DOM manipulation using jQuery
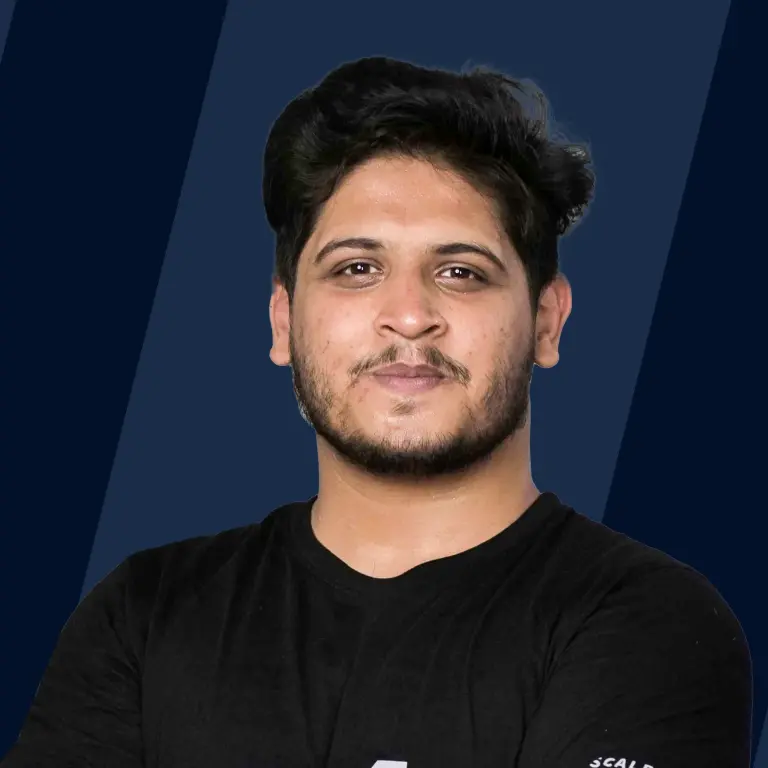
Overview
Manipulation of the DOM is one of the most crucial features of JavaScript and, by extension, jQuery. The technique for representing and interacting with your HTML, XHTML, or XML documents is called DOM, or Document Object Model. Through a programming language, which in the browser is nearly always JavaScript, it enables you to navigate and manipulate your content.
Standard JavaScript can make DOM navigation and manipulation rather difficult. Fortunately, jQuery has a number of DOM-related techniques that make everything much simpler.
Introduction to DOM
The Document Object Model (DOM) is a programming interface for HTML and XML (Extensible Markup Language) documents. It specifies the logical structure of documents as well as how they are accessed and manipulated. DOM is a method of representing a webpage in a structured and systematic order, making it easier for programmers and users to navigate the document.
Using the Document object's commands or methods, we can easily access and manipulate HTML tags, IDs, classes, Attributes, or Elements. DOM provides JavaScript with access to the HTML and CSS of the web page, as well as the ability to add actions to HTML elements. So, in simple terms, the Document Object Model is an API that represents and interacts with HTML or XML documents.
Need of DOM and its properties
The web pages are organized using HTML, and the added functionality is added using Javascript. When an HTML file is loaded into the browser, Javascript cannot directly understand the HTML document. As a result, a corresponding document is created(DOM).
DOM is essentially a representation of the same HTML document in a different format using objects. Javascript easily interprets DOM. For example, Javascript cannot understand tags (<h1></h1>) in an HTML document but can understand object h1 in DOM. Javascript can now use various functions to access each of the objects (h1, p, etc.).
Documents are modeled using objects, and the model includes not only the structure of a document but also its behavior and the objects of which it is composed, similar to how the tag elements with attributes in HTML are modeled.
Properties of DOM
Let's take a look at the document object's properties that can be accessed and modified.
- Window Object: A window object is a browser object that is always at the top of the hierarchy. It is similar to an API in that it is used to set and access all of the browser's properties and methods. The browser generates it automatically.
- Document object: A document object is created when an HTML document is loaded into a window. The 'document' object has several properties that refer to other objects that allow access to and modification of the web page's content. If we need to access any element in an HTML page, we always begin with the 'document' object. The document object is a window object property.
- Form Object: It is defined by form tags.
- Link Object: Link tags are used to describe it.
- Anchor Object: A href tag is used to represent it.
- Form Control Elements: A form may contain a number of control elements, including text fields, buttons, radio buttons, checkboxes, and more.
What is jQuery DOM Manipulation?
To manipulate the content of HTML documents, jQuery provides methods such as attr(), html(), text(), and val() that act as getters and setters. Document Object Model (DOM) -is a World Wide Web Consortium (W3C) standard that allows us to create, modify, or remove elements from HTML or XML documents.
jQuery DOM Manipulation methods
Sl. No. | jQuery Method | Description |
---|---|---|
1 | append() | Inserts content at the end of the element(s) indicated by a selector. |
2 | before() | Inserts content (new or existing DOM elements) before a selector-specified element(s). |
3 | after() | Inserts content (new or existing DOM elements) after a selector-specified element(s). |
4 | prepend() | Insert content at the start of a selector-specified element(s). |
5 | remove() | Removes the element(s) specified by the selector from the DOM. |
6 | replaceAll() | Replace the supplied element with the target element(s). |
7 | wrap() | Wrap an HTML structure around each element defined by the selector. |
Let's look at each of these jQuery DOM manipulation methods in detail.
jQuery after() Method
The jQuery after() method adds content (new or existing DOM elements) after a selector-specified target element(s).
Syntax:
First, specify a selector to obtain the reference to the target element(s) to which you wish to add content, and then call the after() method. As an argument, pass the content string. Any valid HTML element can be used as the content string.
Example:
You can run this code to see the output on any browser. A new <div> with yellow background-color will be added after the <div> with the id #div1.
jQuery before() Method
The jQuery before() method inserts content (new or existing DOM components) before a selector-specified target element(s).
Syntax:
Use a selector to acquire the reference to the target element(s) to which you wish to add the content, and then call the before() method. As an argument, pass the content string, which can be any valid HTML element.
Example:
Run the above code on any web browser to see the output. A new <div> with yellow background-color will be added before the <div> with the id #div1.
jQuery append() Method
The jQuery append() method appends material to the end of a selector-specified target element(s).
Syntax:
To append material to an element(s), first specify a selector expression to acquire the reference, then call the append() method and pass the content string as an argument.
When you run the code on a browser, you will see that the text "World" is added to the end of the <p> tag.
jQuery prepend() Method
The prepend() method of jQuery adds content at the start of an element(s) given by a selector.
Syntax:
To prepend content to an element(s), first specify a selector expression to acquire the reference to the element(s), then call the prepend() method and pass the content string as a parameter.
Example:
You can run the above code on a browser to see the output. You will see that the text "World" is added to the start of the <p> tag.
jQuery remove() Method
The remove() method of jQuery removes the element(s) provided by a selector.
Syntax:
First, supply a selector expression to obtain the reference to an element(s) to be removed from the page, and then invoke the remove() method.
Example:
When you run the code on a broswer, you can see that the <label> element is removed from the web page.
jQuery replaceALL() Method
The replaceAll() method of jQuery replaces all target elements with the given element (s).
Syntax:
The syntax is different here. First, specify a replacement element(s) as a content string, and then use the replaceAll() method with a selector expression to identify a target element(s).
Example:
In the above code, jQuery will replace all paragraph tags with the <span></span> element.
jQuery wrap() Method
Each target element is wrapped with the provided content element by the jQuery wrap() function.
Syntax:
To wrap the target element, specify a selection to acquire the target elements, then call the wrap method and pass the content string(s).
Example:
In the code given above, the span tags are wrapped by the p tags with the help of the wrap() function.
Conclusion
- In this post, we learned about DOM, jQuery DOM manipulation, the definition of jQuery DOM Manipulation, its method kinds, and their methods, along with examples.
- Once you learn these concepts, you will have a basic understanding of jQuery DOM manipulation and will be able to implement it in your web apps.