jQuery Core
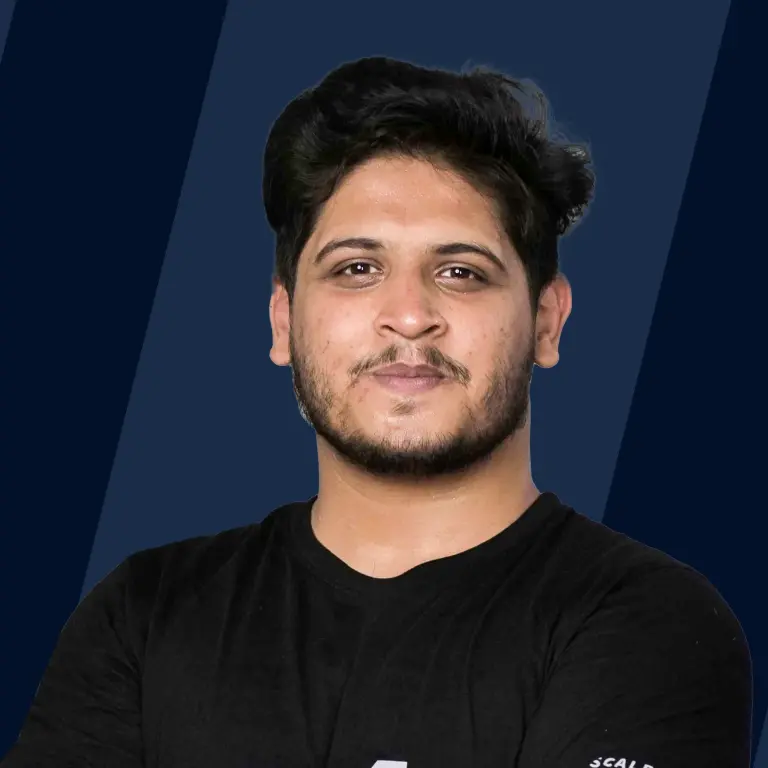
Overview
Previously to jQuery core, we called a function using only jQuery objects, and beginners have trouble deciding where to check the fundamental functions of jQuery.
In this article, we will study how jQuery works as a core element, how to call, select and manipulate it, and also the exceptional cases in detail.
Introduction
What the jQuery object really is and why it functions the way it does are the first and frequently most difficult issues for folks who are unfamiliar with jQuery.
jQuery's architecture attempts to allow developers to pick, interact with, and manipulate all connected components in a single statement using simple, short syntax without having to worry about the problems and caveats that differ between browsers.
In this article, we will look into jQuery fundamental functions and their differences using examples to better understand the concepts. So, without further ado, let's get started.
Before digging into jQuery core functions, explore the basic concepts, call-in functions, and jQuery objects for a better understanding.
$ vs $()
Until now, we've only handled methods on jQuery objects. For example: $( "h1" ).remove();
As mentioned above, the majority of jQuery methods are called on jQuery objects. These methods are said to be part of the $.fn namespace or the jQuery prototype and are best thought of as jQuery object methods.
However, there are specific methods that do not act on a selection. These methods are said to be part of the jQuery namespace and should be considered core jQuery methods.
For new jQuery users, this distinction can be quite confusing:
- Methods called on jQuery selections are in the $.fn namespace and receive and return the selection as this by default.
- Methods in the $ namespace are typically utility-type methods that do not operate with selections; they are not supplied with any arguments by default, and their return value varies.
In a few situations, the names of object methods and core methods are the same, such as $.each() and .each(). In these scenarios, read the documentation carefully to ensure that you are exploring the correct method.
If a method can be called on a jQuery selection, we'll just call it .each(). If it's a utility method (one that isn't called on a selection), we'll refer to it as a method in the jQuery namespace: $.each().
Let’s have this in our minds while we move on to the next topic.
$(document).ready()
A page cannot be securely edited until the document is ready. jQuery detects this ready condition for you. $ contains a code (document).ready() that will only be called when the page's Document Object Model (DOM) is prepared for JavaScript code execution.
The code is written in $(window).on("load", function()... ) will be called whenever the complete page (images or iframes) is ready, not just the DOM.
Example of a $(document).ready() block:
Read/Write Attributes
The properties of an element can contain useful information for your application, so knowing how to read and write them is essential. That is, get and set them.
The .attr() method
The .attr() method performs both getter and setter functions. .attr() accepts either a key and a value or an object containing one or more key/value pairs as a setter.
.attr() as a setter
The following example shows how .attr() works as a setter:
.attr() as a getter
The following example shows how .attr() method works to get (read) the element commands:
Selecting Elements
The most fundamental idea behind jQuery is to pick certain elements and do something with them. Most CSS3 selectors, as well as certain non-standard selectors, are supported by jQuery.
Here are several examples based on type selection, which are as follows:
Selecting Elements by ID
Note: IDs must be unique for each page.
Selecting Elements by Class Name
Selecting Elements by Attribute
Selecting Elements by Compound CSS Selector
Using a Comma-separated List of Selectors to Select Elements:
Working with Selections
Getters & Setters
Some jQuery methods allow you to assign or read a value from a selection.
When called with a value as a parameter, the method is referred to as a setter because it sets (or assigns) that value. When the method is performed without an argument, it obtains (or reads) the element's value.
With the exception of .text(), which obtains the values of all the elements, setters affect all elements in a selection, but getters deliver the requested value only for the first element in the selection.
Example:
Setters return a jQuery object, which allows you to keep calling jQuery functions on your selection. Getters return whatever was asked for. Hence, you can't keep using jQuery functions on the value returned by the getter.
Chaining
If you call a method on a selection and it returns a jQuery object, you can keep using jQuery methods on the object without stopping for a semicolon. This practice is known as chaining.
Here is an example of chaining:
Manipulating Elements
The DOM is manipulated in some way by all of the procedures in this section. Some just modify one of an element's characteristics (also listed in the Attributes category), while others set an element's style properties (also listed in the CSS category).
Others change entire elements (or sets of elements) by inserting, copying, removing, and so on. Because they update the values of properties, and all of these methods are known as setters.
Several of these methods, such as .attr(), .html(), and .val(), also function as "getters", retrieving data from DOM components for subsequent usage.
Manipulating Attributes
The attribute manipulation capabilities of jQuery are vast. The .attr() technique enables more complicated manipulations in addition to basic adjustments. It can either set an explicit value or use the return value of a function to set a value.
When the function syntax is used, the function is given two arguments: the zero-based index of the element whose attribute is being updated and the current value of the attribute being modified.
Example:
The jQuery Object
jQuery returns the elements in a collection when creating new elements (or selecting existing ones). Many new jQuery developers believe that this collection is an array. After all, it has a zero-indexed series of DOM elements, some common array operations, and a length attribute. The jQuery object is much more complex than that.
Working directly with DOM elements turns out to be difficult. The jQuery object defines several methods to make development easier.
Some advantages of jQuery Object include:
Compatibility: It differs between browser vendors and versions in how element methods are implemented. The code below tries to set the inner HTML of an element stored in the target.
Convenience: Many typical DOM modification use cases are difficult to achieve with pure DOM methods. Inserting an element saved in newElement after the target element, for example, necessitates a pretty lengthy DOM technique.
Example:
This works in many cases. However, it fails in the majority of Internet Explorer versions. In that scenario, using pure DOM methods is the recommended way.
DOM Traversing
jQuery allows you to navigate more into what was just selected once you've made an initial choice. Traversing is divided into three categories: parents, children, and siblings. For all of these components, jQuery provides a plethora of simple methods.
It's worth noting that each of these methods can optionally be supplied with text selectors, and some can additionally take another jQuery object to narrow down your selection. Pay attention and consult the API documentation on traversing to learn about the many types of arguments accessible.
Parents
.parent(), .parents(), .parentsUntil(), and .closest() are methods for determining the parents from a selection().
Children
.children() and .find() are two methods for finding child components in a selection(). The difference between these methods is how far into the child tree the selection is made: .children() only works on direct child nodes, whereas .find() can repeatedly traverse into children, children of those children, and it continues.
Siblings
The remaining traversal techniques in jQuery are all concerned with locating sibling choices. There are a few fundamental methods for determining the direction of traversal.
Previous elements can be found with .prev(), next elements with .next(), and both with .siblings(). There are a few additional approaches that build on these core methods: .nextAll(), .nextUntil(), .prevAll(), and .prevUntil() are all functions().
Note - When traversing long distances in documents, be cautious - complicated traversal requires that the document's structure remain constant, which is tough to maintain even if you're the one who created the entire system from server to client. A one-step or two-step traversal is OK, but traversals from one container to another should be avoided.
CSS, Styling, & Dimensions
CSS
In JavaScript, CSS properties that ordinarily include a hyphen must be camelCased. When used as a property name in JavaScript, the CSS property font-size is exfont sizes fontSize.
This does not apply when supplying the name of a CSS property as a string to the .css() method; in that case, either the camelCased or hyphenated form will work.
In production-ready code, it is not suggested to use .css() as a setter. However, when sending in an object to set CSS, CSS properties will be camelCased instead of using a hyphen.
Example:
Using CSS Classes for Styling
The .css() method is useful as a getter. However, it should be avoided as a setter in production-ready code because it is generally desirable to keep presentational information out of JavaScript code. Instead, create CSS rules for classes that define the various visual states and then modify the element's class.
Example:
Classes can also be useful for storing element status information, such as identifying that an element is selected.
Dimensions
jQuery provides a variety of methods for collecting and manipulating element dimension and position information. The code below provides a high-level overview of jQuery's dimensions capabilities.
Example:
Data Methods
There are frequent data about an element that you want to keep with the element. In simple JavaScript, you could do this by adding a property to the DOM element, but you'd have to deal with memory leaks in some browsers. jQuery provides a simple way to save data connected to an element and handles memory difficulties for you.
Example:
An element can store any type of data. .data() will be used to store references to other elements in this article.
Utility Methods
In the $ namespace, jQuery provides various utility functions. These techniques are useful for completing ordinary programming jobs.
Here are some examples of the utility methods:
$.trim()
This function removes previous and following whitespace.
$.each()
It iterates through arrays and objects.
It is possible to iterate over the selection's elements by calling .each() on the selection; however, this should be done using .each() rather than $.each().
Iterating over jQuery and non-jQuery Objects
$.each() is an object iterator tool provided by jQuery, as is .each(), a jQuery collection iterator(). These cannot be used simultaneously.
Furthermore, two useful functions called $.map() and .map() may be used to shorten one of our regular iteration use cases.
On a jQuery collection, .each() is used directly. It iterates through the collection, calling a callback on each matched entry. The callback is given the index of the current element in the collection as an argument.
The value (in this example, the DOM element) is also provided, but the callback is invoked within the context of the currently matched element, so this keyword points to the current element, as is required in other jQuery callbacks.
Using jQuery’s .index() Function
.index() is a jQuery object method that searches for a certain element within the jQuery object that it is called on. This method has four different signatures, each with its own set of meanings, which can be difficult. Each signature is supported by index().
This specific function is used in several ways in different functions, which are as follows:
- .index() with No Arguments
- .index() with a String Argument
- .index() with a jQuery Object Argument
- .index() with a DOM Element Argument
Avoiding Conflicts with Other Libraries
The jQuery namespace contains the jQuery library and nearly all of its plugins. As a general rule, global objects are also saved within the jQuery namespace. Thus, there should be no conflict between jQuery and any other library (like prototype.js, MooTools, or YUI).
Having stated that, there is one exception: by default, jQuery abbreviates jQuery with the symbol $. As a result, if you use another JavaScript library that uses the $ variable, you may encounter issues with jQuery.
To avoid these conflicts, set jQuery to no-conflict mode immediately after it is loaded onto the page and before using jQuery on your page.
Conclusion
- In this article, we learned what jQuery cores are, examples of them, and how to pick, alter, and even deal with them in a clear and detailed manner.
- We also learned about the jQuery object, traversing, CSS, styling, dimension, data, and utility functions, and a few special cases.
- You should now have a better understanding of the fundamental concepts and be well on your way to writing your code.