Null and Undefined in JavaScript
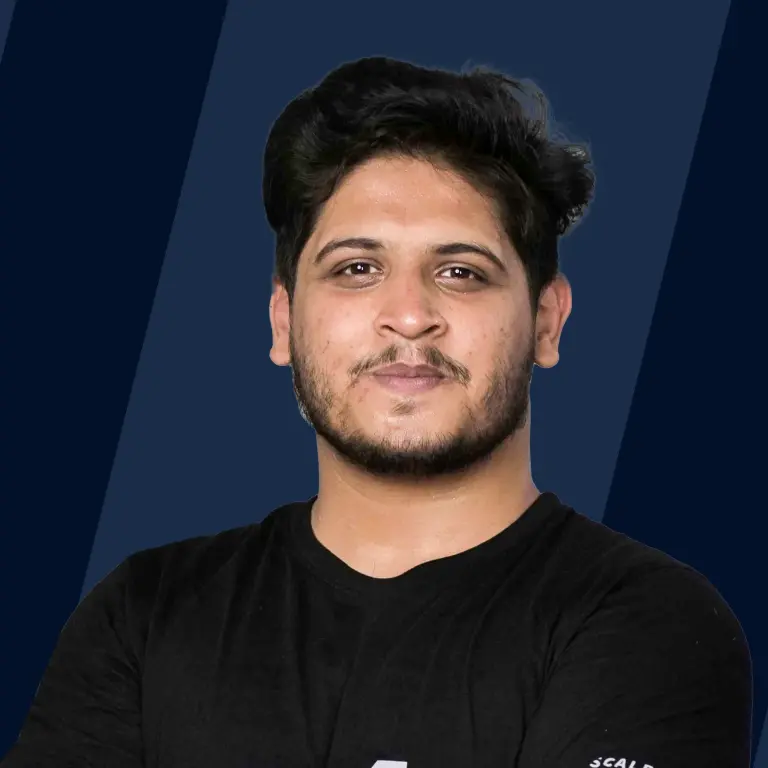
Overview
Null in JavaScript means an empty value and is also a primitive type in JavaScript. The variable which has been assigned as null contains no value. Undefined, on the other hand, means the variable has been declared, but its value has not been assigned.
What is Null?
We have heard the word "null" many times in English. Its literal meaning is having no value. The same meaning is analogous to the meaning of null in programming.
Null is basically an assignment value given to a variable. The variable which has been assigned as null contains no value.
When we assign null as a value to any variable, it means that it is empty or blank. It is to show that the variable has no value. Also, null is an object in JavaScript. When it gets assigned to a variable, it represents no value. The setting of the value must be done manually by the user as JavaScript never sets the value as null. An object can be emptied by setting it to null.
Syntax of Null and Undefined in JavaScript
Here we have assigned the value null to variable x.
What is Undefined?
There is a subtle difference between null and undefined, but as a programmer, it is important that we understand it clearly.
As the name suggests, undefined means "not defined". So we declare a variable but do not assign a value to it, the variable becomes undefined.
Unlike null, the value of an undefined variable is set by JavaScript as undefined. The variable gets created at the run-time. When we do not pass an argument for a function parameter, the default value is taken as undefined. Besides, when a function doesn't return a value, it returns undefined.
Syntax of Null and Undefined in JavaScript
Type of Null and Undefined
In JavaScript, to check the type of variable, we use the "typeof" operator.
Null-
Output-
object
As discussed above, the type of null is an object.
Example-
Output-
object
Here we have assigned the null value to variable x and then checked its type.
Undefined-
Output-
As discussed above, the type of undefined is undefined itself. So basically, it is a data type.
Example-
Output-
Here we have declared variable x and then checked its type. During run time, undefined gets assigned to the variable x.
Why is Null an Object?
Basically, null is a primitive type of a variable in JavaScript. Many people consider it a bug in JavaScript as it considers null as an object. Changing or fixing this bug will break the existing codebase, so it is not yet changed. The type of null is an object. That is why it is treated as an object.
There is another theory behind this. The initial version of JavaScript had values stored as 32 bits. The first 3 bits represented the data type, and the remaining bits represented the value. So for all the objects, the type started from 000. Null typically means empty, so it had all 0s stored in 32 bits. So the JavaScript reads the first 3 digits of null, which are all 0 and hence treats it as an object. The figure represents the same concept used in the initial version of JavaScript.
In APIs, null is often used or returned in a place where an object can be expected as the output but no object is relevant. This shows the absence of an object as null is returned.
Differentiating Using isNaN()
The difference between null and undefined in JavaScript can also be made while performing arithmetic operations like- addition, subtraction, multiplication, division etc.
While performing various arithmetic operations, null is converted to 0, and then the operation is performed, while undefined is converted to NaN. This is so because while performing arithmetic operations, the function toNumber() gets called on it, which converts null to 0 and undefined to NaN.
Output-
We can see that when we perform arithmetic operations on null, the value gets converted to 0 and then the further operations get performed. When we perform arithmetic operations on undefined, it prints NaN as the value is undefined and not a number. Use this Online JavaScript Compiler to compile your code.
Undefined vs Null
The following table summarises the difference between null and undefined in JavaScript.
Area | Undefined | Null |
---|---|---|
Definition | Undefined means the variable has been declared, but its value has not been assigned. | Null means an empty value or a blank value. |
The typeof operator | The typeof() operator returns undefined for an undefined variable. | The typeof() operator returns the type as an object for a variable whose value is assigned as null. |
On performing arithmetic operations | It returns NaN on performing arithmetic operations. | converts to 0 then perform the operation |
Is it an assignment value? | No, as there is no value assigned to the variable, it becomes undefined. | Yes, as we assign null to a variable, it is an assignment value. |
Examples
Example 1-
Output-
As we know, when we initialize a variable as null, and perform arithmetic operations on it, then it gets converted to 0. We have calculated the exponent of the base, and here the exponent is 0 so the value becomes 1.
Example 2-
Output-
As discussed above, we haven't passed any argument for 'h' so it takes the default value as undefined. And we have performed arithmetic operations on variables out of which one is undefined, so the result is NaN.
JavaScript is the language that powers the modern web. Join Scaler Topics certification course and become a front-end development expert.
Conclusion
- Null is basically an assignment value. The variable which has been assigned as null contains no value and is empty.
- Undefined is when we declare a variable but do not assign a value to it(define it), the variable becomes undefined.
- Null is a type of object.
- The data type of undefined is undefined.
- When we perform arithmetic operations on null, the value gets converted to 0, and then the further operations get performed. When we perform arithmetic operations on undefined, it prints NaN as the value is undefined and not a number.