Sizzle Selectors
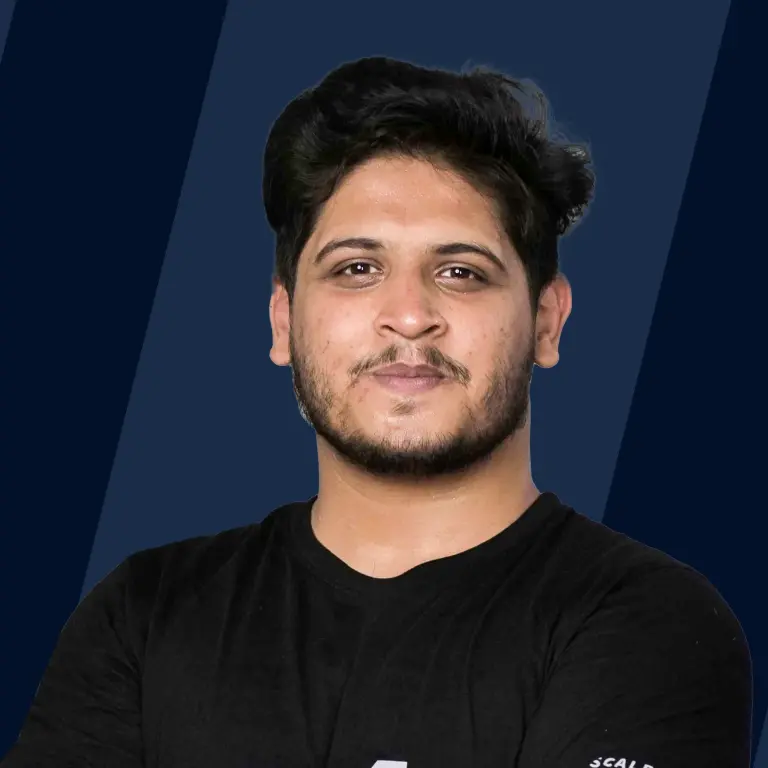
Overview
Almost every active website in the world utilizes jQuery. However, using it without optimization can make the DOM quite slow. Other JavaScript libraries, such as SizzleJS, are similarly affected. To ensure the performance of your DOM, you must adhere to some recommended practices.
Introduction to Sizzle Selector
The motto of jQuery is "choose something and do some operations with it". jQuery developers can provide the selection criteria and then get to work with the outcome. jQuery has a very simple API to choose elements. When picking ids, simply add # as the prefix to the id name. If you want to choose a class, you can use . as the prefix to the class name.
Understanding what happens behind the scenes in jQuery is critical for a variety of reasons. One of the most crucial factors is the rich client's performance. Understanding how jQuery selects items will speed up page loading since various websites are utilizing jQuery code.
Sizzle is a JavaScript selector library that provides sophisticated methods for element selection. You can choose depending on the text included (or not present) within elements, the presence of child elements within a parent, or the absence of those elements.
Attribute selectors and pseudo-class selectors can be used in both stylesheets and the Monetate Element Selector field. Sizzle selectors can only be used in the Element Selector field.
About CSS Specificity
If two or more CSS rules point to the same HTML element, the selector with the highest specificity value wins, and its style declaration is applied to that HTML element.
Consider specificity to be a score or rank that decides which style declaration is eventually applied to an element.
Example:
Another example:
In this example, we've introduced a class selector (called "test") and assigned it the color green. The text is now green (even though we have specified a red color for the element selector "p"). This is due to the class selector being prioritized:
Hierarchy of Specificity
Every CSS selector has a position in the hierarchy of specificity.
A selector's specificity level is defined by four categories:
- Inline styles - For example, <h1 style="color: pink;">
- IDs - For example, #navbar
- Attribute selectors, classes, and pseudo-classes - For example, .test, :hover
- Elements and pseudo-elements - For instance, h1, :before
The following table provides some examples of ways to determine specificity values:
Selector | Specificity Value | Calculation |
---|---|---|
p | 1 | 1 |
p.test | 11 | 1+10 |
p#demo | 101 | 1+100 |
p tag with style="color: pink" attribute | 1000 | 1000 |
#demo | 100 | 100 |
.test | 10 | 10 |
p.test1.test2 | 21 | 1+10+10 |
#navbar p#demo | 201 | 100+1+100 |
* | 0 | 0 (the universal selector is ignored) |
The selector with the highest specificity value will be chosen and implemented.
Example:
A: h1 B: h1#content C: <h1 id="content" style="color: pink;">Heading</h1>
- A has a specificity of 1 (one element selector).
- B has 101 specificity (one ID reference + one element selection).
- C has a specificity of 1000 (inline styling).
In this example, the third rule ( C ) has the highest specificity value (1000).
How jQuery Selects Elements Using Sizzle?
HTML documents consist of several elements or tags. An HTML document structure has the appearance of a tree. In an ideal world, all HTML documents would be 100% legitimate XML documents.
Browsers will forgive you if you forget to close a div. Finally, the browser engine will see a properly structured XML document. The XML is then rendered as a web page on the browser by the browser engine.
DOM elements are XML elements that are used once a page has been rendered. JavaScript is used to alter the DOM elements produced in memory by the browser.
A nice example of tree structure (DOM) manipulation is the command shown below, which hides the header element. jQuery has to get to that particular element to hide the header tag.
Browsers do provide helper functions for navigating to specific types of DOM elements. For instance, you can use the document.getElementById method to get a DOM element with the id name "header".
Now let's look at another example. The following code collects all h1 elements in an HTML page.
Browsers don't provide much assistance if you want something intricate, such as the example given below. Traversing the tree structure was difficult for two reasons:
a) The DOM specification is not so intuitive. b) All browsers did not implement the DOM specification in the same way.
Later, the selection API was released. This specification is supported by the most recent versions of all major browsers, including Internet Explorer 8. But Internet Explorer 7 and 6 do not provide support for it.
The selection API provides the querySelectorAll function and allows developers to write complex jQuery selector queries. For example, document.querySelectorAll("#myId>table>tr>td:nth-of-type(even)").
If you're using Internet Explorer 8 or the latest version of any other modern web browser, the jQuery code jQuery('#header p') won't hit Sizzle. A querySelectorAll call will serve that query.
However, if your browser is Internet Explorer 6 or 7, Sizzle will get called for the jQuery code jQuery('#header p'). This could be the reason why some applications execute substantially slower on Internet Explorer 6/7 than on Internet Explorer 8 because Sizzle's element retrieval is slower than a native browser method.
The Selection Procedure
jQuery can make many things faster on a website with the help of its optimizations. We will see some examples and try to figure out the path jQuery takes. Let's get started.
$('#header')
If the input string is a single word and is trying to find out an id, it invokes a document.getElementById() method and returns the id of an element. It doesn't invoke sizzle.
$('#header a') on a Modern web Browser
jQuery uses the querySelectorAll() method to fulfil a request if the browser supports this method. It doesn't invoke sizzle.
$('.header a[href!="xyz"]') on a Modern Web Browser
In this situation, jQuery will attempt to make use of the querySelectorAll() method, but an error will occur (at least on some browsers like Firefox).
Since the querySelectorAll() function does not accept some selection criteria, the browser will throw an exception. When an exception is thrown by the browser, in this situation, jQuery will forward the request towards Sizzle. It supports CSS-3 selectors much more.
$('.header a') on Internet Explorer 6/7
Because the querySelectorAll() method is not available in IE6/7, this request will be forwarded to Sizzle by jQuery. Now we will take a closer look at how Sizzle handles this situation.
The selection string, which is '.header a', is obtained using Sizzle. It divides the string into 2 parts and puts it in a variable named parts.
The following step distinguishes Sizzle from the rest of the selector engines. Sizzle starts with the outermost selector string rather than checking for elements having the class name "header" first and then continuing down.
In this scenario, Sizzle begins searching for all the a elements in the HTML document. Then, Sizzle calls the find function. Sizzle uses the find function to determine the type of pattern that this string matches with. Sizzle deals with the string a in this situation.
Sizzle then goes over all of the matching definitions one at a time. In this scenario, because a is a legitimate tag element, a match for TAG will be found. Then, the below function will get called.
The result now includes all the a elements.
The next step is to check whether each of these components has a parent element that matches .header. A call to the dirCheck method will be performed to test that. In a nutshell, this is how the call is made:
The dirCheck function returns if each checkSet element passed the test. The method preFilter is then invoked. The key code for this method is as follows:
In the example that we considered, this is what will be checked:
This process gets repeated for each element in the checkSet. Elements that do not meet the criteria will be rejected.
Implications on Performance
We can optimize the selector queries since we have a better knowledge of the working of Sizzle. Let's have a look at two selectors performing the same action as an example.
Because Sizzle works from right to left, in the first scenario, Sizzle will collect all elements that have the class name job and then filter the obtained list.
Sizzle will pick up only the p elements having the class name job in the second case. And, then it filters the obtained list. In the second situation, the selection criterion on the rightmost side is more specific. Hence, it will perform better than the first case.
So if you are using Sizzle, go more specific on the rightmost side and less specific on the left. That will lead to efficient performance.
Let's look at another example:
Since the selection query on the right-hand side is more specific in the second case, it will perform better than the first one.
Features of Sizzle Selectors
The following are the main characteristics of Sizzle:
- It is completely standalone (no dependencies on libraries)
- Most frequently used selectors perform competitively.
- Minified and gzipped to 4KB
- Highly extensible with a simple API
- Designed for maximum efficiency with event delegation
- Easily interpretable IP assignment
Selector Possibilities:
- Supports CSS 3 Selector
- Complete Unicode support
- Supports escaped selectors #id:value
- Contains text
(text) - Multiple
(div,p) - Complex
(a#id) - Has selector
(div) - Not attribute value [name!=value]
- Position selectors - : first, : last, : odd, : even, : lt, : gt, : eq
- Simple Form: Selectors: text, input, checkbox, image, file, password, reset, submit, button
- Header selector: header
- Features of the Code
Conclusion
In this article, we’ve learned about jQuery AJAX Sizzle selectors, how to use them, and their features with examples in detail. You can apply your own code with the knowledge gathered here and do it in your way.