jQuery AJAX Methods
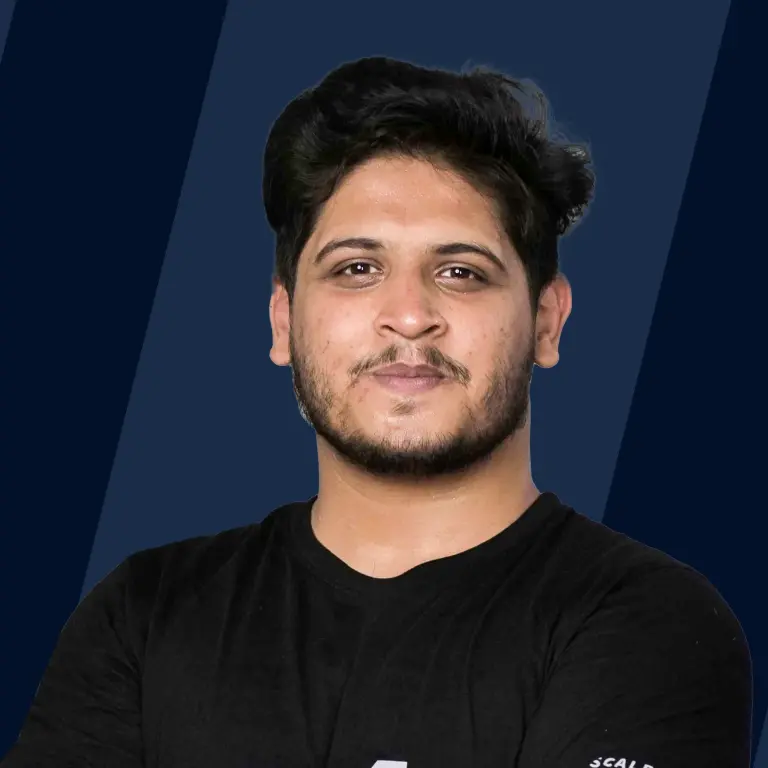
Overview
While jQuery provides several Ajax-related convenience functions, the $.ajax() method is at the heart of them all, and understanding it is critical. We'll go through it first, and then go over the convenience methods shortly.
It's common practice to choose the $.ajax() function over the jQuery convenience methods. As you can see, it provides things that conventional methods do not, and its syntax facilitates reading.
What are Ajax Methods?
Ajax (Asynchronous JavaScript And XML) is a programming language used to construct asynchronous web applications. It is client-side and makes use of a variety of web technologies. Ajax has made it easier to asynchronously send and retrieve data from a server without interfering with the appearance and functionality of the existing page.
Ajax is not a programming language, and it requires a built-in browser that supports the XMLHttpRequest object, which sends data to the server.
jQuery Ajax Methods
The jQuery library includes a comprehensive set of Ajax features. These approaches enable a user to load data from a server without having to refresh the browser.
The following are some examples of Ajax capabilities:
jQuery.get()
This method assists us in retrieving data from the server using the GET HTTP Request. This function returns an XMLHttpRequest object as well.
Syntax:
- url - the string containing the url through which data is transmitted.
- Data -This is an optional argument that holds the key-value pair that was transmitted to the server.
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
- Type is an optional argument that returns a data type following the callback function, such as html, xml, json, text, or jsonp.
Example:
jQuery.getJSON()
This function retrieves json data from the server using the GET HTTP Request.
Syntax:
- url - the string containing the url through which data is supplied.
- Data - This is an optional argument that holds the key/value pair that was transmitted to the server.
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
jQuery.getScript()
This method uses an HTTP GET Request to load and run the javascript file.
Syntax:
- url is the string containing the url through which data is transmitted.
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
jQuery.post()
This method uses an HTTP POST Request to load a web page.
Syntax:
- url - the string containing the url through which data is transmitted.
- data - an optional argument that contains a key and value pair that is transmitted to the server.
- callback - an optional parameter that specifies a function that will be executed when the ajax request is successful.
- type - an optional argument that returns a data type following the callback function, such as html, xml, json, text, or jsonp.
Example:
load()
This method retrieves data or an object from the server and applies the returned html after a successful request to the matched element.
Syntax:
- url - This is the string containing the url through which data is transmitted.
- Data - This is an optional argument that holds the key/value pair that was transmitted to the server.
- Callback - This is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
serialize()
This method aids in serializing a collection of input elements into a set of data array elements. This jQuery ajax function has no parameters.
Example:
serializeArray()
The only difference between this method and the serialize() method is that it returns a json data structure. This procedure does not have any parameters either.
Example:
ajaxComplete()
This method adds a function that will be executed after the ajax request ends. When the callback function is successfully executed, this method stitches a function.
Syntax:
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
ajaxStart()
This method adds a function that will be executed before the ajax request begins.
Syntax:
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
ajaxError()
This method adds a function that is called when an ajax request fails. This is also an example of an ajax event.
Syntax:
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
ajaxSend()
Just after the ajax request is sent, this method calls a function. This is an ajax event as well.
Syntax:
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
ajaxStop()
This method adds a function that is performed immediately after the ajax request is completed.
Syntax:
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
ajaxSuccess()
After the ajax request is successfully finished, this method calls a function.
Syntax:
- Callback is an optional parameter that specifies a function that will be executed when the ajax request is successful.
Example:
Conclusion
- This is a reference for jQuery Ajax Methods. Here we explore the fundamental concept of jQuery Ajax methods with syntax and examples.
- I encourage you to experiment with the code samples and try to modify the code to get a better understanding of the capabilities of jQuery Ajax Methods.