jQuery more Effects
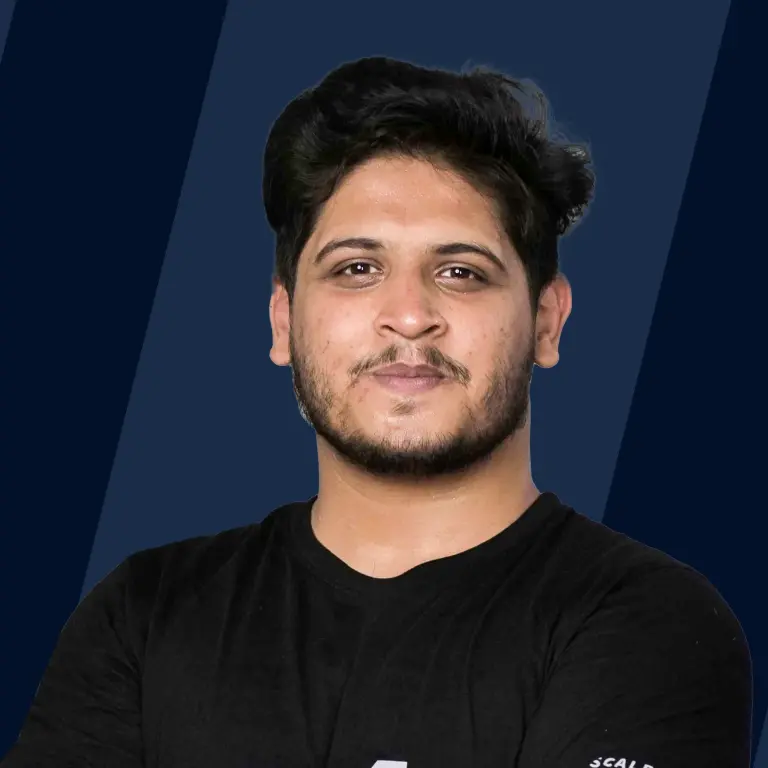
Overview
We can use jQuery to add effects to web pages. We have different types of jQuery effects like fading, sliding, hiding/showing, and animation. There are various methods in jQuery for creating effects on a web page.
Introduction
jQuery effects improve your website's interactivity. jQuery provides a remarkably simple interface for achieving a wide range of spectacular effects such as show, hide, fade-in, fade-out, slide-up, slide-down, toggle, and so on.
We may easily apply commonly used effects using jQuery methods with minimal preparation. This article will go through all the essential jQuery methods used for producing visual effects.
Effects in jQuery
Apart from fading, display, and sliding effects, it has three other types, which are as follows:
- Animate
- Chaining
- Callback Function
animate() method: The jQuery animate() method generates custom animations by modifying a DOM element's CSS numerical values, such as width, height, margin, padding, opacity, top, left, and so on.
jQuery chaining: jQuery method chaining allows us to invoke many jQuery methods on the same element with a single sentence(s). This improves performance because we don't have to parse the entire page every time to find the element when we utilize chaining.
Callback Method: Callback methods are required as JavaScript (jQuery) code execution is asynchronous because jQuery effects may take some time to complete, and the following lines of code may be performed while the effects are still being executed.
To manage asynchronous code execution, jQuery allows you to pass a callback function in all effective methods, and this callback function is only run when the effect is finished.
About jQuery animate() Method
The animate() method of jQuery allows you to create custom animations.
The jQuery animate() method helps to generate custom animations by modifying CSS numerical values of a DOM element, such as width, height, margin, padding, opacity, top, left, and so on.
Syntax
The animate() method of jQuery cannot be used to animate non-numerical properties such as color, background color, etc. You can use the jQuery plugin jQuery.Color to animate such properties.
You may use any jQuery selector to select any DOM element and then animate it with the jQuery animate() method.
Parameters
Here is a list of all the options that offer you complete control over the animation:
- properties - A necessary parameter that defines the CSS properties to be animated; this is the call's only obligatory parameter.
- speed - An optional string specifying one of three predefined speeds ("slow," "normal," or "rapid") or numbering of milliseconds for the animation to run (e.g., 1000).
- callback - An optional parameter representing a function that is to be executed after the animation is finished.
Prerequisites for Animation:
- As part of the effect, the animate() method will not make hidden components visible. For instance, if you call $(selector).hide().animate(height: "20px", 500), the animation will play, but the element will remain hidden.
- To move a DOM element as part of an animation, we must first set its position to relative, fixed, or absolute because all HTML elements have a static position by default and cannot be moved using the animate() method.
Example:
The example below shows how to use the animate() method to move a <div> element to the right until it reaches the left value of 250px. When we click the left mouse button, the same <div> element returns to its original location.
Custom Speed Animation
We can animate different CSS numerical attributes of a DOM element (for example, width, height, or left) at varying speeds.
Example:
Let's rewrite the previous example, this time animating the right movement of <div> with a speed value of 1000 milliseconds and the left movement with a speed parameter of 5000 milliseconds.
Predefined Value Animation
As the value of CSS numeric properties, we can use the strings "show", "hide", and "toggle".
Example:
The following is an example of setting the left property of an element to hide or show using two buttons.
Please keep in mind that using these values for ANY of the numeric CSS attributes will get the same result. For example, if you set an element's width or height to hidden, it will hide the element regardless of its width or height.
Animation with Several Properties
We can use the jQuery animate() method to animate many CSS properties of an element simultaneously.
Example:
The example below shows how to animate various CSS properties of a <div> element. When we press the "Move Right" button, this <div> begins to move to the right until it reaches a left property value of 250px, at which point the element's opacity is reduced to 0.2 and the box's width and height are reduced to 100px.
When we press the "Move Left" button, the box returns to its original location and size.
Animation with Queue Capability
Consider the following scenario: You need to apply various animations, which require you to use the animate() method many times, one after the other. In such cases, jQuery handles these animation requests via a first-in-first-out (FIFO) queue, allowing you to construct fascinating animations depending on your imagination.
Example:
In the following example, we call the animate() method four times to move a <div> in multiple directions one by one.
About jQuery delay() Method
The jQuery delay() method postpones the execution of queued functions. It is the easiest way to create a delay between queued jQuery effects. The jQuery delay() method starts a timer to prevent the next item in the queue from being executed.
Syntax:
- speed - The speed parameter is optional. It specifies the delay's pace. It has three possible values: slow, rapid, and milliseconds.
- queueName - This is an optional parameter as well. It specifies the queue's name. Its default value is "fx", which represents the normal queue effect.
Example:
jQuery delay() example with different values:
Conclusion
- In this article, we will see how jQuery animate and delay methods work, applying methods, and their types and uses in detail.
- Practice and write your own code to make it more understandable for future studies.