What is the main() Function in C?
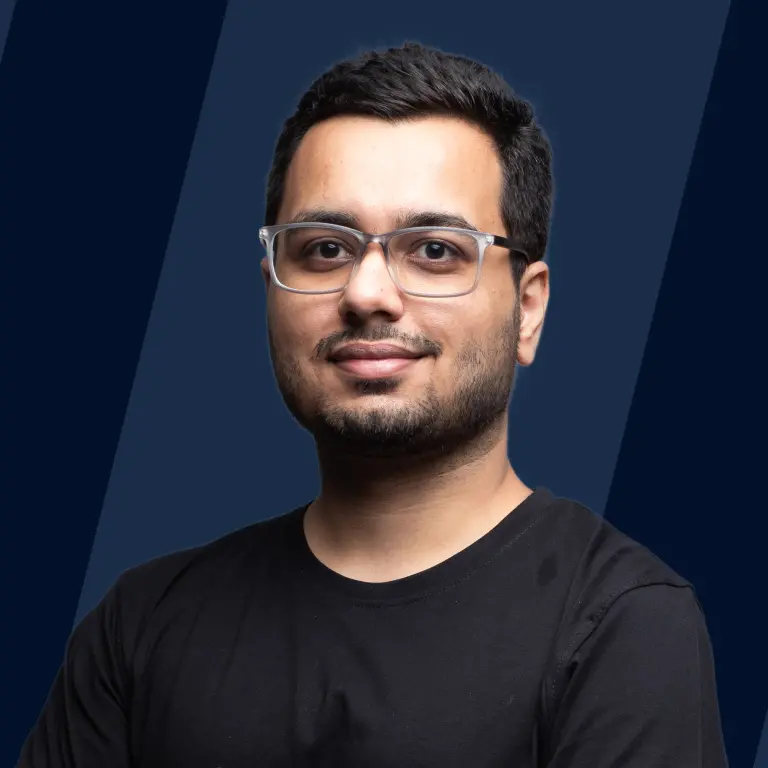
Introduction
Usually, a program starts with the main function in almost every programming language. The main function in C is the starting point of program execution.
Like other programming languages like C++, Java, etc., the main program in C is a critical function that marks the beginning of the program execution. Since C Language is a sequentially executed procedural language, the statement or instructions written in the main function in C are executed sequentially in the order it is written. In simple terms, the main function in C is the entry point of any C program.
The main function can call various other functions. Initially, the operating system controls the main function in C. When the main function in C calls the function, it passes the control to the called function for its execution. After the entire execution of the calling function is completed, the function returns the control to the main function. The program is completed once all the statements inside the main function in C (whether function call or other statements) are executed.
Refer to the image below to see the basic prototype of the main function.
Importance of the main() Function
Since we now know the basic function of the main function in C, let us learn some of its importance.
- The main function in C is called by the Operating System at the run time, not the compile time.
- The main function in C marks the beginning of any program in C.
- The main function in C is the first function to be executed by the Operating System.
- The main function in C starts with an opening bracket ({) and ends with a closing bracket(}). It can return an int or void data type.
- The Operating System hands over the control to the main function for the execution of other functions.
- The main function in C is user-defined; hence, we can also pass parameters to the main function.
Note: The main is a predefined keyword in the C language, so we cannot change its name and meaning.
Refer to the next section to see the syntax and examples of the main function in C.
Syntax of main() Function in C
The syntax of the main function is straightforward. There can be two types of main function prototypes: the first without any arguments and the second with arguments.
Let us see both the prototype syntaxes:
Main function in C without any argument
In the syntax above, the return type is taken as int to check if the program has been executed successfully or not. So, if the returned value from the main function in C is , then the program has been executed successfully. Else, there is some error. We put the return 0; statement as the last statement to mark the ending of the main function in C and to check whether the program has been executed successfully.
main function in C without any argument
In the syntax above, the return type is int, and the reason for that has been discussed in the above paragraph. This main function prototype has arguments: int argc, char *argv[]. Here, argc is an integer-type nonnegative value representing the number of arguments to be passed to the main function from the console. On the other hand, the argv[] is the pointer to the first element of the arguments array. The argv[] array contains all the arguments in an array. The arguments array is sized (argc + 1) and contains a null value at the end.
Note: When a return statement is used in the main function in C, the return value is passed as the argument to the implicit call to the exit() function. The value returned by the exit function tells the operating system whether the program has been executed successfully or not.
For more explanation and examples, refer to the section: Types of the main() function.
Program to Print a Statement Using main() Function
Let us take a simple example to understand the working of the main function in the C language.
Output:
In the example above, we have called the predefined printf() function, which prints the data inside it.
Program to Call Nested Function Using main() Function
Let us take a more complex example in which the main function is calling a function that itself calls another function. This example depicts the flow of the main function and various other subsequent function calls in the C language.
Output:
Types of the main() Function
There can be five types of main function prototypes. Let us learn about them in detail with syntax and examples.
a. void main()
A void keyword refers to an empty data type. To depict that the main function in c is not returning any value, we can use the void keyword as the return type of the function.
Let us first see the syntax and then the example of this prototype.
Syntax:
Example:
Output:
b. int main()
An int keyword references an integer data type. We can use the int keyword as the return type of the main function in C to depict that the function is returning an integer value. If the returned value from the main function in C is , then the program has been executed successfully. Otherwise, there is some error.
Let us first see the syntax and then the example of this prototype.
Syntax:
Example:
Output:
c. int main(int argc, char **argv)
As seen above, the int keyword indicates that the function returns an integer value. When running the function, we can also pass the arguments argc and argv from the command line (as command-line arguments).
Here, argc is an integer-type nonnegative value representing the number of arguments to be passed to the main function from the console. On the other hand, the argv[] is the pointer to the first element of the arguments array. The argv[] array contains all the arguments in an array. The arguments array has a size of (argc + 1) and contains a null value at the end.
Let us first see the syntax and then the example of this prototype.
Syntax:
Example:
Output:
d. int main(void)
Here, the int keywords tell that the program will return an integer value and take noting as an argument; hence, it uses void.
Let us first see the syntax and then the example of this prototype.
Syntax:
Example:
Output:
Note: The int main(void) and int main() are similar as both return an integer. The difference is that we can pass more than one argument to the int main() function, whereas for the int main(void), we can not pass any argument.
e. void main(void)
The void main(void) function neither takes an argument nor returns a value.
Let us first see the syntax and then the example of this prototype.
Syntax:
Example:
Output:
Conclusion
- The main program in c is a compulsory function that marks the beginning of the program execution. The instructions for the main function in C are executed sequentially in the order they are written.
- The main function can call various other functions. Initially, the operating system controls the main function in C.
- The operating system calls the main function C at the run time, not the compile time.
- The main function in C starts with an opening bracket ({) and ends with a closing bracket(}). It can return an int or void data type.
- The main function in C is user-defined; hence, we can also pass parameters to the main function.
- In the case of an int return type, if the returned value from the main function in C is , then the program has executed successfully; else, there is some error.