List in Data Structure
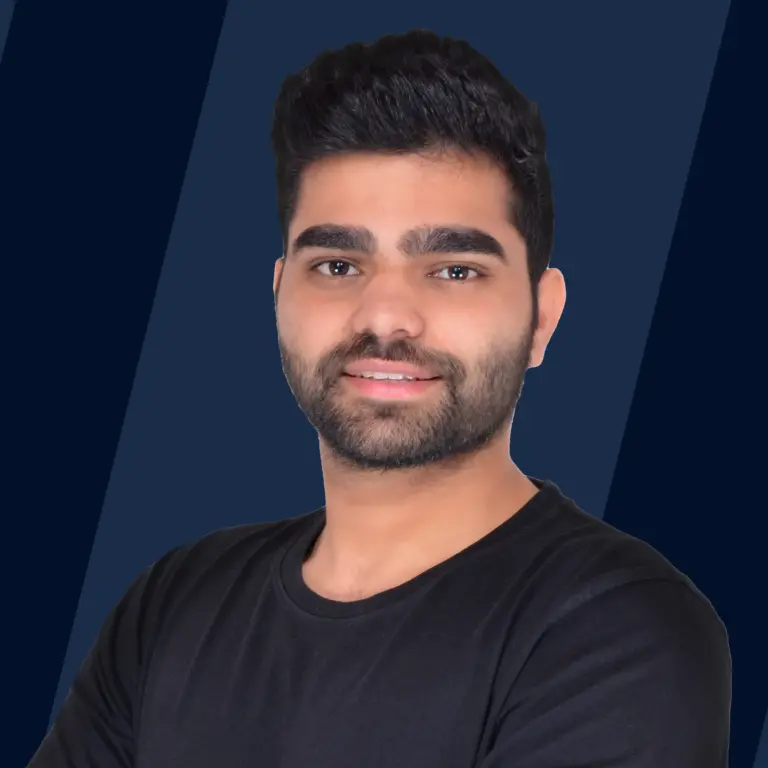
Overview
List is a data structure that stores elements in an ordered and sequential manner. A list can store repetitive elements which means a single element can occur more than once in a list.
Takeaways
Subsetting is accessing the part of a list from any start index to the end index.
What is a List?
List is an ordered data structure that is used to store different or same elements in a sequential manner.
The list is similar to array in data structure but in the array, we can store only the elements which are of same data-type whereas in the list in the data structure, with some of the programming languages like python and javascript we can store elements with different data types also.
Let's see an example for array and list to know the difference better.
Javascript
C++
As seen above in the example, list1 in javascript is storing an integer, boolean as well as string in its list data structure.
Whereas the array in C++ above is storing only integer elements.
There is no need to define static size to the list i.e we can add more elements to the list dynamically and as the new elements get added to the list, the size of the list increases dynamically.
Sub-setting
Subsetting is extracting a part of the list. We can access the list elements through the indexes.
Let's first see an example of accessing an element of the list in python.
The above list named list 2 contains strings, integers, and boolean values.
Each element is present at a particular index in the list as shown in the above list (list2). These indexes are used to access the elements of the list.
Suppose we want to access element 65 from the list then it can be accessed by: list2[2]
Similarly, list2[0] = "Scaler"
list[1] = 45
list[3] = true
Index in a list data structure starts from 0. Now, if we want to access any part of the list together then we use the subsetting concept.
For example, if we want to access [45,65, true] from the list2 then we can do that by list2[1:4] in python.
list2[1:4] - It starts picking the element in list2 from index 1 and stops at index just before 4 i.e 3. The synatx for subsetting in python is :
The syntax given above starts picking the elements from start_index and goes till end_index.
We can also use negative index to get the last element of the list directly in python.
So, list2[-1] = true
Operation on List Data Structure
There are various operations that can be performed on the list data structure. some of the basic operations that are mostly performed on list data structure are :
- Add or insert operation - We can add more elements to the list. The elements added can be of any data type in the case of python and javascript. Operations such as list. append() are used in python to insert elements to the list. Also, many languages use list.push() operation to insert new elements to the list.
- Replace or reassign operation - using replace and reassign operations we can replace an already existing element in the list with another element. we make use of the index of the list elements to replace or reassign any element.
- Delete or remove operations - By delete and remove operation we can easily delete or remove any list elements. list. pop(), list. pop(index) are some of the operations used in python and other languages to remove an element from that particular index or the end of the list.
- Find or lookup or search operations - If we want to search and fetch any element present in the list then we can use find and lookup or search operations on the list.
Usage of the List Data Structure in Different Programming Languages
the list data structure is used a lot in real-life applications where data need to be stored in a sequential and ordered manner.
We can implement CRUD - Create, Read, Update and delete operations on the list data structure in different programming languages.
CRUD Operation on the List in Python :
CRUD Operation on List in Java :
CRUD Operation on List in C++ :
CRUD operation on List in Javascript :
Conclusion
- list in the data structure is an ordered data structure that stores elements sequentially and can be accessed by the index of the elements.
- list in the data structure can store different or same data types elements depending on the type of programming language that is being used.
- we can perform add/insert, delete/remove, update and search/lookup operations on list in data structure.