Convert List to Array in Java
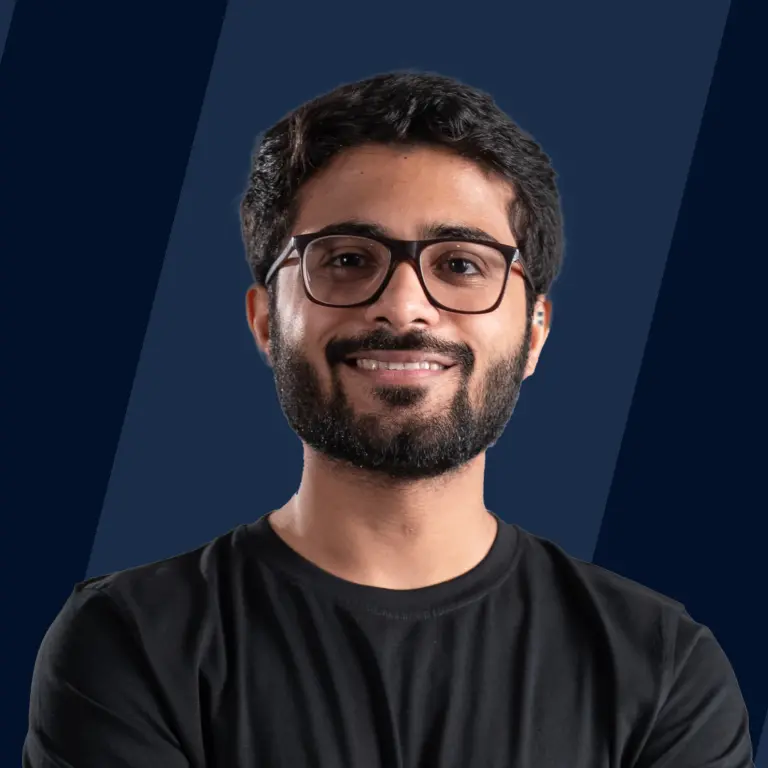
Introduction
The ArrayList class, which is part of the java.util package, is a resizable array. The distinction between a built-in array and an ArrayList in Java is that the size of built-in arrays cannot be changed. An ArrayList, on the other hand, can have elements added and removed at any time.
Syntax:
There can be different ways to convert a list to an array in Java. Let's discuss each of them one by one.
To convert list to array in java is an extremely fundamental part of programming. Sometimes, while writing code, a function might only accept an array as a parameter. In addition, there are a few advantages that Arrays have over ArrayLists.
- ArrayLists typically pre-allocate around double the memory one actually requires so that it can append objects quickly.
- Hence, if one never adds any more items, it results in a waste of space.
Difference Between List and Array in Java
Point of Comparison | Array | List |
---|---|---|
Size | Static in size. Must specify size while initializing. | Dynamic in size. Need not specify size while initializing. |
Performance | Have a higher performance because of their fixed size. | Lower performance due to additional memory allocation/deallocation operations. |
Storage Capability | Can store both objects and primitive types. | Can store just objects. |
Capacity | To find out the capacity, use length() method | To find out the capacity, use size() method |
Add elements | Use assignment operator | Use add() method |
Ways to Convert List to Array in Java
ArrayList to Array in Java is done using the toArray() method of the List Interface as described below.
1. toArray() method
The toArray() method is a popular method used to convert list to array in java. It returns an array containing all of the ArrayList’s elements.
If the order in which this collection’s elements are returned by its iterator is guaranteed, this function must return the elements in the same order.
The toArray() method returns an Object array (Object[]). Before utilising it as an Integer object, we must first typecast it to Integer. We receive a compilation error if we don’t typecast.
Syntax 2.1:
2. toArray(T[] arr)
In this method, an array that has already been defined is passed as a parameter. When the array’s size is higher than or equal to the list’s size, the list’s elements are added to the array. Else, a new array will be constructed and filled.
Casting is unnecessary because the type of the returned array is given by the parameter’s type.
Syntax 2.2:
Algorithm
To convert a list to array in java, follow the following steps:
START
- If list not declared yet, declare and define the same
- If array defined, go to step 5, else, continue
- Use Syntax 2.1
- Print the array using a for loop and typecast the value before printing it. Break.
- Use Syntax 2.2
- If array’s size is higher than or equal to list’s size, list added to array, else new array formed and filled
- Print the array using a for loop.
END
Example Program
Now that we have seen the algorithm to convert list to array in java, let us implement the same:
Output:
Conclusion
- List to Array conversion can be performed using any one of the following methods:
- toArray() method
- toArray(T[] arr) method
- The toArray() method returns an array containing all of the ArrayList’s elements. All elements of the returned object are instances of Object class and hence need to be typecasted to a suitable data type.
- The toArray(T[] arr) method does not return anything. It performs the modifications in the array passed as argument.