How to Find the Length of an Array in C?
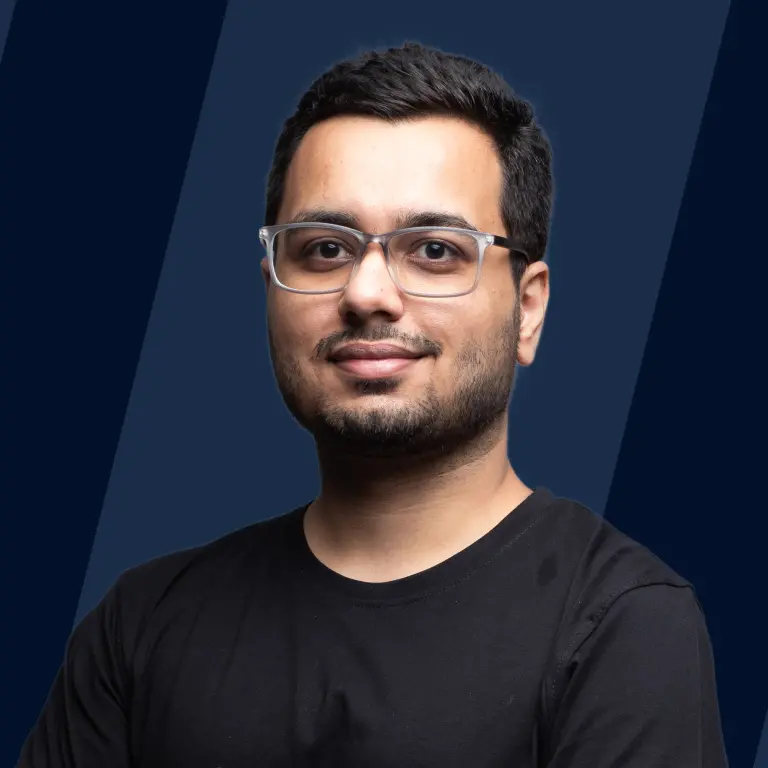
Overview
We require the length of arrays in different operations like iterating over the array, copying the array, and many more, so here in this article, we will look at how to find length of array using different methods.
Arrays have a fixed length that is specified in the declaration of the array. In C, there is no built-in method to get the size of an array. A little effort is required to get the length of the array by utilizing the in-built methods or pointers in C. It is possible to find the length of an array in multiple ways.
In this tutorial, we will consider two methods :
- Using sizeof() operator.
- Using pointer arithmetic.
Programming Logic to Calculate the Length of an Array in C
- Determine the size of an array using the sizeof operator, e.g. sizeof(arr).
- Determine the size of a datatype value using the sizeof(datatype).
- To find the array's length (how many elements there are), divide the total array size by the size of one datatype that you are using.
So, the logic will look like this :
Length of Array = size of array/size of 1 datatype that you are using to define an array.
The logic is elaborated further programmatically in the below section [Using sizeof()].
1. Using sizeof()
Let me ask you a question. Can you specify the length of the array given below?
After taking some time, you may answer this question but, if you get an array size of the array given above, it will be difficult and time-consuming but, the question is why will you count the elements manually when we have a sizeof() operator in C language.
So, let me introduce you to how to use the sizeof() operator to count Array elements.
The sizeof() operator in C calculates the size of passed variables or datatype in bytes. We cannot calculate the size of the array directly using sizeof(), we will use the programming logic defined above to find the length.
Syntax to calculate array length using sizeof() :
In the above syntax :
- arr_size : variable to store the size of an array.
- sizeof(name_of_array) : simple name given to the array we are calculating the size of.
- sizeof(name_of_array[index]) : size of one element of array.
Now let's understand why this formula works to calculate the length of an array :
To calculate the size of the whole array we multiply the size of the 1 array element into several array elements. Therefore to calculate length of array we divide size of whole array i.e sizeof(name_of_array) by size of one array element i.e sizeof(name_of_array[index]). Hence we get the length of that array.
C Program to Calculate the Length of Integer Array Using sizeof()
Now, let's have a look at a C program that calculates the length of an integer array using the sizeof() operator.
C Program :
Output :
C Program to Calculate the Length of char Array Using sizeof()
Now, let's have a look at a C program that calculates the length of a char array using the sizeof() operator.
C Program :
Output :
2. Using Pointer Arithmetic
Calculating the length of an array in C using pointer arithmetic as a hack. The solution is short as compared to the sizeof() operator.
The following expression is used to calculate the length of an array :
- size_variable : used to store the size of an array.
- &array_name : returns a pointer
- (&array_name + 1) = address of elements ahead.
- (&array_name + 1) - array_name = difference between address of elements ahead and array gives the length of array.
Let's take some C programs to understand this better :
C Program to Calculate the Length of int Array Using Pointer Arithmetic
C Program :
Output :
C Program to Calculate the Length of char Array Using Pointer Arithmetic
C Program :
Output :
Conclusion
So, let's summarize what we have learned in this tutorial :
- There is no such built-in method to calculate the length of an array in C, we have to calculate it using expressions.
- There are two ways by which we can create an expression and calculate the length of an array in C.
- Using the sizeof() operator and using pointer arithmetic.
- The sizeof() operator in C calculates the size of passed variables or datatype in bytes. Therefore to find the array’s length, divide the total array size by the size of one datatype that you are using.
- The Pointer arithmetic is a hack where the difference between the address of elements ahead and the array gives the length of an array.