What is jQuery?
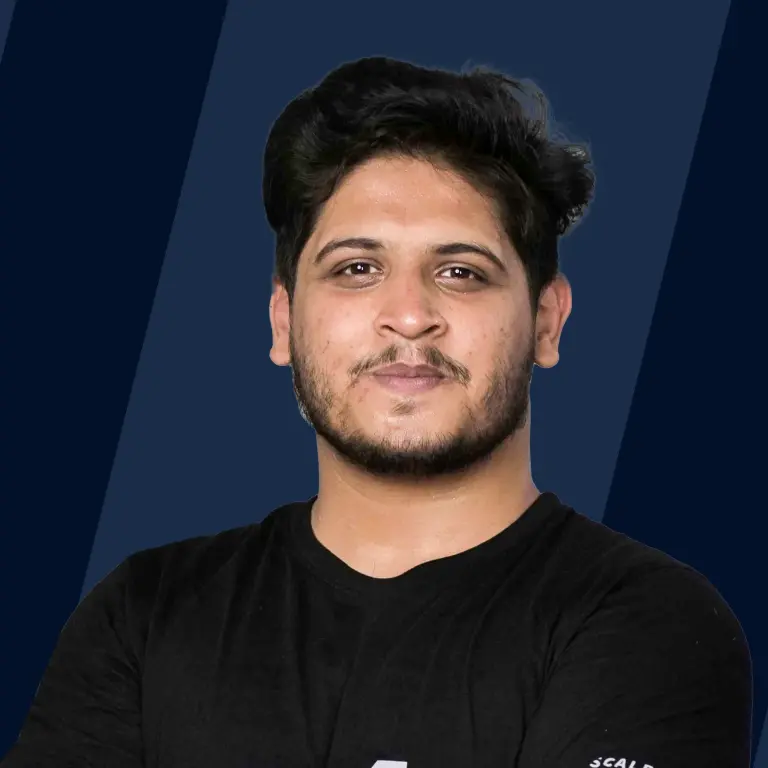
Overview
jQuery is a fast and concise JavaScript library created in 2006 by John Resig with the nice motto: Write less, do more. For rapid web development, jQuery simplifies HTML document traversal, event handling, animation, and Ajax interactions.
What is jQuery?
Let us understand what jQuery is. jQuery is a fast and lightweight Javascript library. John Resig created this library in 2006.
jQuery was created to make HTML DOM tree traversal and manipulation, event handling, CSS animation, and Ajax easier.
jQuery can be used to find a specific HTML element in an HTML document with a specific ID, class, or attribute. We can use jQuery to change one or more of the same element's attributes, such as color, visibility, and so on. jQuery can also be used to make a website more interactive by responding to events such as mouse clicks.
Prerequisites
The following will be necessary before you can start this guide:
- A basic understanding of HTML and CSS.
- An understanding of programming fundamentals. While it is possible to begin writing jQuery without advanced JavaScript knowledge, familiarity with the concepts of variables and data types will be extremely beneficial.
Basic Syntax
The following is the basic syntax for selecting HTML elements and then performing some action on the selected element(s):
Any jQuery statement begins with a dollar sign $, followed by a selector inside the braces (). This syntax $(selector) is sufficient for returning the selected HTML elements. However, if you need to perform any action on the selected element(s), the action() part is required.
The $() factory function is a synonym for the jQuery() function. So, if you're using another JavaScript library and the $ sign conflicts with something else, you can replace it with the jQuery name and use the function jQuery() instead of the $ sign.
Why jQuery?
I hope now you have an idea about the question "what is jQuery". Now let's see why do we use jQuery. jQuery makes it much easier to use JavaScript on your website. jQuery wraps many common tasks that require many lines of JavaScript code into methods that can be called with a single line of code.
jQuery can be used to create Ajax-powered applications. It can be used to simplify, condense, and reuse code. jQuery makes traversing the HTML DOM tree easier. It can also handle events, animate, and add ajax support to web applications.
DOM Traversal and Manipulation
To manipulate the content of HTML documents, jQuery provides methods such as attr(), html(), text(), and val() that act as getters and setters.
Document Object Model (DOM) - is a World Wide Web Consortium (W3C) standard that allows us to create, modify, or remove elements from HTML or XML documents.
Here are some basic operations which you can perform on DOM elements with the help of jQuery standard library methods -
- Extract the content of an element
- Modify an element's content
- Inserting a child element beneath an existing element
- Putting a parent element above an existing one
- Inserting a new element before or after an existing one
- Replace one existing element with another.
- Delete an existing element
- Wrapping content within an element
Event Handling
A jQuery Event is the result of an action that jQuery can detect (JavaScript). When one of these events occurs, you can use a custom function to do whatever you want with it. These custom functions are called Event Handlers.
Consider the following scenario: you want to click a <div> in an HTML document and then perform some action in response to this click. To accomplish this, bind a jQuery click event to the <div> element and then define an action against the click event.
The following is jQuery syntax for binding a click event to all <div> elements in an HTML document:
The following step is to define an action in response to the click event. The syntax for defining a function that will be executed when the click event is fired is as follows. This function is known as the jQuery Event Handler.
AJAX
AJAX is an acronym for Asynchronous JavaScript and XML, and it allows us to load data from the server without having to refresh the browser page.
SYNTAX:
The load() method has a simple syntax -
All of the parameters are described below.
- URL: This is the web address of the server-side resource to which the request is being sent. A CGI, ASP, JSP, or PHP script that generates data dynamically or from a database could be used.
- Data: The data parameter is optional and it specifies a set of query string key-value pairs to send with the request.
- Callback function: After the response data has been loaded into the matched set's elements, a callback function is called. The first parameter passed to this function is the server response text, and the second parameter is the status code.
jQuery Features
jQuery makes various programming tasks easier by requiring less code. Here is a list of the most important core features that jQuery supports.
- DOM manipulation − Using a cross-browser open-source selector engine called Sizzle, jQuery made it simple to select DOM elements, negotiate them, and modify their content.
- Event handling − jQuery provides an elegant way to capture a wide range of events, such as a user clicking on a link, without cluttering the HTML code with event handlers.
- AJAX Support − Using AJAX technology, jQuery can help you create a responsive and feature-rich site.
- Animations − You can use the numerous animation effects that jQuery includes internally in your websites.
- Lightweight − JQuery is a very lightweight library - about 19KB in size (Minified and gzipped).
- Cross-Browser Support − Cross-Browser Support jQuery is cross-browser compatible and performs well in IE 6.0 and later, Firefox 2.0 and later, Safari 3.0 and later, Chrome, and Opera 9.0 and later.
- Latest Technology − JQuery supports CSS3 selectors and basic XPath syntax.
Setting up jQuery
jQuery can be used in two ways:
- You can install the jQuery library on your local machine and include it in your HTML code.
- You can include the jQuery library into your HTML code directly from the Content Delivery Network (CDN).
jQuery - Local Installation
You can install the most recent version of jQuery on your web server and incorporate the downloaded library into your code. For better performance, we recommend downloading the compressed version of the library.
- To get the most recent version, go to https://jquery.com/download/.
- Put the downloaded jquery-3.6.0.min.js file in your website's directory, for example, /jquery/jquery-3.6.0.js.
- Finally, as shown below, include this file in your HTML markup file.
jQuery - CDN-Based Installation
You can directly include the jQuery library into your HTML code from a Content Delivery Network (CDN). Several CDNs offer a direct link to the most recent jQuery library, which you can include in your program.
jQuery - Setup with Typescript
If you're using typings, you can include it like this:
- Install typings npm install typings -g
- Download jquery.d.ts (run this command in the root dir of your project): typings install dt~jquery --global --save
After setting up the definition file, import the alias ($) in the desired TypeScript file to use it as you normally would.
You may need to compile with --allowSyntheticDefaultImports—add "allowSyntheticDefaultImports": true in tsconfig.json.
Create Your Very First jQuery Application
Let's create a hello world program using jQuery. First of all, create an HTML file (index.html), and include the jQuery library in your code.
Here is the code for your very first jQuery application:
The jQuery code above (written inside the <script> tags) means that when DOM elements are ready or fully loaded, execute the jQuery script to dynamically create a message and append it to the HTML tag with the id helloDiv.
Advantages of jQuery
- A diverse set of plug-ins. Developers can use jQuery to build plug-ins on top of the JavaScript library.
- A sizable development community
- jQuery comes with thorough documentation.
- It is far more user-friendly than standard javascript and other javascript libraries.
- jQuery makes it simple for users to create Ajax templates. Ajax allows for a more streamlined interface in which actions can be performed on pages without requiring the entire page to be reloaded.
- Because of its lightweight and powerful chaining capabilities, jQuery is more powerful.
Disadvantages of jQuery
- While jQuery has a large library, depending on how much customization you need on your website, the functionality may be limited, forcing you to use raw javascript in some cases.
- While the JQuery javascript file is relatively small (25-100KB depending on the server), it is still a strain on the client computer and possibly your web server as well if you intend to host the jQuery script on your own web server.
How to Call jQuery Library Functions?
Because almost everything we do with jQuery reads or manipulates the document object model (DOM), we must ensure that we begin adding events and so on as soon as the DOM is ready.
If you want an event to appear on your page, call it from within the $(document).ready() function. Everything contained within it will load as soon as the DOM is loaded and before the page contents.
How to Use Custom Scripts?
It is preferable to put your custom code in the custom JavaScript file: custom.js, as shown below.
Keep this file in the /jquery directory, and then include the custom.js file in your HTML file as follows.
Using Multiple Libraries
- You can use multiple libraries at the same time without them clashing. You can, for example, combine the jQuery and MooTools javascript libraries. For more information, see the jQuery noConflict Method.
- Many JavaScript libraries, including jQuery, use $ as a function or variable name. In the case of jQuery, $ is simply an alias for jQuery, so all functionality is available without using $.
- Run the $.noConflict() method to return control of the $ variable to the library that first implemented it. This allows us to ensure that jQuery does not conflict with other libraries' $ objects.
Here is a simple method for avoiding any conflict:
This technique is especially effective when combined with the .ready() method's ability to alias the jQuery object, as we can use $ within the .ready() without fear of conflict later.
Conclusion
- You learned what is jQuery, how to use jQuery to select and manipulate elements, as well as how events and effects work together to create an interactive web experience for the user.
- You should now have a better understanding of jQuery's capabilities and be well on your way to writing your own code.