HTML Attribute Manipulation using jQuery
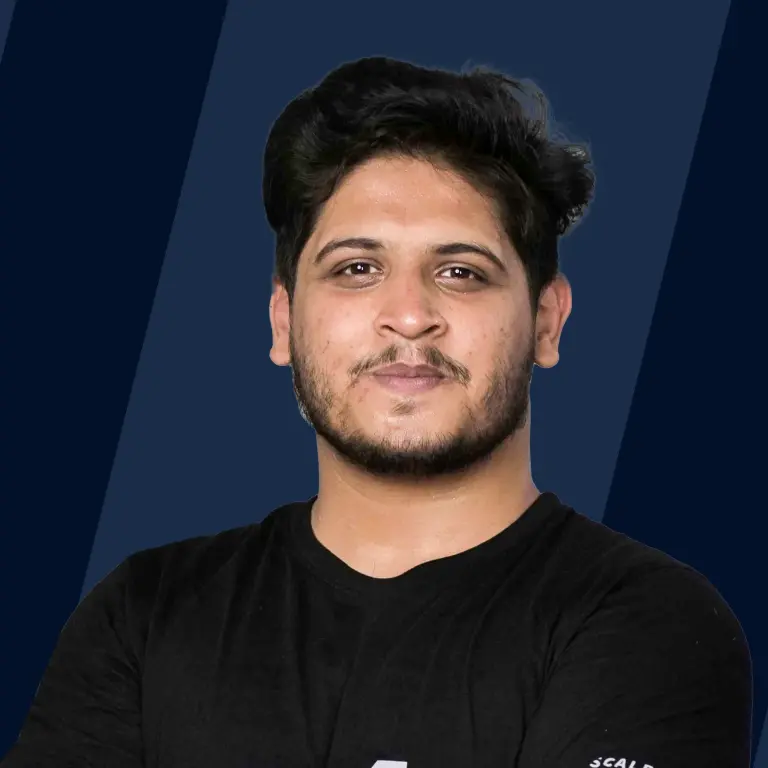
Overview
Let's understand jQuery html attribute manipulation and the methods used to implement it. Three simple but useful jQuery DOM modification methods are:html() - sets or returns the content of chosen elements in HTML format, text() - sets or returns the text content of selected elements in HTML format (including HTML markup) and val() - sets or returns the value of form fields.
About HTML attributes
jQuery is commonly used to manipulate numerous characteristics associated with HTML elements. Every HTML element can have a variety of standard and custom attributes (i.e., properties) that are used to describe the features of the HTML elements.
jQuery allows us to easily manipulate an element's attributes (Get and Set). Let's start the discussion by learning about HTML standards and custom attributes.
HTML Standard Attribute
Some of the more common attributes are −
- className
- tagName
- id
- href
- title
- rel
- src
- style
Example: Consider the following code snippet for HTML markup for an image element:
Syntax:
The tag name in this element's HTML is img, and the markup for id, src, alt, class, and title specifies the element's attributes, which each consist of a name and a value.
Get Standard Attributes
The jQuery attr() method returns the value of any standard attribute from the matched HTML element(s). We'll use jQuery Selectors to find the desired element(s) and then use the attr() method to get the element's attribute value.
If a given selector matches several elements, it returns a list of values that you may iterate over using jQuery Array methods.
Example:
The following jQuery application retrieves the href and title elements of an anchor <a> element.
When you click the button "Get Attributes", you will see the values for the attributes href and title for the <a> tag.
Set Standard Attributes
The jQuery attr(name, value) method is used to set the value of any standard HTML element attribute(s). We'll use jQuery Selectors to find the desired element(s) and then use the attr(key, value) method to set the element's attribute value.
If a given selector matches more than one element, it will set the attribute value for all of the matched elements.
Example:
The following jQuery code will set the title attribute of an anchor <a> element:
When you click the "Set Attribute" button, jQuery will set the attribute for the title attribute and will display the changed value for title.
HTML Custom data-* Attributes (Example)
The HTML specification allows us to include our own custom attributes with DOM elements to convey more information about the element. The names of these properties begin with data-.
Example:
Here's an example of how we used data-copyright, a custom attribute, to provide information about the image's copyright.
Get Data Attributes
The data() method of jQuery is used to retrieve the value of any custom data property from a matched HTML element(s). We'll use jQuery Selectors to find the desired element(s), and then we'll use the data() method to get the element's attribute value.
Example:
The following jQuery program retrieves the author-name and year characteristics of a <div> element:
Set Custom Attributes
To set the value of any custom attribute of the matched HTML element, use the jQuery data(name, value) method(s). We'll use jQuery Selectors to find the desired element(s), and then use the data() method to set the element's attribute value.
If a given selector matches more than one element, it will set the attribute value for all of the matched elements.
Example:
The following jQuery application will set the author-name attribute of a <div> element:
Introduction - Manipulate HTML Attributes using jQuery
The attribute manipulation capabilities of jQuery are broad. The .attr() technique enables for more complicated manipulations in addition to basic adjustments. It can either set an explicit value or use the return value of a function to set a value.
The function syntax takes two arguments: the zero-based index of the element whose attribute is being updated and the current value of the attribute being altered.
Example:
Manipulating a single attribute:
Manipulating multiple attributes:
Using a function to determine an attribute's new value:
jQuery methods to manipulate the HTML element attribute
Sl. No. | jQuery Method | Description |
---|---|---|
1 | attr() | Get or set the value of the target element's specific attribute(s). |
2 | prop() | Get or set the value of the target element's specified property(s). |
3 | html() | Obtain or assign html material to the specified target element(s). |
4 | text() | Text for the specified target element can be retrieved or set(s). |
5 | val() | Get or set the value property of the specified target element. |
Now let's dive deep into each of these jQuery methods to understand how they work.
jQuery attr() Method
The jQuery attr() method is used to get or set the value of a DOM element's given attribute.
Syntax:
To begin, specify a selector to obtain an element's reference and call the attr() function with the attribute name parameter. Pass the value parameter together with the name parameter to set the value of an attribute.
Example:
In the preceding example, $('p').attr('style') retrieves the style attribute of the first <p> element in an HTML page. It does not retrieve all of the style properties of <p> elements.
jQuery prop() Method
The jQuery prop() function returns or sets the value of a DOM element's supplied property(s).
Syntax:
To begin, specify a selection to obtain the reference to an element(s) and call the prop() method. To obtain the value of a property, use the name option. To change the value of a property, use the value and name parameters.
Example:
$('p').prop('style') returns an object in the preceding example. Using object, you may obtain various style characteristics.
jQuery html() Method
The html() function of jQuery returns or sets HTML content to the specified DOM element(s).
Syntax:
To begin, specify a selector to obtain an element's reference, and then call the html() method without supplying any parameters to obtain the inner html content. To change the html content, use the html content string parameter.
Example:
jQuery text() Method
The text() function of jQuery returns or sets the text content of the specified DOM element(s).
Syntax:
To begin, specify a selector to obtain an element's reference, and then invoke the text() method to obtain the textual content of an element. Pass the content string as a parameter to set the text content.
Example:
Please keep in mind that the text() method only returns text content within the element, not the innerHtml.
jQuery val() Method
The val() function of jQuery returns or sets the value attribute of the specified DOM element(s).
Syntax:
To begin, specify a selector to obtain an element's reference, and then invoke the val() method to obtain the value of the value property. Pass the content string as a parameter to set the text content.
Example:
In the preceding example, the val() method returns the value of the value attribute. If an element lacks a value property, the val() function returns null.
Conclusion
- We learned about Attributes, HTML manipulation, the definition of jQuery HTML Attribute Manipulation, its method types, and their methods, as well as examples, in this article.
- You will have a basic understanding of these concepts after going through this article and will be able to write your own code with it.