable.
Example:
Output:
How to Convert a Set into an Array in JavaScript?
If we want to convert a Set in JavaScript into an array, we just need to pass that set into the array using the spread operator. If we notice, we are not converting a set into an array but just getting an array from a Set.
Example:
In the above-given example, we used the spread operator with the Set to get the array of values or items of the specified Set.
WeakSets in JavaScript
In addition to Sets, we have something called WeakSets also which are nothing but Sets with limited functionalities. As we create a Set in JavaScript, the same way we create a WeakSet, we just need to replace the Set() constructor with the WeakSet() constructor.
Syntax:
WeakSets are almost the same as WeakMaps that we saw in the previous section of this article. If you have read the WeakMaps description given above, this might sound repetitive to you, anyway. As WeakSets are comparatively weak in power, they are called "Weak" Sets. WeakSets are different from Sets because of the following aspects:
1. Only Objects as data items
Here also we have only one option for data items in WeakSets which is Object, again doesn't matter whether it is an empty Object or not.
Example:
2. No overview
No overview simply means that we can not iterate over the WeakSet data items. It is because WeakSets do not have the in-built prototype method for iteration unlike the forEach() method in Sets.
Example:
Output:
3. Can't clear WeakSet
It is not possible to clear a WeakSet. Yes, we literally can not clear a WeakSet, unlike Sets where we can clear the Set using its Set.prototype.clear() method.
Example:
In the above-given example, you can see that we easily cleared the Set. Now let us try to do the same with WeakSet.
Example:
In the above-given example, you can see that when we tried to clear the WeakSet we got a TypeError in the console. And the third console.log statement proves that the WeakSet can not be cleared as its data is still the same as before. (click on the arrow icon to expand the WeakMap and see its data)
Now you might be thinking that if WeakSets have so many limitations and are so weak then why would anyone use them or why do they even exist at all? Because WeakSets provide a better way than Sets for security and privacy purposes.
Set Properties and Methods
Method/Property | Details | Return Value |
---|---|---|
add(value) | It adds a new element to a Set | Set Object |
delete(value) | It removes the element from a Set using its key | Boolean |
values() | It returns all the keys present in a Set | SetIterator object |
keys() | It returns all the keys present in a Set (same as values()) | SetIterator object |
entries() | It returns all the entries from the Set (same as values()) | SetIterator object |
clear() | It clears the Map by removing all the items from a Set | - |
has(key) | It checks if the element is present in the Set or not using a key | Boolean |
forEach() | It iterates over the Set | - |
size | It returns the number of entries present in a Set | Number |
Conclusion
- Map and Set are the newly introduced collection types in JavaScript.
- Map is used for storing keyed data items inside a single variable.
- Map can also use objects as keys.
- Maps are indexed while Objects are unindexed.
- WeakMaps are the Maps with limited functionalities.
- Set is used for storing unique data items inside a single variable.
- WeakSets are the Sets with limited functionalities.