Switch Case in JavaScript
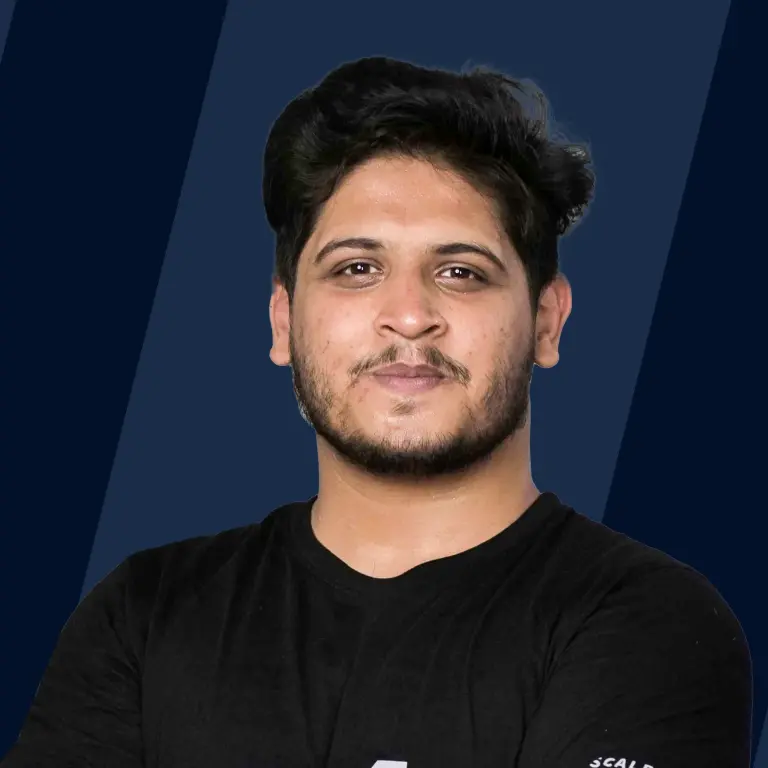
Overview
The switch statement evaluates an expression, matching the expression's value to a case clause, and executes statements associated with that case, as well as statements in cases that follow the matching case.
Introduction
Imagine we are making a website to display the day of the week. The app will simply take a number between 1 to 7 and display the day associated with it (i.e., 1 will be Monday, 2 will be Tuesday, and so on). Now to achieve this, we can use the if-else statement.
We'll take the user input and then store it in a variable. Then we'll write seven if statements where we'll compare the variable with the number associated with each day of the week. If the number matches, we'll display the output; otherwise, we'll have to write an else statement asking the user to enter a valid number.
As we can see that the above process is quite complex. In javascript, situations, where an expression produces different outputs with respect to different cases, can be handled by switch case.
Switch case in javascript can be defined as an equivalent of an if-else statement. The switch statement takes an expression and evaluates it with respect to other cases, and then outputs the values associated with the case that matches the expression.
Also Read- Statements in JavaScript
Flowchart of switch case
Following is a flow chart that describes the working of the switch case in javascript.
In the above flowchart, the switch case starts with the expression. Then it evaluates the expression for case 1. If it passes the expression, then the switch case will execute code block 1, and the operation will terminate.
Although if it fails the first case, then the expression will be evaluated with respect to case 2. If it passes the expression, then the switch case will execute code block 3, and the operation will terminate otherwise, the expression will evaluate the next case.
If no case passes the expression, then the switch statement will execute the default code.
Syntax
Syntax explanation
The expression is the condition or the value that needs to be evaluated. It is evaluated with respect to the first case, then the second case, and so on.
The case is declared by using case keyword followed by the caseValue. This caseValue is evaluated with respect to expression.
The break keyword breaks out of the switch block. It is used to stop the execution of the switch case. When the expression evaluates true wrt a case, it keeps executing all the statements until it encounters the break keyword. The break keyword is optional.
The default statement is executed when the expression fails to evaluate true with respect to any case. The default statement is optional.
How switch statement work in JavaScript
The switch case in javascript takes an expression and tests for its equality. This expression could be a single integer, an enumerated value, or a String object.
The Javascript compiler will show warnings/errors if the equation isn't of a single integer, enumerated value, or String object type.
This is followed by a break keyword, which is used to break the switch case. The break keyword is not mandatory (Although if the break keyword is not there, then the switch case will keep executing the code statements associated with all the cases after the case that evaluates the expression true).
In the end, there is a default statement which is equivalent to the else of the if-else statement. This is used to execute a code if no case is found true. The default statement is not mandatory as well.
Description of JavaScript Switch Statement
The switch case first takes up an expression, and then it evaluates the expression with the cases present. The evaluation of the cases happens sequentially, i.e., the switch statement evaluates the expression with the first case, then the second case, and so on.
The switch case evaluates the cases with the expression using the strict equality (===) operator, which means it wouldn't do any type conversions while comparing values.
If the case passes the evaluation, then the switch case would execute the code associated with the case and then terminate the loop (only if the break is present; otherwise, it would keep evaluating other cases).
If the case fails the evaluation, the switch case evaluates the expression with the next case, and so on. If the expression fails for all the cases, then it proceeds to check if there is a default statement.
The default statement is an optional statement that is used by the switch case when the expression fails for all the cases.
If the default statement is present, its code gets executed, and the switch case for the given expression terminates.
When to Use The Switch Statement
Switch case in javascript can be used at places where our output needs to be dependent on the value an expression holds.
For example, imagine we are making a display board for the price of grocery items. The user will provide us with the grocery name as input, and we have to display its price. In this case, the groceryPrice can be used as an expression for our switch case.
The cases or the possible values of groceryPrice could be the grocery items that are available. The output statement would be the price of the grocery item. We can also add a default case, which would return a statement for the items that aren't present.
Examples
In the first function call, the expression is Cookies, now the switch case will evaluate the expression with the first case, which is Cookies. The expression and the case will hold true for === operator. Thus, the switch case will execute the code associated with first case, and the switch case in javascript will terminate.
In the second function call, the expression is Fruits, now the switch case will evaluate the expression with the first case, Cookies. Since it doesn't match with the first case so the switch case will proceed to evaluate the expression with the second case. Since the expression doesn't even match with the second case (which is milk) so it will proceed to the third case (which is Fruits). The expression and the case will hold true for === operator thus, the switch case will execute the code associated with third case and the switch case in javascript will terminate.
In the third function call, the expression is Peanut, now the switch case will evaluate it with cases as done before, but since it doesn't match any of the cases, the switch case in javascript will execute the statement associated with the default.
Here is another example of the discount offers of various goods.
The code snippet assigns a discount value based on the product category. It uses a switch case statement in JavaScript. The variable productCategory is set to 'electronics'. The switch case checks the value of productCategory and assigns the corresponding discount percentage to the variable discount. Since productCategory is 'electronics', the case 'electronics' is matched, and discount is set to 10. The code then prints the discount value using console.log(). In this case, the output will be "Discount: 10%". If the productCategory doesn't match any case, the default case will set discount to 0.
Break Statement
In the switch case, the break keyword is used associated with the cases to terminate the switch case once the expression matches that particular case.
When the switch case evaluates the expression with the cases, it returns either true or false. Once the true state is achieved, the switch case keeps processing the code statements until either it encounters a break or it reaches the end of the switch case.
What if We Don't Use Break Statements
The switch case stops evaluating the expression once it matches a particular case, i.e., once a case evaluates true with respect to the expression, the switch case will output all the statements until it encounters a break.
If we do not use a break statement, the switch case will execute all the statements it encounters after the case for which the expression has been evaluated true.
For Example:
In the first function call, even though the expression matches with the first case but the switch case will continue executing till the last case as the break statement is not present.
Similarly, in the second function call, the expression will first process the code associated with the second case (as it matches the expression), then it keeps processing the code associated with the rest of the cases.
Since in the third function call, there is no case matching the expression, and the switch case will only process code associated with the default statement and then break the switch case.
Although the switch case in javascript will stop executing once it encounter the break statement.
Example:
In the above example, the switch case evaluates the expression with the first case, and it returns true, then it executes the code associated with the first case. Since the break isn't present in the first case, it executes the code associated with the second case. Since there isn't a break in the second case as well, so it is associated with the code associated with the third case.
Since the third case has break associated with it so the switch case in javascript stops executing.
Grouping of Case
We have already discussed how the switch case executes all the statements it encounters after the case that evaluates true for the expression. The execution of the switch case stops only when it encounters a break.
Sometimes this property of the switch case is used to manipulate the output. The break keyword is removed in such a manner that it either processes the same statement for multiple cases (thus making those cases a group) or multiple statements in a sequential manner for a case. This process is also known as fall-through.
There are two ways in which we can group the cases in switch case in javascript:
Single Operations:
This method takes advantage of the fact that the switch case will keep executing the code statements once the expression evaluates true for an expression until it encounters a break.
This operation is usually used when we want to achieve the same output for multiple cases. In this method, we omit the break for the cases that we want to group. Now once the expression matches any case, it will keep executing irrespective of the case until it finds a break statement.
Example:
In the first and second function call, the expressions are Cookies and Fruits. In both cases, once the expression matches the case, it evaluates all the statements until the break statement. Since all three cases have the same statement associated with it so they can be considered as a group of cases.
In the third function call, as usual, one statement is associated with the case, so it gets executed.
Chained Operations
In this method, the cases and the statement are grouped in a sequence such that different inputs will process different outputs.
The cases in this need not be sequential, i.e., we can add a case with a lower numeric value before a case with a higher numeric value according to our preference. Also, we can add a string with numeric cases.
Example:
In the above example, the function takes the points and outputs the gifts someone will be getting in the exchange for those points.
In the first function call, the input matches with the third case, so it outputs the last statement only.
In the second function call, the input matches with the first case, so it outputs all the statements until it encounters a break. In this case, the chain forms that keep concatenating all the values from case with value 30 up to case with value 10.
In the third function, the input matches with the second case, so it outputs all the statements until it encounters a break. In this case, the chain forms that keep concatenating all the values from case with value 20 up to case with value 10.
In the fourth function call, the input doesn't match any case, so the switch case in javascript outputs the default statement.
Switch vs if…else
If else | Switch case |
---|---|
Its input is an expression that can be a specific condition or a range | It's input is an expression that can be an enumerated value of a string object |
It has less readable syntax | It has more readable syntax |
Multiple if blocks can not be processed unless each condition is true | Multiple cases can be processed if we remove the break statement |
It tests equality as well as logical expressions | It tests only equality. |
It is relatively slow for large values | It works fast for large values because the compiler creates a jump table |
Browser Compatibility
Browser | Chrome | Edge | Firefox | Internet Explorer | Opera | Safari |
---|---|---|---|---|---|---|
Version (min) | 1 | 12 | 1 | 4 | 4 | 1 |
The mentioned values are the minimum version of the browser required to support the given statements.
Conclusion
- The switch case in javascript is used when we have to proceed in a different manner for different cases of an expression.
- The switch statement takes an expression and evaluates it with respect to all the cases and executes the code statement that is associated with the matching case.
- If all the cases fail to satisfy the expression, we can add a default statement.
- The break keyword is used to stop the execution of the switch case once it has processed the code statement that is associated with the matching case.
- We can group cases to manipulate the output. It can be done either by Single operations or chained operations.
- In single operations, the cases are grouped together by omitting the break keyword to provide common output.
- In chain operations, the outputs are structured in such a manner that, for one case, it would output multiple statements.
- The switch case can be considered as an alternative to the if-else statement.