malloc() Function in C
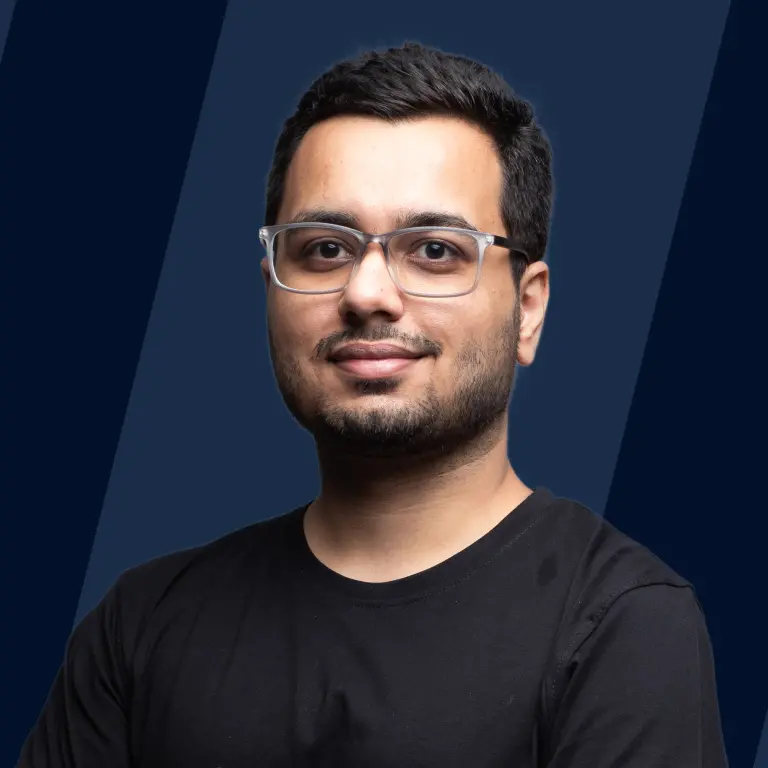
Overview
There are two types of memory in C, one is static memory and another is dynamic memory. To allocate the dynamic memory one method is in the built-in C programming language method malloc(). malloc() function allocates particular size bytes of memory and returns the pointer pointing towards the allocated memory. The malloc() in C returns a void type pointer, which means we can assign the dynamic memory for any data type (primitive or user-defined). If the memory space of required bytes is unavailable, it will return a NULL pointer. malloc in C is defined in the <stdlib.h> header file which means to use the malloc() function we must have to include this header file in the code.
Syntax of malloc() Function in C
Malloc function in C only creates a single memory block at a time and the allocated memory address contains the garbage value initially. Syntax of malloc in C function is:
To allocate a memory block for the variable of type data_type syntax of malloc in C looks like:
Here, sizeof() function will return the size of the data_type in bytes and malloc will allocate this many bytes in memory. Also, caste_type should be same as data_type.
Parameters of malloc() Function in C
The Malloc function in C only takes a single parameter which represents the number of bytes of memory malloc going to allocate. Usually, the sizeof() function is used to get the number of bytes required because the size of every data type may vary on different compilers.
Return Value of malloc() Function in C
The return value or return type of the malloc() in C is a void pointer that points toward the memory allocated by the malloc function in C. Return type as a void pointer provides the advantage that we can assign a void pointer to any data type pointer. malloc() function in C may return the NULL value as the return value. When the empty or free memory of the device is less than the requested memory for the malloc() function then malloc in C will return NULL.
Note:
Initially, the memory allocated by the malloc() function in C contains the garbage value.
Example
In this example, we will simply allocate the memory to an integer variable using the malloc() function. Code:
Output:
Explanation: In the above code, we included the <stdlib.h> header file to use the malloc() function of C. Then we allocated a memory block of the size of an integer variable and stored the location in the var pointer. Printed the garbage value of the address pointed by var, then assigned some value to it and printed in the last.
Note:
- Types of memory in C: There are two types of memory in the C programming language static memory and dynamic memory. When a programmer declares the variable for the primitive or user-defined data type then the memory allocated to them is static memory or stack memory. The memory which is allocated to the variables using malloc() or calloc() functions in the C programming language comes under the category of dynamic memory or heap memory.
- Memory Leak problem: Static memory is automatically allocated by the compiler when the variable is declared and when the variable goes out of scope then it is automatically deallocated by the compiler but dynamic memory is not deallocated automatically or when the variable goes out of scope. For this either memory should be free using the free() operator otherwise it will remain there until the program runs which leads to a memory leak. A Memory leak has a disadvantage as the memory which is not in use is still inaccessible. It may take the whole memory of the device and the program terminates due to lack of memory. To learn more about memory allocation, malloc(), calloc(), and free() functions in C please refer to the article Dynamic Memory Allocation in C.
More Examples
- In the last example, we haven't used the free() function to deallocate the memory. Now in this function, we will use the free() function for the deallocation of the memory.
Code:
Explanation:
In the above code, we allocated the memory to the var pointer using the malloc in the C function. After that, we deallocated the memory by using the free() function, and after that var pointer, will become the Dangling pointer.
To learn about the dangling pointer please refer to the article Dangling Pointer in C.
- Let's take an example where we will request 10 power 17 Bytes of memory, which is not possible and the malloc function will return the NULL pointer.
Code:
Output:
Explanation: In the above code, we demanded a large amount of memory which was not free to allocate, and the NULL is returned by the compiler.
Conclusion
- To allocate the dynamic memory one method is in the built-in C programming language method malloc() which is defined in the <stdlib.h> header file.
- malloc() function allocates particular size bytes of memory and returns the pointer pointing towards the allocated memory.
- When the empty or free memory of the device is less than the requested memory for the malloc() function then malloc in C will return NULL.
- There are two types of memory in the C programming language static memory or stack memory and dynamic memory or heap memory.
- Memory allocated by the malloc in C doesn’t deallocate when the variable goes out of scope, it deallocates when the program ends or uses the free() function.
- A memory leak is a disadvantage as the memory is not in use and is also not free to use. It may take the whole memory of the device and the program terminates due to lack of memory.