parse() JSON JavaScript
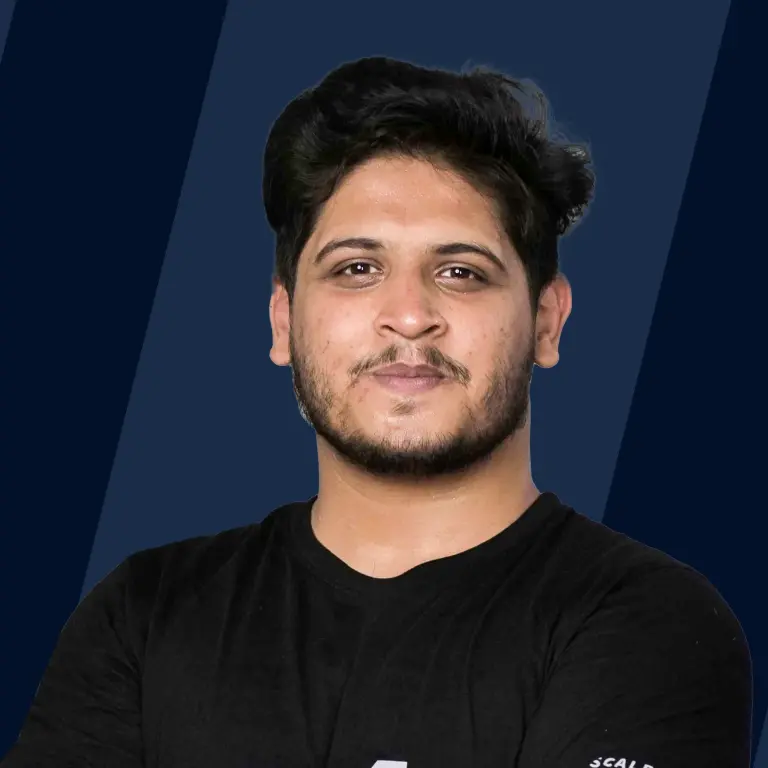
Overview
In JavaScript, the process of converting a JSON string to a JavaScript object is known as JSON parsing. The JSON.parse() function is used to parse a JSON string to an object. It creates a JavaScript object described by the string. In short, JSON.parse() converts a JSON string to a JavaScript object.
What is JSON.parse()
JSON stands for JavaScript Object Notation. As programmers, we often exchange data to and from a web server. So JSON is commonly used to exchange data to and from a web server. When data is received from a web server, it is in the form of a string. JavaScript object contains key-value pairs. However, we cannot access the data in the form of the string, and hence it makes it difficult to manipulate the string to perform operations on it. This is where JSON.parse() comes to play. It converts the string to a JavaScript object, and hence objects can store values and are more beneficial to store data and retrieve the value corresponding to the key.
Syntax
The syntax takes in a JSON string and converts it to a JavaScript object. This is to parse JSON JavaScript.
Parameters
text(compulsory)- It is a JSON string that is to be converted as an object.
reviver(optional)- It is an optional parameter and is a function. It acts like a filter and is used to check each key before returning the value. If the value satisfies the condition, the set of actions will be performed. It is used to modify the values that are parsed. This will be discussed in-depth in the later section.
Return value
It returns a value corresponding to the text. It either returns a JavaScript object, JavaScript array, null value etc.
Exceptions
The string that is to be converted should be a valid JSON string. If it isn't so, the SyntaxError exception will be thrown.
Example-
Output-
As we have not provided the closing quote, the syntax error occurs.
Example
Output-
Our input string got converted to an object. We can now access the value corresponding to the key using the dot "." operator.
The following program is in continuation with the above program.
Output-
Handling JSON.parse() Special Cases with Revivers
The reviver is a function, and it is optional. Basically, reviver acts as a filter and checks each key or value before returning the value. "Key":"Value" together is known as property. If the property returns undefined, the reviver function removes that property from the object. While we parse JSON JavaScript, it is important that we return all untransformed values at the end of the parse function as, if we don't do it, those properties will get deleted.
Example-
Output-
We can see that the reviver function performed transformations on the string. We can also see that our resulting object doesn't have the property which returned undefined, which means it got deleted from the resulting object.
Error Handling
The error handling mechanism is pivotal in the JSON.parse function. An error might occur while we parse JSON JavaScript. The program throws an error if our data is incorrectly formatted or written. Even if the error is in one place, the entire string is not parsed from the point where the error occurs. This can be handled using the try-catch block. The try block will execute the program normally, and if any error occurs, it will be caught by the catch block and displayed on the console.
Example-
Output-
While parsing JSON JavaScript, the try block executes code written within, and if any error occurs, the catch block will catch it and display it on the console.
Array Data
When we perform JSON.parse on a JSON string derived from an array, it converts it to a JavaScript array. It converts JSON array string to JavaScript array. It is used to parse JSON array strings in JavaScript.
Example-
Output-
In the above image, we can see that a JSON array string gets converted to a JavaScript array.
Dates
JSON does not allow Date objects. If you want to write a date, write it as a string, then convert it later after parsing to a date object by using the Date() constructor.
Example-
Output-
We get the date along with the time zone.
Empty Values
JSON allows null and empty strings (" ") as values, but it doesn't allow undefined as valid values. So we should replace the undefined values with similar empty values like null. We have to add null manually to parse JSON javascript.
Example-
Output-
In the above program, we can see that undefined was replaced by null as it is a valid JSON value.
Parsing JSON Data in JavaScript
We use the JSON.parse function to get the parsed data for parsing JSON data. It converts the string to a JavaScript object, and hence objects can store values in them. Each individual value can be accessed using the dot notation(.).
Example
Output-
Parsing Nested JSON Data in JavaScript
JSON objects and arrays can be nested, which means a JSON object can contain another JSON object.
Example-
Output-
Encoding Data as JSON in JavaScript
The JavaScript object needs to be transferred to a server in the form of a string. The JSON.stringify() method is the opposite of JSON.parse() method. It converts a JavaScript object to a JSON string.
Example-
Output-
The object gets converted to a JSON string.
Using JSON.parse() Safely
It is important that we use the JSON.parse() function along with the try and catch block to parse our data as even a small error can crash our application, so it is always used with the try and catch block for smooth running.
Let us look at an example-
Output-
As we can see, a small error crashed our application, and the further part didn't get executed as the code above it caused an error. This can be prevented using the try and catch block as any error gets caught by the catch block and the further code runs if it is error-free.
Output-
As we can see, after implementing the try and catch block, the exception gets handled, and the rest of the code runs properly.
Browser Compatibility
All popular browsers can parse JSON javascript and are compatible with the JSON.parse() function.
Conclusion
- In JavaScript, the process of converting a JSON string to a JavaScript object is known as JSON parsing.
- The JSON.parse() function is used to parse a JSON string to a JavaScript object. It converts a JSON string to an object. It is used to parse JSON JavaScript.
- It takes in two parameters- text(compulsory) and reviver(optional).
- It is advised that one should use the try and catch block for effective error handling when we parse JSON javascript.
- The JSON.stringify() method is the opposite of the JSON.parse() method, and it is used to convert a JavaScript object to a string.