JavaScript JSON stringify() Method
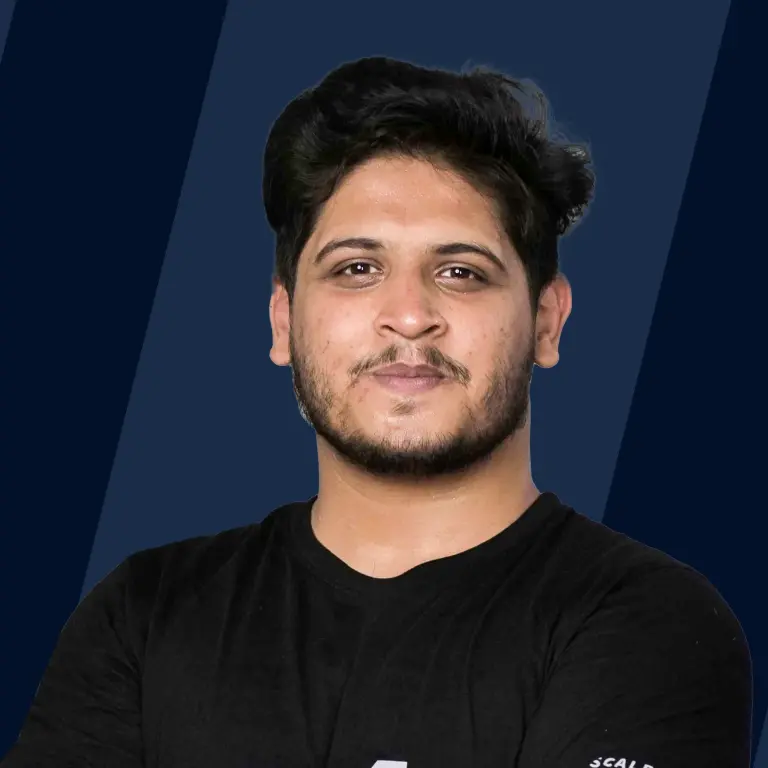
Overview
The JSON stringify() method in Javascript takes in a Javascript variable or object as an input and converts it into an equivalent JSON string. Apart from just this, the method also provides a lot of flexibility in how we can create these strings by using replacer and space arguments.
What is JSON Stringify Function?
If you have ever worked with web servers and web applications, you would know the importance of JavaScript Object Notation or JSON. JSON is widely used for data interchange, especially among web servers, as it is not only universally accepted but also simple and easy to understand by both humans and computers.
But, the data that we usually have on our applications are written in Javascript, mostly in the form of Javascript objects. Converting each object or Javascript value into a JSON string manually is cumbersome. A better method to accomplish the same is by using JSON.stringify() function. This is a simple yet very useful function, which takes any Javascript object or value as an input, and converts it into a JSON string which can then be used for data exchange. The output that we get from this method needs no further modifications and can be sent as it is, to communicate with servers.
Syntax
The syntax to use the JSON stringify method is:
The JSON stringify function call involves three parameters, namely value,repeater, and space. Let us look at each of these parameters in detail.
Parameters
The JSON stringify method has three parameters:
- Value
- Replacer
- Space
Value
The value parameter has to be included in the function call. It signifies the javascript object or value that has to be converted into a JSON string. This is why it is mandatory to include this parameter in the function call.
Replacer
The replacer parameter, unlike the value, is an optional parameter. It is basically used to signify whether any changes are to be made to the Javascript object or value before converting the object/value into the resultant JSON string. We will discuss the details of this parameter in the upcoming sections.
Space
The space parameter is also an optional parameter just like the replacer parameter. As is clear by the namespace, it is used to signify what kind of whitespace characters need to be used in between each key in the resultant JSON string. We will look into this parameter in the upcoming sections in detail.
Return Value
The JSON stringify function returns a JSON string corresponding to the javascript object or value passed in as the value parameter.
Exceptions
Two exceptions to keep in mind while working with the JSON stringify() method is:
- The JSON stringify() method does not support circular object references and will throw a Type Error if we try to stringify an object containing a circular reference.
- A BigInt value cannot be stringified in JSON, and hence will throw a Type Error if we try to stringify an object containing a BigInt value.
Example of JSON Stringify() Method
Let us look at a simple example where we use the JSON stringify method with just the value parameter.
Output:
Explanation :
In this example, we have an object named location. This object is converted into a JSON string using the JSON stringify method. Now, let us discuss the other two parameters in detail.
The Replacer Parameter
Consider that you have your personal data as a javascript object. Now you need to convert this object into a JSON string, but the catch is you don't want to include each key in the JSON string. Instead, you want only some of the keys to be included. Doing this manually would end up taking a lot of time, especially when dealing with many such objects. This is why the JSON to stringify method also provides a second parameter called the replacer.
The replacer argument is used to signify if any changes are to be made to the object before converting the object into a JSON string. To be very precise, the replacer argument refers to which particular keys of the Javascript objects have to be included in the string and which ones should be left out. Now, we can do so in two ways: in the form of an array or in the form of a function.
Replacer as an Array
In the first method where we use an array as the replacer parameter, the array will basically contain a list of the keys of the objects that have to be included in the resultant JSON string. Only these keys of the javascript object which are in the replacer array will be converted into the JSON string. Hence, you can think of a replacer as a sort of selector. When no value is specified for this argument, all the keys of the object are converted into the JSON string. Let us look at an example of using the replacer parameter in the form of an array.
Output:
Explanation:
As you can see in the example above, although the original object personalData also had the occupation key, as it was not mentioned in the replacer array, it was not included in the final JSON string.
Replacer as a Function
Using the array as a list of keys that we want to include in the final string is a great method, but consider a case where your object has a thousand or maybe even more keys, and you need to discard only some of them. Including so many keys manually in an array is cumbersome and time-consuming. This is why we can also use a function instead. This function will basically have some code that will decide which keys are to be included and which should be discarded.
Hence, the way this function will work under the hood is that the input to this function will be each key-value pair of the Javascript object, and then based on the code written inside the function, some keys will be included and others discarded. Let's take a look at the syntax for such a function.
The way JSON stringify method works is if we give it a key-value pair inside an object in which the value is a number, boolean, string, or null, the method will convert all these into the JSON string. But, if the value part of the key is undefined, a function or a symbol won't be included as these values are not supported by the function. So, it will automatically skip these values.
This is what our replacer function will make use of. If we don't want to include a particular key in the final JSON string, we will just return undefined from the function, and hence that key-value pair will be ignored. For all other keys, we will return the corresponding keys so they are included. Let us look at an example.
Output:
But this is not the only way we can use the replacer function. It can also be used to alter some values based on a condition before it is converted to a string or as we know JSON stringify method doesn't include functions or symbol values in its final string, we can first convert them into a string and then pass it to the JSON stringify function, so they are also included.
Let us look at an example.
Output:
Here is a JSON validator to validate your JSON files.
The Space Argument
The third argument is called the space parameter. As we can see in the previous examples, the final JSON string obtained looks very crowded and dense, This is because JSON by default doesn't add any space between consecutive keys. This string is a bit difficult to read and understand. This is where our third parameter comes in.
As its name suggests, the space parameter indicates the amount of space that should be added to each level of the final string. Again, this parameter can also have two types of values: number and string.
Space Parameter as a Number
When the space parameter is defined as a number, it will add the specified number of spaces in between consecutive key-value pairs in the resultant JSON string. But, the maximum number of spaces allowed is 10, while the minimum allowed is 0. So anything greater than 10 is taken as 10 and anything less than1is taken as 0.
Let us look at an example.
Output:
Space Parameter as a String
When defined as a string, the JSON stringify method uses that string in between adjacent keys, instead of space. The thing to note here is that the maximum length of the string acceptable is 10. Any string having a length greater than 10 will be shortened to 10 characters and used.
Let us look at an example.
Output:
toJSON() Behavior
Javascript also provides another feature where you can include a function named toJSON() in your object. When this function has been defined, the JSON stringify() method will stringify the result of this toJSON() function instead of the complete object.
Let us look at an example of the same.
Output:
As you can see, the complete object was not turned into a JSON string, instead only the output of the toJSON() function was stringified.
Points to Remember When Using stringify()
Some points to remember while working with JSON stringify() method are:
- If your object contains Boolean, String, or Number tyes, these are first converted to primitive data types and then stringified.
- If a toJSON() method is defined, then the complete object will not be converted into a JSON string. Instead only the output of the toJSON() method will be stringified.
- Functions, null values, and symbols are omitted when converting an object to a JSON string.
- The numbers Infinity and nan are also considered null values and are hence omitted.
Issues
There are some issues that can occur while working with JSON stringify() method. Let us have a look at both these issues.
The Issue with JSON.stringify() When Serializing Circular References
JSON does not support object references. Hence, if an object reference is passed to the JSON.stringify() method it will raise an exception. This is also true for circular references. Circular references are those in which an object tries to reference itself, either directly or indirectly. Let us look at an example where we try to stringify an object that references itself.
Output:
As you can see, this code gives a Type Error. In case you want to convert objects with circular references, you can use a library that provides the required functionality.
The Issue with Plain JSON.stringify for Use as JavaScript
JSON was not a subset of Javascript initially. This means that some of the functionality available in Javascript was not supported by JSON, especially in strings. For example, the literal code points \u2028 and \u2029 were acceptable in Javascript but not as a JSON string. But, as JSON evolved, this changed and now these are acceptable in both languages.
The issue occurs when you are using an older Javascript engine. In such a case, your code may break if you directly substitute the output of the JSON stringify method into a Javascript string.
The Well-Formed JSON stringify() Specification
As explained, due to compatibility issues, sometimes the JSON stringify method would produce some unwanted errors in encoded strings. This was especially common when the string had lone surrogates, and hence the errors would prevent the string to be encoded in UTF-8 or UTF-16 format.
In an attempt to prevent ill-formed Unicode strings from the JSON stringify method, the well-formed JSON stringify() specification was introduced. This allows stringification of lone surrogates into well-formed JSON strings, which have no problem being encoded in the UTF-8 or the UTF-16 format.
Browser Compatibility
The JSON stringify() method has compatibility with all mostly used browsers.
The only exception is Internet Explorer, where special care should be taken when working with well-formed JSON stringify(), as some browsers are not compatible with it.
Conclusion
- The Javascript JSON stringify() method takes any Javascript object or value as an input and converts the object/value into a JSON string.
- The JSON stringify method has three parameters:
- Value: the javascript object or value that has to be converted into a JSON string.
- Replacer: an array or function which signifies changes to the resultant string.
- Space: a number or string which specifies the number and type of space to be included in between keys.
- The JSON stringify function in Javascript returns a JSON string.
- Exceptions of this method are:
- Throws exception with circular reference objects.
- Throws exception with BigInt.
- The well-formed JSON stringify() specification allows stringification of lone surrogates into well-formed JSON strings.
- This method is compatible with most browsers, but care should be taken with well-formed JSON stringify.