jQuery AJAX Introduction
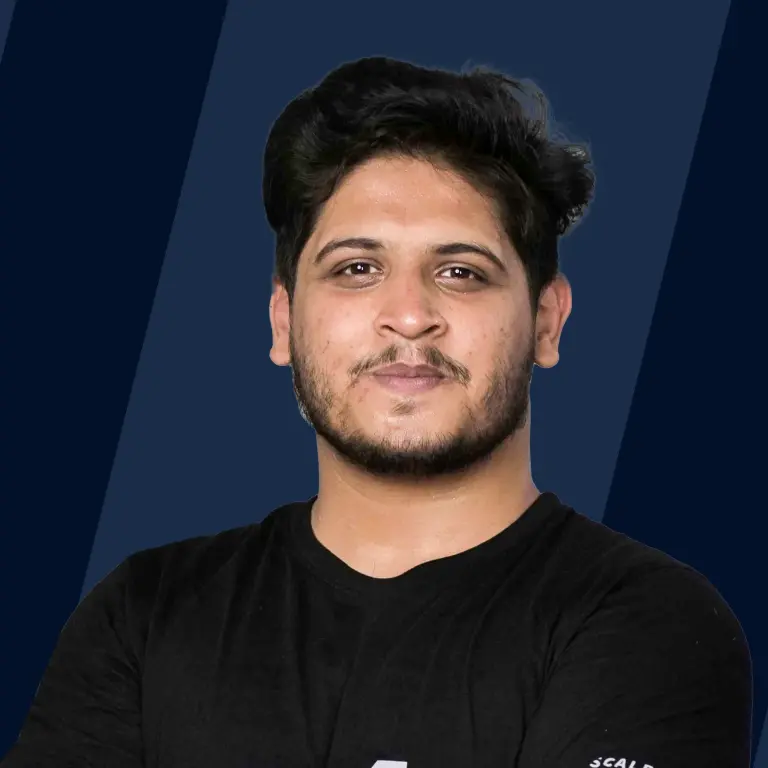
Overview
Regular AJAX code might be difficult to write because different browsers utilize different syntaxes for AJAX implementation. This implies you'll need to develop additional code to test for different browsers.
However, the jQuery team has taken care of this, allowing us to create an AJAX capability with just one line of code. In this article, let's understand how jQuery AJAX works and their syntaxes in detail.
Introduction
AJAX is an expression that stands for Asynchronous JavaScript and XML, and it allows us to load data from the server without having to refresh the browser page. In jQuery, the ajax() method does an AJAX call.
Loading Simple Data
Using JQuery AJAX, you can easily load any static or dynamic data. JQuery provides the load() function for this purpose.
Here is the basic syntax for loading simple data:
All of the parameters are described here:
- URL - This is the web address of the server-side resource to which the request is being sent. A CGI, ASP, JSP, or PHP script that creates data dynamically or from a database could be used.
- Data - An object whose attributes are serialized into appropriately encoded arguments and given to the request. This optional parameter represents the object. If the POST method is chosen, the request is made. If it is not specified, the GET method is used.
- Callback - After the answer data has been loaded into the matched set's elements, a callback function is called. The first parameter supplied to this function is the server response text, and the second parameter is the status code.
Getting JSON Data
AJAX code can be used to get JSON data. AJAX allows you to get responses asynchronously. It saves bandwidth by not reloading the page. In some cases, the server will return a JSON string in response to your request.
The jQuery utility function getJSON() decodes the returned JSON string and passes it to the callback function as the first parameter for further action.
The syntax for the getJSON() method is as follows:
All of the parameters are described here:
- URL - The URL of the server-side resource accessed using the GET technique.
- Data - The name/value pairs used to generate a query string to be attached to the URL or a nicely formatted and encoded query string.
- Callback - When the request is finished, a callback function is called. The first parameter to this callback is the data value obtained from digesting the response body as a JSON string, and the second is the status.
Passing Data to the Server
Many times, you gather user input and send it to the server for further processing. jQuery AJAX made it simple to send collected data to the server by using the data argument of any Ajax method.
Here’s an example of how data can be passed as a variable using the ajax method:
jQuery AJAX Methods
You've seen the fundamentals of AJAX with JQuery. The table below includes all of the key JQuery AJAX methods that you can use depending on your programming requirements.
Sl. No | Method | Description |
---|---|---|
1 | jQuery.ajax(options) | Make an HTTP request to load a remote page. |
2 | jQuery.ajaxSetup(options) | Configure global AJAX request settings. |
3 | jQuery.get(url, [data], [callback], [type]) | Using an HTTP GET request, load a remote page. |
4 | jQuery.getJSON(url, [data], [callback]) | Use an HTTP GET request to load JSON data. |
5 | jQuery.getScript(url, [callback]) | An HTTP GET request is used to load and run a JavaScript file. |
6 | jQuery.post(url, [data], [callback], [type]) | Using an HTTP POST request, load a remote page. |
7 | load(url, [data], [callback]) | Load and inject HTML from a remote file into the DOM. |
8 | serialize( ) | Serializes a collection of input components into a data string. |
9 | serializeArray( ) | Like the .serialize() method, it sets all forms and form elements but returns a JSON data structure for you to work with. |
jQuery AJAX Events
Throughout the AJAX call progress life cycle, you can call multiple jQuery methods.
The following approaches are possible based on various events/stages:
Sl. No | Method | Description |
---|---|---|
1 | ajaxComplete(callback) | Attach a function that will be called once an AJAX request is completed. |
2 | ajaxStart(callback) | Attach a function that will be run anytime an AJAX request is initiated and none is already active. |
3 | ajaxError(callback) | Add a method that will be called anytime an AJAX request fails. |
4 | ajaxSend(callback) | Attach a function that will be run before an AJAX request is submitted. |
5 | ajaxStop(callback) | Attach a function that will be executed once all AJAX queries have been completed. |
6 | ajaxSuccess(callback) | Attach a function that will be called once an AJAX request is completed. |
jQuery AJAX Options
The jQuery.ajax(options) method uses an HTTP request to load a remote page. The XMLHttpRequest created by $.ajax() is returned.
In most circumstances, you won't need to manipulate the object directly, but it is available if you need to manually abort the request.
This method's simple syntax is as follows:
Here are some of the parameters used in this method:
Options - A collection of key/value pairs that define the Ajax request. All of the options are optional.
Sl. No | Option | Description |
---|---|---|
1 | async | A Boolean indicating whether the request should be processed immediately. True is the default value. |
2 | beforeSend | A function that is called before the request is sent. |
3 | complete | Request is completed when the callback function is called. |
4 | contentType | A string containing the MIME content type that should be used for the request. Application/x-www-form-urlencoded is the default value. |
5 | data | A map or string that is submitted together with the request to the server. |
6 | dataFilter | A function for dealing with the raw response data of an XMLHttpRequest. This is a pre-filtering function used to clean up the response. |
7 | dataType | A string describing the type of data to be returned from the server (XML, HTML, JSON, or script). |
8 | error | If the request fails, a callback function is executed. |
9 | global | A Boolean indicating whether this request will trigger global AJAX event handlers. True is the default value. |
10 | ifModified | A Boolean value that indicates whether the server should check to see if the page has been updated before replying to the request. |
Conclusion
- In this article, we covered the most powerful of jQuery's Ajax capabilities, $.ajax(). It enables you to make Ajax requests while having complete control over how the request is submitted to the server and how the answer is handled.
- This function provides the tools you need to meet all your project's requirements if none of the abbreviated functions is a good fit.
- To have a better grasp of the function's possibilities, we encourage you to experiment with the code samples and try to adapt the code to use some of the other choices accepted by the settings argument.