Introduction to jQuery AJAX
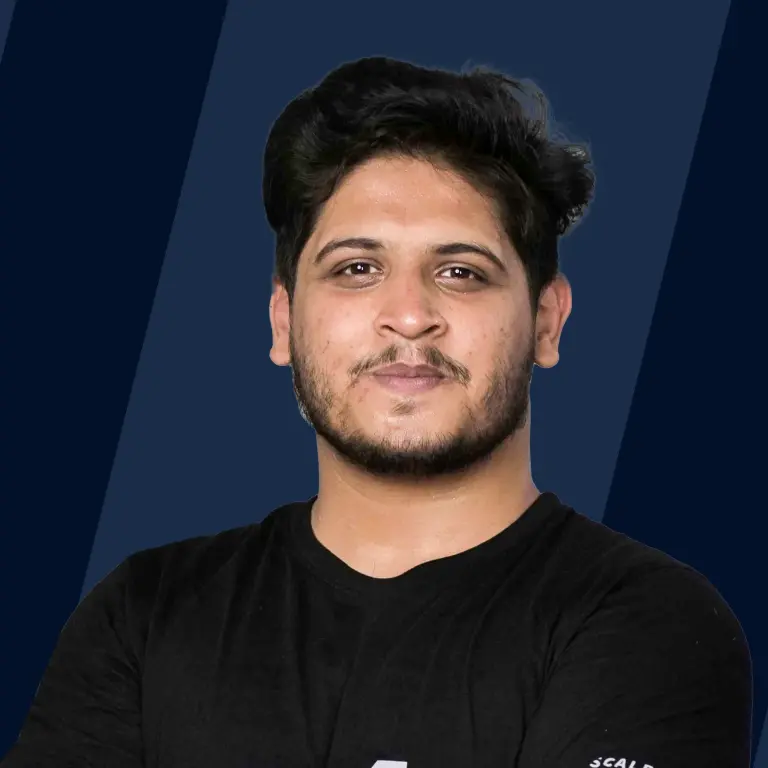
Overview
Regular AJAX code may be challenging to create because different browsers use different syntaxes for AJAX implementation. This means you'll have to write extra code to test for different browsers. The jQuery team has taken care of this for us, allowing us to add AJAX capabilities with just one line of code. So, in this jQuery AJAX tutorial, we'll look at how jQuery ajax works and its syntaxes in depth.
Introduction
AJAX is an expression that means Asynchronous JavaScript and XML, and it allows us to load data from the server without having to refresh the browser page. The ajax() method in jQuery performs an AJAX call. It connects with the server via an asynchronous HTTP request.
jQuery includes a comprehensive collection of AJAX methods for building web apps. It is commonly used for requests. Without further ado, let’s hop into the jQuery ajax tutorial.
Syntax
Here is the basic syntax for loading simple data:
All of the parameters are described here:
- URL - The server-side resource to which the request is directed. A CGI, ASP, JSP, or PHP script that creates data dynamically or from a database could be used.
- Data - This optional parameter specifies an object, the characteristics of which are processed into appropriately encoded arguments that will be sent to the request.
- After the response data has been loaded into the elements of the matched set, a callback function is triggered. The first parameter supplied to this function is the server response text, and the second parameter is the status code.
What is AJAX?
Ajax is an acronym for Asynchronous Javascript And XML. Ajax is just a method of loading data from the server to the web browser without having to reload the entire page.
Ajax is using the JavaScript-based XMLHttpRequest object to transmit and receive data to and from a web server asynchronously, in the background, without interfering with the user's experience.
Ajax has become so prevalent that it is difficult to find an application that does not use it to some extent. Gmail, Google Maps, Google Docs, YouTube, Facebook, Flickr, and other large-scale Ajax-driven internet applications are examples.
All the Ajax Methods of jQuery
Sl. No. | jQuery Ajax Methods | Description |
---|---|---|
1 | ajax() | Sends an HTTP asynchronous request to the server. |
2 | get() | Sends an HTTP GET request to the server to retrieve data. |
3 | post() | Sends a HTTP POST request to the server to submit or load data. |
4 | getJSON() | Sends an HTTP GET request to the server to load JSON-encoded data. |
5 | getScript() | Sends an HTTP GET request to the server to load the JavaScript file, which is ultimately executed. |
6 | load() | Sends an HTTP request to the server to retrieve HTML or text content and add it to a DOM element(s). |
jQuery Ajax Events Table
Sl. No. | jQuery Ajax Events | Description |
---|---|---|
1 | ajaxComplete() | Register a handler function that will be called after Ajax requests are finished. |
2 | ajaxError() | When an Ajax request finishes with an error, register a handler function to be called. |
3 | ajaxSend() | Before sending an Ajax request, register a handler function that will be called. |
4 | ajaxStart() | Set up a handler function that will be called when the first Ajax request is made. |
5 | ajaxStop() | When all of the Ajax requests have been finished, register a handler function to be called. |
6 | ajaxSuccess() | When an Ajax request is successful, register a handler function to be called. |
Getting JSON Data
JSON data can be obtained using the AJAX code. AJAX enables you to get responses remotely. By not reloading the page, it saves bandwidth. In some instances, the server will respond to your request with a JSON string.
The jQuery utility function getJSON() decodes the returned JSON string and delivers it as the first parameter to the callback function for further action. Before sending an Ajax request, register a handler function that will be called.
Syntax
The syntax for the getJSON() method is as follows:
All of the parameters are described here.
- The URL of the server-side resource is accessed using the GET technique.
- The data is the name/value pairs used to generate a query string to be attached to the URL, or a nicely formatted and encoded query string.
- When the request is finished, a callback function is called. The first parameter to this callback is the data value obtained from digesting the response body as a JSON string, and the second is the status.
Passing Data to the Server
The user's input is frequently collected, and then it is sent to the server to be processed further. JQuery AJAX made it simple to send collected data to the server by using the data argument of any Ajax method.
Here’s an example of how to pass data in a jquery ajax call:
Advantages of jQuery Ajax
- Cross-browser compatibility
- Simple methods to employ
- Capability to send GET and POST requests
- Loading of JSON, XML, HTML, or scripts
- When you can change parts of your page without refreshing, which causes the browser to flicker and the statusbar to run, your page will be more pleasant to use.
- You save bandwidth by simply loading the data required to update the page rather than refreshing the complete page.
Disadvantages of jQuery Ajax
- The Back and Forward buttons cannot be used to go between different states of the page since the modifications are made by JavaScript on the client and do not register in the browser's history.
- The user cannot bookmark a certain state for the same reason.
- AJAX-loaded data will not be indexed by any of the major search engines.
- People using browsers that do not support JavaScript or have JavaScript disabled will be unable to access the AJAX capabilities you provide.
Conclusion
- In this article, we discussed $.ajax, jQuery's most powerful Ajax capability.
- It allows you to send Ajax requests while maintaining total control over how the request is sent to the server and how the response is processed. If none of the shortened functions is a good fit, this function offers you the tools you need to accomplish all of your project's requirements.
- Now you can experiment with the code samples and try to modify the code to use some of the other options permitted by the settings parameter to get a better understanding of the function's capabilities.