Type Conversion in JavaScript
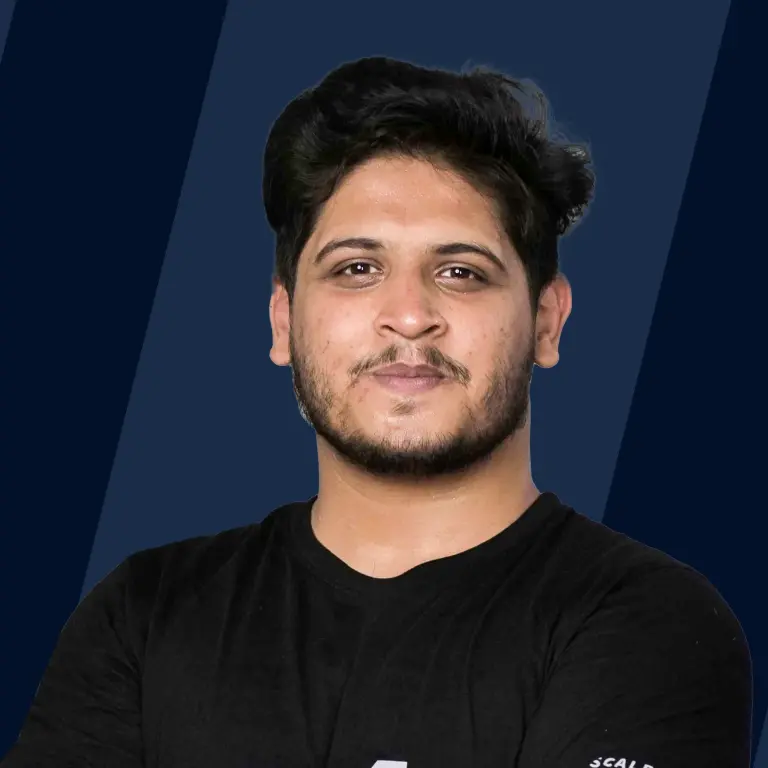
Type conversion in JavaScript is an algorithm that allows us to manipulate the function’s data types from one to another. Also, sometimes, the Javascript compiler automatically does these conversions and ensures that the function gets the valid inputs according to the operator used. In this article, we will dive deep into type conversion in javascript and learn more about these conversions.
Types of type Conversion in JavaScript
- Implicit Conversion
- Explicit Conversion
JavaScript Implicit Conversion
Implicit type conversion in Javascript refers as when converting the values of one data type to another automatically.
Implicit Conversion to String
- Implicit type conversion in javascript to String refers to the automatic conversion of non-string values into string type when they are used in a context where a string is expected.
- This happens behind the scenes without explicit instruction from the developer.
Example:
Implicit Conversion to Number
- Implicit type conversion in javascript refers as when converting to Number in JavaScript occurs automatically when non-numeric values are used in numeric contexts, such as arithmetic operations or comparisons.
- JavaScript tries to convert non-numeric values to numbers to perform the operation.
Example:
Non-numeric String Results to NaN
In JavaScript, converting non-numeric strings to numbers using Number() results in NaN, indicating "Not-a-Number". For instance, Number("Hello"), Number("true"), or Number("") all yield NaN because these strings lack numeric value. For example:
Implicit Boolean Conversion to Number
Conversion of an expression into a boolean is known as boolean conversion.
null Conversion to Number
When null is implicitly used in a numeric context, such as in arithmetic operations or comparisons, it is converted to the number 0. This implicit conversion ensures that operations involving null behave predictably within numeric contexts.
Example:
In this example, null is implicitly converted to the number 0 when used in addition with the number 10, resulting in the value 10.
undefined used with number, boolean, or null
Utilizing undefined in numeric, boolean, or null operations yields NaN, indicating an undefined or unrepresentable value.
Converting Dates to Numbers
Transforming date objects into numeric values, often represented as milliseconds since January 1, 1970, involves using the getTime() method.
Converting Numbers to Dates
Creating date objects from numeric values entails instantiating a new date using the desired timestamp.
JavaScript Explicit Conversion
The conversion of one data type to another according to our need and using built-in methods is called as explicit type casting in javascript.
We will discuss some built-in methods of javascript type conversion explicitly.
Convert to Number Explicitly
This involves explicitly converting a value to a numeric type using the Number() function.
Convert to String Explicitly
Tis involves convert any other data types to strings, with the help of either String() or toString().
Convert to Boolean Explicitly
This involves explicitly converting a value to a boolean type using the Boolean() function. It ensures that the value is interpreted as either true or false, facilitating boolean operations and logic.
JavaScript Type Conversion Table
In the table below are some of the most common JavaScript values conversion to Number, String, and Boolean :point_down:
Value | String conversion | Number conversion | Boolean conversion |
---|---|---|---|
20 | '20' | 20 | true |
'20' | '20' | 20 | true |
false | 'false' | 0 | false |
true | 'true' | 1 | true |
0 | '0' | 0 | false |
'0' | '0' | 0 | true |
NaN | 'NaN' | NaN | false |
null | 'null' | 0 | false |
undefined | 'undefined' | NaN | false |
[] | '' | 0 | true |
"" | "" | 0 | false |
[23] | '23' | 23 | true |
[10,23] | '10,23' | NaN | true |
" " | ' ' | 0 | true |
['fun'] | 'fun' | NaN | true |
['fun','enjoy'] | 'fun,enjoy' | NaN | true |
function(){} | 'function(){}' | NaN | true |
{} | '[object Object]' | NaN | true |
Infinity | 'Infinity' | Infinity | true |
-Infinity | '-Infinity' | -Infinity | true |
Conclusion
- Type conversion in JavaScript is converting one Data type to another as required for the function to work correctly. The difference between implicit and explicit type conversion is that implicit conversions convert the values automatically, while explicit type conversion is manually done by developers.
- Explicit conversion has a major role in manipulating data types in the way we want for the specific function.