jQuery Event Handling Overview
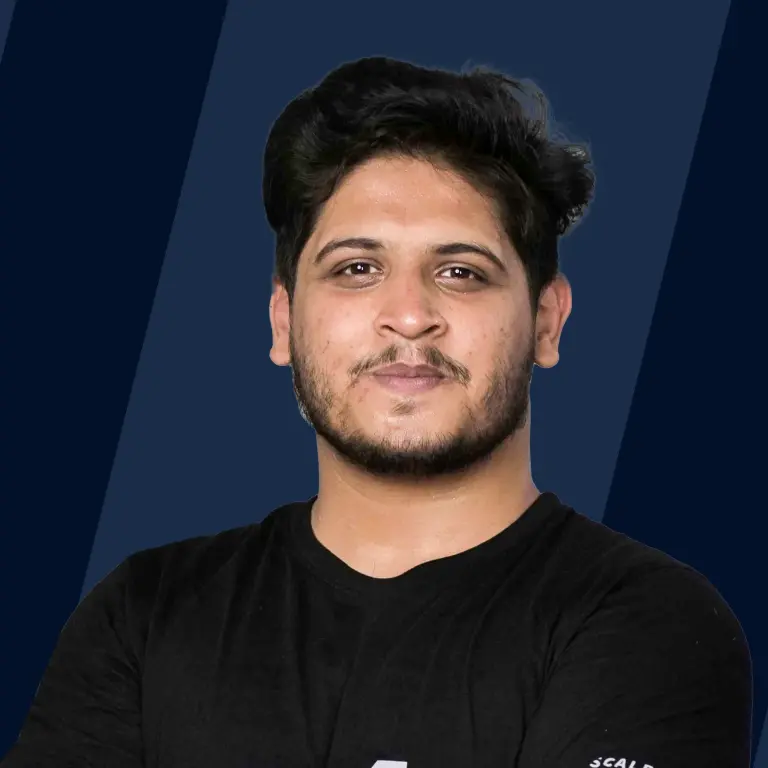
Overview
Any modern web application would be impossible to imagine without an event. The mechanism for creating an interactive web page is events. jQuery is intelligent enough to handle any event that occurs on an HTML page. Let us first attempt to define an event.
Introduction
Let us first understand jQuery. jQuery is a lightweight and quick Javascript library. This library was founded in 2006 by John Resig. jQuery was developed to facilitate HTML DOM tree navigation and manipulation, event handling, CSS animation, and Ajax.
Interaction is the heart of web pages. Events are actions that users take, such as moving their mouse over the website, clicking on items, and typing in text boxes. In addition to these, there are events, such as when the page is loaded, when the video begins or is paused, and so on.
An event is launched when something changes from being static on a webpage, which means that the browser effectively indicates that something has happened. This announcement enables developers to listen to events and respond appropriately.
Let us dive deep into what jQuery event handlers are and what the events are capable of.
What are jQuery Events?
A jQuery Event is the consequence of an action detected by jQuery (JavaScript). When one of these events occurs, you can create a custom function to perform almost anything with it. Event Handlers is the term used to describe these unique functions.
These methods, which include .click(), .focus(), .blur(), .change(), and others, are shorthand for jQuery's .on() method.
The on method is helpful when you want to pass an object containing many events and handlers, when you want to pass data to the event handler, when you are working with custom events, or when you want to tie the same handler function to multiple events.
Examples of Some Common jQuery Events
The following are some instances of common events:
- A single mouse click
- Loading of a website
- Moving the mouse over an element
- Filling out an HTML form
- A keystroke on your keyboard, etc.
Before jumping into the lists of DOM events, let us understand them better. As previously said, there are numerous event types, but user events, such as when someone clicks on an element or writes into a form, are likely the easiest to understand.
These types of events occur on an element, when a user clicks on a button. While user interactions are not the only sorts of DOM events, they are the easiest to grasp when first starting. The Mozilla Developer Network contains a comprehensive list of available DOM events.
The table below lists some of the most important DOM events.
Mouse | Keyboard | Form | Document |
---|---|---|---|
click | keypress | submit | load |
dblclick | keydown | change | resize |
hover | keyup | select | scroll |
mousedown | blur | unload | |
mouseup | focusin | ready |
and much more. The detailed jQuery event methods and references will be covered later.
jQuery Event Binding Syntax
The bind() event in jQuery is used to attach one or more event handlers to items from a set. It defines a function that will be executed when the event happens. It is commonly used in conjunction with other jQuery events. $ is the syntax (selector).
For example, the following syntax is used to associate a click event with any of the DOM elements:
jQuery Event Examples
There are many syntax approaches to jQuery events used for specialized functions such as binding, which are briefly detailed here.
jQuery click() Event
The click() method adds an event handler function to an HTML element. When the user clicks on the HTML element, the function is called.
The following is an example: When a click event occurs on a <p> element, the current <p> element is hidden.
jQuery dblclick() Event
The dblclick() method adds an event handler function to an HTML element.
When the user double-clicks on the HTML element, the function is called:
jQuery mouseenter() Event
The mouseenter() technique associates an HTML element with an event handler function.
When the mouse pointer enters the HTML element, the function is called:
jQuery mouseleave() Event
The event handler function for an HTML element is attached by the mouseleave() method.
When the mouse pointer exits the HTML element, the function is called:
jQuery mousedown() Event
The mousedown() technique connects an HTML element to an event handler code.
When the left, middle, or right mouse button is pressed when the mouse is over an HTML element, the function is executed:
jQuery mouseup() Event
The mouseup() method attaches an event handler function to an HTML element.
When you release the left, center, or right mouse button when the mouse is over an HTML element, the function is called:
jQuery hover() Event
The hover() method combines the mouseenter() and mouseleave() methods into one function.
When the mouse enters the HTML element, the first function is called, and the second function is called when the mouse exits the HTML element:
jQuery focus() Event
An HTML form field's event handler function is attached by the focus() method. When the form field is focused, the following function is called:
jQuery blur() Event
By using the blur() method, you may add an event handler to a field on an HTML form. When the attention shifts away from the form field, the function is called:
jQuery Event Object
Every event handler method receives an event object from jQuery. The event object contains critical properties and methods for cross-browser consistency, such as target, pageX, pageY, relatedTarget, and so on.
The following event properties/attributes are available and safe to access across platforms:
- pageX - For mouse events, defines the event's horizontal coordinate relative to the page origin.
- pageY - For mouse events, defines the event's vertical coordinate relative to the page origin.
- screenX - Specifies the horizontal coordinate of the event relative to the screen origin for mouse events.
- screenY - the vertical coordinate of the event about the screen origin for mouse events.
And much more...
this Keyword in the Event Handler
So, if the event handler is called, the HTML element's color is modified. As a result, you make the most of it.
This refers to the HTML element that is now handling the event, the HTML element that "owns" the copy of doSomething() each time the function is called.
Bubbling and Capturing
Event Bubbling
Event bubbling is a technique for directing an event to its intended target that works as follows:
An event is directed to an object (such as a button) when it is clicked.
If the object has an event handler, the event handler is invoked. The event then bubbles up to the object's parent (much like a bubble in water).
Syntax:
- type: A term used to describe the type of event.
- listener: The function we wish to invoke when the specified type of event happens.
- userCapture: A Boolean value. The event phase is indicated by a Boolean value. By default, useCapture is set to false. It indicates that it is in the bubbling stage.
event.stopPropagation()
The stopPropagation() method prevents the event from bubbling up the DOM tree and notifying any parent handlers of the event. It does not accept any type of argument. To see if this method was ever called, we can use event.isPropagationStopped() (on that event object).
This method also applies to custom events produced by trigger().
Let’s have an example of killing a bubble event with the following code:
event.preventDefault()
The preventDefault() method cancels the event if it is cancelable, which means that the event's default action will not take place.
This is useful, for example, when:
- Clicking on a "Submit" button prevents the form from being submitted.
- When you click on a link, it stops following the URL.
Example:
Event Capturing
Event bubbling will begin with the innermost element and progress to the outermost element. Event Capturing will begin execution from the outermost element to the innermost element.
However, jQuery will make use of event bubbling. We can capture events by using the following example:
The third parameter in the addEventListener function tells the browser whether to use event bubbling or event capturing. It is set to false by default. By default, event bubbling will occur. If this is true, event capturing will occur.
Removing Event Handlers
Unbind() removes event handlers from specified components. When an event happens, this method can delete all or selected event handlers or stop specified functions from running.
Using an event object, this function can also unbind event handlers. This method is used to release an event from within itself (like removing an event handler after the event has been triggered a certain number of times).
Example:
Syntax:
Conclusion
- In this article, we learned what jQuery event handlers are, examples of them, event binding syntax, jQuery event examples with sample core codes to try, jQuery event object, bubbling and capturing, removing event handlers, and so on.
- You should now have a better knowledge of the capabilities of event handlers and be well on your way to building your code.