What are Keyboard Events in JavaScript?
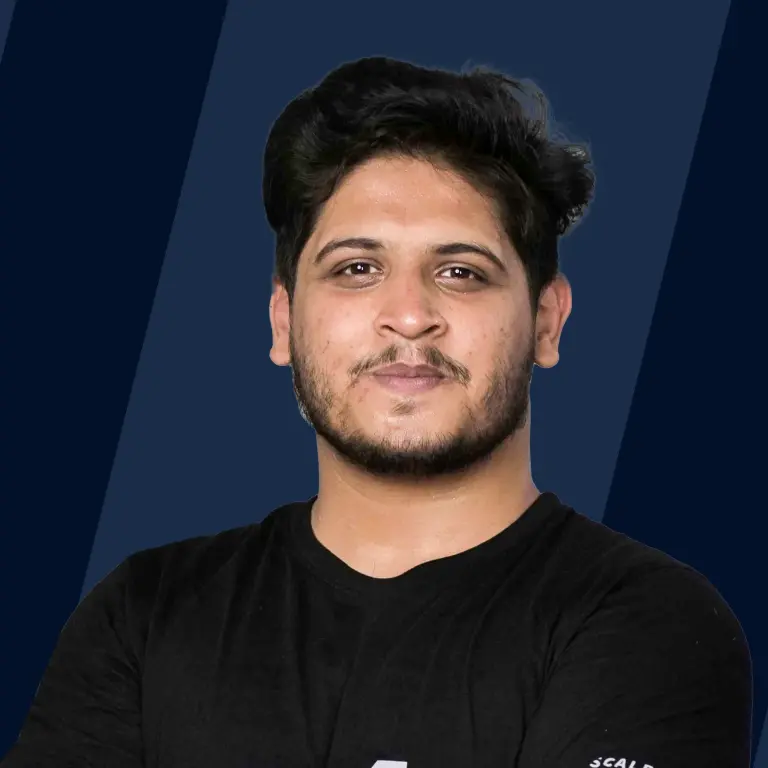
The interaction of the user with the keyboard, triggers various keyboard events.
When a user presses any keyboard key, a keyboard event is triggered. The three main keyboard events in JavaScript are, keydown, keypress, and keyup. The event types such as keydown, keypress, or keyup describe what sort of keyboard activity occurred.
All the keyboard events in JavaScript that are triggered when a user presses a key belongs to the KeyboardEvent Object.
The Keyboard events in javascript just show the interaction between the user and the key of the keyboard, it does not indicate any contextual meaning of that interaction.
Note: Keyboard events are triggered only when the user actually interacts with the key. It may not be triggered when the user is using a different mode for entering the text, like the handwriting system on a graphics tablet.
The KeyboardEvent Object
The Event object in JavaScript is the parent of all event objects like, InputEvent, KeyboardEvent, WheelEvent, ClipboardEvent, MouseEvent, TouchEvent etc.
The user interactions with a keyboard key (or more than one keyboard key along with the modifiers) is described by the KeyboardEvent Object in JavaScript. The KeyboardEvent() constructor is used to create a new KeyboardEvent object in JavaScript
The event objects in JavaScript have two properties i.e. key property, which gives us the character & code property, which gives us the physical key code.
For example, let us assume that the user pressed the D character from the keyboard. Then, event.code will result in KeyD and event.key will result in d (i.e. lowercase)
Key | event.code | event.key |
---|---|---|
D | KeyD | d |
Whereas, if the user pressed the Shift + D combination from the keyboard. Then, event.code will result in KeyD and event.key will result in D (i.e uppercase)
Key | event.code | event.key |
---|---|---|
Shift+D | KeyD | D |
The value of event.key can vary based on different languages, but the event.code value always remains the same.
Types of Main Keyboard Events
The three main keyboard events are as follows:
- keydown: This event is triggered when the user presses a key of the keyboard and it is triggered repeatedly while the user is holding down the key for a long. In simple language keydown means a key has been pressed. To record a keydown event in JavaScript, use the code below:
Alert Output:
- keypress: This event is triggered when the user presses a numeric, punctuation, or alphabetic key from the keyboard not any other key like the right arrow key, home key, etc. Like keydown, the keypress also triggers the event repeatedly while the user is holding down the key. In simple language keypress means that a key with a character value has been pressed. To record a keypress event in JavaScript, use the code below:
Alert Output:
Note: keypress event is highly device-dependent and therefore some browsers no longer support this event.
- keyup: This event is triggered when the user releases a key of the keyboard. In simple languagekeyup means, that a key has been released. To record a keyup event in JavaScript, use the code below:
Alert Output:
In all three code snippets above, we added the addEventListener() method to the document, i.e. we attached the event handler to the window so that it could listen to all the keyboard events.
Event Types
Some events that belong to KeyboardEvent Objects are as follows:
- onkeydown: This event is triggered when the user is pressing a key.
- onkeypress: This event is triggered when the user presses a key.
- onkeyup: This event is triggered when the user releases a key.
Handling Keyboard Events
For handling the keyboard events in JavaScript, the following steps are followed:
- Firstly, we select the element on which we want the keyboard event to be triggered. We can select the element by using various methods like: getDocumentById, getElementsByClassName, querySelector etc.
- Then, we use addEventListener() for registering an event handler to the selected element.
Let us see an example of selecting an input-box with an id name txtValue.
The following code example is an illustration of how to handle keyboard events in JavaScript:
All the three event handlers mentioned above will be called, if we press a character key.
KeyboardEvent Sequence
When the user presses a key on the keyboard, three keyboard events in JavaScript are triggered in the following sequential order:
- keydown
- keypress
- keyup
Let us suppose we have a input-box.
Whenever a user presses a character key , then keydown & keypress events are triggered before any change is made to the input-box. As soon as a change is made to the input-box, the keyup event is triggered. If the user holds down a character key for a long time, then the keydown & keypress are triggered in an auto-repeat manner until the user releases the key.
Whereas, when the user presses a non-character key, only the keydown event is triggered followed by the last event i.e. keyup event which is triggered when the user releases the key. If the user holds down the non-character key for a long time, then the keydown event is triggered in an auto-repeat manner until the user releases the key.
Let us see the following code below to understand the sequence in which the keyboard events are triggered in JavaScript for character keys as well as non-character keys.
JavaScript Code:
CSS styling
Note: Modifier keys like 'Backspace', 'Shift', ‘Ctrl’, ‘CapsLock’ etc. are non-character keys, therefore these keys don't have a ‘keypress’ event attached to them.
The keyboard Event Properties
Following are some of the main keyboard event properties in JavaScript:
- altKey: It returns a boolean value. When the Alt (or Option in macOS) key was pressed when the event was generated it returns true.
Output: (assuming that the user pressed an Alt key)
- ctrlKey: Like altKey it identifies whether the Ctrl key was pressed when the key event was fired or not and returns a boolean value.
Output:
- shiftKey: Like aliKey & ctrlKey it identifies whether the shift key was pressed or not.
Output:
- location: This property return the number giving the location of where the key is present on the keyboard (or any other input device which the user is using).
Output:
- metaKey : This returns a boolean value of whether the meta key was pressed or not. If the meta key was pressed it returns true, else it returns false.
Output:
- which : This property return the Unicode character code of the key that fired the onkeydown or onkeyup event.
Output:
Apart from these properties, there are many more properties as well.
Conclusion
- The interaction of the user with the keyboard keys triggers the keyboard events in Javascript.
- The three main keyboard events in JavaScript are, keydown, keypress, and keyup
- When the user presses a character key, the keydown, keypress, and keyup events are triggered in a sequential manner. While, if the user presses a non-character key, then only the keydown and keyup events are triggered.
- The keyboard event object has two main properties: the key property which returns the value of the key pressed and the code property returns the physical code of the key pressed.