jQuery Selector Overview
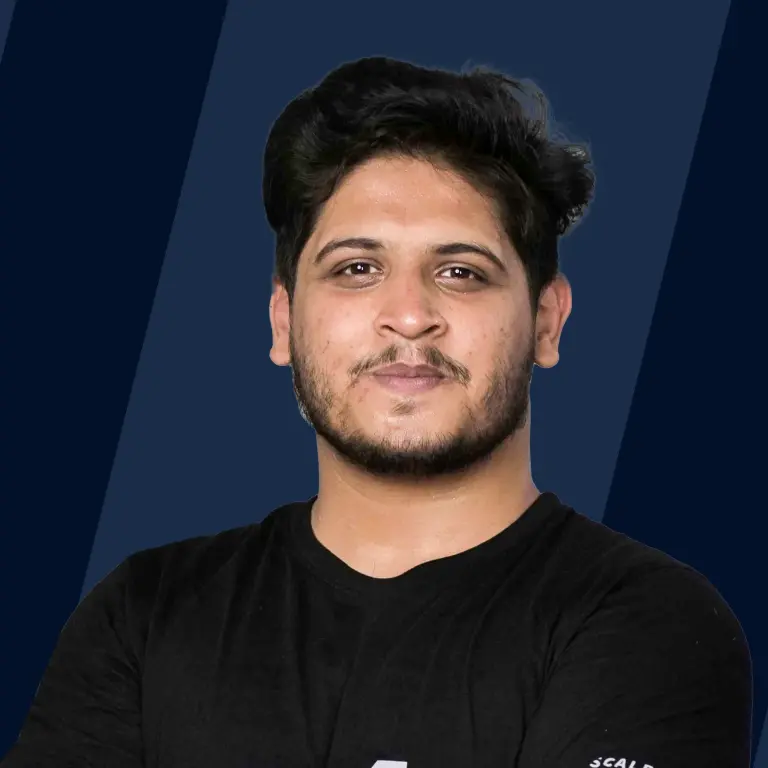
Overview
Web developers often need to locate and edit some content on the web page while creating web applications. jQuery makes it easy for developers with selectors.
There are different types of selectors in jQuery. Let's dive deep into jQuery selectors and see some of the most important types of selectors in jQuery.
Introduction to jQuery Selectors
The jQuery selectors allow us to find and interact with HTML elements. We can use any valid CSS selectors with a jQuery selector.
The JavaScript equivalents of jQuery selector are document.querySelectorAll() and document.querySelector().
jQuery Selector Syntax
The syntax of the jQuery selector is a $ symbol followed by the selector in brackets (). This is known as the factory function.
Here's an example:
This is called a universal selector, and it selects all elements in the HTML document.
The $() Factory Function
We use the factory function to create a new instance of jQuery.
Now let's look at some commonly used jQuery selectors along with the factory function.
Sl. No | Selector | Description |
---|---|---|
1 | Universal selector | It selects all elements in the document. |
2 | Tag selector | It selects elements based on the tag name. |
3 | ID selector | It selects elements with a specific ID in the document. |
4 | Class selector | It selects elements with a specific class in the document. |
5 | Attribute Equals Selector | It selects all elements that have the specified attribute with a given value in the document. |
Let's look at each of these types of selectors.
Universal selector - Select all elements in a document. It should be used with care because it can be extremely slow in some cases.
Example:
Tag selector - Selects all elements based on the tag name.
Example:
Id Selector - This selector is used to fetch an element with a given id. It checks the id attribute of an HTML element. We use a # to represent the id selector.
Example:
Class Selector - The class selector is used to select all HTML elements with a given class. For example, if you want to select all buttons with the class name btn-primary, use $('.btn-primary'). We use a period (.) to represent the class selector.
Attribute Equals Selector - This selector is used to select all elements that have the specified attribute with a given value. An example is, $('[type=text]'). This will select all input tags with their type attribute set to text.
A Simple Example to See the Use of Tag Selector
Let us understand the working of selectors with the help of an example. In this example, we will select all <button> tags and bind a click event handler to display an alert message.
Here is another example that uses the JQuery tag selector:
In this example, we used the jQuery tag selector to select all button elements and add a click event handler that changes the text of the button when clicked.
Here, you might have noticed the usage of the this keyword. It represents the DOM element when we are in a callback function.
How to Use Selectors?
Now, let us learn how to use different jQuery selectors.
Sl. No. | Selector | Example | Description |
---|---|---|---|
1 | Tag Selector | $('p') | Tag selector selects all elements that match with the given tag/element name |
2 | Id Selector | $('#txtname') | Id selector selects a single element that matches with the given id name. |
3 | Class selector | $('.btn-primary') | Class selector selects all elements that match the given class name. |
4 | Attribute Equals Selector | $('[type=text]') | Attribute Equals selector selects all elements having the specified attribute with a given value. |
5 | Universal Selector | $('*') | Universal selector selects all elements available in the document object model(DOM). |
1. Tag Selector
As the name indicates, this selector is used to select all elements based on the tag name. Let's see an example. If you want to select all <h2> tags in an HTML document, here is how we do it:
This code will select all <h2> tags on a web page and set the CSS text-alignment property to center.
2. Id Selector
The Id Selector is used to select an HTML element by its id. The id should be prepended with a # symbol.
3. Class Selector
This selector is used to select all elements on a web page that has the specified CSS class applied. The class name should be prepended with a period.
Here's an example.
Using Multiple Classes
In a real-world application, elements can have multiple classes. If we have to select the elements based on multiple classes instead of just one, use the selector as follows.
Notice that we've used two classes shape and round ('.shape.round'). This means that all elements that have these two CSS classes applied to them should be selected.
To select all elements with any of the specified classes, separate the class names with a comma.
4. Attribute Equals Selector
Attribute Equals Selector can be used to fetch elements from a web page based on an attribute and its value. Normally, we use this selector to select form elements such as input fields, etc.
Let's see an example:
In this example, we first select all input tags from the form with the type attribute set to password from the document. Then we changed the disabled attribute of the selected elements to true so that the fields are disabled.
This selection also allows you to use attributes directly without specifying the tag name. Here's an example.
In this example, the selector is [data-id=1]. This will select all elements with the data-id attribute set to 1.
Instead, if we use button[data-id=1] as the parameter, it will select only the button elements with the same attribute.
5. Universal Selector
The universal selector is used to select all elements on a web page.
Using the universal selector may cause performance issues in some cases.
Let's see an example of using a universal selector:
This code selects all elements on the web page and changes the color to red. If there are several hundreds of elements on the web page, it is not recommended to use this selector.
Conclusion
- In this post, we learned about different selectors in jQuery.
- Selectors are used to finding and interacting with HTML elements on a web page.
- The most commonly used selectors are Tag selector, Id selector, Class selector, Attribute Equals selector, and Universal selector.
- Any valid CSS selectors can be used with the jQuery selector.