JavaScript Ternary Operator
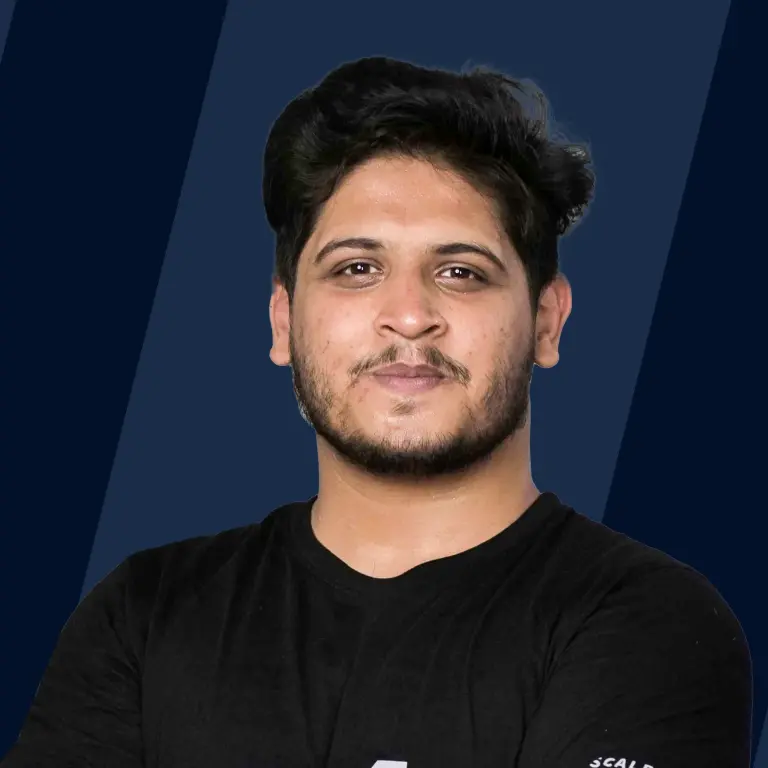
JavaScript's Ternary Operator (?:) condenses conditional logic with three operands, offering an alternative to if-else statements. Enhancing code readability and simplicity, it enables concise representation of conditional blocks within expressions. This article explores its essence, emphasizing its role in streamlining code for clearer, efficient programming.
Syntax of Ternary Operator in Javascript
After a short introduction on what is the ternary operator and a basic example of it, let's dive into its syntax.
Syntax
The conditional operator in javascript is basically a combination of a question mark and a colon. To use this operator, you need to write a conditional expression, followed by a question mark (?).
Parameters Of Ternary Operator In Javascript
You need to provide two statements or expressions separated by a colon. The first expression ( expression_if_true ) evaluates when the condition results in a true value, and the second expression ( expression_if_false ) evaluates when the condition results in a false value.
condition: An expression whose value is used as a condition.
exprIfTrue: An expression which is executed if the condition evaluates to a truthy value (one which equals or can be converted to true).
exprIfFalse: An expression which is executed if the condition is falsy (that is, has a value which can be converted to false).
Description Of Ternary Operator In Javascript
It is used to make our code short and simpler.
For example: Suppose you want to check if a person is eligible to vote or not; you will write something like this:
The output of the above code will be Eligible. But, using the ternary operator, the code becomes very short and readable in simple cases:
In both cases, the output will be the same ,i.e, Eligible.
Characteristics of Ternary Operator
- The expression consists of three operands: the condition, value if true, and value if false.
- The evaluation of the condition should result in either a true/false or a boolean value.
- The true value lies between “?” & “:” and is executed if the condition returns true. Similarly, the false value lies after “:” and is executed if the condition returns false.
Nested Ternary Operators
Similar to nested if-else blocks, we can also use nested ternary operators. i.e., we can use ternary operators within another ternary operator.
Suppose you want to find the largest of three numbers.
Using the if-else block:
Using the Ternary Operator in Javascript:
Basically, one can convert any if-else block to a ternary operator statement. But, converting complex if-else blocks is not recommended, as it will make it harder for someone to read.
Examples of Ternary Operator in Javascript
So, after having some knowledge of how the Ternary Operator works, let's see some examples to get more idea of it.
A simple example
Suppose you want to check if a person passed or failed an examination. This is how the if-else block would look like:
Using the Ternary Operator, we can write the above block in just one line.
In both cases, the output is:
Handling null values
Ternary Operator in Javascript can also be used to check null values.
The following values get converted to false in JavaScript:
- null ;
- NaN ;
- 0 ;
- empty string ( "" or '' or `` );
- undefined .
Using the Ternary Operator in Javascript, we can handle these cases easily.
For example:
The output of the above code is:
Explanation: In the first console statement, we pass "Mark" to the function. So, the value of the variable name is "Mark". This variable has a value other than empty string, null, and NaN, this results in true condition. Thus returning the expression_if_true statement, which is "name".
In the second console statement, we pass no arguments to the function. So the value of the variable "name", remains undefined. This results in a false condition. Thus returning the expression_if_false statement, which is "No argument was passed".
Conditional chains
In this example we will explain about Ternary Chaining. Just like if - else if....else if - else chain, we can also chain Ternary Operator in Javascript.
Chaining of if-else block:
Chaining of Ternary Operator in Javascript:
Here, we write another ternary statement in the place of "expression_if_false". We can add as many ternary operators as needed.
let's implement chaining in an example:
Suppose you want to check the air quality of an area. So, you have the air quality index, and you have to write the code to check how unhealthy the area is.
Using if-else block one would write:
Using Ternary Operator in Javascript:
Value of result for both the codes:
Browser Compatibility
The below table explains that what is the minimum version of a specific browser required to support ternary operator:
Browser | Minimum Version Required |
---|---|
Chrome | 1 |
Edge | 12 |
Firefox | 1 |
Internet Explorer | 3 |
Opera | 3 |
Safari | 1 |
Chrome for Android | 18 |
Firefox for Android | 4 |
Conclusion
- A Ternary Operator in Javascript is a special operator which accepts three operands.
- It is also known as the conditional operator in javascript.
- Ternary Operator in Javascript makes our code clean and simpler.
- It can be chained like an if-else if....else if-else block.
- It can also be nested like a nested if-else block.
- It helps to handle null or undefined values easily.
- Though we can convert any if-else block to a ternary operator statement, we should not do this. Converting a complex if-else block will make it harder to read.